Java语言程序设计 第十四章 (14.1、14.2、14.3、14.4、12.5、14.6)
2018-01-16 21:50
459 查看
程序小白,希望和大家多交流,共同学习
因为没有第十版的汉语电子书,(有的汉语版是第八版,使用的还是Swing)这部分内容只能使用英语版截屏。
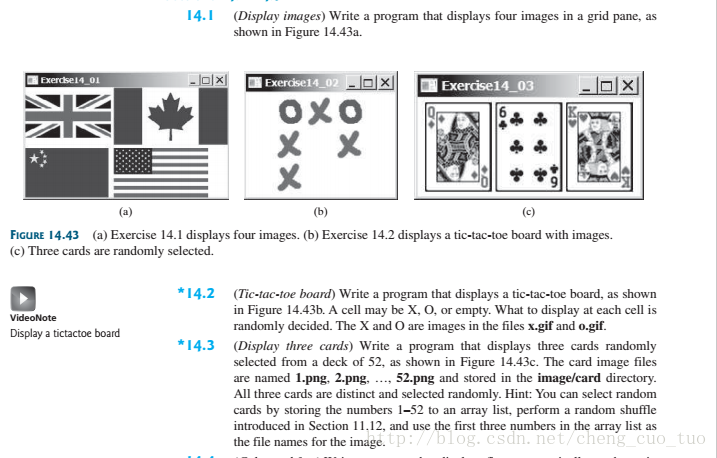
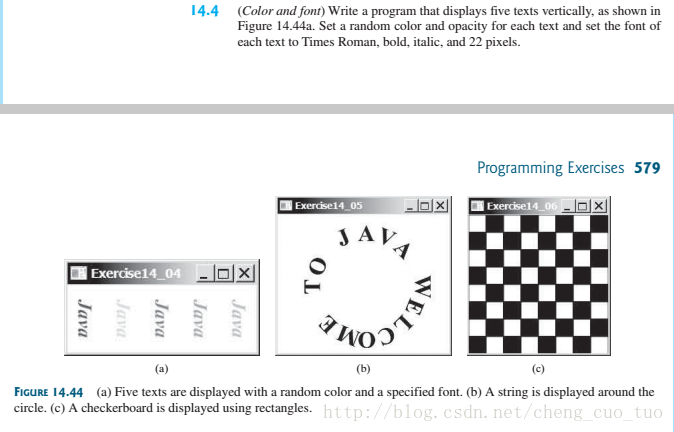

14.1
14.2
14.3
14.4_1
14.4_2
14.4_3
14.5
15.6
因为没有第十版的汉语电子书,(有的汉语版是第八版,使用的还是Swing)这部分内容只能使用英语版截屏。
14.1
//将image文件夹中的照片加载出来 import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.image.ImageView; import javafx.geometry.Insets; import javafx.scene.layout.GridPane; public class ShowFlag extends Application { @Override public void start(Stage primaryStage) { GridPane pane = new GridPane(); pane.setPadding(new Insets(5, 5, 5, 5)); pane.setHgap(10); pane.setVgap(10); ImageView p1 = new ImageView("image/flag5.gif"); ImageView p2 = new ImageView("image/flag3.gif"); ImageView p3 = new ImageView("image/flag2.gif"); ImageView p4 = new ImageView("image/flag6.gif"); pane.add(p1, 0, 0); pane.add(p2, 1, 0); pane.add(p3, 0, 1); pane.add(p4, 1, 1); Scene scene = new Scene(pane); primaryStage.setTitle("ShowFlag"); primaryStage.setScene(scene); primaryStage.show(); } }
14.2
import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.image.ImageView; import javafx.scene.layout.GridPane; import javafx.geometry.Insets; public class WellChessboard extends Application { @Override public void start(Stage primaryStage) { GridPane pane = new GridPane(); pane.setPadding(new Insets(8, 8, 8, 8)); pane.setHgap(8); pane.setVgap(8); 10345 for (int column = 0; column < 3; column++) { for (int row = 0; row < 3; row++) { int i = (int)(Math.random() * 3); if (i != 2) { pane.add(getNode(i), column, row); } } } Scene scene = new Scene(pane); primaryStage.setTitle("WellChessboard"); primaryStage.setScene(scene); primaryStage.show(); } //随机生成井字游戏中的操作 public ImageView getNode(int i) { if (i == 0) { return new ImageView("image/o.gif"); } else { return new ImageView("image/x.gif"); } } }
14.3
//随机显示一副扑克牌中任意三张 //采取的随机方式:数组转列表,列表调用Collections.shuffle()方法 import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.layout.HBox; import javafx.geometry.Insets; import javafx.scene.image.ImageView; import java.util.Arrays; import java.util.ArrayList; import java.util.Collections; public class ShowCard extends Application { @Override public void start(Stage primaryStage) { HBox pane = new HBox(5); pane.setPadding(new Insets(5, 5, 5, 5)); Integer[] array = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52}; ArrayList<Integer> list = new ArrayList<>(Arrays.asList(array)); Collections.shuffle(list); ImageView p1 = new ImageView("image/card/" + Integer.valueOf(list.get(0)) + ".png"); ImageView p2 = new ImageView("image/card/" + Integer.valueOf(list.get(1)) + ".png"); ImageView p3 = new ImageView("image/card/" + Integer.valueOf(list.get(2)) + ".png"); pane.getChildren().addAll(p1, p2, p3); Scene scene = new Scene(pane); primaryStage.setTitle("ShowCard"); primaryStage.setScene(scene); primaryStage.show(); } }
14.4_1
