两个进程之间的通信
2017-11-17 16:00
471 查看
下面写一下我做的一个两个App进程之间的通信方式,用一个简单的例子来体现
相当于一个加法计算器:
首先这里需要创建两个modle,一个是客户端App,一个是服务端App
这里用的是dataBinding的写法,所以需要在grid中写个配置
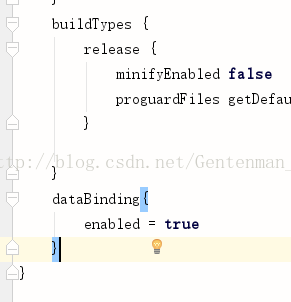
还有一个自己封装的工具包:
TypeCast:
public class TypeCast {
public static final String TAG = "TypeCast";
/**
* Convert Object to Integer
* 注:
* TypeCast.toInt(null, -1)); // Return:-1,Not:0
* TypeCast.toInt("", -1)); // Return:-1,Not:0
*
* @param value a Object
* @param defaultValue Value to return if value is null、empty or convert failure.
* @return Returns the converted value if it exists, or defaultValue
*/
public static int toInt(Object value, int defaultValue) {
if (value == null) {
return defaultValue;
}
try {
return Integer.valueOf(value.toString());
} catch (NumberFormatException e1) {
// Log.e(TAG, "toInt() Convert String to Integer, defaultValue: " + defaultValue, e1);
try {
return Double.valueOf(value.toString()).intValue();
} catch (NumberFormatException e2) {
// Log.e(TAG, "toInt() Convert String to Double, defaultValue: " + defaultValue, e2);
return defaultValue;
}
}
}
/**
* Convert Object to Long
* 注:
* TypeCast.toLong(null, -1)); // Return:-1,Not:0
* TypeCast.toLong("", -1)); // Return:-1,Not:0
*
* @param value a Object
* @param defaultValue Value to return if value is null、empty or convert failure.
* @return Returns the converted value if it exists, or defaultValue
*/
public static long toLong(Object value, long defaultValue) {
if (value == null) {
return defaultValue;
}
try {
return Long.valueOf(value.toString());
} catch (NumberFormatException e1) {
// Logger.e(TAG, "toLong() Convert String to Long, defaultValue: " + defaultValue, e1);
try {
return Double.valueOf(value.toString()).longValue();
} catch (NumberFormatException e2) {
// Logger.e(TAG, "toLong() Convert String to Double, defaultValue: " + defaultValue, e2);
return defaultValue;
}
}
}
/**
* Convert Object to Float
* 注:
* TypeCast.toFloat(null, -1.0f)); // Return:-1.0f,Not:0
* TypeCast.toFloat("", -1.0f)); // Return:-1.0f,Not:0
*
* @param value a Object
* @param defaultValue Value to return if value is null、empty or convert failure.
* @return Returns the converted value if it exists, or defaultValue
*/
public static float toFloat(Object value, float defaultValue) {
if (value == null) {
return defaultValue;
}
try {
return Float.valueOf(value.toString());
} catch (NumberFormatException e) {
// Logger.e(TAG, "toFloat() Convert String to Float, defaultValue: " + defaultValue, e);
return defaultValue;
}
}
/**
* Convert Object to Double
* 注:
* TypeCast.toDouble(null, -1.0)); // Return:-1.0,Not:0
* TypeCast.toDouble("", -1.0)); // Return:-1.0,Not:0
*
* @param value a Object
* @param defaultValue Value to return if value is null、empty or convert failure.
