(安卓) 购物车二级列表,计算和全反选 以及 EventBus(消息传递)
2017-11-22 09:56
417 查看
先看效果图: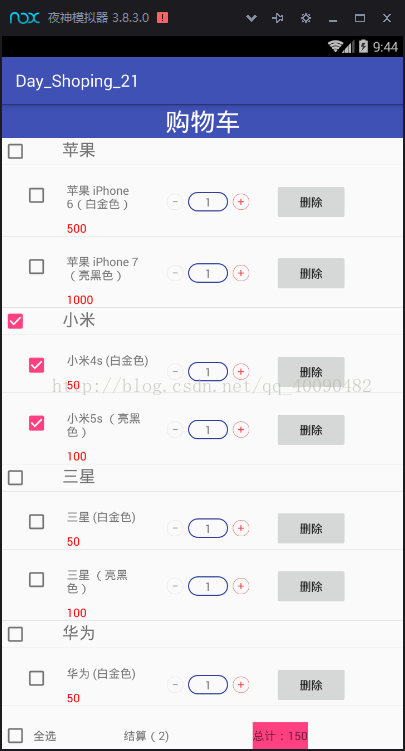
MainActivity;
import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.widget.CheckBox; import android.widget.ExpandableListView; import android.widget.TextView; import org.greenrobot.eventbus.EventBus; import org.greenrobot.eventbus.Subscribe; import java.util.ArrayList; import java.util.List; import bwie.com.day_shoping_21.adpader.MyAdapter; import bwie.com.day_shoping_21.bean.GoodsBean; import bwie.com.day_shoping_21.prasenter.Presaenter; import bwie.com.day_shoping_21.view.IView; public class MainActivity extends AppCompatActivity implements IView{ private ExpandableListView elv; private List<List<GoodsBean.DataBean.DatasBean>> data=new ArrayList<List<GoodsBean.DataBean.DatasBean>>(); private CheckBox mQuanxuan; private TextView zongjia; private TextView heji; private MyAdapter myAdapter; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); elv = (ExpandableListView) findViewById(R.id.elv); mQuanxuan = (CheckBox) findViewById(R.id.quanxuan); zongjia = (TextView) findViewById(R.id.zongji); heji = (TextView) findViewById(R.id.heji); Presaenter presaenter = new Presaenter(); presaenter.attachView(this); presaenter.getNews(); //注册EventBus EventBus.getDefault().register(this); mQuanxuan.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { //判断全选的状态 myAdapter.checkALLStatus(mQuanxuan.isChecked()); } }); } @Override public void success(List<GoodsBean.DataBean> news) { //遍历一级列表 for (int i = 0; i <news.size() ; i++) { GoodsBean.DataBean dataBean = news.get(i); //得到二级的对象 List<GoodsBean.DataBean.DatasBean> datas = dataBean.getDatas(); //将其添加到二级列表中 data.add(datas); } //适配器 myAdapter = new MyAdapter(this, news, data); //设置适配器 elv.setAdapter(myAdapter); //将一级列表的展开标志隐藏 elv.setGroupIndicator(null); //循环集合 for (int i = 0; i <news.size() ; i++) { //将二级列表展开 elv.expandGroup(i); } } @Override public void failed(Exception e) { } //接收适配器传过来的值 @Subscribe public void onMessageEvent(MessageEvent event) { //接收适配器(Myadpader)传递过来的值 ,将全选的状态设置为true或者false mQuanxuan.setChecked(event.isCheck()); } //接收适配器传过来的数量和总价 @Subscribe public void onMessageEvent(CountPrice event) { zongjia.setText("结算("+event.getCount()+")"+""); heji.setText("总计:"+event.getPrice()+""); } @Override protected void onDestroy() { super.onDestroy(); EventBus.getDefault().unregister(this); } }适配器所用到的类:
public class CountPrice { private int count; private int price; public int getCount() { return count; } public void setCount(int count) { this.count = count; } public int getPrice() { return price; } public void setPrice(int price) { this.price = price; } }
public class MessageEvent { private boolean check; public boolean isCheck() { return check; } public void setCheck(boolean check) { this.check = check; } }适配器:(主要判断都在这里)
import android.content.Context; import android.view.View; import android.view.ViewGroup; import android.widget.BaseExpandableListAdapter; import android.widget.Button; import android.widget.CheckBox; import android.widget.ImageView; import android.widget.TextView; import org.greenrobot.eventbus.EventBus; import java.util.List; import bwie.com.day_shoping_21.CountPrice; import bwie.com.day_shoping_21.Mess 12785 ageEvent; import bwie.com.day_shoping_21.R; import bwie.com.day_shoping_21.bean.GoodsBean; /** * Created by 迷人的脚毛!! on 2017/11/21. */ public class