//多次显示一旋转后的字符串 //使用方法就是直接将Label旋转 //但是由于中心不好找,要将边距设置好 import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.layout.HBox; import javafx.scene.paint.Color; import javafx.scene.control.Label; import javafx.scene.text.Font; import javafx.scene.text.FontWeight; import javafx.scene.text.FontPosture; import javafx.geometry.Insets; public class ShowLabel_Java extends Application { @Override public void start(Stage primaryStage) { HBox pane = new HBox(10); pane.setPadding(new Insets(15, 15, 15, 15)); for (int i = 0; i < 5; i++) { Label write = new Label("Java"); write.setFont(Font.font("TimesRomes", FontWeight.BOLD, FontPosture.ITALIC, 22)); write.setRotate(90); //这个设置可以有效的防止旋转后的图形超过面 HBox.setMargin(write, new Insets(15, 0, 15, 0)); //设置Label中字体的颜色 write.setTextFill(Color.color(Math.random(), Math.random(), Math.random(), Math.random())); pane.getChildren().add(write); } Scene scene = new Scene(pane); primaryStage.setTitle("ShowLabel"); primaryStage.setScene(scene); primaryStage.show(); } }
14.4_2
//多次显示一旋转后的字符串 //使用VBox将字一一旋转后,再放在HBox中 //使得单词分开,不好看 import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.layout.HBox; import javafx.scene.layout.VBox; import javafx.scene.control.Label; import javafx.scene.text.Font; import javafx.geometry.Insets; import javafx.scene.paint.Color; import javafx.scene.text.FontWeight; import javafx.scene.text.FontPosture; public class ShowHVBox_Java extends Application { @Override public void start(Stage primaryStage) { HBox pane = new HBox(15); pane.setPadding(new Insets(15, 15, 15, 15)); for (int i = 0; i < 5; i++) { pane.getChildren().add(getVBox()); } Scene scene = new Scene(pane); primaryStage.setTitle("ShowHVBox_Java"); primaryStage.setScene(scene); primaryStage.show(); } public VBox getVBox() { VBox pane = new VBox(); String str = "JAVA"; int length = str.length(); Color color = new Color(Math.random(), Math.random(), Math.random(), Math.random()); for (int i = 0; i < length; i++) { Label lb = new Label(str.charAt(i) + ""); lb.setFont(Font.font("TimesRomes", FontWeight.BOLD, FontPosture.ITALIC, 22)); lb.setTextFill(color); lb.setRotate(90); pane.setMargin(lb, new Insets(0, 15, 0, 15)); pane.getChildren().add(lb); } return pane; } }
14.4_3
//多次显示一旋转后的字符串 //每个字符串现在VBox中设置好,然后将面板加到HBox面板中 //中心不好找,要将边距设置好 import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.layout.HBox; import javafx.scene.layout.VBox; import javafx.scene.control.Label; import javafx.scene.text.Font; import javafx.geometry.Insets; import javafx.scene.text.Text; import javafx.scene.paint.Color; import javafx.scene.text.FontWeight; import javafx.scene.text.FontPosture; public class ShowHVBoxText_Java extends Application { @Override public void start(Stage primaryStage) { HBox pane = new HBox(15); pane.setPadding(new Insets(15, 15, 15, 15)); for (int i = 0; i < 5; i++) { pane.getChildren().add(getVBox()); } Scene scene = new Scene(pane); primaryStage.setTitle("ShowHVBoxText_Java"); primaryStage.setScene(scene); primaryStage.show(); } public VBox getVBox() { VBox pane = new VBox(); Color color = new Color(Math.random(), Math.random(), Math.random(), Math.random()); Text lb = new Text("Java"); lb.setFont(Font.font("TimesRomes", FontWeight.BOLD, FontPosture.ITALIC, 22)); lb.setFill(color); lb.setRotate(90); pane.getChildren().add(lb); return pane; } }
14.5
