C++primer plus 第十四章习题答案
2018-03-30 10:27
260 查看
习题一:
Wine.h#ifndef WINE_H_
#define WINE_H_
#include<string>
#include<valarray>
using namespace std;
template<typename T1,typename T2>//声明一个Pair类
class Pair
{
private:
T1 year;
T2 bottles;
public:
Pair(const T1&s,const T2&m):year(s),bottles(m){}
Pair(){}
int Sum()const;
void Set(const T1&s1, const T2&s2);//T1、T2具体化时为数组
void Show(int y)const;
};
template<typename T1, typename T2>
int Pair<T1,T2>::Sum()const
{
return bottles.sum();
}
template<typename T1, typename T2>
void Pair<T1, T2>::Set(const T1&s1, const T2&s2)
{
year = s1;
bottles = s2;
}
template<typename T1,typename T2>
void Pair<T1, T2>::Show(int y)const
{
for (int i = 0; i < y; i++)
cout << year[i] << '\t' << bottles[i] << endl;
}
typedef valarray<int> Arrayint;
typedef Pair<Arrayint, Arrayint>Pairarray;
class Wine
{
private:
Pairarray yb;
string fullname;
int yer;
public:
Wine();
Wine(const int yr[], const int bot[], const char*l, int y);
Wine(const char*l, int y);
void Getbottles();
string& Label();
int sum() const;
void Show()const;
};
#endifWine.cpp#include<iostream>
#include"wine.h"
Wine::Wine(const int yr[], const int bot[], const char*l, int y)
{
fullname = l;
yer = y;
yb.Set(Arrayint(yr, yer), Arrayint(bot, yer));///Arrayint(yr, yer)含有yer个元素的int型数组,每个都初始化为yr
}
Wine::Wine(const char*l, int y)
{
fullname = l;
yer=y;
}
void Wine::Getbottles()
{
Arrayint yr(yer), bt(yer);//将yr、bt分别初始化为含有yer个元素的数组
for (int i = 0; i < yer; i++)
{
cout << "enter the year: ";
cin >> yr[i];
cout << "enter the bottles: ";
cin >> bt[i];
}
while (cin.get() != '\n')
continue;
yb.Set(yr, bt);
}
string& Wine::Label()
{
return fullname;
}
int Wine::sum() const
{
return yb.Sum();
}
void Wine::Show()const
{
cout << "Wine: " << (*this).fullname << endl;
cout << "\tyear\tbottles\n";
yb.Show(yer);
}test.cpp#include<iostream>
#include"wine.h"
int main()
{
using std::cin;
using std::cout;
using std::endl;
cout << "enter name of wine: ";
char lab[50];
cin.getline(lab, 50);
cout << "enter number of years: ";
int yrs;
cin >> yrs;
Wine holding(lab, yrs);
holding.Getbottles();
holding.Show();
const int YRS = 3;
int y[YRS] = { 1993,1995,1998 };
int b[YRS] = { 48,60,72 };
Wine more(y, b, "gushing grape red", YRS);
more.Show();
cout << "Total bottles for " << more.Label() << ": " << more.sum() << endl;
cout << "bye\n";
system("pause");
return 0;
}
显示:
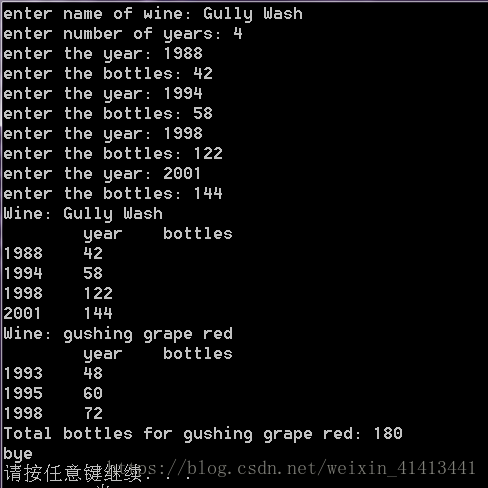
习题二:
Wine.h#ifndef WINE_H_
#define WINE_H_
#include<string>
#include<valarray>
using namespace std;
template<typename T1,typename T2>//声明一个Pair类
class Pair
{
private:
T1 year;
T2 bottles;
public:
Pair(const T1&s,const T2&m):year(s),bottles(m){}
Pair(){}
int Sum()const;
void Set(const T1&s1, const T2&s2);//T1、T2具体化时为数组
void Show(int y)const;
};
template<typename T1, typename T2>
int Pair<T1,T2>::Sum()const
{
return bottles.sum();
}
template<typename T1, typename T2>
void Pair<T1, T2>::Set(const T1&s1, const T2&s2)
{
year = s1;
bottles = s2;
}
template<typename T1,typename T2>
void Pair<T1, T2>::Show(int y)const
{
for (int i = 0; i < y; i++)
cout << year[i] << '\t' << bottles[i] << endl;
}
typedef valarray<int> Arrayint;
typedef Pair<Arrayint, Arrayint>Pairarray;
class Wine:private string,private Pairarray
{
private:
int yer;
public:
Wine() {};
Wine(const int yr[], const int bot[], const char*l, int y);
Wine(const char*l, int y);
void Getbottles();
string& Label();
int sum() const;
void Show()const;
};
#endif
Wine.cpp#include<iostream>
#include"wine.h"
Wine::Wine(const int yr[], const int bot[], const char*l, int y) :Pairarray(Arrayint(yr, yer), Arrayint(bot, yer)),string(l),yer(y)
{
}///私有继承使用类型进行初始化
Wine::Wine(const char*l, int y):string(l),yer(y)
{
}
void Wine::Getbottles()
{
Arrayint yr(yer), bt(yer);//将yr、bt分别初始化为含有yer个元素的数组
for (int i = 0; i < yer; i++)
{
cout << "enter the year: ";
cin >> yr[i];
cout << "enter the bottles: ";
cin >> bt[i];
}
while (cin.get() != '\n')
continue;
Pairarray::Set(yr, bt);///私有继承使用基类方法,因为没有对象名,故利用(类名::方法)调用基类方法
}
string& Wine::Label()
{
return (string &)(*this);///该引用指向用于调用该方法的Wine对象中的继承而来的string对象。
}
int Wine::sum() const
{
return Pairarray::Sum();
}
void Wine::Show()const
{
cout << "Wine: " << (string &)(*this) << endl;
cout << "\tyear\tbottles\n";
Pairarray::Show(yer);
}
test.cpp#include<iostream>
#include"wine.h"
int main()
{
using std::cin;
using std::cout;
using std::endl;
cout << "enter name of wine: ";
