静态顺序表的实现
2018-03-15 18:36
459 查看
实现对顺序表的初始化,头插,头删,尾插,尾删, 任意下标的删除, 任意下标处的的元素删除,任意下标处的元素插入,任意元素的下标返回,任意下标处的元素返回, 删除某个元素, 删除线性表中的所有元素,对线性表判空处理, 以及检查线性表有几个元素
1.定义一个线性表
所谓的线性表就是一段地址连续的存储单元一次存储数据元素的线性结构,而连续的地址空间采用数组的结构,但数组有静态的也有动态的,这里先讨论静态线性表的实现

由上图可以看出,静态线性表包括了元素以及元素的个数,因此定义一个线性表就是定义一个结构体
定义好结构体后便需要对结构体进行初始化了,对其初始化,只需要将其元素的个数设为零即可。
2.对线性表的头插及就是在保证线性表元素个数不超过MAXSIZE的前提下每次将数据插入下标为零的位置处
3.对线性表的头删就是只要线性表不为空,就将先行表的第1号下标的元素到第size - 1号元素一次向前移动
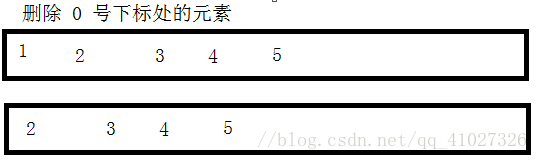
4.对线性表进行尾插,即就是先将线性表的size加1,再定义定义一个计数器让其指向线性表的结尾,每次将新元素出入其末尾即
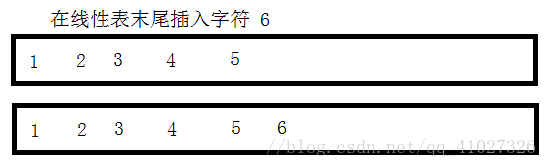
5.对线性表的尾删很简单,就是将线性表的大小size减1即可
6.对线性表的任意位置进行插入和删除
(1)首先在对其插入时必须判断线性表是否已满,以及传来的指针是否为空指针,其次,当删除的时候也必须判断线性表是否为空。当满足以上要求时,再进行插入或者删除才是合理的。在对线性表任意位置插入是及就是定义一个计数其,让其等于要插入的下标,然后将此处的元素以及后面所有的元素都向后移动,最后将需要插入的元素插入到对应的下标位置处。
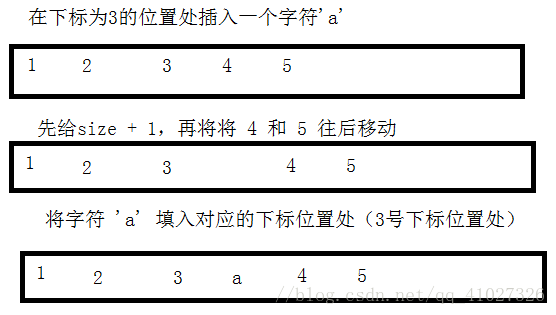
(2)对线性表任意元素的删除就是先找到这个元素,然后将这个元素后面的所有元素向前一次移动,
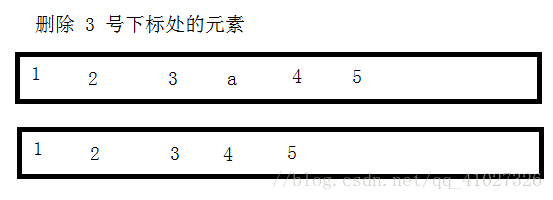
7.返回线性表中某个元素的下标即先定义一个计数器,拿着这个元素对线性表进行便利,将这个元素与线性表中的元素进行比较,如果不相等计数器加1,指针后移,直到相等的时候返回i值
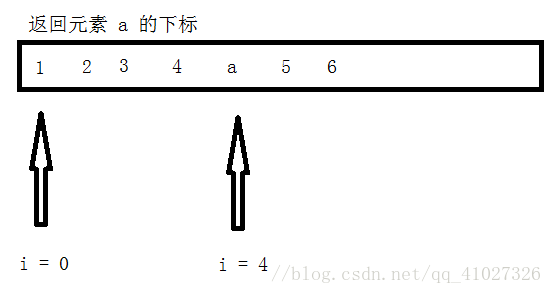
8.删除某个下标对应的元素就是定义一个指针指向该位置,然后将后面的元素一次赋值给前一个元素即可,最后将size减1
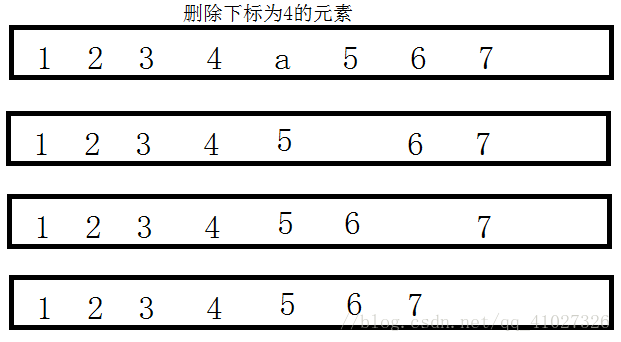
9.最后利用冒泡排序对其进行排序
10.计算线性表大小
11.查看先行表是否为空
12.设置下标为 pos 处的元素为 value
13.返回下标为 pos 的元素的值
14.整体代码
15.运行结果如下
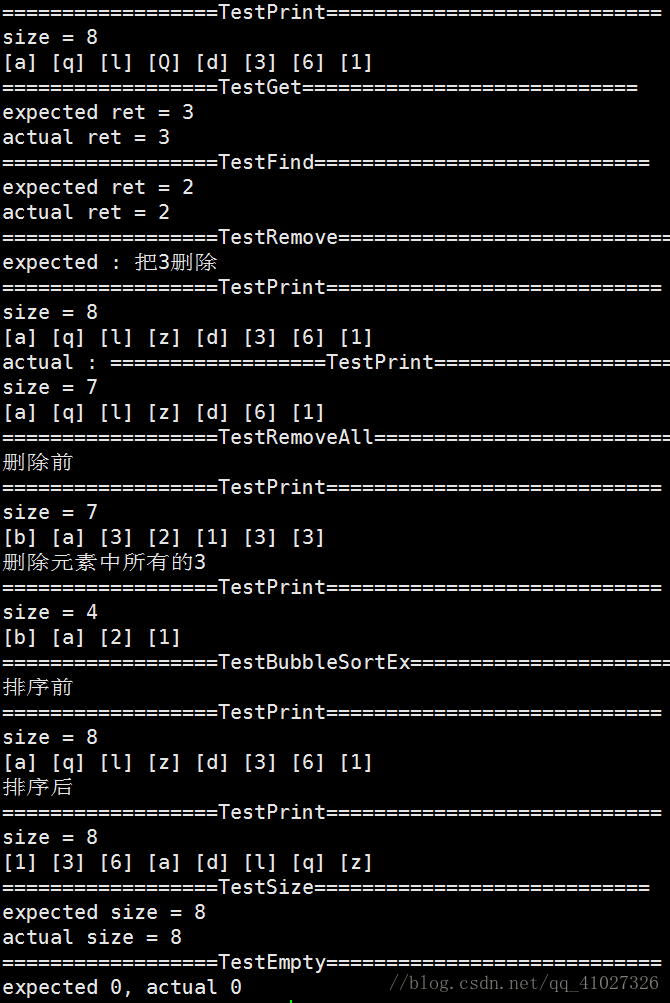
1.定义一个线性表
所谓的线性表就是一段地址连续的存储单元一次存储数据元素的线性结构,而连续的地址空间采用数组的结构,但数组有静态的也有动态的,这里先讨论静态线性表的实现
由上图可以看出,静态线性表包括了元素以及元素的个数,因此定义一个线性表就是定义一个结构体
7 typedef struct Seqlist 8 { 9 int size; 10 SeqListType data[SeqListMaxSize]; 11 }SeqList;//定义一个顺序表
定义好结构体后便需要对结构体进行初始化了,对其初始化,只需要将其元素的个数设为零即可。
11 void InitSeqList(SeqList* seqlist) 12 { 13 seqlist -> size = 0; 14 }
2.对线性表的头插及就是在保证线性表元素个数不超过MAXSIZE的前提下每次将数据插入下标为零的位置处
111 void SeqListPushFront(SeqList* seqlist, SeqListType value) 112 { 113 if(seqlist == NULL) 114 { 115 return;//非法输入 116 } 117 if(seqlist -> size == SeqListMaxSize) 118 { 119 return;//线性表已满 120 } 121 seqlist -> size++; 122 int i = 0; 123 for(i = (seqlist -> size) - 1 ; i > 0; i--) 124 { 125 seqlist -> data[i] = seqlist -> data[i - 1]; 126 } 127 seqlist -> data[0] = value; 128 }
3.对线性表的头删就是只要线性表不为空,就将先行表的第1号下标的元素到第size - 1号元素一次向前移动
153 void SeqListPopFront(SeqList* seqlist) 154 { 155 if(seqlist == NULL) 156 { 157 return; 158 } 159 if(seqlist -> size == 0) 160 { 161 return; 162 } 163 seqlist -> size--; 164 int i = 0; 165 for(i = 1; i <= seqlist -> size; i++) 166 { 167 seqlist -> data[i -1] = seqlist -> data[i]; 168 } 169 }
4.对线性表进行尾插,即就是先将线性表的size加1,再定义定义一个计数器让其指向线性表的结尾,每次将新元素出入其末尾即
22 void SeqListPushBack(SeqList* seqlist, SeqListType value) 23 { 24 if(seqlist == NULL) 25 { 26 return;//非法输入 27 } 28 if(seqlist -> size == SeqListMaxSize) 29 { 30 return;//线性表已满 31 } 32 seqlist -> size++; 33 seqlist -> data[seqlist -> size - 1] = value; 34 }
5.对线性表的尾删很简单,就是将线性表的大小size减1即可
72 void SeqListPopBash(SeqList* seqlist) 73 { 74 if(seqlist == NULL) 75 { 76 return;//非法输入 77 } 78 if(seqlist -> size == 0) 79 { 80 return;//线性表为空 81 } 82 seqlist -> size--; 83 }
6.对线性表的任意位置进行插入和删除
(1)首先在对其插入时必须判断线性表是否已满,以及传来的指针是否为空指针,其次,当删除的时候也必须判断线性表是否为空。当满足以上要求时,再进行插入或者删除才是合理的。在对线性表任意位置插入是及就是定义一个计数其,让其等于要插入的下标,然后将此处的元素以及后面所有的元素都向后移动,最后将需要插入的元素插入到对应的下标位置处。