* @return Returns the converted value if it exists, or defaultValue
*/
public static double toDouble(Object value, double defaultValue) {
if (value == null) {
return defaultValue;
}
try {
return Double.valueOf(value.toString());
} catch (NumberFormatException e) {
// Logger.e(TAG, "toDouble() Convert String to Double, defaultValue: " + defaultValue, e);
return defaultValue;
}
}
}
客户端:
在这里先把values的东西写上:
colors:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="colorPrimary">#3F51B5</color>
<color name="colorPrimaryDark">#303F9F</color>
<color name="colorAccent">#FF4081</color>
</resources>
dimens:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<dimen name="wrapper_vertical_margin">16dp</dimen>
<dimen name="wrapper_horizontal_margin">16dp</dimen>
<dimen name="line_height">64dp</dimen>
</resources> strings:
<resources>
<string name="app_name">CalculatorClient</string>
<string name="calculator_button">求和计算</string>
<string name="calculator_result_label">结果:</string>
<string name="calculator_result">0</string>
<string name="calculator_num1_label">Num1:</string>
<string name="calculator_num2_label">Num2:</string>
</resources>
styles:
<resources>
<!-- Base application theme. -->
<style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar">
<!-- Customize your theme here. -->
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
</style>
<style name="calculator_wrapper">
<item name="android:layout_width">match_parent</item>
<item name="android:layout_height">@dimen/line_height</item>
<item name="android:orientation">horizontal</item>
</style>
<style name="calculator_label">
<item name="android:layout_width">0dp</item>
<item name="android:layout_height">match_parent</item>
<item name="android:layout_weight">3</item>
<item name="android:gravity">center_vertical|end</item>
<item name="android:paddingBottom">@dimen/wrapper_vertical_margin</item>
<item name="android:paddingLeft">@dimen/wrapper_horizontal_margin</item>
<item name="android:paddingRight">@dimen/wrapper_horizontal_margin</item>
<item name="android:paddingTop">@dimen/wrapper_vertical_margin</item>
<item name="android:textSize">24sp</item>
</style>
<style name="calculator_value">
<item name="android:layout_width">0dp</item>
<item name="android:layout_height">match_parent</item>
<item name="android:layout_weight">5</item>
<item name="android:gravity">center_vertical</item>
<item name="android:paddingBottom">@dimen/wrapper_vertical_margin</item>
<item name="android:paddingLeft">@dimen/wrapper_horizontal_margin</item>
<item name="android:paddingRight">@dimen/wrapper_horizontal_margin</item>
<item name="android:paddingTop">@dimen/wrapper_vertical_margin</item>
<item name="android:textSize">24sp</item>
<item name="android:inputType">number</item>
</style>
<style name="calculator_button">
<item name="android:layout_width">match_parent</item>
<item name="android:layout_height">@dimen/line_height</item>
<item name="android:text">@string/calculator_button</item>
<item name="android:textSize">24sp</item>
</style>
</resources>
主界面的 xml
import android.app.Service;
import android.content.ComponentName;
import android.content.Intent;
import android.content.ServiceConnection;
import android.databinding.DataBindingUtil;
import android.os.Bundle;
import android.os.IBinder;
import android.os.RemoteException;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.view.View;
import com.bwei.czx.service.IMyAidlInterface;
import com.bwei.czx.service33.databinding.ActivityMainBinding;
public class MainActivity extends AppCompatActivity {
ActivityMainBinding mainBinding;
IMyAidlInterface mInterface;
ServiceConnection mConn = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName componentName, IBinder iBinder) {
mInterface = IMyAidlInterface.Stub.asInterface(iBinder);
}
@Override
public void onServiceDisconnected(ComponentName componentName) {
mInterface = null;
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
mainBinding = DataBindingUtil.setContentView(this, R.layout.activity_main);
Intent intent = new Intent();
MainActivity中:
import android.app.Service;
import android.content.ComponentName;
import android.content.Intent;
import android.content.ServiceConnection;
import android.databinding.DataBindingUtil;
import android.os.Bundle;
import android.os.IBinder;
import android.os.RemoteException;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.view.View;
import com.bwei.czx.service.IMyAidlInterface;
import com.bwei.czx.service33.databinding.ActivityMainBinding;
