(转)Android中动态添加view,删除view,获取view的内容
2017-11-24 11:13
525 查看
原地址:http://blog.csdn.net/hustlwz/article/details/52693768
最近在项目中碰到一个需求,在编辑一个红包详情页面的时候,需要动态的添加使用条件,并且可以删除,最后提交数据的时候需要获取到条件里面的数据。网上搜了一些答案没有比较满意的解决方法,所以写下此文。
效果图:
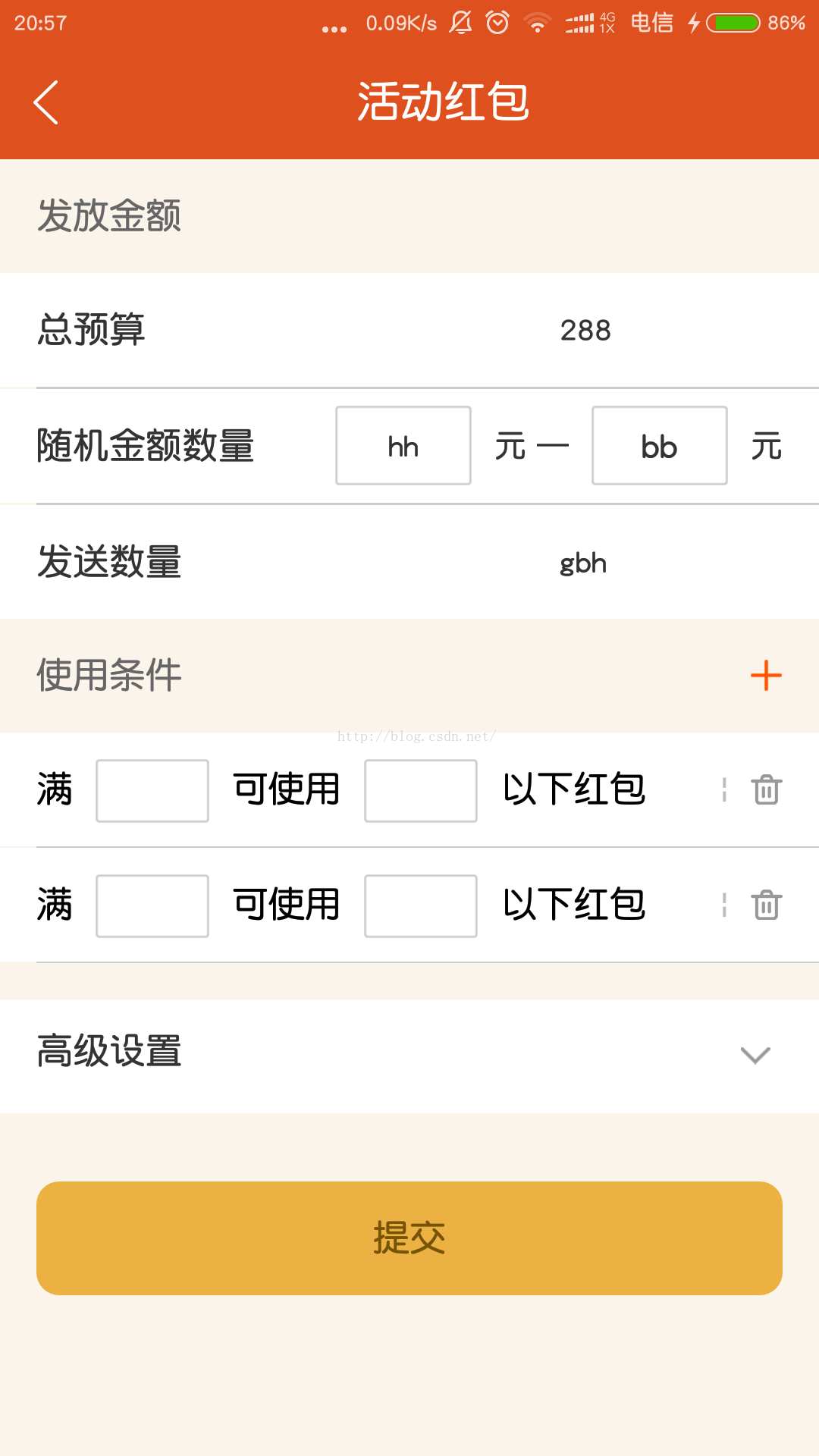
解决思路:
1.使用布局的addview方法,即可动态添加。
2.动态删除需要获取到当前条目在布局中的位置或者对象,然后用removeview方法删除。
3.提交服务器需要获取条目中的数据,可以通过维护一个hashmap集合来遍历获取其中的内容。
废话不多说了,上代码!
