Java程序:迷宫地图生成器
2018-02-18 20:41
288 查看
1、运行效果
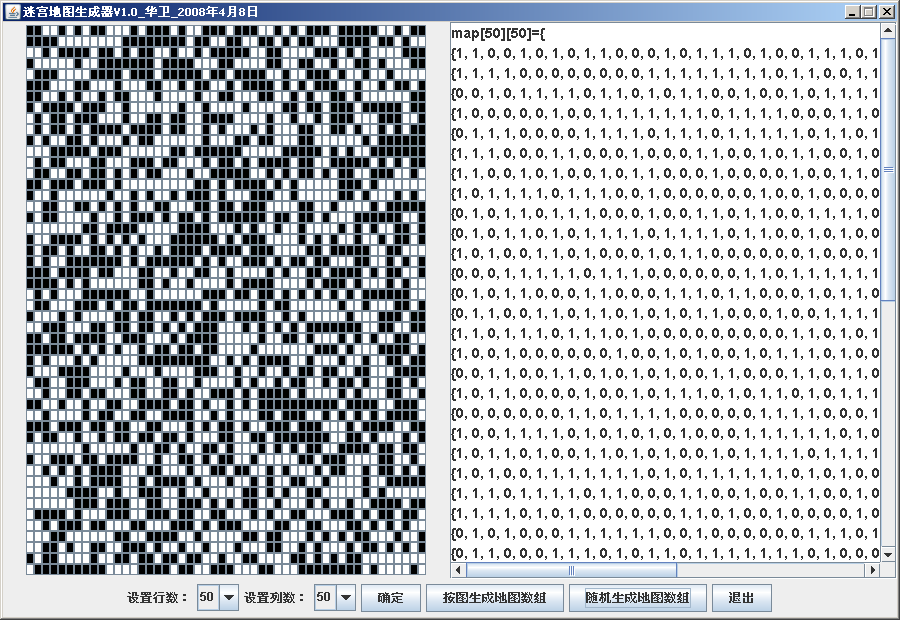
可以在【0,50】之间随意设置行数和列数,比如设置为25行25列的迷宫地图数组。
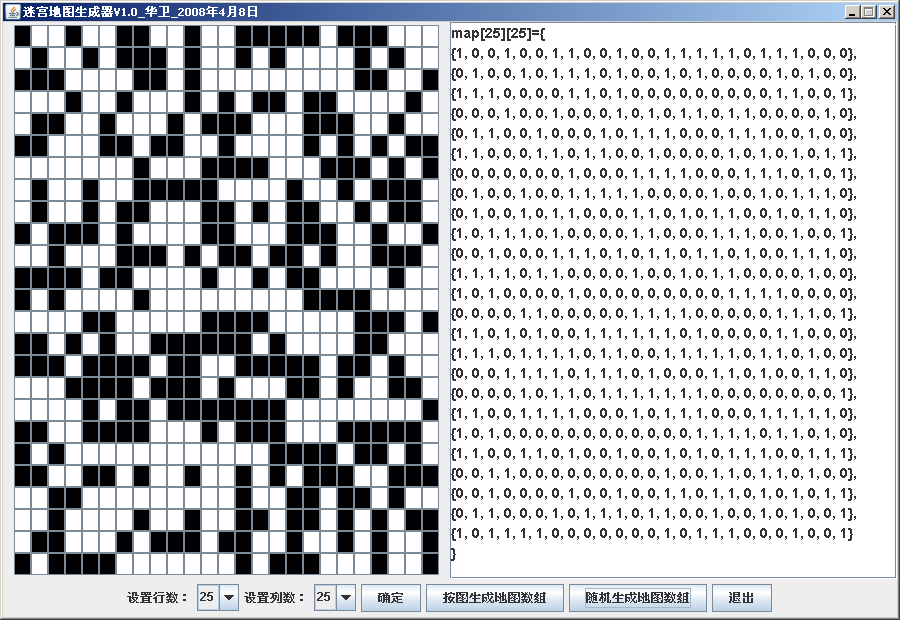
迷宫地图的每一个方格,如果是白色,单击就变成黑色,如果是黑色,单击就变成白色。黑色对应数组里的1,白色对应数组里的0。因为没有采用路径搜索算法来设置有效的迷宫地图(存在从左上角到右下角的路径),所以需要“随机”和“人工”相结合才能得到一个有效的迷宫地图数组。
2、Java源代码
可以在【0,50】之间随意设置行数和列数,比如设置为25行25列的迷宫地图数组。
迷宫地图的每一个方格,如果是白色,单击就变成黑色,如果是黑色,单击就变成白色。黑色对应数组里的1,白色对应数组里的0。因为没有采用路径搜索算法来设置有效的迷宫地图(存在从左上角到右下角的路径),所以需要“随机”和“人工”相结合才能得到一个有效的迷宫地图数组。
2、Java源代码
/** 功能:迷宫地图生成器 作者:华卫 日期:2008年4月8日 */ import java.awt.BorderLayout; import java.awt.Color; import java.awt.Font; import java.awt.GridLayout; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.WindowAdapter; import java.awt.event.WindowEvent; import java.util.Random; import javax.swing.JButton; import javax.swing.JComboBox; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; import javax.swing.JScrollPane; import javax.swing.JTextArea; import javax.swing.ScrollPaneConstants; public class MapGenerator extends JFrame implements ActionListener { /** * 常量、变量定义部分 */ static MapGenerator mg; JPanel backPanel, centerPanel, leftPanel, rightPanel, bottomPanel; JButton btnOK, btnGenerate, btnRandom, btnExit; JButton[][] btnBlock; JScrollPane scrollPane; JTextArea txtMap; JLabel lblRow, lblCol; StringBuffer buffer; JComboBox rowList, colList; boolean[][] m_bSelected; int[][] map; int ROW, COL; public static void main(String arg[]) { mg = new MapGenerator("迷宫地图生成器V1.0_华卫_2008年4月8日"); } public void setRow(int row) { ROW = row; } public void setCol(int col) { COL = col; } public void initialize() { /** * 设计地图生成器界面 * */ if (ROW == 0) { ROW = 50; } if (COL == 0) { COL = 50; } backPanel = new JPanel(new BorderLayout()); centerPanel = new JPanel(new GridLayout(1, 2)); leftPanel = new JPanel(new GridLayout(ROW, COL)); rightPanel = new JPanel(new GridLayout(1, 1)); bottomPanel = new JPanel(); rowList = new JComboBox(); for (int i = 3; i <= 50; i++) { rowList.addItem(String.valueOf(i)); } colList = new JComboBox(); for (int i = 3; i <= 