char lab[50];
cin.getline(lab, 50);
cout << "enter number of years: ";
int yrs;
cin >> yrs;
Wine holding(lab, yrs);
holding.Getbottles();
holding.Show();
const int YRS = 3;
int y[YRS] = { 1993,1995,1998 };
int b[YRS] = { 48,60,72 };
Wine more(y, b, "gushing grape red", YRS);
more.Show();
cout << "Total bottles for " << more.Label() << ": " << more.sum() << endl;
cout << "bye\n";
system("pause");
return 0;
}
习题三
Queuetp.h
#ifndef QUEUETP_H_
#define QUEUETP_H_
#include<string>
#include<cstring>
#include<iostream>
using namespace std;
template<typename T>
class Queuetp
{
private:
struct Node {
T item;
struct Node*next;
};
Node*front;
Node*rear;
int items;
const int qsize;
Queuetp(const Queuetp&q) :qsize(10) {}//复制构造函数设置为伪私有方法,因为目前并不需要他们
Queuetp&operator=(const Queuetp&q) { return *this; }//将赋值运算符设置为伪私有方法,因为目前并不需要他们
public:
Queuetp(int qsize = 10);
~Queuetp();
bool isempty()const;
bool isfull()const;
int queuecount()const;
bool enqueue(T&item);
bool dequeue(T&item);
};
template<typename T>
Queuetp<T>::Queuetp(int qsize) :qsize(10)
{
rear = front = NULL;
items = 0;
}
template<typename T>
Queuetp<T>::~Queuetp()
{
Node*temp;
while (front != NULL)
{
temp = front;
front = front->next;
delete temp;
}
}
template<typename T>
bool Queuetp<T>::isempty()const
{
return items==0;
}
template<typename T>
bool Queuetp<T>::isfull()const
{
return items==qsize;
}
template<typename T>
int Queuetp<T>::queuecount()const
{
return items;
}
template<typename T>
bool Queuetp<T>::enqueue(T&item)
{
if (isfull())
return false;
Node *add = new Node;
item = add->item;
items++;
add->next = NULL;
if (front == NULL)
front = add;
else rear->next = add;
rear = add;
return true;
}
template<typename T>
bool Queuetp<T>::dequeue(T&item)
{
if (isempty())
return false;
item = front->item;
items--;
Node*temp = front;
front = front->next;
delete temp;
if (items==0)
rear = NULL;
return true;
}
class Worker
{
private:
string fullname;
long id;
public:
Worker():fullname("no one"),id(0L){}
Worker(const std::string&s,long n):fullname(s),id(n){}
~Worker() {};
void set();
void show() const;
};
void Worker::show() const
{
cout << "name: " << fullname << endl;
cout << "ID: " << id << endl;
}
void Worker::set()
{
cout << "enter the worker's name: ";
getline(cin, fullname);
cout << "eneter worker's ID: ";
cin >> id;
while (cin.get() != '\n')
continue;
}
#endif
Queuetp.cpp#inc
4000
lude<iostream>
#include"queuetp.h"
using namespace std;
const int SIZE = 5;
int main()
{
Queuetp<Worker*>lolas(SIZE);
Worker* temp;
int ct;
for(ct=0;ct<SIZE;ct++)
{
cout << "Enter the command:\n"
<< "A or a enter queue, "
<< "P or p delete queue, "
<< "Q or q quit.\n";
char ch;
cin >> ch;
while (strchr("aApPq", ch) == NULL)
{
cout << "Please enter a p or q: ";
cin >> ch;
}
if(ch == 'q')
break;
switch (ch )
{
case'a':
case'A':
temp = new Worker;
cin.get();
temp->set();
if (lolas.isfull())
cout << "the queue is already full\n";
else
lolas.enqueue(temp);
break;
case 'p':
case'P':
if (lolas.isempty())
cout << "the queue is empty\n";
else
{
lolas.dequeue(temp);
temp->show();
}
}
}
cout << "the total count is: ";
cout << lolas.queuecount();
cout << "\nbye!!!\n";
system("pause");
return 0;
}习题四
person.h#ifndef PERSON_H_
#define PERSON_H_
#include<string>
using namespace std;
class person
{
private:
std::string name;
std::string firstname;
protected:
virtual void data()const;
virtual void get();
public:
person(const char*s, const char*t):name(s),firstname(t){}
person():name("none"),firstname("none"){}
person(const person &p);
virtual void show()const=0;
virtual ~person() = 0;
void set() { get(); }
};
class Gunslinger :virtual public person
{
private:
int count;
protected:
void data()const;
void get();
public:
Gunslinger() :person(), count(0){}
Gunslinger(const char*s,const char*t,int c=0):person(s,t),count(0){}
Gunslinger(const person&pr,int c=0):person(pr),count(0){}
void set();
void show()const;
double Draw()const;
};
class pokerplayer :virtual public person
{
protected:
void data()const;
public:
pokerplayer():person(){}
pokerplayer(const char*s, const char*t, int c = 0) :person(s, t) {}
pokerplayer(const person&pr) :person(pr){}
void set() { person::set(); }
void show()const;
int Draw()const;
};
class baddude:public pokerplayer,public Gunslinger
{
protected:
void data()const;
void get();
public:
baddude(){}
baddude(const char*f, const char*l,int c)
:person(f,l), Gunslinger(f,l,c), pokerplayer(f, l) {}