1.先上item的布局
[html] view
plain copy
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="51dp"
android:id="@+id/ll_condition_edit_redbag2"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="50dp"
android:background="@color/bg_white_color"
android:layout_gravity="center_vertical"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_marginLeft="16dp"
android:layout_marginRight="10dp"
android:gravity="center_vertical"
android:text="满"
android:textColor="@color/text_black_color"
android:textSize="16sp" />
<EditText
android:id="@+id/ed_high"
android:layout_width="50dp"
android:layout_height="28dp"
android:layout_centerVertical="true"
android:background="@drawable/edit_bg_white"
android:gravity="center"
android:hint=""
android:textColor="@color/text_dark_color"
android:textColorHint="@color/text_hint_color"
android:textSize="14sp" />
<TextView
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
android:gravity="center_vertical"
android:text="可使用"
android:textColor="@color/text_black_color"
android:textSize="16sp" />
<EditText
android:id="@+id/ed_low"
android:layout_width="50dp"
android:layout_height="28dp"
android:layout_centerVertical="true"
android:background="@drawable/edit_bg_white"
android:gravity="center"
android:hint=""
android:textColor="@color/text_dark_color"
android:textColorHint="@color/text_hint_color"
android:textSize="14sp" />
<TextView
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
android:gravity="center_vertical"
android:text="以下红包"
android:textColor="@color/text_black_color"
android:textSize="16sp" />
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="right|center_vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_marginRight="10dp"
android:gravity="center_vertical"
android:text="|"
android:textSize="16sp" />
<ImageView
android:id="@+id/iv_del_item"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_gravity="right"
android:paddingRight="16dp"
android:src="@drawable/icon_del"
/>
</LinearLayout>
</LinearLayout>
<ImageView
android:layout_width="match_parent"
android:layout_height="0.5dp"
android:layout_alignParentEnd="true"
android:layout_alignParentRight="true"
android:layout_marginLeft="16dp"
android:background="@color/text_hint_color" />
</LinearLayout>
2.把这个view插入到布局中,并放入到一个集合中,方便最后上传时使用
[java] view
plain copy
//1创建集合管理红包条件
HashMap<Integer,LinearLayout> conditions = new HashMap<>();
int itemId =0;
//红包使用条件的默认加入位置
private int position = 7;
private void createConditon() {
//每次创建一个view
LinearLayout ll_limit = (LinearLayout) View.inflate(this, R.layout.item_condition_edit_redbag, null);
//删除按钮
ImageView iv_del_item = (ImageView) ll_limit.findViewById(R.id.iv_del_item);
//给每个删除条目绑定一个id
iv_del_item.setTag(itemId);
iv_del_item.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//获取绑定的id
int itemId = (int) v.getTag();
//布局中删除指定位置的view
ll_content_edit_redab2.removeView(conditions.get(itemId));
//集合中删除指定的item对象
conditions.remove(itemId);
//记录position最新位置
position--;
}
});
//把当前对象保存到集合中
conditions.put(itemId,ll_limit);
ll_content_edit_redab2.addView(ll_limit, position);
//每次添加以后,位置会变动
position++;
//每调用一次id++,防止重复
itemId++;
}
代码注释已经很详细了,简单提下:我用position记录view在布局中的位置,用itemid记录绑定的view
