循环链表 CircleList的实现并解决约瑟夫环问题
2018-03-22 08:57
756 查看
本篇分析一下LinkList的另一个子类CircleList的实现,可参考以下两篇博客:
(1)单链表LinkList的实现
(2)静态单链表StaticLinkList的实现
1、任意数据元素都有一个前驱和一个后继;
2、所高的数1居元素的关系构成一个逻辑上的环。
(2)实现上
1、循环链表是一种特殊的的单链表;
2、尾结点的指针域保存了首结点的地址。
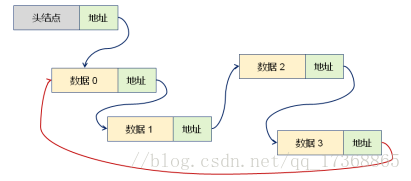
2、定义内部函数 last_to_first(),用于将单链表首尾相连;
3、特殊处理:考虑首元素的插入和删除操作(因为尾结点要指向首元素);
4、重新实现清空操作和遍历操作。(因为在 LinkList 里面是根据尾结点的next指针为空作为结束标记来实现清空和遍历操作,循环链表里面的尾结点不是空)。
插入位置为0的操作
(1)头结点和尾结点均指向新结点;
(2)新结点成为首结点插入链表。
删除位置为0的操作
(1)头结点和尾结点指向位置为 1 的结点;
(2)安全销毁首结点。
代码实现:
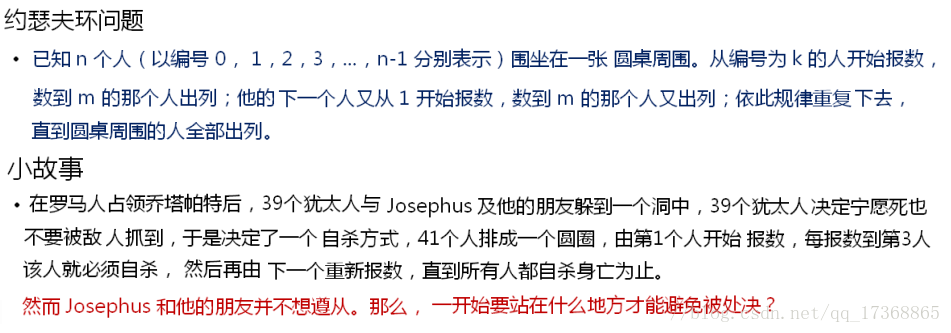
运行结果:
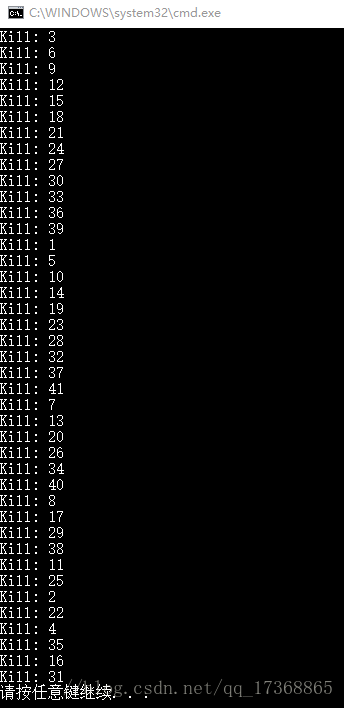
所以,约瑟夫和他的朋友应该站在16和31这两个位置才能避免这次处决。
(1)单链表LinkList的实现
(2)静态单链表StaticLinkList的实现
循环链表的定义
(1)概念上1、任意数据元素都有一个前驱和一个后继;
2、所高的数1居元素的关系构成一个逻辑上的环。
(2)实现上
1、循环链表是一种特殊的的单链表;
2、尾结点的指针域保存了首结点的地址。
循环链表的逻辑构成
循环链表的实现思路
1、通过模板定义 CircleList 类,继承自 LinkList 类;2、定义内部函数 last_to_first(),用于将单链表首尾相连;
3、特殊处理:考虑首元素的插入和删除操作(因为尾结点要指向首元素);
4、重新实现清空操作和遍历操作。(因为在 LinkList 里面是根据尾结点的next指针为空作为结束标记来实现清空和遍历操作,循环链表里面的尾结点不是空)。
实现要点
重点考虑首元素的插入和删除操作。插入位置为0的操作
(1)头结点和尾结点均指向新结点;
(2)新结点成为首结点插入链表。
删除位置为0的操作
(1)头结点和尾结点指向位置为 1 的结点;
(2)安全销毁首结点。
代码实现:
#ifndef _CIRCLELIST_H_ #define _CIRCLELIST_H_ #include "LinkList.h" namespace DTLib { template < typename T > class CircleList : public LinkList<T> { protected: typedef typename LinkList<T>::Node Node; int mod(int i) const // O(1) 归一化取余即可(即使坐标不是从1而是从0开始) { return(this->m_length == 0) ? 0 : (i % this->m_length); } // 获得尾结点 Node* last() const // O(n) { return this->position(this->m_length - 1)->next; } void last_to_first() const // O(n) { last()->next = this->m_header.next; } public: bool insert(const T& e) // O(n) { return insert(this->m_length, e); } bool insert(int i, const T& e) // O(1) + O(n) + O(n) ==> O(2n+1) ==> O(n) { bool ret = true; i = i % (this->m_length + 1); // O(1) 为什么加1?因为+1是代表 插入节点后该节点的相对位置(虽然此时未插入进去)不是现在循环链表的相对位置 ret = LinkList::insert(i, e); // O(n) if (ret && (i == 0)) { last_to_first(); // 首尾相连 // O(n) } return true; } bool remove(int i) // O(n) { bool ret = true; i = mod(i); if (i == 0) { Node* toDel = this->m_header.next; if (toDel != NULL) { this->m_header.next = toDel->next; this->m_length--; if (this->m_length > 0) { last_to_first(); if (this->m_current == toDel) // 删除的结点正好是current所指向结点 { this->m_current = toDel->next; } } else // 没有结点 { this->m_header.next = NULL; this->m_current = NULL; } this->destroy(toDel); // 保证异常安全 } else { ret = false; } } else // 删除非首结点的情况 { ret = LinkList<T>::remove(i); } return ret; } bool set(int i, const T& e) // O(n) { return LinkList<T>::set(mod(i), e); } T get(int i) const // O(n) { return LinkList<T>::get(mod(i)); } bool get(int i, const T& e) const // O(n) { return LinkList<T>::get(mod(i), e); } int find(const T& e) const // O(n) { int ret = -1; // 把循环链表变成单链表不行,因为在LinkList<T>::find(e)中可能发生异常, // 这样就无法执行last_to_first(),导致循环链表的状态被损坏。需要重新实现find函数。 /* last()->next = NULL; ret = LinkList<T>::find(e); last_to_first();*/ Node* slider = this->m_header.next; for (int i = 0; i < this->m_length; i++) { if (slider->value == e) // 这里即使发生异常,循环链表的状态也不会发生改变 { ret = i; break; } slider = slider->next; } return ret; } void clear() // O(n+1) ==> O(n) { while (this->m_length > 1) // O(n) { remove(1); // 使用remove(1)因为remove(0)效率比较低 // O(1) } if (this->m_length == 1) // O(1) { Node* toDel = this->m_header.next; this->m_header.next = NULL; this->m_length = 0; this->m_current = NULL; this->destroy(toDel); } } // 重新实现遍历操作 bool move(int i, int step) // O(n) { return LinkList<T>::move(mod(i), step); } bool end() // O(1) { return (this->m_length == 0) || (this->m_current == NULL); } ~CircleList() // O(n) { clear(); } }; } #endif
应用案例——用循环链表解决约瑟夫环问题
#include <iostream> #include "CircleList.h" using namespace std; using namespace DTLib; void josephus(int n, int s, int m) { CircleList<int> cl; for (int i = 1; i <= n; i++) { cl.insert(i); } cl.move(s - 1, m - 1); // 移动到s-1处,每一次移动m-1次 while (cl.length() > 0) { cl.next(); cout << "Kill: " << cl.current() << endl; cl.remove(cl.find(cl.current())); } } int main(void) { josephus(41, 1, 3); return 0; }
运行结果:
所以,约瑟夫和他的朋友应该站在16和31这两个位置才能避免这次处决。
相关文章推荐
- Java语言解决约瑟夫环问题(链表实现)
- PHP实现单向链表解决约瑟夫环问题
- 循环列表的Java实现,解决约瑟夫环问题
- 循环链表解决约瑟夫环问题
- 循环链表解决约瑟夫环问题
- 单循环链表解决约瑟夫环问题
- 用java实现链表并解决约瑟夫环问题
- 使用链表实现环结构以解决约瑟夫环问题
- 用单向循环链表解决约瑟夫环(Joseph)问题
- 单循环链表 解决约瑟夫环的问题
- 约瑟夫环问题(不带头结点单循环链表实现和数组实现)
- 循环列表的Java实现,解决约瑟夫环问题
- [转]用单向循环链表解决约瑟夫环问题
- PHP实现的基于单向链表解决约瑟夫环问题示例
- 第十课 循环链表,约瑟夫环问题的解决
- 循环链表解决约瑟夫环问题
- 循环链表 约瑟夫环问题实现
- 用个循环链表解决约瑟夫环问题
- 使用C#循环链表解决约瑟夫环的问题
- 自己实现集合框架(五):利用单链表解决约瑟夫环问题