Android搜索建议(搜索联想)
2018-01-04 00:00
162 查看
Android的搜索建议,简言之,就是说当用户输入某一个关键词后,系统自动给出几个含有相近关键词的搜索建议。
效果图如下:
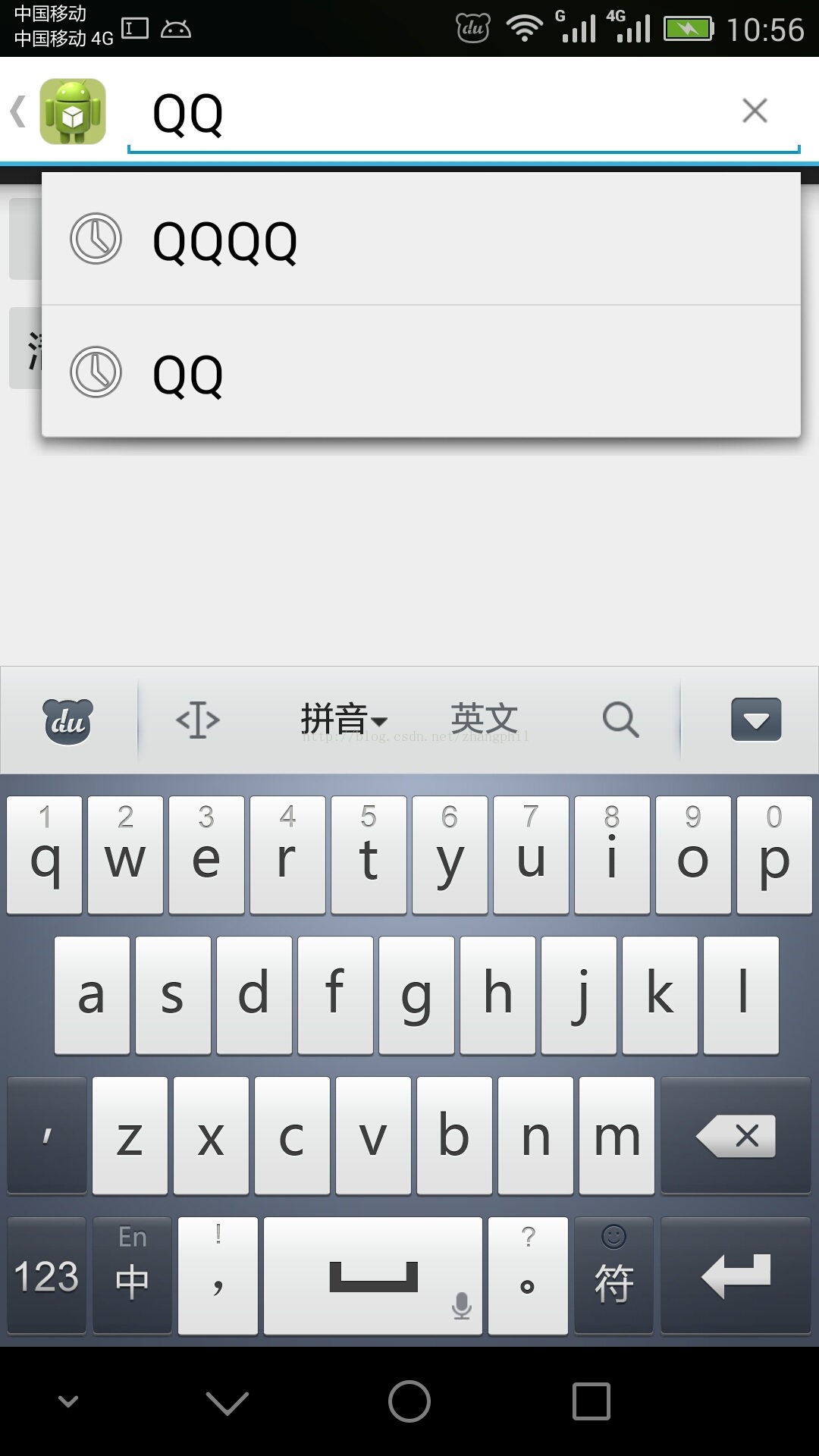
MainActivity.java
onSearchRequested将触发Android系统自动弹出搜索框。MainActivity需要的布局文件activity_main.xml很简单,两个按钮,一个触发搜索事件,一个触发清除搜索历史记录动作:
AndroidManifest.xml的内容:
SearchActivity.java文件:
ZhangPhilSuggestionProvider.java
searchable.xml
完整的项目全部代码文件我已经上传到CSDN,可以点击下载链接下载到本地,解压后导入到Eclipse运行演示。CSDN下载链接:
http://download.csdn.net/detail/zhangphil/8603509
效果图如下:
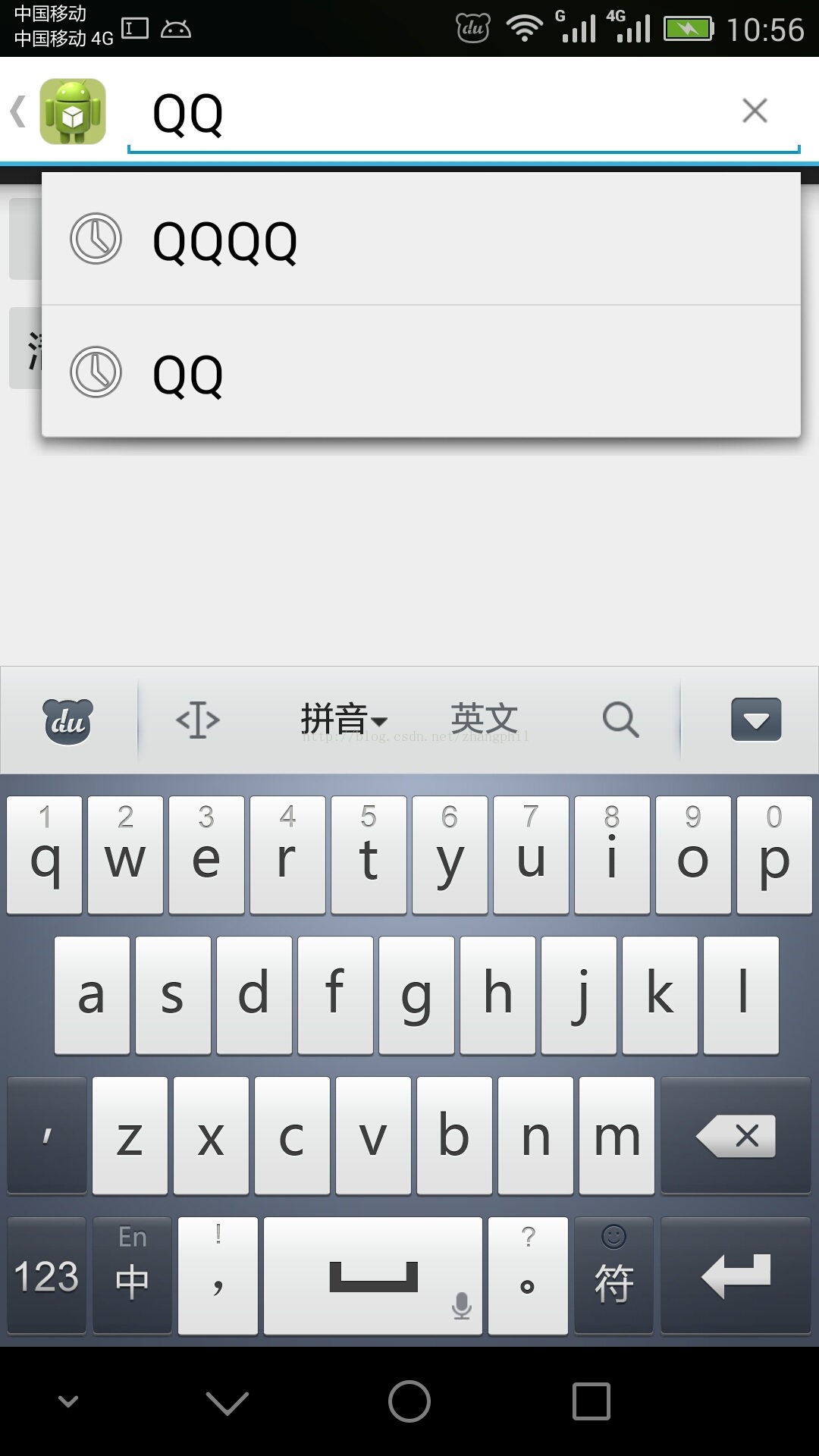
MainActivity.java
package zhangphil.search; import android.support.v7.app.ActionBarActivity; import android.os.Bundle; import android.provider.SearchRecentSuggestions; import android.view.View; import android.widget.Button; public class MainActivity extends ActionBarActivity { @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Button search = (Button) findViewById(R.id.search); search.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // 发起搜索 search(); } }); Button clear = (Button) findViewById(R.id.clear); clear.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { clear(); } }); } private void search() { // 向Android系统发起搜索请求。 this.onSearchRequested(); } // onSearchRequested()方法可以重载 ,也可以不用重载。取决于是否有更多参数或数据传递给下一步操作。 // 通常代码运行逻辑需要传递更多数据给下一个动作时候,则可以重载此方法以完成更多数据的传递。 // 如果不重载,也可以。Android系统会启动默认的搜索框。(在UI的上方) @Override public boolean onSearchRequested() { Bundle bundle = new Bundle(); bundle.putString("some key", "some value"); this.startSearch("输入搜索的关键词"/** 初始化搜索框中的提示词 */ , true, bundle, false /** 此处若为true,则将启动手机上的全局设置的那个搜索,比如Google搜索 */ ); return true; } // 清除全部历史搜索记录 private void clear() { SearchRecentSuggestions suggestions = new SearchRecentSuggestions(this, ZhangPhilSuggestionProvider.AUTHORITY, ZhangPhilSuggestionProvider.MODE); // 出于用户隐私的考虑,给APP提供清除所有用户搜索历史记录的选项。 suggestions.clearHistory(); } }
