Java设计模式之装饰者模式
2017-12-16 21:30
309 查看
装饰模式(Decorator):动态地给一个对象添加一些额外的职责,就增加功能来说,装饰模式比生成子类更为灵活。
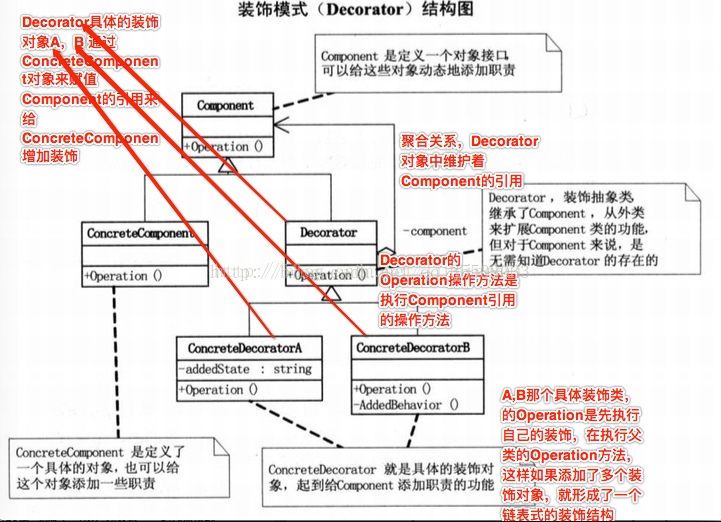
Component是定义一个对象接口,可以给这些对象动态地添加职责:
装饰模式客户端调用代码:
运行结果:
具体对象的操作
A中的new state 具体装饰对象A的操作
B中的新增行为 具体装饰对象B的操作
C没有特殊行为 具体装饰对象C的操作Component 是定义一个对象接口,可以给这些对象动态的添加职责,ConcreteComponent是定义了一个具体的对象,也可以给这个对象添加一些职责。Decorator,装饰抽象类,继承了Component,从外类来扩展Component类的功能,单对于Component来说,是无需知道Decorator的存在的,至于ConcreteDecorator就是具体的装饰对象,起到给Component添加职责的功能。装饰模式是利用SetComponent来对对象进行包装,这样每个装饰对象的实现就和如何使用这个对象分离开了,每个装饰对象只关心自己的功能,不需要关心如何被添加到对象链当中。
Component是定义一个对象接口,可以给这些对象动态地添加职责:
public abstract class Component {//Component是定义一个对象接口,可以给这些对象动态地添加职责 public abstract void operation(); }
public class ConcreteComponent extends Component {//ConcreteComponent是定义一个具体的对象,也可以给这个对象添加一些职责 @Override public void operation() { System.out.println("具体对象的操作"); } }Decorator,装饰抽象类:
/** * Decorator,装饰抽象类,继承了Component,从外类来扩展Component类的功能,但对于Component来说, * 是无需知道Decorator的存在的 */ public abstract class Decorator extends Component { protected Component component; public Component getComponent() { return component; } public void setComponent(Component component) { this.component = component; } @Override public void operation() { if (component != null) { component.operation(); } } } class ConcreteDecoratorA extends Decorator { private String addedState; @Override public void operation() { // 首先运行原Component的operation(),再执行本类的功能,如addedState,相当于对原Component进行了装饰 super.operation(); addedState = "A中的new state "; System.out.println(addedState + "具体装饰对象A的操作"); } } class ConcreteDecoratorB extends Decorator { @Override public void operation() { super.operation(); addedBehavior(); System.out.println("具体装饰对象B的操作"); } public void addedBehavior() { System.out.print("B中的新增行为 "); } } class ConcreteDecoratorC extends Decorator { @Override public void operation() { super.operation(); System.out.println("C没有特殊行为 " + "具体装饰对象C的操作"); } }
装饰模式客户端调用代码:
/** * 装饰模式客户端调用代码,装饰的过程更像是层层包装,用前面的对象装饰后面的对象 */ public class DecoratorClient { public static void main(String[] args) { ConcreteComponent concreteComponent = new ConcreteComponent(); ConcreteDecoratorA concreteDecoratorA = new ConcreteDecoratorA(); ConcreteDecoratorB concreteDecoratorB = new ConcreteDecoratorB(); ConcreteDecoratorC concreteDecoratorC = new ConcreteDecoratorC(); concreteDecoratorA.setComponent(concreteComponent); concreteDecoratorB.setComponent(concreteDecoratorA); concreteDecoratorC.setComponent(concreteDecoratorB); concreteDecoratorC.operation(); } }
运行结果:
具体对象的操作
A中的new state 具体装饰对象A的操作
B中的新增行为 具体装饰对象B的操作
C没有特殊行为 具体装饰对象C的操作Component 是定义一个对象接口,可以给这些对象动态的添加职责,ConcreteComponent是定义了一个具体的对象,也可以给这个对象添加一些职责。Decorator,装饰抽象类,继承了Component,从外类来扩展Component类的功能,单对于Component来说,是无需知道Decorator的存在的,至于ConcreteDecorator就是具体的装饰对象,起到给Component添加职责的功能。装饰模式是利用SetComponent来对对象进行包装,这样每个装饰对象的实现就和如何使用这个对象分离开了,每个装饰对象只关心自己的功能,不需要关心如何被添加到对象链当中。
相关文章推荐
- Java设计模式之---装饰者模式
- JAVA设计模式初探之装饰者模式
- java基础_设计模式_装饰者模式
- Java——设计模式——装饰者模式
- Java设计模式之装饰者模式
- 设计模式(Java)-装饰者模式
- java设计模式之装饰者模式Decorator
- JAVA设计模式初探之装饰者模式
- JAVA设计模式之装饰者模式
- JavaWeb中使用Filter以及装饰者设计模式解决全局编码格式问题
- Java设计模式透析--装饰者模式(二)
- java设计模式-装饰者模式
- java常用设计模式(装饰者模式)
- 设计模式之装饰者(java)
- JAVA设计模式初探之装饰者模式
- 设计模式-Python Java装饰者模式
- JAVA设计模式之装饰者模式
- java设计模式-装饰者模式
- Java设计模式之装饰者模式
- Java设计模式透析--装饰者模式(二)