MyAdapter extends BaseExpandableListAdapter { private Context context; private List<GoodsBean.DataBean> list; private List<List<GoodsBean.DataBean.DatasBean>> data; public MyAdapter(Context context, List<GoodsBean.DataBean> list, List<List<GoodsBean.DataBean.DatasBean>> data) { this.list = list; this.context = context; this.data = data; } @Override public int getGroupCount() { return list.size(); } @Override public int getChildrenCount(int groupPosition) { return data.get(groupPosition).size(); } @Override public Object getGroup(int groupPosition) { return list.get(groupPosition); } @Override public Object getChild(int groupPosition, int childPosition) { return data.get(groupPosition).get(childPosition); } @Override public long getGroupId(int groupPosition) { return groupPosition; } @Override public long getChildId(int groupPosition, int childPosition) { return childPosition; } @Override public boolean hasStableIds() { return false; } @Override public View getGroupView(final int i, boolean b, View view, ViewGroup viewGroup) { final ViewHodler1 viewHodler1; if(view==null){ view=View.inflate(context, R.layout.a_view,null); viewHodler1=new ViewHodler1(); viewHodler1.name=view.findViewById(R.id.a_name); viewHodler1.abox=view.findViewById(R.id.a_box); view.setTag(viewHodler1); }else{ viewHodler1 = (ViewHodler1) view.getTag(); } final GoodsBean.DataBean dataBean = list.get(i); final String title = dataBean.getTitle(); viewHodler1.abox.setChecked(dataBean.isCheck()); viewHodler1.name.setText(title); //对一级的checkBox设置监听 viewHodler1.abox.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { //点击改变状态值 dataBean.setCheck(viewHodler1.abox.isChecked()); //通过一级的是否勾选,改变二级CheckBox状态 dataCheboxZT(i,viewHodler1.abox.isChecked()); EventBus.getDefault().post(jiSuan()); //通过判断是否全选,改变全选的状态 QuanxuangZT(listCheckboxQX()); notifyDataSetChanged(); } }); return view; } @Override public View getChildView(final int i, final int i1, boolean b, View view, ViewGroup viewGroup) { final ViewHodler2 viewHodler2; if(view==null){ view=View.inflate(context,R.layout.b_view,null); viewHodler2=new ViewHodler2(); viewHodler2.title=view.findViewById(R.id.b_title); viewHodler2.price=view.findViewById(R.id.b_price); viewHodler2.bbox=view.findViewById(R.id.b_box); viewHodler2.jia = view.findViewById(R.id.b_jia); viewHodler2.jian = view.findViewById(R.id.b_jian); viewHodler2.num = view.findViewById(R.id.b_num); viewHodler2.del = view.findViewById(R.id.b_del); view.setTag(viewHodler2); }else{ viewHodler2 = (ViewHodler2) view.getTag(); } final GoodsBean.DataBean.DatasBean datasBean = data.get(i).get(i1); viewHodler2.title.setText(datasBean.getType_name()); viewHodler2.price.setText(datasBean.getPrice()+""); viewHodler2.bbox.setChecked(datasBean.isCheck()); // viewHodler2.num.setText(datasBean.getCou()+""); //对二级里面的checkBox设置监听 viewHodler2.bbox.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { //二级的checkBox的点击时改变datasBean的属性 datasBean.setCheck(viewHodler2.bbox.isChecked()); datasBean.setCou(1); //计算勾选状态下的价格和数量 EventBus.getDefault().post(jiSuan()); //判断二级的CheckBox是全选,改变一级的checkBox listCheckboxZT(i,dataCheckboxZT(i)); //判断一级的checkbox是否选中,改变全选的状态值 QuanxuangZT(listCheckboxQX()); //刷新适配器 notifyDataSetChanged(); } }); //减法的点击事件 viewHodler2.jian.