50; i++) { colList.addItem(String.valueOf(i)); } rowList.setSelectedIndex(ROW - 3); colList.setSelectedIndex(COL - 3); btnOK = new JButton("确定"); btnGenerate = new JButton("按图生成地图数组"); btnRandom = new JButton("随机生成地图数组"); btnBlock = new JButton[ROW][COL]; btnExit = new JButton("退出"); m_bSelected = new boolean[ROW][COL]; map = new int[ROW][COL]; lblRow = new JLabel("设置行数:"); lblCol = new JLabel("设置列数:"); txtMap = new JTextArea(""); txtMap.setEditable(false); txtMap.setFont(new Font("Gorgia", Font.BOLD, 14)); scrollPane = new JScrollPane(txtMap, ScrollPaneConstants.VERTICAL_SCROLLBAR_AS_NEEDED, ScrollPaneConstants.HORIZONTAL_SCROLLBAR_AS_NEEDED); this.setContentPane(backPanel); backPanel.add(centerPanel, "Center"); backPanel.add(bottomPanel, "South"); centerPanel.add(leftPanel); centerPanel.add(rightPanel); for (int i = 0; i < ROW; i++) { for (int j = 0; j < COL; j++) { btnBlock[i][j] = new JButton(""); btnBlock[i][j].setBackground(Color.WHITE); leftPanel.add(btnBlock[i][j]); map[i][j] = 0; } } rightPanel.add(scrollPane); bottomPanel.add(lblRow); bottomPanel.add(rowList); bottomPanel.add(lblCol); bottomPanel.add(colList); bottomPanel.add(btnOK); bottomPanel.add(btnGenerate); bottomPanel.add(btnRandom); bottomPanel.add(btnExit); // 设置窗口 this.setSize(900, 620); this.setResizable(false); this.setLocationRelativeTo(null); // 让窗口在屏幕居中 // 将窗口设置为可见的 this.setVisible(true); // 注册监听器 this.addWindowListener(new WindowAdapter() { public void windowClosed(WindowEvent e) { System.exit(0); } }); btnOK.addActionListener(this); btnGenerate.addActionListener(this); btnRandom.addActionListener(this); btnExit.addActionListener(this); for (int i = 0; i < ROW; i++) { for (int j = 0; j < COL; j++) { btnBlock[i][j].addActionListener(this); } } } public MapGenerator(String name) { super(name); initialize(); } public void actionPerformed(ActionEvent e) { buffer = new StringBuffer(); if (e.getSource() == btnGenerate) { buffer.append("map[" + ROW + "][" + COL + "]={\n"); for (int i = 0; i < ROW; i++) { buffer.append("{"); for (int