198 void SeqListInsert(SeqList* seqlist, int pos, SeqListType value) 199 { 200 if(seqlist == NULL) 201 { 202 return;//非法输入 203 } 204 if(pos == 0) 205 { 206 SeqListPushFront(seqlist, value); 207 } 208 if(pos > seqlist -> size) 209 { 210 return; 211 } 212 seqlist -> size++; 213 int i = 0; 214 for( i = (seqlist -> size) - 1; i >= pos + 1; i--) 215 { 216 seqlist -> data[i] = seqlist -> data[i - 1]; 217 } 218 seqlist -> data[pos] = value; 219 220 }
(2)对线性表任意元素的删除就是先找到这个元素,然后将这个元素后面的所有元素向前一次移动,
428 void SeqListRemove(SeqList* seqlist, SeqListType to_remove) 429 { 430 if(seqlist == NULL) 431 { 432 return; 433 } 434 if(seqlist -> size == 0) 435 { 436 return; 437 } 438 int pos = SeqListFind(seqlist, to_remove);//找到 to_remove的下标 439 if(pos == -1) 440 { 441 return;//没有找到 442 } 443 SeqListErase(seqlist, pos); 444 }
7.返回线性表中某个元素的下标即先定义一个计数器,拿着这个元素对线性表进行便利,将这个元素与线性表中的元素进行比较,如果不相等计数器加1,指针后移,直到相等的时候返回i值
381 int SeqListFind(SeqList* seqlist, SeqListType to_find) 382 { 383 if(seqlist == NULL) 384 { 385 return -1;//非法输入 386 } 387 if(seqlist -> size == 0) 388 { 389 return -1;//非法输入 390 } 391 int i = 0; 392 for(i = 0; i < seqlist -> size; i++) 393 { 394 if(to_find == seqlist -> data[i]) 395 { 396 return i; 397 } 398 } 399 return -1; 400 }
8.删除某个下标对应的元素就是定义一个指针指向该位置,然后将后面的元素一次赋值给前一个元素即可,最后将size减1
249 void SeqListErase(SeqList* seqlist, int pos) 250 { 251 if(seqlist == NULL) 252 { 253 return; 254 } 255 if(pos < 0 || pos >= seqlist -> size) 256 { 257 return;//非法位置 258 } 259 if(seqlist -> size == 0) 260 { 261 return;//线性表为空 262 } 263 int i = 0; 264 for(i = pos; i < seqlist -> size - 1; i ++) 265 { 266 seqlist -> data[i] = seqlist -> data[i + 1]; 267 } 268 seqlist -> size--; 269 270 }
9.最后利用冒泡排序对其进行排序
518 void Swap(SeqListType* a, SeqListType* b) 519 { 520 SeqListType tmp = 0; 521 tmp = *a; 522 *a = *b; 523 *b = tmp; 524 } 576 int Greater(SeqListType* a, SeqListType* b) 577 { 578 return *a < *b; 579 } 580 581 void SeqListBubbleSortEx(SeqList* seqlist, Cmp Greater) 582 { 583 if(seqlist == NULL) 584 { 585 return;//非法输入 586 } 587 int count = 0; 588 for(count = 0; count < seqlist -> size -1; count++) 589 { 590 int current = 0; 591 for(current = 0; current < seqlist -> size -1 - count; current++) 592 { 593 if(!Greater(&seqlist -> data[current], &seqlist -> data[current + 1])) 594 { 595 Swap(&seqlist -> data[current], &seqlist -> data[current +1]); 596 } 597 598 } 599 } 600 601 }
10.计算线性表大小
631 int SeqListSize(SeqList* seqlist) 632 { 633 if(seqlist == NULL) 634 { 635 return; 636 } 637 return seqlist -> size; 638 }
11.查看先行表是否为空
676 void TestEmpty() 677 { 678 TESTHEADER; 679 SeqList seqlist; 680 InitSeqList(&seqlist); 681 SeqListPushFront(&seqlist, '1'); 682 SeqListPushFront(&seqlist, '6'); 683 SeqListPushFront(&seqlist, '3'); 684 SeqListPushFront(&seqlist, 'd'); 685 SeqListPushFront(&seqlist, 'z'); 686 SeqListPushFront(&seqlist, 'l'); 687 SeqListPushFront(&seqlist, 'q'); 688 SeqListPushFront(&seqlist, 'a'); 689 int ret = SeqListEmpty(&seqlist); 690 printf("expected 0, actual %d\n", ret); 691 692 }
12.设置下标为 pos 处的元素为 value
299 int SeqListSet(SeqList* seqlist, int pos, SeqListType value) 300 { 301 if(seqlist == NULL) 302 { 303 return 1; 304 } 305 if(pos < 0 || pos > seqlist -> size) 306 { 307 return 1; 308 } 309 seqlist -> data[pos] = value; 310 }
13.返回下标为 pos 的元素的值
340 int SeqListGet(SeqList* seqlist, int pos, SeqListType* value) 341 { 342 if(seqlist == NULL) 343 { 344 return 1; 345 } 346 if(seqlist -> size == 0) 347 { 348 return 1; 349 } 350 *value = seqlist -> data[pos]; 351 return 0; 352 }