baddude(int c, const person &p):person(p), Gunslinger(p,c), pokerplayer(p) {}
baddude(const Gunslinger &g)
:person(g), Gunslinger(g),pokerplayer(g) {}
baddude(const pokerplayer&po, int c)
:person(po), Gunslinger(po, c), pokerplayer(po) {}///为什么需要这么多构造函数
void set();
void show()const;
double Gdraw()const;
int Cdraw()const;
};
#endif
person.cpp#include "person.h"
#include<iostream>
person::~person()
{
}
void person::data()const
{
cout << "name: " << name << endl;
cout << "first name: " << firstname << endl;
}
void person::get()
{
cout << "enter the name: ";
getline(cin, name);
cout << "enter the first name: ";
getline(cin, firstname);
while (cin.get() != '\n')
continue;
}
void person::show()const
{
data();
}
void Gunslinger::data()const
{
cout << "the count is " << count << endl;
cout << "the gunlar's baqiang time " << Gunslinger::Draw() << endl;
}
void Gunslinger::get()
{
cout << "enter the count: ";
cin >> count;
while (cin.get() != '\n')
continue;
}
void Gunslinger::set()
{
cout << "enter the Gunslinger name: ";
person::get();
get();
}
void Gunslinger::show()const
{
person::data();
data();
}
double Gunslinger::Draw()const
{
return rand() % 3 + 1;
}
int pokerplayer::Draw()const
{
return rand() % 52 + 1;
}
void pokerplayer::data()const
{
cout << "The cards :" << Draw() << endl;
}
void pokerplayer::show()const
{
cout << "pokerplayer: ";
person::show();
data();
}
void baddude::data()const
{
Gunslinger::data();
pokerplayer::data();
cout << "The next cards: " << Cdraw() << endl;
cout << "The time of BadDude get the gun: " << Gdraw() << endl;
}
void baddude::get()
{
Gunslinger::get();
}
void baddude::set()
{
cout << "Enter BadDude name: \n";
person::get();
get();
}
void baddude::show()const
{
cout << "BadDude: \n";
person::data();
data();
}
double baddude::Gdraw()const
{
return Gunslinger::Draw();
}
int baddude::Cdraw()const
{
return pokerplayer::Draw();
}
test.cpp#include<iostream>
#include"person.h"
const int Size = 5;
using namespace std;
int main()
{
person* sit[Size];//抽象基类不能创建对象但是能够创建指针或引用
int ct ;
for (ct = 0; ct < Size; ct++)
{
char choice;
cout << "enter the person: \n"
<< "g:Gunslinger p:pokerplayer " << "b:baddude q:quit\n";
cin >> choice;
while (strchr("gpbq", choice) == NULL)
{
cout << "please enter a g、p、b、q:";
cin >> choice;
}
if (choice == 'q')
break;
switch (choice)//输入各种person的参数信息
{
case'g':sit[ct] = new Gunslinger;//基类指向它的派生类
break;
case'p':sit[ct] = new pokerplayer;
break;
case'b':sit[ct] = new baddude;
break;
}
cin.get();
sit[ct]->set();
}
cout << "\nhere is your staff:\n";
int i;
for (i = 0; i < ct; i++)//输出各种person的参数信息
{
cout << endl;
sit[ct]->show();
}
for (i = 0; i < ct; i++)
{
delete sit[ct];
}
cout << "bye:\n";
system("pause");
return 0;
}第五题
emp.h#include<iostream>
#include<string>
class abstr_emp
{
private:
std::string fname;
std::string lname;
std::string job;
public:
abstr_emp();
abstr_emp(const std::string& fn, const std::string& ln, const std::string& j);
virtual void Showall()const;
virtual void Setall();
friend std::ostream&operator<<(std::ostream&os, const abstr_emp&e);
virtual ~abstr_emp() = 0;
};
class employee :public abstr_emp
{
public:
employee();
employee(const std::string& fn, const std::string& ln, const std::string& j);
virtual void Showall()const;
virtual void Setall();
};
class manager :virtual public abstr_emp
{
private:
int inchargeof;
protected:
int Inchargeof()const { return inchargeof; }
int &Inchargeof() { return inchargeof; }
public:
manager();
manager(const std::string& fn, const std::string& ln, const std::string& j, int ico = 0);
manager(const abstr_emp&e, int ico = 0);
manager(const manager&m);
virtual void Showall()const;
virtual void Setall();
};
class fink :virtual public abstr_emp
{
private:
std::string reportsto;
protected:
const std::string Reportsto()const { return reportsto; }
std::string& Reportsto() { return reportsto; }
public:
fink();
fink(const std::string& fn, const std::string& ln, const std::string& j,const std::string& rpo);
fink(const abstr_emp& e, const std::string rpo);
fink(const fink&e);
virtual void Showall()const;
virtual void Setall();
};
class highfink: public manager,public fink
{
public:
highfink();
highfink(const std::string& fn, const std::string& ln, const std::string& j, std::string& rpo, int ico=0);
highfink(const abstr_emp&e, const std::string & rpo, int ico);
highfink(const fink&f, int ico);