onSearchRequested将触发Android系统自动弹出搜索框。MainActivity需要的布局文件activity_main.xml很简单,两个按钮,一个触发搜索事件,一个触发清除搜索历史记录动作:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent"> <Button android:id="@+id/search" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/search" /> <Button android:id="@+id/clear" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/clear" /> </LinearLayout>
AndroidManifest.xml的内容:
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="zhangphil.search" android:versionCode="1" android:versionName="1.0" > <uses-sdk android:minSdkVersion="8" android:targetSdkVersion="22" /> <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name=".MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> <meta-data android:name="android.app.default_searchable" android:value=".SearchActivity" /> </activity> <activity android:name=".SearchActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.SEARCH" /> </intent-filter> <meta-data android:name="android.app.searchable" android:resource="@xml/searchable" /> </activity> <provider android:name=".ZhangPhilSuggestionProvider" android:authorities="ZhangPhil_AUTHORITY" /> </application> </manifest>
SearchActivity.java文件:
package zhangphil.search; import android.app.Activity; import android.app.SearchManager; import android.content.Intent; import android.os.Bundle; import android.provider.SearchRecentSuggestions; import android.widget.Toast; public class SearchActivity extends Activity { @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); Intent intent = getIntent(); if (Intent.ACTION_SEARCH.equals(intent.getAction())) { // 存关键词 saveMyRecentQuery(intent); } } private void saveMyRecentQuery(Intent intent) { // 把搜索的关键词保存到Android系统的自带的数据库中。 // 如无必要定制,则此过程可以使用标准、通用的方法。 