14.整体代码
//seqlist.h文件 1 #define pragama once 2 3 #define SeqListType char 4 5 #define SeqListMaxSize 1000 6 7 typedef struct Seqlist 8 { 9 int size; 10 SeqListType data[SeqListMaxSize]; 11 }SeqList;//定义一个顺序表 12 13 typedef int(*Cmp)(SeqListType*, SeqListType*);//函数指针的定义 14 15 void SeqListInit(SeqList *seqlist);//初始化线性表 16 17 void TestPrint(SeqList *seqlist);//打印 18 19 void SeqListPushBack(SeqList* seqlist, SeqListType value);//尾插 20 21 void SeqListPopBack(SeqList* seqlist);//尾删 22 23 void SeqlistPushFront(SeqList* seqlist, SeqListType value);//头插 24 25 void SeqListPopFront(SeqList* seqlist);//头删 26 27 void SeqListInsert(SeqList* seqlist, int pos, SeqListType value);//在 pos 下标处插处 value 28 29 void SeqListErase(SeqList* seqlist, int pos);//删除 pos 下标处的元素 30 31 int SeqListSet(SeqList* seqlist, int pos, SeqListType value);//设置下标 pos 处的元素值为 value 32 33 int SeqListGet(SeqList* seqlist, int pos, SeqListType* value);//返回下标是 pos 的元素的值 34 35 int SeqListFind(SeqList* seqlist, SeqListType to_find);//返回 to_find 的下标 36 37 void SeqListRemove(SeqList* seqlist, SeqListType to_remove);//删除某个元素 to_remove 38 39 void SeqListRemoveAll(SeqList* seqlist, SeqListType to_remove);//删除线性表中所有的 to_remove 40 41 void SeqListBubbleSort(SeqList* seqlist);//对线性表的元素进行排序 42 43 void SeqListBubbleSortEx(SeqList* seqlist, Cmp Greater); 44 45 int SeqListSize(SeqList* seqlist);//获取线性表的元素个数 46 47 int SeqListEmpty(SeqList* seqlist);//判断线性表是否为空
1 #include"seqlist.h"
2 #include<stdio.h>
3
4 #define TESTHEADER printf("==================%s============================\n", __FUNCTION__)
5 /*
6 *
7 * 初始化
8 *
9 */
10
11 void InitSeqList(SeqList* seqlist)
12 {
13 seqlist -> size = 0;
14 }
15
16 /*
17 *
18 * 尾插
19 *
20 *
21 */
22 void SeqListPushBack(SeqList* seqlist, SeqListType value) 23 { 24 if(seqlist == NULL) 25 { 26 return;//非法输入 27 } 28 if(seqlist -> size == SeqListMaxSize) 29 { 30 return;//线性表已满 31 } 32 seqlist -> size++; 33 seqlist -> data[seqlist -> size - 1] = value; 34 }
35 /////////////////////////////////////////////////////////////////////////////////////
36 ///////////////////////////测试代码//////////////////////////////////////////////////
37 /////////////////////////////////////////////////////////////////////////////////////
38 void TestPushBack()
39 {
40 TESTHEADER;
41 SeqList seqlist;
42 InitSeqList(&seqlist);
43 SeqListPushBack(&seqlist, 'a');
44 SeqListPushBack(&seqlist, 'c');
45 SeqListPushBack(&seqlist, 'd');
46 SeqListPushBack(&seqlist, 'b');
47 SeqListPushBack(&seqlist, 'z');
48 SeqListPushBack(&seqlist, 'g');
49 SeqListPushBack(&seqlist, 'q');
50 TestPrint(&seqlist);
51 }
52 ///////////////////////////////////////////////////////////////////////////////////////
53 //////////////////////测试代码/////////////////////////////////////////////////////////
54 ///////////////////////////////////////////////////////////////////////////////////////
55 void TestPrint(SeqList* seqlist)
56 {
57 TESTHEADER;
58 printf("size = %d\n", seqlist -> size);
59 int i = 0;
60 for(i = 0; i < seqlist -> size; i++)
61 {
62 printf("[%c] ", seqlist -> data[i]);
63 }
64 printf("\n");
65 }
66
67 /*
68 *
69 * 尾删
70 *
71 */
72 void SeqListPopBash(SeqList* seqlist)
73 {
74 if(seqlist == NULL)
75 {
76 return;//非法输入
77 }
78 if(seqlist -> size == 0)
79 {