highfink(const manager&m, const std::string&rpo);
highfink(const highfink&h);
virtual void Showall()const;
virtual void Setall();
};
emp.cpp#include"emp.h"
#include<iostream>
using namespace std;
abstr_emp::abstr_emp() :fname("none"), lname("none"), job("none")
{
}
abstr_emp::abstr_emp(const std::string& fn, const std::string& ln, const std::string& j):fname(fn),lname(ln),job(j)
{
}
void abstr_emp::Showall()const
{
cout << "the type's name is: " << fname << " " << lname << endl;
cout << "his job is: " << job << endl;
}
void abstr_emp::Setall()
{
cout << "please enter the fname: ";
getline(cin, fname);
cout << "\nplease enter the lname: ";
getline(cin, lname);
cout << "\nplease enter his job: ";
getline(cin, job);
}
std::ostream&operator<<(std::ostream&os, const abstr_emp&e)
{
os << "the e's name is: " << e.fname << " " << e.lname << endl;
os << " the e's job is: " << e.job << endl;
return os;
}
abstr_emp::~abstr_emp()
{
}
///////employee
employee::employee() :abstr_emp()
{
}
employee::employee(const std::string& fn, const std::string& ln, const std::string& j) : abstr_emp(fn, ln, j)
{
}
void employee::Showall()const
{
cout << "show the employee's data\n";
abstr_emp::Showall();
}
void employee::Setall()
{
cout << "enter the employee's data\n";
abstr_emp::Setall();
}
//////manager
manager::manager() :abstr_emp()
{
inchargeof = 0;
}
manager::manager(const std::string& fn, const std::string& ln, const std::string& j, int ico) : abstr_emp(fn, ln, j), inchargeof(ico)
{
}
manager::manager(const abstr_emp&e, int ico) : abstr_emp(e), inchargeof(ico)///调用默认复制构造函数
{
}
manager::manager(const manager&m) : abstr_emp(m)
{
inchargeof =m.inchargeof;
}
void manager::Showall()const
{
cout << "show the manager's data\n";
abstr_emp::Showall();
cout << "enter number is: "<< inchargeof<<endl;
}
void manager::Setall()
{
cout << "enter the manager's data\n";
abstr_emp::Setall();
cout << "enter number is: ";
cin >> inchargeof;
while (cin.get() != '\n')
continue;
}
//////////fink
fink::fink() :abstr_emp()
{
reportsto = "none";
}
fink::fink(const std::string& fn, const std::string& ln, const std::string& j, const std::string& rpo) : abstr_emp(fn, ln, j), reportsto(rpo)
{
}
fink::fink(const abstr_emp& e, const std::string rpo) : abstr_emp(e), reportsto(rpo)
{
}
fink::fink(const fink&e) : abstr_emp(e)
{
reportsto = e.reportsto;
}
void fink::Showall()const
{
cout << "show the fink's data\n";
abstr_emp::Showall();
cout << "the report person is: " << reportsto << endl;
}
void fink::Setall()
{
cout << "enter the fink's data\n";
abstr_emp::Setall();
cout << "enter report person is: ";
getline(cin, reportsto);
}
highfink::highfink() :abstr_emp(), manager(), fink()
{
}
highfink::highfink(const std::string& fn, const std::string& ln, const std::string& j, std::string& rpo, int ico) : abstr_emp(fn, ln, j), manager(fn, ln, j, ico), fink(fn, ln, j, rpo)
{
}
highfink::highfink(const abstr_emp&e, const std::string & rpo, int ico) : abstr_emp(e), manager(e, ico), fink(e, rpo)
{
}
highfink::highfink(const fink&f, int ico) : abstr_emp(f), manager(f, ico), fink(f)
{
}
highfink::highfink(const manager&m, const std::string&rpo) : abstr_emp(m), manager(m), fink(m, rpo)
{
}
highfink::highfink(const highfink&h) : abstr_emp(h), manager(h), fink(h)
{
}
void highfink::Showall()const
{
cout << "show the highfink's data\n";
manager::Showall();
fink::Showall();
}
void highfink::Setall()
{
cout << "enter the highfink's data\n";
manager::Setall();
fink::Setall();
}
test.cpp#include<iostream>
using namespace std;
#include"emp.h"
int main()
{
employee em("trip", "harris", "thumper");
cout << em << endl;
em.Showall();
manager ma("amorphia", "sprindragon", "nuancer", 5);
cout << ma << endl;
ma.Showall();
fink fi("oggs","oggs", "olier", "juno barr");
cout << fi << endl;
fi.Showall();
highfink hf(ma, "curly kew");
hf.Showall();
cout << "please enter a key for next phase:\n";
cin.get();
highfink hf2;
hf2.Setall();
cout << "using an abstr_emp* pointer:\n";
abstr_emp* tri[4] = { &em,&fi,&hf,&hf2 };
for (int i = 0; i < 4; i++)
tri[i]->Showall();
system("pause");
return 0;
}部分结果
Wine.h#ifndef WINE_H_
#define WINE_H_
#include<string>
#include<valarray>
using namespace std;
template<typename T1,typename T2>//声明一个Pair类
class Pair
{
private:
T1 year;
T2 bottles;
public:
Pair(const T1&s,const T2&m):year(s),bottles(m){}
Pair(){}
int Sum()const;
