C# 生成多张excel 并打包
2018-01-02 14:48
411 查看
自述:在工作中遇到很多有趣的需求,在实施过程中看到了自己的很多不足。
仅在这里记录收获,积累成就感,愉悦自己,感谢他人!
{
HSSFWorkbook workbook = new HSSFWorkbook();
ISheet sheet = workbook.CreateSheet(“Sheet1”);
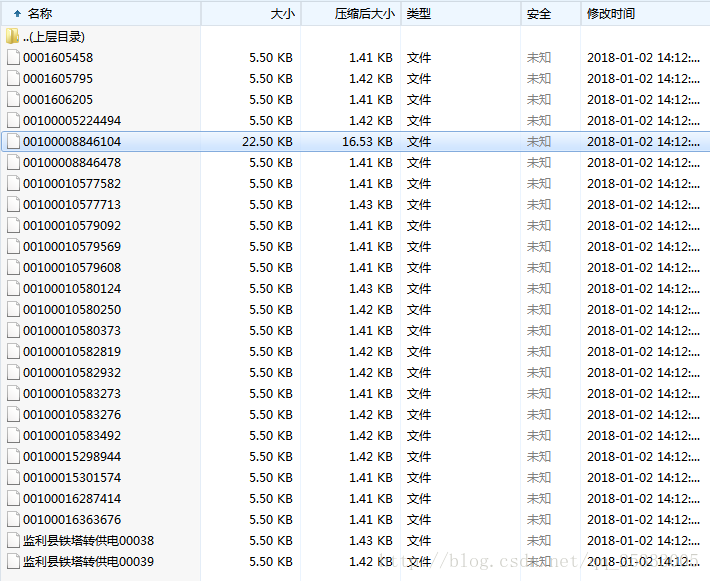
注:excel 和压缩的处理稍显粗糙。期待改进!一路积累,一路成长!欢迎见证!
仅在这里记录收获,积累成就感,愉悦自己,感谢他人!
// <summary> /// 将数据部分处理生成excel 并打包成zip /// </summary> /// <param name="datas">数据列表</param> public static void CreatZip(List<AmmeterProportionLogBll.AmmeterProportionLog_Aduit_Export> datas) { HttpContext curContext = HttpContext.Current; curContext.Response.ContentType = "application/zip"; curContext.Response.ContentEncoding = Encoding.UTF8; curContext.Response.Charset = ""; curContext.Response.AppendHeader("Content-Disposition", "attachment;filename=" + HttpUtility.UrlEncode(DateTime.Now.ToString("yyyyMMddhhmmss") + ".zip", Encoding.UTF8)); //处理数据生成excel表 以K_V形式保存 Workbooks Dictionary<string, HSSFWorkbook> Workbooks = new Dictionary<string, HSSFWorkbook>(); datas.ForEach(p => Workbooks.Add(p.txtAmmeterNum, Export0(p))); //遍历Workbooks 生成zip文件 curContext.Response.BinaryWrite(PackageManyZip(Workbooks).GetBuffer()); curContext.Response.End(); curContext.Response.Close(); }
//生成excel表 截取表的部分显示public static HSSFWorkbook Export2(AmmeterProportionLogBll.AmmeterProportionLog_Aduit_Export m)
{
HSSFWorkbook workbook = new HSSFWorkbook();
ISheet sheet = workbook.CreateSheet(“Sheet1”);
//设置6列 表中涉及多张图片处理 sheet.SetColumnWidth(0, 20 * 256 + 200); sheet.SetColumnWidth(1, 20 * 256 + 200); sheet.SetColumnWidth(2, 20 * 256 + 200); sheet.SetColumnWidth(3, 20 * 256 + 200); sheet.SetColumnWidth(4, 20 * 256 + 200); sheet.SetColumnWidth(5, 20 * 256 + 200); ICellStyle dateStyle = workbook.CreateCellStyle(); IDataFormat format = workbook.CreateDataFormat(); dateStyle.DataFormat = format.GetFormat("yyyy-mm-dd"); int rowIndex = 0;//行标记 #region 表头及样式 { IRow headerRow = sheet.CreateRow(rowIndex); headerRow.HeightInPoints = 25; headerRow.CreateCell(0).SetCellValue("Excel大标题"); ICellStyle headStyle = workbook.CreateCellStyle(); headStyle.Alignment = HorizontalAlignment.Center; headStyle.VerticalAlignment = VerticalAlignment.Center; IFont font = workbook.CreateFont(); font.FontHeightInPoints = 20; font.Boldweight = 700; headStyle.SetFont(font); headerRow.GetCell(0).CellStyle = headStyle; //合并单元格 sheet.AddMergedRegion(new NPOI.SS.Util.CellRangeAddress(rowIndex, rowIndex, 0, 5)); rowIndex++; } #endregion #region 两种样式 ICellStyle CellStyle = workbook.CreateCellStyle(); CellStyle.Alignment = HorizontalAlignment.Left; CellStyle.VerticalAlignment = VerticalAlignment.Center; //字体 IFont CellFont = workbook.CreateFont(); CellFont.FontHeightInPoints = 10; CellFont.Boldweight = 700; CellStyle.SetFont(CellFont); ICellStyle rightheadStyle = workbook.CreateCellStyle(); rightheadStyle.Alignment = HorizontalAlignment.Right; rightheadStyle.VerticalAlignment = VerticalAlignment.Center; //文本样式 2 IFont rightfont = workbook.CreateFont(); rightfont.FontHeightInPoints = 10; rightfont.Boldweight = 700; rightheadStyle.SetFont(rightfont); #endregion #region 区域、核查日期 { rowIndex++; IRow headerRow = sheet.CreateRow(rowIndex); headerRow.HeightInPoints = 20; headerRow.CreateCell(0).SetCellValue("区域:" + m.hfAreaName); headerRow.GetCell(0).CellStyle = CellStyle; sheet.AddMergedRegion(new NPOI.SS.Util.CellRangeAddress(rowIndex, rowIndex, 0, 1)); headerRow.CreateCell(2).SetCellValue("核查日期:" + m.txtCurrentDateInfo.ToString()); headerRow.GetCell(2).CellStyle = CellStyle; sheet.AddMergedRegion(new NPOI.SS.Util.CellRangeAddress(rowIndex, rowIndex, 2, 5)); rowIndex++; } #endregion #region 分别呈现A单位 B单位 C单位的图片X张(0<=X<=12) 每个单位占2列 { int R_CMCCP = DealPhoto(sheet, workbook, m.tdPhoto_CMCCP, rowIndex, 0, 12); int R_CTCCP = DealPhoto(sheet, workbook, m.tdPhoto_CTCCP, rowIndex, 2, 12); int R_CUCCP = DealPhoto(sheet, workbook, m.tdPhoto_CUCCP, rowIndex, 4, 12); rowIndex = Math.Max(Math.Max(R_CMCCP, R_CTCCP), R_CUCCP); } #endregion return d24f workbook; }
/// <summary> /// 分别对多张图片进行列表呈现 /// </summary> /// <param name="Path">图片路径</param> /// <param name="StartCol">起始列</param> /// <param name="MaxNum">限制显示图片张数/param> private static int DealPhoto(ISheet sheet, HSSFWorkbook workbook, string Path, int rowIndex, int StartCol, int MaxNum) { int RowNum = rowIndex - 1; if (Path != "") { string[] paths = Path.Split(';'); int i = 0; for (i = 0; i < paths.Length && i < MaxNum; i++) { if (i % 2 == 0) { IRow headerRow = sheet.CreateRow(rowIndex + i / 2); headerRow.HeightInPoints = 80; RowNum++; } AddPieChartMerage(sheet, workbook, paths[i], RowNum, StartCol + i % 2, 0); } } else { IRow headerRow = sheet.CreateRow(rowIndex); headerRow.HeightInPoints = 80; sheet.AddMergedRegion(new NPOI.SS.Util.CellRangeAddress(rowIndex, rowIndex, StartCol, StartCol + 1)); RowNum++; } return ++RowNum; } //图片处理 private static void AddPieChartMerage(ISheet sheet, HSSFWorkbook workbook, string totalFileURL, int row, int