80 return;//线性表为空
81 }
82 seqlist -> size--;
83 }
84
85 /////////////////////////////////////////////////////////////////////////////////////
86 ////////////////////////测试代码/////////////////////////////////////////////////////
87 /////////////////////////////////////////////////////////////////////////////////////
88 void TestPopBash()
89 {
90 TESTHEADER;
91 SeqList seqlist;
92 InitSeqList(&seqlist);
93 SeqListPushBack(&seqlist, 'a');
94 SeqListPushBack(&seqlist, 'd');
95 SeqListPushBack(&seqlist, 'g');
96 SeqListPushBack(&seqlist, 't');
97 SeqListPushBack(&seqlist, 'x');
98 SeqListPushBack(&seqlist, 'z');
99 printf("尾删前\n");
100 TestPrint(&seqlist);
101 SeqListPopBash(&seqlist);
102 printf("尾删后\n");
103 TestPrint(&seqlist);
104 }
105
106 /*
107 *
108 * 头插
109 *
110 */
111 void SeqListPushFront(SeqList* seqlist, SeqListType value) 112 { 113 if(seqlist == NULL) 114 { 115 return;//非法输入 116 } 117 if(seqlist -> size == SeqListMaxSize) 118 { 119 return;//线性表已满 120 } 121 seqlist -> size++; 122 int i = 0; 123 for(i = (seqlist -> size) - 1 ; i > 0; i--) 124 { 125 seqlist -> data[i] = seqlist -> data[i - 1]; 126 } 127 seqlist -> data[0] = value; 128 }
129 /////////////////////////////////////////////////////////////////////////////////////
130 //////////////////////////////////测试代码///////////////////////////////////////////
131 /////////////////////////////////////////////////////////////////////////////////////
132
133 void TestPushFront()
134 {
135
136 TESTHEADER;
137 SeqList seqlist;
138 InitSeqList(&seqlist);
139 SeqListPushFront(&seqlist, 'a');
140 SeqListPushFront(&seqlist, 'd');
141 SeqListPushFront(&seqlist, 'g');
142 SeqListPushFront(&seqlist, 't');
143 SeqListPushFront(&seqlist, 'x');
144 SeqListPushFront(&seqlist, 'z');
145 TestPrint(&seqlist);
146 }
147
148 /*
149 *
150 * 头删
151 *
152 */
153 void SeqListPopFront(SeqList* seqlist) 154 { 155 if(seqlist == NULL) 156 { 157 return; 158 } 159 if(seqlist -> size == 0) 160 { 161 return; 162 } 163 seqlist -> size--; 164 int i = 0; 165 for(i = 1; i <= seqlist -> size; i++) 166 { 167 seqlist -> data[i -1] = seqlist -> data[i]; 168 } 169 }
170 /////////////////////////////////////////////////////////////////////////////////////
171 //////////////////////////测试代码///////////////////////////////////////////////////
172 /////////////////////////////////////////////////////////////////////////////////////
173
174 void TestPopFront()
175 {
176 TESTHEADER;
177 SeqList seqlist;
178 InitSeqList(&seqlist);
179 SeqListPushFront(&seqlist, 'r');
180 SeqListPushFront(&seqlist, 'w');
181 SeqListPushFront(&seqlist, 'q');
182 SeqListPushFront(&seqlist, 'c');
183 SeqListPushFront(&seqlist, 'z');
184 SeqListPushFront(&seqlist, 'd');
185 printf("删除前\n");
186 TestPrint(&seqlist);
187 SeqListPopFront(&seqlist);
188 printf("头删一个元素\n");
189 TestPrint(&seqlist);
190 }
191
192 /*
193 *
194 * 在 pos 处插入元素 value
195 *
196 */
197
198 void SeqListInsert(SeqList* seqlist, int pos, SeqListType value) 199 { 200 if(seqlist == NULL) 201 { 202 return;//非法输入 203 } 204 if(pos == 0) 205 { 206 SeqListPushFront(seqlist, value); 207 } 208 if(pos > seqlist -> size) 209 { 210 return; 211 } 212 seqlist -> size++; 213 int i = 0; 214 for( i = (seqlist -> size) - 1; i >= pos + 1; i--) 215 { 216 seqlist -> data[i] = seqlist -> data[i - 1]; 217 } 218 seqlist -> data[pos] = value; 219 220 }