void Set(const T1&s1, const T2&s2);//T1、T2具体化时为数组
void Show(int y)const;
};
template<typename T1, typename T2>
int Pair<T1,T2>::Sum()const
{
return bottles.sum();
}
template<typename T1, typename T2>
void Pair<T1, T2>::Set(const T1&s1, const T2&s2)
{
year = s1;
bottles = s2;
}
template<typename T1,typename T2>
void Pair<T1, T2>::Show(int y)const
{
for (int i = 0; i < y; i++)
cout << year[i] << '\t' << bottles[i] << endl;
}
typedef valarray<int> Arrayint;
typedef Pair<Arrayint, Arrayint>Pairarray;
class Wine
{
private:
Pairarray yb;
string fullname;
int yer;
public:
Wine();
Wine(const int yr[], const int bot[], const char*l, int y);
Wine(const char*l, int y);
void Getbottles();
string& Label();
int sum() const;
void Show()const;
};
#endifWine.cpp#include<iostream>
#include"wine.h"
Wine::Wine(const int yr[], const int bot[], const char*l, int y)
{
fullname = l;
yer = y;
yb.Set(Arrayint(yr, yer), Arrayint(bot, yer));///Arrayint(yr, yer)含有yer个元素的int型数组,每个都初始化为yr
}
Wine::Wine(const char*l, int y)
{
fullname = l;
yer=y;
}
void Wine::Getbottles()
{
Arrayint yr(yer), bt(yer);//将yr、bt分别初始化为含有yer个元素的数组
for (int i = 0; i < yer; i++)
{
cout << "enter the year: ";
cin >> yr[i];
cout << "enter the bottles: ";
cin >> bt[i];
}
while (cin.get() != '\n')
continue;
yb.Set(yr, bt);
}
string& Wine::Label()
{
return fullname;
}
int Wine::sum() const
{
return yb.Sum();
}
void Wine::Show()const
{
cout << "Wine: " << (*this).fullname << endl;
cout << "\tyear\tbottles\n";
yb.Show(yer);
}test.cpp#include<iostream>
#include"wine.h"
int main()
{
using std::cin;
using std::cout;
using std::endl;
cout << "enter name of wine: ";
char lab[50];
cin.getline(lab, 50);
cout << "enter number of years: ";
int yrs;
cin >> yrs;
Wine holding(lab, yrs);
holding.Getbottles();
holding.Show();
const int YRS = 3;
int y[YRS] = { 1993,1995,1998 };
int b[YRS] = { 48,60,72 };
Wine more(y, b, "gushing grape red", YRS);
more.Show();
cout << "Total bottles for " << more.Label() << ": " << more.sum() << endl;
cout << "bye\n";
system("pause");
return 0;
}
显示:
习题二:
Wine.h#ifndef WINE_H_
#define WINE_H_
#include<string>
#include<valarray>
using namespace std;
template<typename T1,typename T2>//声明一个Pair类
class Pair
{
private:
T1 year;
T2 bottles;
public:
Pair(const T1&s,const T2&m):year(s),bottles(m){}
Pair(){}
int Sum()const;
void Set(const T1&s1, const T2&s2);//T1、T2具体化时为数组
void Show(int y)const;
};
template<typename T1, typename T2>
int Pair<T1,T2>::Sum()const
{
return bottles.sum();
}
template<typename T1, typename T2>
void Pair<T1, T2>::Set(const T1&s1, const T2&s2)
{
year = s1;
bottles = s2;
}
template<typename T1,typename T2>
void Pair<T1, T2>::Show(int y)const
{
for (int i = 0; i < y; i++)
cout << year[i] << '\t' << bottles[i] << endl;
}
typedef valarray<int> Arrayint;
typedef Pair<Arrayint, Arrayint>Pairarray;
class Wine:private string,private Pairarray
{
private:
int yer;
public:
Wine() {};
Wine(const int yr[], const int bot[], const char*l, int y);
Wine(const char*l, int y);
void Getbottles();
string& Label();
int sum() const;
void Show()const;
};
#endif
Wine.cpp#include<iostream>
#include"wine.h"
Wine::Wine(const int yr[], const int bot[], const char*l, int y) :Pairarray(Arrayint(yr, yer), Arrayint(bot, yer)),string(l),yer(y)
{
}///私有继承使用类型进行初始化
Wine::Wine(const char*l, int y):string(l),yer(y)
{
}
void Wine::Getbottles()
{
Arrayint yr(yer), bt(yer);//将yr、bt分别初始化为含有yer个元素的数组
for (int i = 0; i < yer; i++)
{
cout << "enter the year: ";
cin >> yr[i];
cout << "enter the bottles: ";
cin >> bt[i];
}
while (cin.get() != '\n')
continue;
Pairarray::Set(yr, bt);///私有继承使用基类方法,因为没有对象名,故利用(类名::方法)调用基类方法
}
string& Wine::Label()
{
return (string &)(*this);///该引用指向用于调用该方法的Wine对象中的继承而来的string对象。
}
int Wine::sum() const
{
return Pairarray::Sum();
}
void Wine::Show()const
{
cout << "Wine: " << (string &)(*this) << endl;
cout << "\tyear\tbottles\n";
Pairarray::Show(yer);
}
test.cpp#include<iostream>
#include"wine.h"
int main()
{
using std::cin;
using std::cout;
using std::endl;
cout << "enter name of wine: ";
char lab[50];
cin.getline(lab, 50);
cout << "enter number of years: ";
int yrs;
cin >> yrs;
Wine holding(lab, yrs);
holding.Getbottles();
holding.Show();
const int YRS = 3;
int y[YRS] = { 1993,1995,1998 };
int b[YRS] = { 48,60,72 };
Wine more(y, b, "gushing grape red", YRS);
more.Show();
cout << "Total bottles for " << more.Label() << ": " << more.sum() << endl;