public class MainActivity extends AppCompatActivity {
ActivityMainBinding mainBinding;
IMyAidlInterface mInterface;
ServiceConnection mConn = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName componentName, IBinder iBinder) {
mInterface = IMyAidlInterface.Stub.asInterface(iBinder);
}
@Override
public void onServiceDisconnected(ComponentName componentName) {
mInterface = null;
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
mainBinding = DataBindingUtil.setContentView(this, R.layout.activity_main);
Intent intent = new Intent();
//输入包名,第二个输入包名.服务端那边的服务名
intent.setComponent(new ComponentName("com.bwei.czx.service","com.bwei.czx.service.CalculatorService"));
bindService(intent,mConn, Service.BIND_AUTO_CREATE);
mainBinding.calculatorButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
int num1 = TypeCast.toInt(mainBinding.etNum1.getText().toString(),0);
int num2 = TypeCast.toInt(mainBinding.etNum2.getText().toString(),0);
Log.i("=======","num1="+num1+"num2="+num2);
try {
//防御性判断
if(mInterface != null){
int result = mInterface.add(num1,num2);
mainBinding.tvResult.setText(String.valueOf(result));
}
} catch (RemoteException e) {
e.printStackTrace();
}
}
});
}
@Override
protected void onDestroy() {
if(mInterface != null){
unbindService(mConn);
}
super.onDestroy();
}
}
服务端:
CalculatorService
import android.app.Service;
import android.content.Intent;
import android.os.IBinder;
import android.os.RemoteException;
public class CalculatorService extends Service {
private IBinder mBinder = new IMyAidlInterface.Stub() {
@Override
public int add(int num1, int num2) throws RemoteException {
return num1 + num2;
}
};
@Override
public IBinder onBind(Intent intent) {
return mBinder;
}
}
然后在服务端创建一个aidl
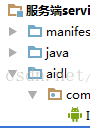
interface IMyAidlInterface {
int add(int num1,int num2);
}
之后把服务端创建的aidl复制,粘贴到客户端里面。
写完就可以运行了。
相当于一个加法计算器:
首先这里需要创建两个modle,一个是客户端App,一个是服务端App
这里用的是dataBinding的写法,所以需要在grid中写个配置
还有一个自己封装的工具包:
TypeCast:
public class TypeCast {
public static final String TAG = "TypeCast";
/**
* Convert Object to Integer
* 注:
* TypeCast.toInt(null, -1)); // Return:-1,Not:0
* TypeCast.toInt("", -1)); // Return:-1,Not:0
*
* @param value a Object
* @param defaultValue Value to return if value is null、empty or convert failure.
* @return Returns the converted value if it exists, or defaultValue
*/
public static int toInt(Object value, int defaultValue) {
if (value == null) {
return defaultValue;
}
try {
return Integer.valueOf(value.toString());
} catch (NumberFormatException e1) {
// Log.e(TAG, "toInt() Convert String to Integer, defaultValue: " + defaultValue, e1);
try {
return Double.valueOf(value.toString()).intValue();
} catch (NumberFormatException e2) {
// Log.e(TAG, "toInt() Convert String to Double, defaultValue: " + defaultValue, e2);
return defaultValue;
}
}
}
/**
* Convert Object to Long
* 注:
* TypeCast.toLong(null, -1)); // Return:-1,Not:0
* TypeCast.toLong("", -1)); // Return:-1,Not:0
*
* @param value a Object
* @param defaultValue Value to return if value is null、empty or convert failure.
* @return Returns the converted value if it exists, or defaultValue
*/
public static long toLong(Object value, long defaultValue) {
if (value == null) {
return defaultValue;
}
try {
return Long.valueOf(value.toString());
} catch (NumberFormatException e1) {
// Logger.e(TAG, "toLong() Convert String to Long, defaultValue: " + defaultValue, e1);
try {
return Double.valueOf(value.toString()).longValue();
} catch (NumberFormatException e2) {
// Logger.e(TAG, "toLong() Convert String to Double, defaultValue: " + defaultValue, e2);
return defaultValue;
}
}
}
/**
* Convert Object to Float
* 注:
* TypeCast.toFloat(null, -1.0f)); // Return:-1.0f,Not:0
* TypeCast.toFloat("", -1.0f)); // Return:-1.0f,Not:0
*
* @param value a Object
* @param defaultValue Value to return if value is null、empty or convert failure.
* @return Returns the converted value if it exists, or defaultValue
*/
public static float toFloat(Object value, float defaultValue) {
if (value == null) {
return defaultValue;
}
try {
return Float.valueOf(value.toString());
} catch (NumberFormatException e) {
// Logger.e(TAG, "toFloat() Convert String to Float, defaultValue: " + defaultValue, e);
return defaultValue;
}
}
/**
* Convert Object to Double
* 注:
* TypeCast.toDouble(null, -1.0)); // Return:-1.0,Not:0
* TypeCast.toDouble("", -1.0)); // Return:-1.0,Not:0
*
* @param value a Object
* @param defaultValue Value to return if value is null、empty or convert failure.