3.上传的代码:
[java] view
plain copy
Set<Map.Entry<Integer, LinearLayout>> items = conditions.entrySet();
//转换为迭代器
Iterator iter = items.iterator();
while (iter.hasNext()) {
Map.Entry entry = (Map.Entry) iter.next();
LinearLayout val = (LinearLayout) entry.getValue();
EditText ed_high = (EditText) val.findViewById(R.id.ed_high);
EditText ed_low = (EditText) val.findViewById(R.id.ed_low);
String s1 = ed_high.getText().toString().trim();
String s2 = ed_low.getText().toString().trim();
if (TextUtils.isEmpty(s1) &&TextUtils.isEmpty(s2)){
Toast.makeText(this,"限制条件填写不完整",Toast.LENGTH_SHORT).show();
return;
}
limits.add(new RedbagLimit(s1,s2));
}
final String s = new Gson().toJson(limits);
LogUtil.d("limit",s);
[java] view
plain copy
<pre style="font-family: 宋体; font-size: 10.5pt; background-color: rgb(255, 255, 255);"><pre name="code" class="java">List<RedbagLimit> limits = new ArrayList<>();
class RedbagLimit{
String price ;
String useable;
RedbagLimit(String price ,String useable){
this.price = price;
this.useable = useable;
}
}
因为上传的时候需要封装成一个数组,所以我先遍历获取数据再封装到集合中,通过gson转换成字符串。
第一次发文,有不对的地方请指正!
最近在项目中碰到一个需求,在编辑一个红包详情页面的时候,需要动态的添加使用条件,并且可以删除,最后提交数据的时候需要获取到条件里面的数据。网上搜了一些答案没有比较满意的解决方法,所以写下此文。
效果图:
解决思路:
1.使用布局的addview方法,即可动态添加。
2.动态删除需要获取到当前条目在布局中的位置或者对象,然后用removeview方法删除。
3.提交服务器需要获取条目中的数据,可以通过维护一个hashmap集合来遍历获取其中的内容。
废话不多说了,上代码!
1.先上item的布局
[html] view
plain copy
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="51dp"
android:id="@+id/ll_condition_edit_redbag2"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="50dp"
android:background="@color/bg_white_color"
android:layout_gravity="center_vertical"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_marginLeft="16dp"
android:layout_marginRight="10dp"
android:gravity="center_vertical"
android:text="满"
android:textColor="@color/text_black_color"
android:textSize="16sp" />
<EditText
android:id="@+id/ed_high"
android:layout_width="50dp"
android:layout_height="28dp"
android:layout_centerVertical="true"
android:background="@drawable/edit_bg_white"
android:gravity="center"
android:hint=""
android:textColor="@color/text_dark_color"
android:textColorHint="@color/text_hint_color"
android:textSize="14sp" />
<TextView
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
android:gravity="center_vertical"
android:text="可使用"
android:textColor="@color/text_black_color"
android:textSize="16sp" />
<EditText
android:id="@+id/ed_low"
android:layout_width="50dp"
android:layout_height="28dp"
android:layout_centerVertical="true"
android:background="@drawable/edit_bg_white"
android:gravity="center"
android:hint=""
android:textColor="@color/text_dark_color"
android:textColorHint="@color/text_hint_color"
android:textSize="14sp" />
<TextView
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
android:gravity="center_vertical"
android:text="以下红包"
android:textColor="@color/text_black_color"
android:textSize="16sp" />
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="right|center_vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_marginRight="10dp"
android:gravity="center_vertical"
android:text="|"
android:textSize="16sp" />
<ImageView