cout << "bye\n";
system("pause");
return 0;
}
习题三
Queuetp.h
#ifndef QUEUETP_H_
#define QUEUETP_H_
#include<string>
#include<cstring>
#include<iostream>
using namespace std;
template<typename T>
class Queuetp
{
private:
struct Node {
T item;
struct Node*next;
};
Node*front;
Node*rear;
int items;
const int qsize;
Queuetp(const Queuetp&q) :qsize(10) {}//复制构造函数设置为伪私有方法,因为目前并不需要他们
Queuetp&operator=(const Queuetp&q) { return *this; }//将赋值运算符设置为伪私有方法,因为目前并不需要他们
public:
Queuetp(int qsize = 10);
~Queuetp();
bool isempty()const;
bool isfull()const;
int queuecount()const;
bool enqueue(T&item);
bool dequeue(T&item);
};
template<typename T>
Queuetp<T>::Queuetp(int qsize) :qsize(10)
{
rear = front = NULL;
items = 0;
}
template<typename T>
Queuetp<T>::~Queuetp()
{
Node*temp;
while (front != NULL)
{
temp = front;
front = front->next;
delete temp;
}
}
template<typename T>
bool Queuetp<T>::isempty()const
{
return items==0;
}
template<typename T>
bool Queuetp<T>::isfull()const
{
return items==qsize;
}
template<typename T>
int Queuetp<T>::queuecount()const
{
return items;
}
template<typename T>
bool Queuetp<T>::enqueue(T&item)
{
if (isfull())
return false;
Node *add = new Node;
item = add->item;
items++;
add->next = NULL;
if (front == NULL)
front = add;
else rear->next = add;
rear = add;
return true;
}
template<typename T>
bool Queuetp<T>::dequeue(T&item)
{
if (isempty())
return false;
item = front->item;
items--;
Node*temp = front;
front = front->next;
delete temp;
if (items==0)
rear = NULL;
return true;
}
class Worker
{
private:
string fullname;
long id;
public:
Worker():fullname("no one"),id(0L){}
Worker(const std::string&s,long n):fullname(s),id(n){}
~Worker() {};
void set();
void show() const;
};
void Worker::show() const
{
cout << "name: " << fullname << endl;
cout << "ID: " << id << endl;
}
void Worker::set()
{
cout << "enter the worker's name: ";
getline(cin, fullname);
cout << "eneter worker's ID: ";
cin >> id;
while (cin.get() != '\n')
continue;
}
#endif
Queuetp.cpp#inc
4000
lude<iostream>
#include"queuetp.h"
using namespace std;
const int SIZE = 5;
int main()
{
Queuetp<Worker*>lolas(SIZE);
Worker* temp;
int ct;
for(ct=0;ct<SIZE;ct++)
{
cout << "Enter the command:\n"
<< "A or a enter queue, "
<< "P or p delete queue, "
<< "Q or q quit.\n";
char ch;
cin >> ch;
while (strchr("aApPq", ch) == NULL)
{
cout << "Please enter a p or q: ";
cin >> ch;
}
if(ch == 'q')
break;
switch (ch )
{
case'a':
case'A':
temp = new Worker;
cin.get();
temp->set();
if (lolas.isfull())
cout << "the queue is already full\n";
else
lolas.enqueue(temp);
break;
case 'p':
case'P':
if (lolas.isempty())
cout << "the queue is empty\n";
else
{
lolas.dequeue(temp);
temp->show();
}
}
}
cout << "the total count is: ";
cout << lolas.queuecount();
cout << "\nbye!!!\n";
system("pause");
return 0;
}习题四
person.h#ifndef PERSON_H_
#define PERSON_H_
#include<string>
using namespace std;
class person
{
private:
std::string name;
std::string firstname;
protected:
virtual void data()const;
virtual void get();
public:
person(const char*s, const char*t):name(s),firstname(t){}
person():name("none"),firstname("none"){}
person(const person &p);
virtual void show()const=0;
virtual ~person() = 0;
void set() { get(); }
};
class Gunslinger :virtual public person
{
private:
int count;
protected:
void data()const;
void get();
public:
Gunslinger() :person(), count(0){}
Gunslinger(const char*s,const char*t,int c=0):person(s,t),count(0){}
Gunslinger(const person&pr,int c=0):person(pr),count(0){}
void set();
void show()const;
double Draw()const;
};
class pokerplayer :virtual public person
{
protected:
void data()const;
public:
pokerplayer():person(){}
pokerplayer(const char*s, const char*t, int c = 0) :person(s, t) {}
pokerplayer(const person&pr) :person(pr){}
void set() { person::set(); }
void show()const;
int Draw()const;
};
class baddude:public pokerplayer,public Gunslinger
{
protected:
void data()const;
void get();
public:
baddude(){}
baddude(const char*f, const char*l,int c)
:person(f,l), Gunslinger(f,l,c), pokerplayer(f, l) {}
baddude(int c, const person &p):person(p), Gunslinger(p,c), pokerplayer(p) {}
baddude(const Gunslinger &g)
:person(g), Gunslinger(g),pokerplayer(g) {}
baddude(const pokerplayer&po, int c)
:person(po), Gunslinger(po, c), pokerplayer(po) {}///为什么需要这么多构造函数
void set();
void show()const;
double Gdraw()const;
int Cdraw()const;
};
#endif
