牛客练习赛13 A B C F【二分+思维】
2018-03-21 18:01
393 查看
链接:https://www.nowcoder.com/acm/contest/70/A
来源:牛客网
题目描述
定义一个数字为幸运数字当且仅当它的所有数位都是4或者7。
比如说,47、744、4都是幸运数字而5、17、467都不是。
现在,给定一个字符串s,请求出一个字符串,使得:
1、它所代表的整数是一个幸运数字;
2、它非空;
3、它作为s的子串(不是子序列)出现了最多的次数(不能为0次)。
请求出这个串(如果有多解,请输出字典序最小的那一个)。
输入描述:
串s(1 <= |s| <= 50)。s只包含数字字符,可以有前导零。
输出描述:
一个串表示答案。
无解输出-1。
示例1
输入
047
输出
4
示例2
输入
16
输出
-1
分析:直接暴力;
链接:https://www.nowcoder.com/acm/contest/70/B
来源:牛客网
题目描述
定义一个数字为幸运数字当且仅当它的所有数位都是4或者7。
比如说,47、744、4都是幸运数字而5、17、467都不是。
定义next(x)为大于等于x的第一个幸运数字。给定l,r,请求出next(l) + next(l + 1) + … + next(r - 1) + next(r)。
输入描述:
两个整数l和r (1 <= l <= r <= 1000,000,000)。
输出描述:
一个数字表示答案。
示例1
输入
2 7
输出
33
示例2
输入
7 7
输出
7
分析:打表预处理,二分搜索即可;
链接:https://www.nowcoder.com/acm/contest/70/C
来源:牛客网
题目描述
定义一个数字为幸运数字当且仅当它的所有数位都是4或者7。
比如说,47、744、4都是幸运数字而5、17、467都不是。
假设现在有一个数字d,现在想在d上重复k次操作。
假设d有n位,用d1,d2,…,dn表示。
对于每次操作,我们想要找到最小的x (x < n),使得dx=4并且dx+1=7。
如果x为奇数,那么我们把dx和dx+1都变成4;
否则,如果x为偶数,我们把dx和dx+1都变成7;
如果不存在x,那么我们不做任何修改。
现在请问k次操作以后,d会变成什么样子。
输入描述:
第一行两个整数n,k表示d的长度和操作次数。
第二行一个数表示d。数据保证不存在前导零。
1 <= n <= 100,000
0 <= k <= 1000,000,000
输出描述:
一个数字表示答案。
示例1
输入
7 4
4727447
输出
4427477
示例2
输入
4 2
4478
输出
4478
分析:K比较大,刚开始我用优先队列剪枝写,认为用不完K,结果TLE了,后来才发现447和477是个需要特判的点(改的麻烦了);
链接:https://www.nowcoder.com/acm/contest/70/F
来源:牛客网
题目描述
在一个n*n的国际象棋棋盘上有m个皇后。
一个皇后可以攻击其他八个方向的皇后(上、下、左、右、左上、右上、左下、右下)。
对于某个皇后,如果某一个方向上有其他皇后,那么这个方向对她就是不安全的。
对于每个皇后,我们都能知道她在几个方向上是不安全的。
现在我们想要求出t0,t1,…,t8,其中ti表示恰有i个方向是”不安全的”的皇后有多少个。
输入描述:
第一行两个整数n,m表示棋盘大小和皇后数量。
接下来m行每行两个整数ri,ci表示皇后坐标。
1 <= n, m <= 100,000
1 <= ri, ci <= n
数据保证没有皇后在同一个位置上。
输出描述:
一行九个整数表示答案。
空格隔开,结尾无空格
示例1
输入
8 4
4 3
4 8
6 5
1 6
输出
0 3 0 1 0 0 0 0 0
示例2
输入
10 3
1 1
1 2
1 3
输出
0 2 1 0 0 0 0 0 0
分析:暴力每个点,预处理存在的点的行列和对角线的两端值;
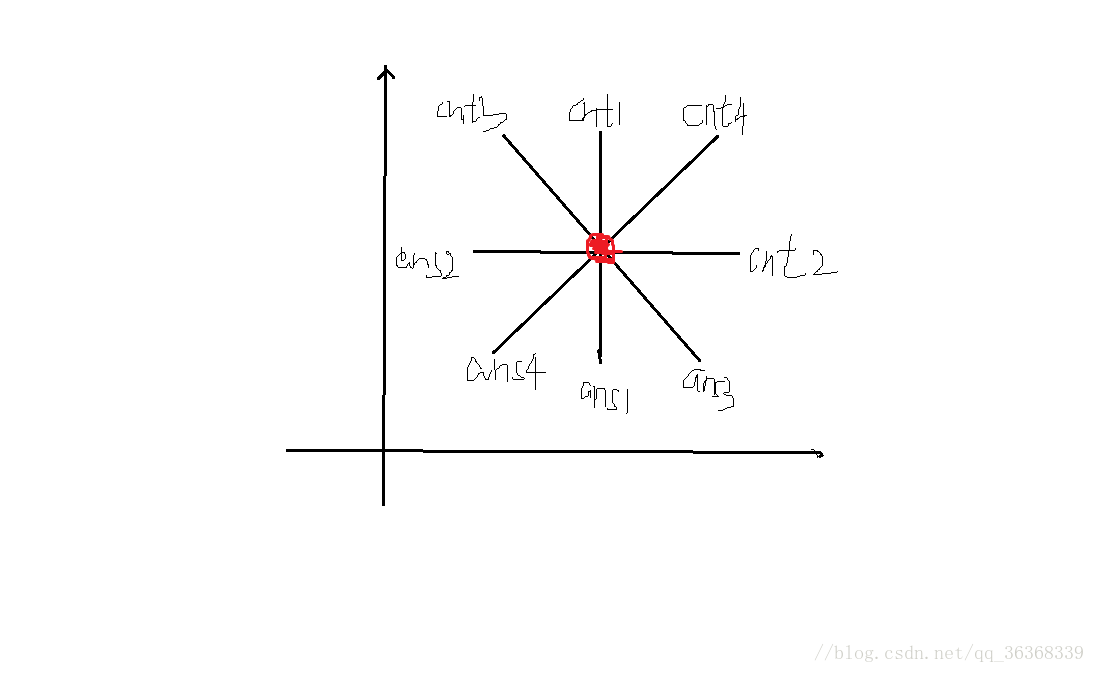
来源:牛客网
题目描述
定义一个数字为幸运数字当且仅当它的所有数位都是4或者7。
比如说,47、744、4都是幸运数字而5、17、467都不是。
现在,给定一个字符串s,请求出一个字符串,使得:
1、它所代表的整数是一个幸运数字;
2、它非空;