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { int num =datasBean.getCou(); if(num==1){ return; } //给控件赋值 viewHodler2.num.setText(--num+""); //给控件赋值以后在赋值给控件本身 datasBean.setCou(num); //当点击选中Checkbox的时候 if(viewHodler2.bbox.isChecked()){ //进行计算赋值传递给主MainActivity进行显示 EventBus.getDefault().post(jiSuan()); } notifyDataSetChanged(); } }); //加的点击事件 viewHodler2.jia.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // Toast.makeText(context, "0", Toast.LENGTH_SHORT).show(); int num = datasBean.getCou(); ++num; //给控件赋值 viewHodler2.num.setText(num+""); //当给控件赋值以后在赋给控件本身 datasBean.setCou(num); //当点击选中Checkbox的时候 if(viewHodler2.bbox.isChecked()){ //进行继续 EventBus.getDefault().post(jiSuan()); } notifyDataSetChanged(); } }); //删除的点击事件 viewHodler2.del.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { List<GoodsBean.DataBean.DatasBean> datasBeen = data.get(i); GoodsBean.DataBean.DatasBean remove = datasBeen.remove(i1); if(datasBeen.size()==0){ data.remove(i); list.remove(i); } EventBus.getDefault().post(jiSuan()); notifyDataSetChanged(); } }); return view; } @Override public boolean isChildSelectable(int i, int i1) { return true; } class ViewHodler1{ CheckBox abox; TextView name; } class ViewHodler2{ CheckBox bbox; TextView title,price,time,num; ImageView jia,jian; Button del; } /*** * 改变一级checkbox的状态 */ private void listCheckboxZT(int i,boolean flag){ GoodsBean.DataBean dataBean = list.get(i); dataBean.setCheck(flag); } /** * 改变全选的状态 */ private void QuanxuangZT(boolean flag){ MessageEvent messageEvent = new MessageEvent(); messageEvent.setCheck(flag); EventBus.getDefault().post(messageEvent); } /** * 改变二级checkbox的状态 */ private void dataCheboxZT(int i,boolean flag){ List<GoodsBean.DataBean.DatasBean> datasBeen = data.get(i); for (int j = 0; j < datasBeen.size(); j++) { datasBeen.get(j).setCheck(flag); } } /** * 判断一级的checkbox是否全部选中 */ private boolean listCheckboxQX(){ for (int i = 0; i <list.size() ; i++) { GoodsBean.DataBean dataBean = list.get(i); List<GoodsBean.DataBean.DatasBean> datas = dataBean.getDatas(); for (int j = 0; j < datas.size(); j++) { GoodsBean.DataBean.DatasBean datasBean = datas.get(j); if(!datasBean.isCheck()){ return false; } } } EventBus.getDefault().post(jiSuan()); return true; } /** * 判断二级的CheckBox是否全部选中 */ private boolean dataCheckboxZT(int i){ List<GoodsBean.DataBean.DatasBean> datasBeen = data.get(i); for (int j = 0; j < datasBeen.size(); j++) { if(!datasBeen.get(j).isCheck()){ return false; } } return true; } //==================================================================================================== /** * 计算选择中时的价格和数量 */ private CountPrice jiSuan(){ int cout=0; int price=0; for (int i = 0; i <list.size() ; i++) { GoodsBean.DataBean dataBean = list.get(i); List<GoodsBean.DataBean.DatasBean> datas = dataBean.getDatas(); for (int j = 0; j < datas.size(); j++) { GoodsBean.DataBean.DatasBean datasBean = datas.get(j); if(datasBean.isCheck()){ cout+=datasBean.getCou(); price+=datasBean.getPrice()*datasBean.getCou(); } } } CountPrice countPrice = new CountPrice(); countPrice.setCount(cout); countPrice.setPrice(price); return countPrice; } //=============================================== /** * 全选的时候改变所有的checkbox的状态 */ public void checkALLStatus(boolean flag){ for (int i = 0; i <list.size() ; i++) { GoodsBean.DataBean dataBean = list.get(i); dataBean.setCheck(flag); List<GoodsBean.DataBean.DatasBean> datas = dataBean.getDatas(); for (int j = 0; j < datas.size(); j++) { GoodsBean.DataBean.DatasBean datasBean = datas.get(j); datasBean.setCheck(flag); } } EventBus.getDefault().post(jiSuan()); notifyDataSetChanged(); } }==================================================================================================以上是主要类 接下来就是比较常写的一些解析类:
HttpUtils:
import android.os.Handler; import android.text.TextUtils; import android.util.Log; import java.io.IOException; import java.util.Map; import bwie.com.day_shoping_21.GsonUtlis; import okhttp3.Call; import okhttp3.Callback; import okhttp3.OkHttpClient; import okhttp3.Request; import okhttp3.Response; /** * Created by 迷人的脚毛!! on 2017/11/21. */ public class HttpUtils { private static final String TAG = "HttpUtils"; private static Handler handler=new Handler(); private static volatile HttpUtils instance; private HttpUtils(){ } public static HttpUtils getInstance() { if (null == instance) { synchronized (HttpUtils.class) { if (instance == null) { instance = new HttpUtils(); } } } return instance; } public void get(String url, Map<String,String> map, final CallBack callBack, final Class cls){ // http://www.baoidu.com/login?mobile=11111&password=11111&age=1&name=zw // 1.http://www.baoidu.com/login --------? key=value&key=value // 2.http://www.baoidu.com/login? --------- key=value&key=value // 3.http://www.baoidu.com/login?mobile=11111 -----&key=value&key=value if (TextUtils.isEmpty(url)){ return; } StringBuffer sb = new StringBuffer(); sb.append(url); if (url.contains("?")){ //如果包含并且是最后一位,就是第二种类型 if (url.indexOf("?")== url.length()-1){ }else{ //如果包含? 并且不是最后一位 sb.append("&"); } }else{ //不包含? 对应的是第一种类型 sb.append("?"); } //遍历map集合进行拼接,拼接的形式是key=values for(Map.Entry<String,String> entry:map.entrySet()){ sb.append(entry.getKey()) .append("=") .append(entry.getValue()) .append("&"); } //如果存在&号,把最后一个&去掉 if (sb.indexOf("&")!=-1){ // lastIndexOf 最后一个 sb.deleteCharAt(sb.lastIndexOf("&")); } Log.i(TAG, "get url: " + sb); OkHttpClient client = new OkHttpClient(); Request build = new Request.Builder() .get() .url(sb.toString()) .build(); Call call = client.newCall(build); call.enqueue(new Callback() { @Override public void onFailure(Call call, final IOException e) { handler.post(new Runnable() { @Override public void run() { //调用自己的回调接口回传回去 callBack.onFailed(e); } }); } @Override public void onResponse(Call call, Response response) throws IOException { final String reutl = response.body().string(); //请求成功之后解析,通过自己的回调接口将数据传输回去 handler.post(new Runnable() { @Override public void run() { Object o; //如果是空的话 if (TextUtils.isEmpty(reutl)){ o=null; Log.i(TAG,"为空!!!!!!!!!"+o); }else{ o = GsonUtlis.getInstance().fromJson(reutl, cls); Log.i(TAG,"不不不不不不为空!!!!!!!!!"+o); } callBack.onSuccess(o); } }); } }); } }模型层接口:
public interface CallBack { void onSuccess( Object o); void onFailed( Exception e); }视图层接口:
public interface IView { void success( List<GoodsBean.DataBean> news); void failed( Exception e); }P层
public class Presaenter { private IView iv; public void attachView(IView iv){ this.iv=iv; } public void getNews(){ HashMap<String, String > map = new HashMap<>(); map.put("uri", "evaluation"); HttpUtils.getInstance().get("http://result.eolinker.com/iYXEPGn4e9c6dafce6e5cdd23287d2bb136ee7e9194d3e9", map, new CallBack() { @Override public void onSuccess(Object o) { GoodsBean bean= (GoodsBean) o; if(bean!=null){ List<GoodsBean.DataBean> data = bean.getData(); iv.success(data); } } @Override public void onFailed(Exception e) { iv.failed(e); } },GoodsBean.class); } //定义一个方法让iv等于空 防止内存泄漏问题 public void detachView() { if (iv != null) { iv = null; } } }============================================================================