person.cpp#include "person.h"
#include<iostream>
person::~person()
{
}
void person::data()const
{
cout << "name: " << name << endl;
cout << "first name: " << firstname << endl;
}
void person::get()
{
cout << "enter the name: ";
getline(cin, name);
cout << "enter the first name: ";
getline(cin, firstname);
while (cin.get() != '\n')
continue;
}
void person::show()const
{
data();
}
void Gunslinger::data()const
{
cout << "the count is " << count << endl;
cout << "the gunlar's baqiang time " << Gunslinger::Draw() << endl;
}
void Gunslinger::get()
{
cout << "enter the count: ";
cin >> count;
while (cin.get() != '\n')
continue;
}
void Gunslinger::set()
{
cout << "enter the Gunslinger name: ";
person::get();
get();
}
void Gunslinger::show()const
{
person::data();
data();
}
double Gunslinger::Draw()const
{
return rand() % 3 + 1;
}
int pokerplayer::Draw()const
{
return rand() % 52 + 1;
}
void pokerplayer::data()const
{
cout << "The cards :" << Draw() << endl;
}
void pokerplayer::show()const
{
cout << "pokerplayer: ";
person::show();
data();
}
void baddude::data()const
{
Gunslinger::data();
pokerplayer::data();
cout << "The next cards: " << Cdraw() << endl;
cout << "The time of BadDude get the gun: " << Gdraw() << endl;
}
void baddude::get()
{
Gunslinger::get();
}
void baddude::set()
{
cout << "Enter BadDude name: \n";
person::get();
get();
}
void baddude::show()const
{
cout << "BadDude: \n";
person::data();
data();
}
double baddude::Gdraw()const
{
return Gunslinger::Draw();
}
int baddude::Cdraw()const
{
return pokerplayer::Draw();
}
test.cpp#include<iostream>
#include"person.h"
const int Size = 5;
using namespace std;
int main()
{
person* sit[Size];//抽象基类不能创建对象但是能够创建指针或引用
int ct ;
for (ct = 0; ct < Size; ct++)
{
char choice;
cout << "enter the person: \n"
<< "g:Gunslinger p:pokerplayer " << "b:baddude q:quit\n";
cin >> choice;
while (strchr("gpbq", choice) == NULL)
{
cout << "please enter a g、p、b、q:";
cin >> choice;
}
if (choice == 'q')
break;
switch (choice)//输入各种person的参数信息
{
case'g':sit[ct] = new Gunslinger;//基类指向它的派生类
break;
case'p':sit[ct] = new pokerplayer;
break;
case'b':sit[ct] = new baddude;
break;
}
cin.get();
sit[ct]->set();
}
cout << "\nhere is your staff:\n";
int i;
for (i = 0; i < ct; i++)//输出各种person的参数信息
{
cout << endl;
sit[ct]->show();
}
for (i = 0; i < ct; i++)
{
delete sit[ct];
}
cout << "bye:\n";
system("pause");
return 0;
}第五题
emp.h#include<iostream>
#include<string>
class abstr_emp
{
private:
std::string fname;
std::string lname;
std::string job;
public:
abstr_emp();
abstr_emp(const std::string& fn, const std::string& ln, const std::string& j);
virtual void Showall()const;
virtual void Setall();
friend std::ostream&operator<<(std::ostream&os, const abstr_emp&e);
virtual ~abstr_emp() = 0;
};
class employee :public abstr_emp
{
public:
employee();
employee(const std::string& fn, const std::string& ln, const std::string& j);
virtual void Showall()const;
virtual void Setall();
};
class manager :virtual public abstr_emp
{
private:
int inchargeof;
protected:
int Inchargeof()const { return inchargeof; }
int &Inchargeof() { return inchargeof; }
public:
manager();
manager(const std::string& fn, const std::string& ln, const std::string& j, int ico = 0);
manager(const abstr_emp&e, int ico = 0);
manager(const manager&m);
virtual void Showall()const;
virtual void Setall();
};
class fink :virtual public abstr_emp
{
private:
std::string reportsto;
protected:
const std::string Reportsto()const { return reportsto; }
std::string& Reportsto() { return reportsto; }
public:
fink();
fink(const std::string& fn, const std::string& ln, const std::string& j,const std::string& rpo);
fink(const abstr_emp& e, const std::string rpo);
fink(const fink&e);
virtual void Showall()const;
virtual void Setall();
};
class highfink: public manager,public fink
{
public:
highfink();
highfink(const std::string& fn, const std::string& ln, const std::string& j, std::string& rpo, int ico=0);
highfink(const abstr_emp&e, const std::string & rpo, int ico);
highfink(const fink&f, int ico);
highfink(const manager&m, const std::string&rpo);
highfink(const highfink&h);
virtual void Showall()const;
virtual void Setall();
};
emp.cpp#include"emp.h"
#include<iostream>
using namespace std;
abstr_emp::abstr_emp() :fname("none"), lname("none"), job("none")
{
}
abstr_emp::abstr_emp(const std::string& fn, const std::string& ln, const std::string& j):fname(fn),lname(ln),job(j)