//圆形显示一个字符串,并且每个单词也要指向圆心 //初中数学,找坐标和角度关系 import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.text.Text; import javafx.scene.text.Font; import javafx.scene.paint.Color; import javafx.scene.text.FontWeight; import javafx.scene.text.FontPosture; import javafx.scene.layout.Pane; public class ShowString extends Application { @Override public void start(Stage primaryStage) { Pane pane = new Pane(); String str = "WELCOM TO JAVA"; int length = str.length(); final double centerX = 200, centerY = 200, radius = 100; double divide = 2 * Math.PI / length; for (int i = 0; i < length; i++) { double newX = centerX + radius * Math.cos(i * divide); double newY = centerY + radius * Math.sin(i * divide); Text t = new Text(newX, newY, str.charAt(i) + ""); t.setFill(Color.RED); //Text中的setFont(Font) t.setFont(Font.font("MyFont", FontWeight.BOLD, FontPosture.ITALIC, 15)); //旋转字体 int r = (int)((Math.PI / 2 + i * divide) / (2 * Math.PI) * 360); t.setRotate(r); pane.getChildren().add(t); } Scene scene = new Scene(pane, 400, 400); primaryStage.setTitle("ShowString"); primaryStage.setScene(scene); primaryStage.show(); } }
15.6
//写着写着就写出了兴趣,把方方正正的棋盘改成了 //将面板划分为64份,颜色按照棋盘分布,大小随着窗体大小改变 import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.layout.GridPane; import javafx.scene.paint.Color; import javafx.geometry.Insets; import javafx.scene.shape.Rectangle; import javafx.beans.property.DoubleProperty; import javafx.beans.property.SimpleDoubleProperty; public class Checkerboard extends Application { @Override public void start(Stage primaryStage) { GridPane pane = new GridPane(); //按照黑白存在的规律,先奇数行的白,然后奇数行的黑 //接着偶数行的黑,最后偶数行的白 for (int column = 0; column < 8; column = column + 2) { for (int row = 0; row < 8; row = row + 2) { pane.add(getSquare(column, row, pane, 0), column, row); } } for (int column = 1; column < 8; column = column + 2) { for (int row = 0; row < 8; row = row + 2) { pane.add(getSquare(column, row, pane, 1), column, row); } } for (int column = 0; column < 8; column = column + 2) { for (int row = 1; row < 8; row = row + 2) { pane.add(getSquare(column, row, pane, 1), column, row); } } for (int column = 1; column < 8; column = column + 2) { for (int row = 1; row < 8; row = row + 2) { pane.add(getSquare(column, row, pane, 0), column, row); } } Scene scene = new Scene(pane); primaryStage.setTitle("Checkerbord"); primaryStage.setScene(scene); primaryStage.show(); } //按照给定行和列返回设定确定位置,并返回(使用绑定bind) public Rectangle getSquare(int column, int row, GridPane pane, int judge) { Rectangle re = new Rectangle(); //找到每个格子的起始坐标,面板的宽和高:pane.width,pane.height re.xProperty().bind(pane.widthProperty().divide(8).multiply(row)); re.yProperty().bind(pane.heightProperty().divide(8).multiply(column)); //确定每个格子的高和宽 re.widthProperty().bind(pane.widthProperty().divide(8)); re.heightProperty().bind(pane.heightProperty().divide(8)); if (judge == 0) { re.setFill(Color.WHITE); re.setStroke(Color.WHITE); } else if (judge == 1) { re.setFill(Color.BLACK); re.setStroke(Color.BLACK); } return re; } }
相关文章推荐
- java 语言程序设计 第十二章(12.1、12.2、12.3、12.4、12.5、12.6)
- Java语言程序设计 第十四章 (14.26、14.27、14.28、14.29)
- Java语言程序设计 第十四章 (14.16、14.17、14.18、14.19、14.21、14.22、14.23、14.24、14.25)
- java语言程序设计 第十四章(14.7、14.8、14.9、14.10、14.11、14.12、14.13、14.14、14.15)
- java语言程序设计 第十四章 (样例代码)
- java语言程序设计 14.2 comparable
- Java语言的基本程序设计结构
- 信科及应用技术学院Java语言程序设计、XML语言选用教材
- Java语言程序设计-Eclipse入门之Applet程序
- Java语言程序设计 第十五章 (15.15、15.16、15.17、15.18、15.19、15.20)
- java 语言程序设计-李尊朝 第6章 类和对象 练习答案
- Java语言程序设计-进阶篇(七)多线程与并行程序设计【上】
- java语言程序设计基础篇--第十九章--练习题19.1(修改版)
- 【JAVA语言程序设计第十版 11.2】+ 多态 + 继承
- Java 语言程序设计基础篇原书第八版_第十二章_第八题_程序分享
- java语言程序设计第十版(Introduce to java 10th) 课后习题 chapter7-33
- Java语言程序设计-基础篇-第八版-第二章
- 【JAVA语言程序设计基础篇】--事件驱动程序设计--匿名类监听器
- Java语言程序设计基础(5)【字符串】
- java语言程序设计 第十三章 (13.9、13.10、13.11、13.12)