自定义组合控件实现 购物车加减的简单实现
2017-11-22 20:18
501 查看
简单实现购物车加减
效果图
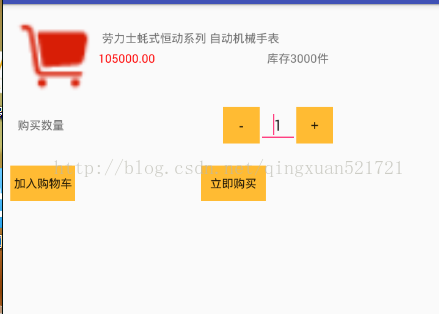
首先在values文件下创建一个attrs样式
attrs样式
[html] view
plain copy
<?xml version="1.0" encoding="utf-8"?>
<resources>
<declare-styleable name="AddDeleteViewStyle">
<attr name="left_text" format="string"/>
<attr name="right_text" format="string"/>
<attr name="middle_text" format="string"/>
</declare-styleable>
</resources>
布局
activity——main
[html] view
plain copy
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="10dp"
>
<LinearLayout
android:id="@+id/ll"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:weightSum="2"
>
<ImageView
android:id="@+id/imageView"
android:layout_width="120dp"
android:layout_height="120dp"
android:src="@drawable/gouwuche2"
/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:weightSum="2"
>
<TextView
android:id="@+id/tv_title"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:src="@mipmap/ic_launcher"
android:padding="5dp"
android:layout_marginTop="20dp"
android:text="劳力士蚝式恒动系列 自动机械手表"
/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:weightSum="2"
>
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:textColor="#f00"
android:text="105000.00"
/>
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="库存3000件"
/>
</LinearLayout>
</LinearLayout>
</LinearLayout>
<LinearLayout
android:id="@+id/lll"
android:layout_below="@+id/ll"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="10dp"
android:weightSum="2"
>
<TextView
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content"
android:text="购买数量"
android:layout_gravity="center"
/>
<!--自定义控件加减器-->
<com. 此处换成自己的包名引入.JiaJianQi
android:id="@+id/adv_main"
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content"
app:left_text="-"
app:middle_text="1"
app:right_text="+">
</com. 此处换成自己的包名引入.JiaJianQi>
</LinearLayout>
<Button
android:layout_below="@id/lll"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="加入购物车"
android:layout_marginTop="20dp"
android:background="@android:color/holo_orange_light"
/>
<Button
android:layout_below="@id/lll"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="立即购买"
android:layout_marginLeft="260dp"
android:layout_marginTop="20dp"
android:background="@android:color/holo_orange_light"
/>
</RelativeLayout>
item_add_delete
[html] view
plain copy
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal"
>
<Button
android:id="@+id/but_delete"
android:layout_width="50dp"
android:layout_height="50dp"
android:text="-"
android:textSize="20dp"
android:background="@android:color/holo_orange_light"
/>
<EditText
android:id="@+id/et_number"
android:layout_width="50dp"
android:inputType="number"
android:layout_height="wrap_content"
android:gravity="center"
/>
<Button
android:id="@+id/but_add"
android:layout_width="50dp"
android:layout_height="50dp"
android:text="+"
android:textSize="20dp"
android:background="@android:color/holo_orange_light"
/>
</LinearLayout>
</LinearLayout>
MainActivity
[html] view
plain copy
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.view.View;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private static final String TAG = "MainActivity";
private JiaJianQi adv;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initView();
}
private void initView() {
adv = (JiaJianQi) findViewById(R.id.adv_main);
adv.OnJiaJianClickListener(new JiaJianQi.OnJiaJianClickListener() {
@Override
public void onAddClick(View v) {
Log.i(TAG, "onAddClick: 执行");
int origin = adv.getNumber();
// if(origin<=4){
origin++;
//Toast.makeText(MainActivity.this, "最多不能多于5个", Toast.LENGTH_SHORT).show();
adv.setNumber(origin);
//}
}
@Override
public void onDelClick(View v) {
int origin = adv.getNumber();
//if(origin==1){
origin--;
Toast.makeText(MainActivity.this, "最少不能小于1个", Toast.LENGTH_SHORT).show();
adv.setNumber(origin);
//}
}
});
}
}
JiaJianQi自定义类
[html] view
plain copy
import android.content.Context;
import android.content.res.TypedArray;
import android.support.annotation.Nullable;
import android.util.AttributeSet;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.LinearLayout;
public class JiaJianQi extends LinearLayout{
private OnJiaJianClickListener listener;