3、它作为s的子串(不是子序列)出现了最多的次数(不能为0次)。
请求出这个串(如果有多解,请输出字典序最小的那一个)。
输入描述:
串s(1 <= |s| <= 50)。s只包含数字字符,可以有前导零。
输出描述:
一个串表示答案。
无解输出-1。
示例1
输入
047
输出
4
示例2
输入
16
输出
-1
分析:直接暴力;
#include<bits/stdc++.h> using namespace std; typedef long long LL; const int MAXN = 50 + 10; char str[MAXN]; int main() { int n, m; scanf("%s", str); int len = strlen(str); int ans1 = 0, ans2 = 0; for(int i = 0; i < len; ++i) { if(str[i] == '4') ans1++; else if(str[i] == '7') ans2++; } if(ans1 + ans2 == 0) printf("-1\n"); else if(ans1 >= ans2) printf("4\n"); else printf("7\n"); return 0; }
链接:https://www.nowcoder.com/acm/contest/70/B
来源:牛客网
题目描述
定义一个数字为幸运数字当且仅当它的所有数位都是4或者7。
比如说,47、744、4都是幸运数字而5、17、467都不是。
定义next(x)为大于等于x的第一个幸运数字。给定l,r,请求出next(l) + next(l + 1) + … + next(r - 1) + next(r)。
输入描述:
两个整数l和r (1 <= l <= r <= 1000,000,000)。
输出描述:
一个数字表示答案。
示例1
输入
2 7
输出
33
示例2
输入
7 7
输出
7
分析:打表预处理,二分搜索即可;
#include<bits/stdc++.h> using namespace std; typedef long long LL; const LL MAXN = 5e6 + 10; LL dp[MAXN]; LL cnt = 0; inline void dfs(LL x, LL y) { if(y == 0) { dp[cnt++] = x; return ; } dfs(x * 10 + 4, y - 1); dfs(x * 10 + 7, y - 1); } inline void init() { for(LL k = 1; k <= 10; ++k) { dfs(4, k - 1); dfs(7, k - 1); } } int main() { LL l, r, sum = 0; init();sort(dp, dp + cnt); // for(int i = 0; i < 10; ++i) { // printf("%lld\n", dp[i]); // } scanf("%lld %lld", &l, &r); for(LL i = l; i <= r; i++) { LL p = lower_bound(dp, dp + cnt, i) - dp; LL ans = (min(r, dp[p]) - i + 1) * dp[p]; sum += ans; i = dp[p]; } printf("%lld\n", sum); return 0; }
链接:https://www.nowcoder.com/acm/contest/70/C
来源:牛客网
题目描述
定义一个数字为幸运数字当且仅当它的所有数位都是4或者7。
比如说,47、744、4都是幸运数字而5、17、467都不是。
假设现在有一个数字d,现在想在d上重复k次操作。
假设d有n位,用d1,d2,…,dn表示。
对于每次操作,我们想要找到最小的x (x < n),使得dx=4并且dx+1=7。
如果x为奇数,那么我们把dx和dx+1都变成4;
否则,如果x为偶数,我们把dx和dx+1都变成7;
如果不存在x,那么我们不做任何修改。
现在请问k次操作以后,d会变成什么样子。
输入描述:
第一行两个整数n,k表示d的长度和操作次数。
第二行一个数表示d。数据保证不存在前导零。