接下来就是布局了:
MainActivity.xml
<?xml version="1.0" encoding="utf-8"?><LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"xmlns:tools="http://schemas.android.com/tools"android:id="@+id/activity_main"android:layout_width="match_parent"android:layout_height="match_parent"android:layout_marginTop="0dp"android:orientation="vertical"tools:context="bwie.com.day_shoping_21.MainActivity"><TextViewandroid:layout_gravity="center_horizontal"android:textSize="30dp"android:layout_marginTop="0dp"android:gravity="center"android:background="@color/colorPrimary"android:textColor="#fff"android:layout_width="match_parent"android:layout_height="wrap_content"android:text="购物车"android:id="@+id/textView" /><ExpandableListViewandroid:id="@+id/elv"android:layout_weight="2"android:layout_width="match_parent"android:layout_height="match_parent"></ExpandableListView><LinearLayoutandroid:layout_width="match_parent"android:layout_height="wrap_content"android:orientation="horizontal"><CheckBoxandroid:id="@+id/quanxuan"android:layout_width="wrap_content"android:layout_height="wrap_content" /><TextViewandroid:layout_width="wrap_content"android:layout_height="wrap_content"android:layout_marginLeft="5dp"android:text="全选" /><TextViewandroid:id="@+id/zongji"android:layout_width="wrap_content"android:layout_height="wrap_content"android:layout_marginLeft="80dp"android:text="合计:0" /><TextViewandroid:id="@+id/heji"android:layout_width="wrap_content"android:layout_height="match_parent"android:layout_marginLeft="100dp"android:background="@color/colorAccent"android:gravity="center"android:text="结算(0)" /></LinearLayout></LinearLayout>一级布局:
<?xml version="1.0" encoding="utf-8"?><LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"android:orientation="horizontal" android:layout_width="match_parent"android:layout_height="match_parent"android:layout_marginTop="30dp"><CheckBoxandroid:id="@+id/a_box"android:layout_width="wrap_content"android:layout_height="wrap_content" /><TextViewandroid:textSize="20dp"android:id="@+id/a_name"android:layout_marginLeft="40dp"android:layout_width="wrap_content"android:layout_height="wrap_content" /></LinearLayout>二级布局
<?xml version="1.0" encoding="utf-8"?><LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"android:orientation="horizontal" android:layout_width="match_parent"android:layout_height="match_parent"android:layout_marginTop="30dp"><CheckBoxandroid:layout_marginTop="20dp"android:id="@+id/b_box"android:layout_marginLeft="25dp"android:layout_width="wrap_content"android:layout_height="wrap_content" /><LinearLayoutandroid:layout_marginTop="20dp"android:layout_width="wrap_content"android:layout_height="wrap_content"android:orientation="vertical"><TextViewandroid:id="@+id/b_title"android:layout_width="100dp"android:layout_height="wrap_content"android:layout_marginLeft="20dp" /><TextViewandroid:layout_marginTop="10dp"android:id="@+id/b_price"android:textColor="#f00"android:layout_width="wrap_content"android:layout_height="wrap_content"android:layout_marginLeft="20dp" /></LinearLayout><LinearLayoutandroid:layout_marginTop="20dp"android:layout_marginLeft="20dp"android:orientation="horizontal"android:layout_width="match_parent"android:layout_height="wrap_content"android:gravity="center