private EditText ed_number;
private String numberStr;
public void OnJiaJianClickListener(OnJiaJianClickListener listener) {
if(listener!=null){
this.listener = listener;
}
}
public interface OnJiaJianClickListener{
void onAddClick(View v);
void onDelClick(View v);
}
public JiaJianQi(Context context) {
this(context,null);
}
public JiaJianQi(Context context, @Nullable AttributeSet attrs) {
this(context, attrs,0);
}
public JiaJianQi(Context context, @Nullable AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
initView(context, attrs, defStyleAttr);
}
private void initView(Context context, AttributeSet attrs, int defStyleAttr) {
View.inflate(context, R.layout.item_add_delete,this);
Button but_add=findViewById(R.id.but_add);
Button but_delete=findViewById(R.id.but_delete);
ed_number=findViewById(R.id.et_number);
//styleable.AddDeleteViewStyle它是attrs里的样式
TypedArray typedArray = context.obtainStyledAttributes(attrs, R.styleable.AddDeleteViewStyle);
String left_text = typedArray.getString(R.styleable.AddDeleteViewStyle_left_text);
String middle_text = typedArray.getString(R.styleable.AddDeleteViewStyle_middle_text);
String right_text = typedArray.getString(R.styleable.AddDeleteViewStyle_right_text);
but_delete.setText(left_text);
but_add.setText(right_text);
ed_number.setText(middle_text);
//释放资源
typedArray.recycle();
but_add.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View view) {
listener.onAddClick(view);
}
});
but_delete.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View view) {
listener.onDelClick(view);
}
});
}
/**
* 对外提供设置EditText值的方法
*/
public void setNumber(int number){
if (number>0){
ed_number.setText(number+"");
}
}
/**
* 得到控件原来的值
*/
public int getNumber(){
int number = 0;
try {
numberStr = ed_number.getText().toString().trim();
number = Integer.valueOf(numberStr);
} catch (Exception e) {
number = 0;
}
return number;
}
}
效果图
首先在values文件下创建一个attrs样式
attrs样式
[html] view
plain copy
<?xml version="1.0" encoding="utf-8"?>
<resources>
<declare-styleable name="AddDeleteViewStyle">
<attr name="left_text" format="string"/>
<attr name="right_text" format="string"/>
<attr name="middle_text" format="string"/>
</declare-styleable>
</resources>
布局
activity——main
[html] view
plain copy
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="10dp"
>
<LinearLayout
android:id="@+id/ll"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:weightSum="2"
>
<ImageView
android:id="@+id/imageView"
android:layout_width="120dp"
android:layout_height="120dp"
android:src="@drawable/gouwuche2"
/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:weightSum="2"
>
<TextView
android:id="@+id/tv_title"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:src="@mipmap/ic_launcher"
android:padding="5dp"
android:layout_marginTop="20dp"
android:text="劳力士蚝式恒动系列 自动机械手表"
/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:weightSum="2"
>
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:textColor="#f00"
android:text="105000.00"
/>
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="库存3000件"
/>
</LinearLayout>
</LinearLayout>
</LinearLayout>
<LinearLayout
android:id="@+id/lll"
android:layout_below="@+id/ll"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="10dp"
android:weightSum="2"
>
<TextView
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content"
android:text="购买数量"
android:layout_gravity="center"
/>
<!--自定义控件加减器-->
<com. 此处换成自己的包名引入.JiaJianQi
android:id="@+id/adv_main"
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content"
app:left_text="-"
app:middle_text="1"
app:right_text="+">
</com. 此处换成自己的包名引入.JiaJianQi>
</LinearLayout>
<Button
android:layout_below="@id/lll"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="加入购物车"
android:layout_marginTop="20dp"
android:background="@android:color/holo_orange_light"
/>
<Button
android:layout_below="@id/lll"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="立即购买"
android:layout_marginLeft="260dp"
android:layout_marginTop="20dp"
android:background="@android:color/holo_orange_light"
/>
</RelativeLayout>
item_add_delete
[html] view
plain copy
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal"
>
<Button
android:id="@+id/but_delete"