221
222 /////////////////////////////////////////////////////////////////////////////////////
223 //////////////////////////////测试代码///////////////////////////////////////////////
224 /////////////////////////////////////////////////////////////////////////////////////
225 void TestInsert()
226 {
227 TESTHEADER;
228 SeqList seqlist;
229 InitSeqList(&seqlist);
230 SeqListPushFront(&seqlist, 'r');
231 SeqListPushFront(&seqlist, 'w');
232 SeqListPushFront(&seqlist, 'q');
233 SeqListPushFront(&seqlist, 'c');
234 SeqListPushFront(&seqlist, 'z');
235 SeqListPushFront(&seqlist, 'd');
236 printf("插入前\n");
237 TestPrint(&seqlist);
238 SeqListInsert(&seqlist, 4, 'A');
239 printf("在下标为4的位置插入一个元素\n");
240 TestPrint(&seqlist);
241 }
242
243 /*
244 *
245 * 删除下标为 pos 的元素
246 *
247 */
248
249 void SeqListErase(SeqList* seqlist, int pos) 250 { 251 if(seqlist == NULL) 252 { 253 return; 254 } 255 if(pos < 0 || pos >= seqlist -> size) 256 { 257 return;//非法位置 258 } 259 if(seqlist -> size == 0) 260 { 261 return;//线性表为空 262 } 263 int i = 0; 264 for(i = pos; i < seqlist -> size - 1; i ++) 265 { 266 seqlist -> data[i] = seqlist -> data[i + 1]; 267 } 268 seqlist -> size--; 269 270 }
271
272 /////////////////////////////////////////////////////////////////////////////////////
273 //////////////////////////////测试代码///////////////////////////////////////////////
274 /////////////////////////////////////////////////////////////////////////////////////
275 void TestErase()
276 {
277 TESTHEADER;
278 SeqList seqlist;
279 InitSeqList(&seqlist);
280 SeqListPushFront(&seqlist, '1');
281 SeqListPushFront(&seqlist, '6');
282 SeqListPushFront(&seqlist, '3');
283 SeqListPushFront(&seqlist, 'd');
284 SeqListPushFront(&seqlist, 'z');
285 SeqListPushFront(&seqlist, 'l');
286 SeqListPushFront(&seqlist, 'q');
287 SeqListPushFront(&seqlist, 'a');
288 TestPrint(&seqlist);
289 printf("删除下标为3的元素\n");
290 SeqListErase(&seqlist, 3);
291 TestPrint(&seqlist);
292 }
293
294 /*
295 *
296 * 设置下标为 pos 处的元素为 value
297 *
298 */
299 int SeqListSet(SeqList* seqlist, int pos, SeqListType value) 300 { 301 if(seqlist == NULL) 302 { 303 return 1; 304 } 305 if(pos < 0 || pos > seqlist -> size) 306 { 307 return 1; 308 } 309 seqlist -> data[pos] = value; 310 }
311
312 /////////////////////////////////////////////////////////////////////////////////////
313 //////////////////////////测试代码///////////////////////////////////////////////////
314 /////////////////////////////////////////////////////////////////////////////////////
315
316 void TestSet()
317 {
318 TESTHEADER;
319 SeqList seqlist;
320 InitSeqList(&seqlist);
321 SeqListPushFront(&seqlist, '1');
322 SeqListPushFront(&seqlist, '6');
323 SeqListPushFront(&seqlist, '3');
324 SeqListPushFront(&seqlist, 'd');
325 SeqListPushFront(&seqlist, 'z');
326 SeqListPushFront(&seqlist, 'l');
327 SeqListPushFront(&seqlist, 'q');
328 SeqListPushFront(&seqlist, 'a');
329 printf("设置前\n");
330 TestPrint(&seqlist);
331 SeqListSet(&seqlist, 3, 'Q');
332 printf("设置后 : data[3] = 'Q'\n");
333 TestPrint(&seqlist);
334 }
335 /*
336 *
337 * 返回下标为 pos 的元素的值
338 *
339 */
340 int SeqListGet(SeqList* seqlist, int pos, SeqListType* value) 341 { 342 if(seqlist == NULL) 343 { 344 return 1; 345 } 346 if(seqlist -> size == 0) 347 { 348 return 1; 349 } 350 *value = seqlist -> data[pos]; 351 return 0; 352 }