String query = intent.getStringExtra(SearchManager.QUERY); SearchRecentSuggestions suggestions = new SearchRecentSuggestions(this, ZhangPhilSuggestionProvider.AUTHORITY, ZhangPhilSuggestionProvider.MODE); suggestions.saveRecentQuery(query, null); Toast.makeText(this, "搜索关键词:" + query ,Toast.LENGTH_LONG).show(); } }
ZhangPhilSuggestionProvider.java
package zhangphil.search; import android.content.SearchRecentSuggestionsProvider; public class ZhangPhilSuggestionProvider extends SearchRecentSuggestionsProvider { // 可随意定义一个可区别的字符串,但注意全局的唯一相同引用。 public final static String AUTHORITY = "ZhangPhil_AUTHORITY"; public final static int MODE = DATABASE_MODE_QUERIES; public ZhangPhilSuggestionProvider() { setupSuggestions(AUTHORITY, MODE); } }
searchable.xml
<?xml version="1.0" encoding="utf-8"?> <searchable xmlns:android="http://schemas.android.com/apk/res/android" android:label="@string/app_name" android:hint="@string/search_hint" android:searchSuggestAuthority="ZhangPhil_AUTHORITY" android:searchSuggestSelection=" ?"> </searchable>
完整的项目全部代码文件我已经上传到CSDN,可以点击下载链接下载到本地,解压后导入到Eclipse运行演示。CSDN下载链接:
http://download.csdn.net/detail/zhangphil/8603509
相关文章推荐
- Android搜索建议(搜索联想)
- Android高仿百度地图公交与位置建议搜索(四)
- 为Android应用增加搜索功能:增加搜索建议
- 嵌入AppBar并且带搜索建议的搜索框(Android)
- 嵌入AppBar并且带搜索建议的搜索框(Android)
- 嵌入AppBar并且带搜索建议的搜索框(Android)
- Android 百度地图 SDK v3_3_0 (五) ---POI搜索和在线建议查询功能
- 嵌入AppBar并且带搜索建议的搜索框(Android)
- 嵌入AppBar并且带搜索建议的搜索框(Android)
- 对 Android 开发者有益的 40 条优化建议
- Android百度地图 - 搜索服务之周边检索
- android应用编译失败 ResXMLTree_node size 类错误,以及 android studio 项目内搜索
- 谷歌中国恢复搜索建议功能
- Android超高仿QQ附近的人搜索展示
- Android中全局搜索(QuickSearchBox)(三)
- Android EditText 软键盘搜索事件
- Android中通过ActionBar为标题栏添加搜索及分享视窗
- Android BLE学习(一): Android搜索BLE设备
- 高效开发Android App的10个建议
- android -- 蓝牙 bluetooth (三)搜索蓝牙