_vertical"><ImageViewandroid:id="@+id/b_jian"android:layout_width="20dp"android:layout_height="20dp"android:src="@drawable/shopcart_minus_grey" /><TextViewandroid:id="@+id/b_num"android:layout_width="wrap_content"android:layout_height="wrap_content"android:layout_marginLeft="5dp"android:background="@drawable/shopcart_add_btn"android:paddingBottom="2dp"android:paddingLeft="20dp"android:paddingRight="20dp"android:paddingTop="2dp"android:text="1" /><ImageViewandroid:id="@+id/b_jia"android:layout_width="20dp"android:layout_height="20dp"android:layout_marginLeft="5dp"android:src="@drawable/shopcart_add_red" /><Buttonandroid:id="@+id/b_del"android:text="删除"android:layout_marginLeft="30dp"android:layout_width="wrap_content"android:layout_height="wrap_content" /></LinearLayout></LinearLayout>依赖:EventBus(消息传递)
compile 'org.greenrobot:eventbus:3.1.1'=============================================================================================一下是加了自定义控件的二级列表的xml:
<?xml version="1.0" encoding="utf-8"?><LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"android:orientation="horizontal" android:layout_width="match_parent"android:layout_height="match_parent"android:layout_marginTop="30dp"><CheckBoxandroid:layout_marginTop="20dp"android:id="@+id/b_box"android:layout_marginLeft="25dp"android:layout_width="wrap_content"android:layout_height="wrap_content" /><LinearLayoutandroid:layout_marginTop="20dp"android:layout_width="wrap_content"android:layout_height="wrap_content"android:orientation="vertical"><TextViewandroid:id="@+id/b_title"android:layout_width="100dp"android:layout_height="wrap_content"android:layout_marginLeft="20dp" /><TextViewandroid:layout_marginTop="10dp"android:id="@+id/b_price"android:textColor="#f00"android:layout_width="wrap_content"android:layout_height="wrap_content"android:layout_marginLeft="20dp" /></LinearLayout><LinearLayoutandroid:layout_width="match_parent"android:layout_height="wrap_content"android:layout_marginLeft="20dp"android:layout_marginTop="20dp"android:gravity="center_vertical"android:orientation="horizontal"><bwie.com.day_shoping_1219.AddDelViewandroid:layout_width="126dp"android:layout_height="match_parent"android:id="@+id/ade"></bwie.com.day_shoping_1219.AddDelView><Buttonandroid:id="@+id/b_del"android:layout_width="wrap_content"android:layout_height="wrap_content"android:layout_marginLeft="30dp"android:text="删除" /></LinearLayout></LinearLayout>适配器:
package bwie.com.day_shoping_1219.adpater;import android.content.Context;import android.view.View;import android.view.ViewGroup;import android.widget.BaseExpandableListAdapter;import android.widget.Button;import android.widget.CheckBox;import android.widget.TextView;import org.greenrobot.eventbus.EventBus;import java.util.List;import bwie.com.day_shoping_1219.AddDelView;import bwie.com.day_shoping_1219.CountPrice;import bwie.com.day_shoping_1219.MessageEvent;import bwie.com.day_shoping_1219.R;import bwie.com.day_shoping_1219.bean.UserBean;import static bwie.com.day_shoping_1219.R.id.ade;/*** Created by 迷人的脚毛!! on 2017/12/19.