353 /////////////////////////////////////////////////////////////////////////////////////
354 /////////////////////////////测试代码////////////////////////////////////////////////
355 /////////////////////////////////////////////////////////////////////////////////////
356
357 void TestGet()
358 {
359 TESTHEADER;
360 SeqList seqlist;
361 InitSeqList(&seqlist);
362 SeqListPushFront(&seqlist, '1');
363 SeqListPushFront(&seqlist, '6');
364 SeqListPushFront(&seqlist, '3');
365 SeqListPushFront(&seqlist, 'd');
366 SeqListPushFront(&seqlist, 'z');
367 SeqListPushFront(&seqlist, 'l');
368 SeqListPushFront(&seqlist, 'q');
369 SeqListPushFront(&seqlist, 'a');
370 SeqListType value = 0;
371 SeqListGet(&seqlist, 5, &value);
372 printf("expected ret = 3\n");
373 printf("actual ret = %c\n", value);
374
375 }
376 /*
377 *
378 *找元素 to_find 并且返回其下标
379 *
380 */
381 int SeqListFind(SeqList* seqlist, SeqListType to_find) 382 { 383 if(seqlist == NULL) 384 { 385 return -1;//非法输入 386 } 387 if(seqlist -> size == 0) 388 { 389 return -1;//非法输入 390 } 391 int i = 0; 392 for(i = 0; i < seqlist -> size; i++) 393 { 394 if(to_find == seqlist -> data[i]) 395 { 396 return i; 397 } 398 } 399 return -1; 400 }
401 ///////////////////////////////////////////////////////////////////////////////
402 ///////////////////测试代码////////////////////////////////////////////////////
403 ///////////////////////////////////////////////////////////////////////////////
404 void TestFind()
405 {
406 TESTHEADER;
407 SeqList seqlist;
408 InitSeqList(&seqlist);
409 SeqListPushFront(&seqlist, '1');
410 SeqListPushFront(&seqlist, '6');
411 SeqListPushFront(&seqlist, '3');
412 SeqListPushFront(&seqlist, 'd');
413 SeqListPushFront(&seqlist, 'z');
414 SeqListPushFront(&seqlist, 'l');
415 SeqListPushFront(&seqlist, 'q');
416 SeqListPushFront(&seqlist, 'a');
417 int ret = SeqListFind(&seqlist, 'l');
418 printf("expected ret = 2\n");
419 printf("actual ret = %d\n", ret);
420 }
421
422 /*
423 *
424 * 删除某个元素 to_remove
425 *
426 */
427
428 void SeqListRemove(SeqList* seqlist, SeqListType to_remove) 429 { 430 if(seqlist == NULL) 431 { 432 return; 433 } 434 if(seqlist -> size == 0) 435 { 436 return; 437 } 438 int pos = SeqListFind(seqlist, to_remove);//找到 to_remove的下标 439 if(pos == -1) 440 { 441 return;//没有找到 442 } 443 SeqListErase(seqlist, pos); 444 }
445
446 ////////////////////////////////////////////////////////////////////////////////
447 ///////////////////////测试代码/////////////////////////////////////////////////
448 ////////////////////////////////////////////////////////////////////////////////
449 void TestRemove()
450 {
451 TESTHEADER;
452 SeqList seqlist;
453 InitSeqList(&seqlist);
454 SeqListPushFront(&seqlist, '1');
455 SeqListPushFront(&seqlist, '6');
456 SeqListPushFront(&seqlist, '3');
457 SeqListPushFront(&seqlist, 'd');
458 SeqListPushFront(&seqlist, 'z');
459 SeqListPushFront(&seqlist, 'l');
460 SeqListPushFront(&seqlist, 'q');
461 SeqListPushFront(&seqlist, 'a');
462 printf("expected : 把3删除\n");
463 TestPrint(&seqlist);
464 printf("actual : ");
465 SeqListRemove(&seqlist, '3');
466 TestPrint(&seqlist);
467 }
468
469 /*
470 *
471 * 删除所有 to_remove 的元素
472 *
473 */
474 void SeqListRemoveAll(SeqList* seqlist, SeqListType to_remove)
475 {
476