1 <= n <= 100,000
0 <= k <= 1000,000,000
输出描述:
一个数字表示答案。
示例1
输入
7 4
4727447
输出
4427477
示例2
输入
4 2
4478
输出
4478
分析:K比较大,刚开始我用优先队列剪枝写,认为用不完K,结果TLE了,后来才发现447和477是个需要特判的点(改的麻烦了);
#include<bits/stdc++.h> using namespace std; typedef long long LL; const LL MAXN = 1e5 + 10; char str[MAXN]; struct node { int a; bool friend operator<(node x, node y) { return x.a > y.a; } }e; int main() { priority_queue<node> q; int n, k; scanf("%d %d\n", &n, &k); int res = n + 1, ans = 0; scanf("%s", str); for(int i = 0; i < n; ++i) { if(str[i] == '4') { if(str[i + 1] == '4' && str[i + 2] == '7' && (i + 1) % 2 == 1) { res = min(res, i); } else if(str[i + 1] == '7' && str[i + 2] == '7' && (i + 1) % 2 == 1) { res = min(res, i); } else if(str[i + 1] == '7' && res == n + 1) { e.a = i; ans++; q.push(e); } } } for(int i = 1; i <= k && (!q.empty()); ++i) { node cnt = q.top(); q.pop(); if((cnt.a + 1) % 2 == 0) { str[cnt.a] = '7'; if(cnt.a - 1 >= 0) { if(str[cnt.a - 1] == '4') { e.a = cnt.a - 1; q.push(e); } } } else { str[cnt.a + 1] = '4'; if(cnt.a + 2 <= n) { if(str[cnt.a + 2] == '7') { e.a = cnt.a + 1; q.push(e); } } } } if(res != n + 1 && k > ans) { int cnt = k - ans; if((res + 1) % 2 == 1) { if(cnt % 2 == 1) { if(str[res + 1] == '4') str[res + 1] = '7'; else if(str[res + 1] == '7') str[res + 1] = '4'; } } } for(int i = 0; i < n; ++i) { printf("%c", str[i]); } puts(""); return 0; }
链接:https://www.nowcoder.com/acm/contest/70/F
来源:牛客网
题目描述
在一个n*n的国际象棋棋盘上有m个皇后。
一个皇后可以攻击其他八个方向的皇后(上、下、左、右、左上、右上、左下、右下)。
对于某个皇后,如果某一个方向上有其他皇后,那么这个方向对她就是不安全的。
对于每个皇后,我们都能知道她在几个方向上是不安全的。
现在我们想要求出t0,t1,…,t8,其中ti表示恰有i个方向是”不安全的”的皇后有多少个。
输入描述:
第一行两个整数n,m表示棋盘大小和皇后数量。
接下来m行每行两个整数ri,ci表示皇后坐标。
1 <= n, m <= 100,000
1 <= ri, ci <= n
数据保证没有皇后在同一个位置上。
输出描述:
一行九个整数表示答案。
空格隔开,结尾无空格
示例1
输入
8 4
4 3
4 8
6 5
1 6
输出
0 3 0 1 0 0 0 0 0
示例2
输入
10 3
1 1
1 2
1 3
输出
0 2 1 0 0 0 0 0 0
分析:暴力每个点,预处理存在的点的行列和对角线的两端值;