*/public class MyAdapter extends BaseExpandableListAdapter {private Context context;private List<UserBean.DataBean> list;private List<List<UserBean.DataBean.ListBean>> data;public MyAdapter(Context context, List<UserBean.DataBean> list, List<List<UserBean.DataBean.ListBean>> data) {this.context = context;this.list = list;this.data = data;}@Overridepublic int getGroupCount() {return list.size();}@Overridepublic int getChildrenCount(int i) {return data.get(i).size();}@Overridepublic Object getGroup(int i) {return list.get(i);}@Overridepublic Object getChild(int i, int i1) {return data.get(i).get(i1);}@Overridepublic long getGroupId(int i) {return i;}@Overridepublic long getChildId(int i, int i1) {return i1;}@Overridepublic boolean hasStableIds() {return false;}@Overridepublic View getGroupView(final int i, boolean b, View view, ViewGroup viewGroup) {final ViewHodler1 viewHodler1;if (view==null){view = View.inflate(context, R.layout.yiji, null);viewHodler1 = new ViewHodler1();viewHodler1.name=view.findViewById(R.id.a_name);viewHodler1.abox=view.findViewById(R.id.a_box);viewHodler1.bianji=view.findViewById(R.id.bianji);view.setTag(viewHodler1);}else{viewHodler1 = (ViewHodler1) view.getTag();}final UserBean.DataBean dataBean = list.get(i);viewHodler1.name.setText(dataBean.getSellerName());viewHodler1.abox.setChecked(dataBean.isCheck());//对一级的checkbox设置监听viewHodler1.abox.setOnClickListener(new View.OnClickListener() {@Overridepublic void onClick(View view) {//点击改变状态值dataBean.setCheck(viewHodler1.abox.isChecked());//通过一级的手否勾选,改变二级的checkbox状态dataCheckboxZT(i,viewHodler1.abox.isChecked());//通过判断是否全选,改变全选的状态QuanxuangZT(listCheckboxQX());notifyDataSetChanged();}});return view;}@Overridepublic View getChildView(final int i, final int i1, boolean b, View view, ViewGroup viewGroup) {final ViewHodler2 viewHodler2;if(view==null){view=View.inflate(context,R.layout.erji,null);viewHodler2=new ViewHodler2();viewHodler2.title=view.findViewById(R.id.b_title);viewHodler2.price=view.findViewById(R.id.b_price);viewHodler2.bbox=view.findViewById(R.id.b_box);viewHodler2.del = view.findViewById(R.id.b_del);viewHodler2.ade=view.findViewById(ade);view.setTag(viewHodler2);}else{viewHodler2 = (ViewHodler2) view.getTag();}final UserBean.DataBean.ListBean datasBean = data.get(i).get(i1);viewHodler2.title.setText(datasBean.getTitle());viewHodler2.price.setText(datasBean.getPrice()+"");viewHodler2.bbox.setChecked(datasBean.isCheck());//对二级里面的checkBox设置监听viewHodler2.bbox.setOnClickListener(new View.OnClickListener() {@Overridepublic void onClick(View view) {//二级的checkbox的点击时改变datatbean的属性datasBean.setCheck(viewHodler2.bbox.isChecked());//计算勾选状态下的价格和数量EventBus.getDefault().post(jiSuan());//判断二级的checkbox是全选,改变一级的checkboxlistCheckboxZT(i,dataCheckboxZT(i));//判断一级的checkbox是否选中,改变全选的状态值QuanxuangZT(listCheckboxQX());//刷新适配器notifyDataSetChanged();}});//自定义控件的加减以及EditText 。这三个的回调接口viewHodler2.ade.setOnAddDelClickLinstener(new AddDelView.OnAddDelClickLinstener() {@Overridepublic void onAddClick(View v) {int num = datasBean.getCou();++num;//给控件赋值viewHodler2.ade.setNumber(num);//当给控件赋值以后在赋给控件本身datasBean.setCou(num);//当点击选中Checkbox的时候if(viewHodler2.bbox.isChecked()){//进行继续EventBus.getDefault().post(jiSuan());}notifyDataSetChanged();}@Overridepublic void onDelClick(View v) {int num = datasBean.getCou();if (num==1){return;}//给控件赋值viewHodler2.ade.setNumber(--num);//给控件赋值以后赋值给控件本身datasBean.setCou(num);if(viewHodler2.bbox.isChecked()){//进行计算赋值传递给主MainActivity进行显示EventBus.getDefault().post(jiSuan());}notifyDataSetChanged();}});//删除的点击事件viewHodler2.del.setOnClickListener(new View.OnClickListener() {@Overridepublic void onClick(View view) {List<UserBean.DataBean.ListBean> listBeen = data.get(i);UserBean.DataBean.ListBean