j = 0; j < COL; j++) { if (j < COL - 1) { buffer.append(map[i][j] + ", "); } else if (i != ROW - 1) { buffer.append(map[i][j] + "}, "); } else { buffer.append(map[i][j] + "}"); } } buffer.append("\n"); } buffer.append("}"); txtMap.setText(buffer.toString()); } else if (e.getSource() == btnRandom) { Random r = new Random(); buffer.append("map[" + ROW + "][" + COL + "]={\n"); for (int i = 0; i < ROW; i++) { buffer.append("{"); for (int j = 0; j < COL; j++) { int k = Math.abs(r.nextInt()) % 2; if (j < COL - 1) { buffer.append(k + ", "); } else if (i != ROW - 1) { buffer.append(k + "}, "); } else { buffer.append(k + "}"); } if (k == 1) { btnBlock[i][j].setBackground(Color.BLACK); map[i][j] = 1; m_bSelected[i][j] = true; } else { btnBlock[i][j].setBackground(Color.WHITE); map[i][j] = 0; m_bSelected[i][j] = false; } } buffer.append("\n"); } buffer.append("}"); txtMap.setText(buffer.toString()); } else if (e.getSource() == btnOK) { mg.setRow(rowList.getSelectedIndex() + 3); mg.setCol(colList.getSelectedIndex() + 3); initialize(); } else if (e.getSource() == btnExit) { System.exit(0); } else { for (int i = 0; i < ROW; i++) { for (int j = 0; j < COL; j++) { if (e.getSource() == btnBlock[i][j]) { m_bSelected[i][j] = !m_bSelected[i][j]; if (m_bSelected[i][j]) { btnBlock[i][j].setBackground(Color.BLACK); map[i][j] = 1; } else { btnBlock[i][j].setBackground(Color.WHITE); map[i][j] = 0; } break; } } } } } }
相关文章推荐
- 我第一天写博客,把以前的程序放上去(用Java写的迷宫)
- Java实现的推箱子游戏,带源码下载地址和地图生成程序
- ArcGIS for Java_Flex程序中浏览自己的ArcGIS Server 9.3中发布的地图
- Java程序StringBuilder的效率,验证字符串操作和字符串生成器的操作效率
- AR增强现实专用汉明码生成器+JAVA核心代码+程序
- 一个矩阵存储的迷宫地图,可行走的点是0,不可走的点是1,程序输出一条可以行走的路径
- 一个求迷宫入口到出口最近距离的程序 JAVA版本
- 我的第一个Java程序
- Java IO 系列----Java程序与其他进程的数据通信
- 【Java】使用程序调用控制tomcat启动
- 通过SmartInvoke运用java与flex轻松构建cs程序(捕获鼠标键盘事件)
- [图]iPod Touch也被破解,正在运行邮件,地图等程序
- Java程序打包成可执行jar的方法
- 写java程序最容易犯的21种错误 选择自 baggio785 的 Blog
- 决策生成器的java实现
- java小程序,12345变54321
- 保护你的JAVA / .net 程序,终极避免被反编译方法研究
- 小程序-----JAVA代码实现文件内容的复制
- crontab定时任务Java程序不执行问题
- Java多线程、断点下载程序