android:layout_width="50dp"
android:layout_height="50dp"
android:text="-"
android:textSize="20dp"
android:background="@android:color/holo_orange_light"
/>
<EditText
android:id="@+id/et_number"
android:layout_width="50dp"
android:inputType="number"
android:layout_height="wrap_content"
android:gravity="center"
/>
<Button
android:id="@+id/but_add"
android:layout_width="50dp"
android:layout_height="50dp"
android:text="+"
android:textSize="20dp"
android:background="@android:color/holo_orange_light"
/>
</LinearLayout>
</LinearLayout>
MainActivity
[html] view
plain copy
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.view.View;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private static final String TAG = "MainActivity";
private JiaJianQi adv;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initView();
}
private void initView() {
adv = (JiaJianQi) findViewById(R.id.adv_main);
adv.OnJiaJianClickListener(new JiaJianQi.OnJiaJianClickListener() {
@Override
public void onAddClick(View v) {
Log.i(TAG, "onAddClick: 执行");
int origin = adv.getNumber();
// if(origin<=4){
origin++;
//Toast.makeText(MainActivity.this, "最多不能多于5个", Toast.LENGTH_SHORT).show();
adv.setNumber(origin);
//}
}
@Override
public void onDelClick(View v) {
int origin = adv.getNumber();
//if(origin==1){
origin--;
Toast.makeText(MainActivity.this, "最少不能小于1个", Toast.LENGTH_SHORT).show();
adv.setNumber(origin);
//}
}
});
}
}
JiaJianQi自定义类
[html] view
plain copy
import android.content.Context;
import android.content.res.TypedArray;
import android.support.annotation.Nullable;
import android.util.AttributeSet;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.LinearLayout;
public class JiaJianQi extends LinearLayout{
private OnJiaJianClickListener listener;
private EditText ed_number;
private String numberStr;
public void OnJiaJianClickListener(OnJiaJianClickListener listener) {
if(listener!=null){
this.listener = listener;
}
}
public interface OnJiaJianClickListener{
void onAddClick(View v);
void onDelClick(View v);
}
public JiaJianQi(Context context) {
this(context,null);
}
public JiaJianQi(Context context, @Nullable AttributeSet attrs) {
this(context, attrs,0);
}
public JiaJianQi(Context context, @Nullable AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
initView(context, attrs, defStyleAttr);
}
private void initView(Context context, AttributeSet attrs, int defStyleAttr) {
View.inflate(context, R.layout.item_add_delete,this);
Button but_add=findViewById(R.id.but_add);
Button but_delete=findViewById(R.id.but_delete);
ed_number=findViewById(R.id.et_number);
//styleable.AddDeleteViewStyle它是attrs里的样式
TypedArray typedArray = context.obtainStyledAttributes(attrs, R.styleable.AddDeleteViewStyle);
String left_text = typedArray.getString(R.styleable.AddDeleteViewStyle_left_text);
String middle_text = typedArray.getString(R.styleable.AddDeleteViewStyle_middle_text);
String right_text = typedArray.getString(R.styleable.AddDeleteViewStyle_right_text);
but_delete.setText(left_text);
but_add.setText(right_text);
ed_number.setText(middle_text);
//释放资源
typedArray.recycle();
but_add.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View view) {
listener.onAddClick(view);
}
});
but_delete.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View view) {
listener.onDelClick(view);
}
});
}
/**
* 对外提供设置EditText值的方法
*/
public void setNumber(int number){
if (number>0){
ed_number.setText(number+"");
}
}
/**
* 得到控件原来的值
*/
public int getNumber(){
int number = 0;
try {
numberStr = ed_number.getText().toString().trim();
number = Integer.valueOf(numberStr);
} catch (Exception e) {
number = 0;
}
return number;
}
}
相关文章推荐
- 自定义View实现东购物车加减控件
- 安卓_手机卫士_第二天(GridView,自定义组合控件实现设置页项,自定义对话框)
- Android自定义组合控件---简单导航栏
- 一个简单的自定义EditText控件实现
- 购物车加减的简单实现
- 简单的实现自定义轮播图加小圆点控件
- Android自定义View 简单实现多图片选择控件
- 简单的自定义组合控件 自定义属性
- 自定义Gallery控件实现简单3D图片浏览器
- 简单的自定义组合控件
- 自定义组合控件实现布局重用
- EasySegmentedBarView简单易用的自定义分段控件,方便快速实现分段效果
- Android使用自定义View实现购物车的加减
- 购物车自定义加减控件
- 选择对话框:自定义组合控件+自定义对话框 实现
- Android自定义组合控件的实现
- 购物车自定义加减控件
- Android进阶——自定义View之重写ViewGroup组合系统控件实现自定义ToolBar模板
- 【Android界面实现】自定义Gallery控件实现简单3D图片浏览器
- 自定义购物车加减控件