col, int MerageColCount) { try { HSSFPatriarch patriarch = (HSSFPatriarch)sheet.CreateDrawingPatriarch(); //照片位置,(col, row)第row+1行col+1列,右下角为( col +1, row +1)第 col +1+1行row +1+1列,宽为100,高为50 HSSFClientAnchor anchor = new HSSFClientAnchor(0, 0, 100, 50, col, row, col + 1, row + 1); int i = 0; foreach (var fileurl in totalFileURL.Split(';')) { string path = fileurl;//图片路径 byte[] bytes = System.IO.File.ReadAllBytes(path); if (!string.IsNullOrEmpty(path)) { int pictureIdx = workbook.AddPicture(bytes, NPOI.SS.UserModel.PictureType.JPEG); anchor = new HSSFClientAnchor(i * 100, 0, i * 100 + 100, 0, col, row, col + 1 + MerageColCount, row + 1); HSSFPicture pict = (HSSFPicture)patriarch.CreatePicture(anchor, pictureIdx); //pict.Resize();用图片原始大小来显示 } i++; } } catch (Exception) { //throw ex; } }//生成zip文件
public static MemoryStream PackageManyZip(Dictionary<string, HSSFWorkbook> Workbooks) { byte[] buffer; MemoryStream returnStream = new MemoryStream(); var zipMs = new MemoryStream(); using (ZipOutputStream zipStream = new ZipOutputStream(zipMs)) { zipStream.SetLevel(7);//压缩程度 foreach (var kv in Workbooks) { string fileName = kv.Key;//文件名为AmmeterNum using (MemoryStream ms = new MemoryStream()) { kv.Value.Write(ms); ms.Position = 0; var streamInput = ms; buffer = new byte[streamInput.Length]; zipStream.PutNextEntry(new ZipEntry(fileName)); while (true) { var readCount = streamInput.Read(buffer, 0, buffer.Length); if (readCount > 0) { zipStream.Write(buffer, 0, readCount); } else { break; } } ms.Flush(); } zipStream.Flush(); } zipStream.Finish(); zipMs.Position = 0; zipMs.CopyTo(returnStream, 5600); } returnStream.Position = 0; return returnStream; }效果如下:
注:excel 和压缩的处理稍显粗糙。期待改进!一路积累,一路成长!欢迎见证!
相关文章推荐
- 使用C#和Excel进行报表开发(三)-生成统计图(Chart)
- [转] C#操作EXCEL,生成图表的全面应用
- CLR via C# 3 读书笔记(10):第2章 生成、打包、部署和管理应用程序与类型 — 2.2 将类型生成为模块
- C# 生成excel时报错,异常来自:HRESULT:0x80028018(TYPE_E_INVDAIAREAD)
- 用C#生成Excel文件的方法和Excel.dll组件生成的方法 选择自 wang8712 的 Blog
- 使用C#和Excel进行报表开发(三)-生成统计图(Chart)1
- c#生成、打包、部署和管理应用程序类型
- C# 如何在Excel 动态生成PivotTable
- C# 多个DataTable导入到Excel多张Sheet表
- (转)C#生成Excel的方法
- C#生成Excel文件后彻底解除占用代码(来着CSDN)
- c# 从sql 数据库生成 excel (转)
- C#/.NET 4.0新特性生成Excel文档
- C# 将数据生成excel并储存
- 用C#生成Excel文件的方法,Excel.dll组件生成的方法和设置用ASP.NET操作访问权限
- 使用C#和Excel进行报表开发(三)-生成统计图(Chart)2
- CLR via C# 3 读书笔记(13):第2章 生成、打包、部署和管理应用程序与类型 — 2.4 将模块组合为程序集(下)
- 简单实现C#生成Excel 2007文件并下载
- C#生成Excel
- 关于C#操作EXCEL,生成图表的全面应用之二(利用Microsoft.Office.Interop.OWC11)