#include<bits/stdc++.h> using namespace std; const int MAXN = 3e5 + 10; const int MAXM = 1e5 + 10; int ans1[MAXN], ans2[MAXN], ans3[MAXN], ans4[MAXN]; int cnt1[MAXN], cnt2[MAXN], cnt3[MAXN], cnt4[MAXN]; int ans[10]; inline void init() { for(int i = 0; i < MAXN; ++i) { ans1[i] = MAXN; ans2[i] = MAXN; ans3[i] = MAXN; ans4[i] = MAXN; cnt1[i] = 0; cnt2[i] = 0; cnt3[i] = 0; cnt4[i] = 0; } } struct node { int a; int b; }s[MAXN]; int main() { int n, m; init(); scanf("%d %d", &n, &m); for(int i = 0; i < m; ++i) { scanf("%d %d", &s[i].a, &s[i].b); ans1[s[i].a] = min(ans1[s[i].a], s[i].b); cnt1[s[i].a] = max(cnt1[s[i].a], s[i].b); ans2[s[i].b] = min(ans2[s[i].b], s[i].a); cnt2[s[i].b] = max(cnt2[s[i].b], s[i].a); int res1 = s[i].a + s[i].b; int res2 = s[i].b - s[i].a + MAXM; ans3[res1] = min(ans3[res1], s[i].b); cnt3[res1] = max(cnt3[res1], s[i].b); ans4[res2] = min(ans4[res2], s[i].b); cnt4[res2] = max(cnt4[res2], s[i].b); } for(int i = 0; i < m; ++i) { int cnt = 0; if(s[i].b > ans1[s[i].a]) cnt++; if(s[i].b < cnt1[s[i].a]) cnt++; if(s[i].a > ans2[s[i].b]) cnt++; if(s[i].a < cnt2[s[i].b]) cnt++; int res1 = s[i].a + s[i].b; int res2 = s[i].b - s[i].a + MAXM; if(s[i].b > ans3[res1]) cnt++; if(s[i].b < cnt3[res1]) cnt++; if(s[i].b > ans4[res2]) cnt++; if(s[i].b < cnt4[res2]) cnt++; ans[cnt]++; } for(int i = 0; i < 9; ++i) { if(i) printf(" "); printf("%d", ans[i]); } puts(""); return 0; }
相关文章推荐
- 牛客练习赛13 幸运数字Ⅱ(BFS,DFS,二分,思路)
- 牛客练习赛13 D 题 幸运数字IV 【康拓逆展开 + 思维】
- 牛客练习赛13 B 幸运数字Ⅱ【前缀和 + 位运算 + 二分】
- 牛客练习赛13_C-幸运数字Ⅲ(思维)
- 牛客练习赛13 B 幸运数字Ⅱ 【暴力】【二分】
- 【牛客练习赛13】 A B C D【康拓展开】 E【DP or 记忆化搜索】 F 【思维】
- 牛客练习赛13-D:幸运数字Ⅳ(思维)
- 牛客练习赛13-B,幸运数字2
- 牛客练习赛13 --d(逆康拓展开)
- 牛客练习赛13 幸运数系列
- 牛客练习赛13-C题幸运数字III
- 牛客练习赛13 E 乌龟跑步 (dp)
- [nowcoder]牛客练习赛13 E 求最值
- 牛客练习赛13 A-幸运数字I
- 牛客练习赛13 B-幸运数字II
- 牛客网 牛客练习赛13 - 幸运数字Ⅱ
- 牛客网 牛客练习赛13-幸运数字Ⅰ
- 牛客练习赛10 B栈和排序【思维】
- 牛客练习赛13-A题
- 牛客练习赛13-B题(另带一份大佬的代码,细节值得学习)