477 if(seqlist == NULL)
478 {
479 return;//非法输入
480 }
481 if(seqlist -> size == 0)
482 {
483 return;//线性表为空
484 }
485 int pos = 0;
486 while(1)
487 {
488 pos = SeqListFind(seqlist, to_remove);
489 if(pos == -1)
490 {
491 return;
492 }
493 SeqListErase(seqlist, pos);
494 }
495 }
496
497 ////////////////////////////////////////////////////////////////////////////////////////
498 ////////////////////////////测试代码////////////////////////////////////////////////////
499 ////////////////////////////////////////////////////////////////////////////////////////
500 void TestRemoveAll()
501 {
502 TESTHEADER;
503 SeqList seqlist;
504 InitSeqList(&seqlist);
505 SeqListPushFront(&seqlist, '3');
506 SeqListPushFront(&seqlist, '3');
507 SeqListPushFront(&seqlist, '1');
508 SeqListPushFront(&seqlist, '2');
509 SeqListPushFront(&seqlist, '3');
510 SeqListPushFront(&seqlist, 'a');
511 SeqListPushFront(&seqlist, 'b');
512 printf("删除前\n");
513 TestPrint(&seqlist);
514 printf("删除元素中所有的3\n");
515 SeqListRemoveAll(&seqlist, '3');
516 TestPrint(&seqlist);
517 }
518 void Swap(SeqListType* a, SeqListType* b)
519 {
520 SeqListType tmp = 0;
521 tmp = *a;
522 *a = *b;
523 *b = tmp;
524 }
525 /*
526 *
527 * 排序
528 *
529 *
530 */
531 void SeqListBubbleSort(SeqList* seqlist)
532 {
533 if(seqlist == NULL)
534 {
535 return;
536 }
537 int count = 0;
538 for(count = 0; count < seqlist -> size -1; count++)
539 {
540 int current = 0 ;
541 for(current = 0; current < seqlist -> size -count - 1; current++)
542 {
543 if(seqlist -> data[current] > seqlist -> data[current + 1])
544 {
545 Swap(&seqlist -> data[current], &seqlist -> data[current + 1]);
546 }
547 }
548 }
549 }
550 ////////////////////////////////////////////////////////////////////////////////////////////////////////
551 ///////////////////////////////测试代码/////////////////////////////////////////////////////////////////
552 ////////////////////////////////////////////////////////////////////////////////////////////////////////
553 void TestBubbleSort()
554 {
555 TESTHEADER;
556 SeqList seqlist;
557 InitSeqList(&seqlist);
558 SeqListPushFront(&seqlist, '1');
559 SeqListPushFront(&seqlist, '6');
560 SeqListPushFront(&seqlist, '3');
561 SeqListPushFront(&seqlist, 'd');
562 SeqListPushFront(&seqlist, 'z');
563 SeqListPushFront(&seqlist, 'l');
564 SeqListPushFront(&seqlist, 'q');
565 SeqListPushFront(&seqlist, 'a');
566 TestPrint(&seqlist);
567 SeqListBubbleSort(&seqlist);
568 TestPrint(&seqlist);
569 }
570
571 /*
572 *
573 * 利用函数指针实现排序
574 *
575 */
576 int Greater(SeqListType* a, SeqListType* b)
577 {
578 return *a < *b;
579 }
580
581 void SeqListBubbleSortEx(SeqList* seqlist, Cmp Greater)
582 {
583 if(seqlist == NULL)
584 {
585 return;//非法输入
586 }
587 int count = 0;
588 for(count = 0; count < seqlist -> size -1; count++)
589 {
590 int current = 0;
591 for(current = 0; current < seqlist -> size -1 - count; current++)
592 {
593 if(!Greater(&seqlist -> data[current], &seqlist -> data[current + 1]))
594 {
595 Swap(&seqlist -> data[current], &seqlist -> data[current +1]);
596 }
597
598 }
599 }
600
601 }
602 ///////////////////////////////////////////////////////////////////////////////////////////////////////
603 //////////////////////////////////测试代码//////////////////////////////////////////////////////////