android:id="@+id/iv_del_item"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_gravity="right"
android:paddingRight="16dp"
android:src="@drawable/icon_del"
/>
</LinearLayout>
</LinearLayout>
<ImageView
android:layout_width="match_parent"
android:layout_height="0.5dp"
android:layout_alignParentEnd="true"
android:layout_alignParentRight="true"
android:layout_marginLeft="16dp"
android:background="@color/text_hint_color" />
</LinearLayout>
2.把这个view插入到布局中,并放入到一个集合中,方便最后上传时使用
[java] view
plain copy
//1创建集合管理红包条件
HashMap<Integer,LinearLayout> conditions = new HashMap<>();
int itemId =0;
//红包使用条件的默认加入位置
private int position = 7;
private void createConditon() {
//每次创建一个view
LinearLayout ll_limit = (LinearLayout) View.inflate(this, R.layout.item_condition_edit_redbag, null);
//删除按钮
ImageView iv_del_item = (ImageView) ll_limit.findViewById(R.id.iv_del_item);
//给每个删除条目绑定一个id
iv_del_item.setTag(itemId);
iv_del_item.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//获取绑定的id
int itemId = (int) v.getTag();
//布局中删除指定位置的view
ll_content_edit_redab2.removeView(conditions.get(itemId));
//集合中删除指定的item对象
conditions.remove(itemId);
//记录position最新位置
position--;
}
});
//把当前对象保存到集合中
conditions.put(itemId,ll_limit);
ll_content_edit_redab2.addView(ll_limit, position);
//每次添加以后,位置会变动
position++;
//每调用一次id++,防止重复
itemId++;
}
代码注释已经很详细了,简单提下:我用position记录view在布局中的位置,用itemid记录绑定的view
3.上传的代码:
[java] view
plain copy
Set<Map.Entry<Integer, LinearLayout>> items = conditions.entrySet();
//转换为迭代器
Iterator iter = items.iterator();
while (iter.hasNext()) {
Map.Entry entry = (Map.Entry) iter.next();
LinearLayout val = (LinearLayout) entry.getValue();
EditText ed_high = (EditText) val.findViewById(R.id.ed_high);
EditText ed_low = (EditText) val.findViewById(R.id.ed_low);
String s1 = ed_high.getText().toString().trim();
String s2 = ed_low.getText().toString().trim();
if (TextUtils.isEmpty(s1) &&TextUtils.isEmpty(s2)){
Toast.makeText(this,"限制条件填写不完整",Toast.LENGTH_SHORT).show();
return;
}
limits.add(new RedbagLimit(s1,s2));
}
final String s = new Gson().toJson(limits);
LogUtil.d("limit",s);
[java] view
plain copy
<pre style="font-family: 宋体; font-size: 10.5pt; background-color: rgb(255, 255, 255);"><pre name="code" class="java">List<RedbagLimit> limits = new ArrayList<>();
class RedbagLimit{
String price ;
String useable;
RedbagLimit(String price ,String useable){
this.price = price;
this.useable = useable;
}
}
因为上传的时候需要封装成一个数组,所以我先遍历获取数据再封装到集合中,通过gson转换成字符串。
第一次发文,有不对的地方请指正!
相关文章推荐
- Android中动态添加view,删除view,获取view的内容
- Android RecyclerView 获取数据实现添加,删除功能
- Android 动态添加view或item并获取数据的实例
- Android开发中动态获取RecyclerView的Item中EditText的内容
- android 时间轴 (可动态添加时间轴的内部内容,没实现删除功能)
- Android LayoutInflater 动态地添加删除View
- Android 动态添加view或item并获取数据
- Android – ListView 中添加按钮,动态删除添加ItemView的操作
- JS 动态添加删除文本 并获取文本值
- Android中ListView动态添加删除项
- 使用代码动态添加、删除view,通过tag寻找控件
- 使用jQuery动态创建一个表格(根据用户输入的内容添加一行数据,并且能逐行删除)
- android 动态背景的实现以及SurfaceView中添加EditText控件
- android开发--详解ListView,动态添加,删除Adapter中的数据项
- android 动态添加自定义TextView
- tableView中动态添加,删除行
- android之SimpleAdapter创建和动态添加.删除SimpleAdapter选中项
- TableView动态的删除,添加cell
- 动态添加删除 Spinner内容
- android 动态背景的实现以及SurfaceView中添加EditText控件