{
}
void abstr_emp::Showall()const
{
cout << "the type's name is: " << fname << " " << lname << endl;
cout << "his job is: " << job << endl;
}
void abstr_emp::Setall()
{
cout << "please enter the fname: ";
getline(cin, fname);
cout << "\nplease enter the lname: ";
getline(cin, lname);
cout << "\nplease enter his job: ";
getline(cin, job);
}
std::ostream&operator<<(std::ostream&os, const abstr_emp&e)
{
os << "the e's name is: " << e.fname << " " << e.lname << endl;
os << " the e's job is: " << e.job << endl;
return os;
}
abstr_emp::~abstr_emp()
{
}
///////employee
employee::employee() :abstr_emp()
{
}
employee::employee(const std::string& fn, const std::string& ln, const std::string& j) : abstr_emp(fn, ln, j)
{
}
void employee::Showall()const
{
cout << "show the employee's data\n";
abstr_emp::Showall();
}
void employee::Setall()
{
cout << "enter the employee's data\n";
abstr_emp::Setall();
}
//////manager
manager::manager() :abstr_emp()
{
inchargeof = 0;
}
manager::manager(const std::string& fn, const std::string& ln, const std::string& j, int ico) : abstr_emp(fn, ln, j), inchargeof(ico)
{
}
manager::manager(const abstr_emp&e, int ico) : abstr_emp(e), inchargeof(ico)///调用默认复制构造函数
{
}
manager::manager(const manager&m) : abstr_emp(m)
{
inchargeof =m.inchargeof;
}
void manager::Showall()const
{
cout << "show the manager's data\n";
abstr_emp::Showall();
cout << "enter number is: "<< inchargeof<<endl;
}
void manager::Setall()
{
cout << "enter the manager's data\n";
abstr_emp::Setall();
cout << "enter number is: ";
cin >> inchargeof;
while (cin.get() != '\n')
continue;
}
//////////fink
fink::fink() :abstr_emp()
{
reportsto = "none";
}
fink::fink(const std::string& fn, const std::string& ln, const std::string& j, const std::string& rpo) : abstr_emp(fn, ln, j), reportsto(rpo)
{
}
fink::fink(const abstr_emp& e, const std::string rpo) : abstr_emp(e), reportsto(rpo)
{
}
fink::fink(const fink&e) : abstr_emp(e)
{
reportsto = e.reportsto;
}
void fink::Showall()const
{
cout << "show the fink's data\n";
abstr_emp::Showall();
cout << "the report person is: " << reportsto << endl;
}
void fink::Setall()
{
cout << "enter the fink's data\n";
abstr_emp::Setall();
cout << "enter report person is: ";
getline(cin, reportsto);
}
highfink::highfink() :abstr_emp(), manager(), fink()
{
}
highfink::highfink(const std::string& fn, const std::string& ln, const std::string& j, std::string& rpo, int ico) : abstr_emp(fn, ln, j), manager(fn, ln, j, ico), fink(fn, ln, j, rpo)
{
}
highfink::highfink(const abstr_emp&e, const std::string & rpo, int ico) : abstr_emp(e), manager(e, ico), fink(e, rpo)
{
}
highfink::highfink(const fink&f, int ico) : abstr_emp(f), manager(f, ico), fink(f)
{
}
highfink::highfink(const manager&m, const std::string&rpo) : abstr_emp(m), manager(m), fink(m, rpo)
{
}
highfink::highfink(const highfink&h) : abstr_emp(h), manager(h), fink(h)
{
}
void highfink::Showall()const
{
cout << "show the highfink's data\n";
manager::Showall();
fink::Showall();
}
void highfink::Setall()
{
cout << "enter the highfink's data\n";
manager::Setall();
fink::Setall();
}
test.cpp#include<iostream>
using namespace std;
#include"emp.h"
int main()
{
employee em("trip", "harris", "thumper");
cout << em << endl;
em.Showall();
manager ma("amorphia", "sprindragon", "nuancer", 5);
cout << ma << endl;
ma.Showall();
fink fi("oggs","oggs", "olier", "juno barr");
cout << fi << endl;
fi.Showall();
highfink hf(ma, "curly kew");
hf.Showall();
cout << "please enter a key for next phase:\n";
cin.get();
highfink hf2;
hf2.Setall();
cout << "using an abstr_emp* pointer:\n";
abstr_emp* tri[4] = { &em,&fi,&hf,&hf2 };
for (int i = 0; i < 4; i++)
tri[i]->Showall();
system("pause");
return 0;
}部分结果
相关文章推荐
- C++ primer plus 第七章习题答案
- 《C++ Primer Plus(第六版)》(29)(第十四章 C++中的代码重用 复习题答案)
- c++ primer plus第十章习题答案
- C++ primer plus(第六版)学习笔记、习题答案(2)
- C++ primer plus(第六版)学习笔记、习题答案(4)
- C++ primer plus(第六版)学习笔记、习题答案(3)
- c++ primer plus第十一章习题答案
- c++ primer plus第九章习题答案
- C++ Primer Plus (第6版)课后习题答案 第二章
- c++ primer plus第三章习题答案
- C++ primer plus(第六版)学习笔记、习题答案(1)
- c++ primer plus第四章习题答案
- c++primer plus 第八章习题答案(自己写的)
- C++ primer plus(第六版)学习笔记、习题答案(5)
- c++ primer plus第五章习题答案
- C++Primer Plus笔记——第四章 复合类型及课后习题答案
- c++ PrimerPlus 第六版中文版编程习题答案
- C++Primer Plus笔记——第二章 开始学习C++及课后习题答案
- C++Primer Plus笔记——第三章 处理数据及课后习题答案
- c++ primer plus第二章习题答案(原创请参考)