604////////////////////////////////////////////////////////////////////////////////////////////////////
605
606 void TestBubbleSortEx()
607 {
608 TESTHEADER;
609 SeqList seqlist;
610 InitSeqList(&seqlist);
611 SeqListPushFront(&seqlist, '1');
612 SeqListPushFront(&seqlist, '6');
613 SeqListPushFront(&seqlist, '3');
614 SeqListPushFront(&seqlist, 'd');
615 SeqListPushFront(&seqlist, 'z');
616 SeqListPushFront(&seqlist, 'l');
617 SeqListPushFront(&seqlist, 'q');
618 SeqListPushFront(&seqlist, 'a');
619 printf("排序前\n");
620 TestPrint(&seqlist);
621 printf("排序后\n");
622 SeqListBubbleSortEx(&seqlist, Greater);
623 TestPrint(&seqlist);
624 }
625
626 /*
627 *
628 * 获取线性表元素的个数
629 *
630 */
631 int SeqListSize(SeqList* seqlist) 632 { 633 if(seqlist == NULL) 634 { 635 return; 636 } 637 return seqlist -> size; 638 }
639
640 //////////////////////////////////////////////////////////////////////////////////////////////////////
641 //////////////////////////测试代码////////////////////////////////////////////////////////////////////
642 //////////////////////////////////////////////////////////////////////////////////////////////////////
643
644 void TestSize()
645 {
646 TESTHEADER;
647 SeqList seqlist;
648 InitSeqList(&seqlist);
649 SeqListPushFront(&seqlist, '1');
650 SeqListPushFront(&seqlist, '6');
651 SeqListPushFront(&seqlist, '3');
652 SeqListPushFront(&seqlist, 'd');
653 SeqListPushFront(&seqlist, 'z');
654 SeqListPushFront(&seqlist, 'l');
655 SeqListPushFront(&seqlist, 'q');
656 SeqListPushFront(&seqlist, 'a');
657 printf("expected size = 8\n");
658 int size = SeqListSize(&seqlist);
659 printf("actual size = %d\n", size);
660 }
661
662 /*
663 *
664 * 判断线性表是否为空为空返回 1,不为空返回 0
665 *
666 */
667 int SeqListEmpty(SeqList* seqlist)
668 {
669 return seqlist -> size ? 0 : 1;
670 }
671
672 /////////////////////////////////////////////////////////////////////////////////////////////
673 /////////////////////////////////////测试代码////////////////////////////////////////////////
674 /////////////////////////////////////////////////////////////////////////////////////////////
675
676 void TestEmpty() 677 { 678 TESTHEADER; 679 SeqList seqlist; 680 InitSeqList(&seqlist); 681 SeqListPushFront(&seqlist, '1'); 682 SeqListPushFront(&seqlist, '6'); 683 SeqListPushFront(&seqlist, '3'); 684 SeqListPushFront(&seqlist, 'd'); 685 SeqListPushFront(&seqlist, 'z'); 686 SeqListPushFront(&seqlist, 'l'); 687 SeqListPushFront(&seqlist, 'q'); 688 SeqListPushFront(&seqlist, 'a'); 689 int ret = SeqListEmpty(&seqlist); 690 printf("expected 0, actual %d\n", ret); 691 692 }
693 int main()
694 {
695 TestPushFront();
696 TestPushBack();
697 TestErase();
698 TestInsert();
699 TestPopFront();
700 TestPopBash();
701 TestSet();
702 TestGet();
703 TestFind();
704
705 TestRemove();
706 TestRemoveAll();
707
708
709 //TestBubbleSort();
710
711 TestBubbleSortEx();
712
713 TestSize();
714
715 TestEmpty();
716 return 0;
717 }
15.运行结果如下
相关文章推荐
- 实现静态顺序表和动态顺序表
- 动态顺序表的代码实现以及与静态代码的区别
- 实现静态顺序表的相关函数
- 顺序表——简单实现(静态数组)
- 静态顺序表的实现(C语言)
- 顺序表的静态存储c语言实现
- 静态和动态顺序表的实现(c语言)
- (五)数据结构之静态查找的简单实现:顺序查找和二分查找
- C语言:静态顺序表的实现和相关操作
- 静态顺序表(C语言实现)
- c语言实现静态顺序表 ------------第一次自己独立完成
- C实现静态顺序表(增删查改基本函数+选择排序+冒泡排序+二分查找)
- 静态顺序表的实现
- 静态顺序表的c实现
- 顺序表的静态和动态实现
- 【C语言】静态顺序表的实现(包括头插、头删、尾插、尾删、查找、删除指定位置)
- 静态顺序表的实现(2)
- 【C语言】实现静态顺序表
- c语言静态顺序表的相关功能实现
- C++实现静态顺序表的增删查改