remove = listBeen.remove(i1);if (listBeen.size()==0){data.remove(i);list.remove(i);}EventBus.getDefault().post(jiSuan());notifyDataSetChanged();}});return view;}@Overridepublic boolean isChildSelectable(int i, int i1) {return false;}class ViewHodler1{CheckBox abox;TextView name,bianji;}class ViewHodler2{CheckBox bbox;TextView title,price;Button del;AddDelView ade;}//改变一级的checkbox的状态private void listCheckboxZT(int i,boolean flag){UserBean.DataBean dataBean = list.get(i);dataBean.setCheck(flag);}//改变二级的checkbox的状态private void dataCheckboxZT(int i,boolean flag){List<UserBean.DataBean.ListBean> listBeen = data.get(i);for (int j = 0; j <listBeen.size() ; j++) {listBeen.get(j).setCheck(flag);}}//改变全选状态private void QuanxuangZT(boolean flag){MessageEvent messageEvent = new MessageEvent();messageEvent.setCheck(flag);EventBus.getDefault().post(messageEvent);}//判断一级的checkbox是否全部选中private boolean listCheckboxQX(){for (int i = 0; i <list.size() ; i++) {UserBean.DataBean dataBean = list.get(i);List<UserBean.DataBean.ListBean> lista = dataBean.getList();for (int j = 0; j <lista.size() ; j++) {UserBean.DataBean.ListBean listBean = lista.get(j);if (!listBean.isCheck()){return false;}}}EventBus.getDefault().post(jiSuan());return true;}//判断二级的checkbox是否全部选中private boolean dataCheckboxZT(int i){List<UserBean.DataBean.ListBean> listBeen = data.get(i);for (int j = 0; j <listBeen.size() ; j++) {if (!listBeen.get(j).isCheck()){return false;}}return true;}private CountPrice jiSuan(){int cout=0;int price=0;for (int i = 0; i <list.size() ; i++) {UserBean.DataBean dataBean = list.get(i);List<UserBean.DataBean.ListBean> datas = dataBean.getList();for (int j = 0; j < datas.size(); j++) {UserBean.DataBean.ListBean datasBean = datas.get(j);if(datasBean.isCheck()){cout+=datasBean.getCou();price+=datasBean.getPrice()*datasBean.getCou();}}}CountPrice countPrice = new CountPrice();countPrice.setCount(cout);countPrice.setPrice(price);return countPrice;}//===============================================/*** 全选的时候改变所有的checkbox的状态*/public void checkALLStatus(boolean flag){for (int i = 0; i <list.size() ; i++) {UserBean.DataBean dataBean = list.get(i);dataBean.setCheck(flag);List<UserBean.DataBean.ListBean> datas = dataBean.getList();for (int j = 0; j < datas.size(); j++) {UserBean.DataBean.ListBean datasBean = datas.get(j);datasBean.setCheck(flag);}}EventBus.getDefault().post(jiSuan());notifyDataSetChanged();}}增加的一点小业务逻辑 自己套用把 点击编辑显示和隐藏控件:主要逻辑:private int isxian=View.VISIBLE;
//在一级列表里面点击时viewHodler1.bianji.setOnClickListener(new View.OnClickListener() {@Overridepublic void onClick(View view) {if(isxian==View.VISIBLE) {isxian=View.GONE;}else{isxian=View.VISIBLE;}notifyDataSetChanged();}});
//在二级列表找到需要隐藏的控件给赋值viewHodler2.del.setVisibility(isxian);
相关文章推荐
- (安卓) 购物车一级列表,计算和全反选 以及 EventBus(消息传递)
- Android仿京东App购物车 二级列表+全选反选+Ok封装+拦截器+结算+商品数量计算
- Android仿京东App购物车 二级列表+全选反选+Ok封装+拦截器+结算+商品数量计算
- 安卓二级列表购物车 略屌略屌 一个类足矣
- Android_二级列表购物车之增删改查,全选反选,加减器,价钱数量计算
- Android_二级列表购物车之增删改查,全选反选,加减器,价钱数量计算
- 仿购物车二级列表Expandablelistview以及价格
- SurfaceView(包含对消息传递的回顾以及对继承View类使用的回顾)制作指南针
- 查询购物车---二级列表实现
- 二级列表完美实现购物车
- 二级列表购物车的全选反选
- 仿京东中购物车列表模块的实现【以及通过Builder的方式创建dialog弹窗 链式调用】
- Andriodjie——二级列表实现购物车
- 二级列表RecyclerView购物车
- WM_ERASEBKGND官方解释(翻译),以及Delphi里所有的使用情况(就是绘制窗口控件背景色,并阻止进一步传递消息)
- EventBus的使用以及消息处理
- Android之利用EventBus发送消息传递示例
- 安卓——WIFI列表以及点击事件
- RecyclerView 购物车 二级列表
- 购物车二级列表