* @return Returns the converted value if it exists, or defaultValue
*/
public static double toDouble(Object value, double defaultValue) {
if (value == null) {
return defaultValue;
}
try {
return Double.valueOf(value.toString());
} catch (NumberFormatException e) {
// Logger.e(TAG, "toDouble() Convert String to Double, defaultValue: " + defaultValue, e);
return defaultValue;
}
}
}
客户端:
在这里先把values的东西写上:
colors:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="colorPrimary">#3F51B5</color>
<color name="colorPrimaryDark">#303F9F</color>
<color name="colorAccent">#FF4081</color>
</resources>
dimens:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<dimen name="wrapper_vertical_margin">16dp</dimen>
<dimen name="wrapper_horizontal_margin">16dp</dimen>
<dimen name="line_height">64dp</dimen>
</resources> strings:
<resources>
<string name="app_name">CalculatorClient</string>
<string name="calculator_button">求和计算</string>
<string name="calculator_result_label">结果:</string>
<string name="calculator_result">0</string>
<string name="calculator_num1_label">Num1:</string>
<string name="calculator_num2_label">Num2:</string>
</resources>
styles:
<resources>
<!-- Base application theme. -->
<style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar">
<!-- Customize your theme here. -->
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
</style>
<style name="calculator_wrapper">
<item name="android:layout_width">match_parent</item>
<item name="android:layout_height">@dimen/line_height</item>
<item name="android:orientation">horizontal</item>
</style>
<style name="calculator_label">
<item name="android:layout_width">0dp</item>
<item name="android:layout_height">match_parent</item>
<item name="android:layout_weight">3</item>
<item name="android:gravity">center_vertical|end</item>
<item name="android:paddingBottom">@dimen/wrapper_vertical_margin</item>
<item name="android:paddingLeft">@dimen/wrapper_horizontal_margin</item>
<item name="android:paddingRight">@dimen/wrapper_horizontal_margin</item>
<item name="android:paddingTop">@dimen/wrapper_vertical_margin</item>
<item name="android:textSize">24sp</item>
</style>
<style name="calculator_value">
<item name="android:layout_width">0dp</item>
<item name="android:layout_height">match_parent</item>
<item name="android:layout_weight">5</item>
<item name="android:gravity">center_vertical</item>
<item name="android:paddingBottom">@dimen/wrapper_vertical_margin</item>
<item name="android:paddingLeft">@dimen/wrapper_horizontal_margin</item>
<item name="android:paddingRight">@dimen/wrapper_horizontal_margin</item>
<item name="android:paddingTop">@dimen/wrapper_vertical_margin</item>
<item name="android:textSize">24sp</item>
<item name="android:inputType">number</item>
</style>
<style name="calculator_button">
<item name="android:layout_width">match_parent</item>
<item name="android:layout_height">@dimen/line_height</item>
<item name="android:text">@string/calculator_button</item>
<item name="android:textSize">24sp</item>
</style>
</resources>
主界面的 xml
import android.app.Service;
import android.content.ComponentName;
import android.content.Intent;
import android.content.ServiceConnection;
import android.databinding.DataBindingUtil;
import android.os.Bundle;
import android.os.IBinder;
import android.os.RemoteException;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.view.View;
import com.bwei.czx.service.IMyAidlInterface;
import com.bwei.czx.service33.databinding.ActivityMainBinding;
public class MainActivity extends AppCompatActivity {
ActivityMainBinding mainBinding;
IMyAidlInterface mInterface;
ServiceConnection mConn = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName componentName, IBinder iBinder) {
mInterface = IMyAidlInterface.Stub.asInterface(iBinder);
}
@Override
public void onServiceDisconnected(ComponentName componentName) {
mInterface = null;
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
mainBinding = DataBindingUtil.setContentView(this, R.layout.activity_main);
Intent intent = new Intent();
//这里写服务类的包名和包名.类名 intent.setComponent(new ComponentName("com.czx.service","com.czx.service.CalculatorService")); bindService(intent,mConn, Service.BIND_AUTO_CREATE); mainBinding.calculatorButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { int num1 = TypeCast.toInt(mainBinding.etNum1.getText().toString(),0); int num2 = TypeCast.toInt(mainBinding.etNum2.getText().toString(),0); Log.i("=======","num1="+num1+"num2="+num2); try { if(mInterface != null){ int result = mInterface.add(num1,num2); mainBinding.tvResult.setText(String.valueOf(result)); } } catch (RemoteException e) { e.printStackTrace(); } } }); } @Override protected void onDestroy() { if(mInterface != null){ unbindService(mConn); } super.onDestroy(); } }
MainActivity中:
import android.app.Service;
import android.content.ComponentName;
import android.content.Intent;
import android.content.ServiceConnection;
import android.databinding.DataBindingUtil;
import android.os.Bundle;
import android.os.IBinder;
import android.os.RemoteException;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.view.View;
import com.bwei.czx.service.IMyAidlInterface;
import com.bwei.czx.service33.databinding.ActivityMainBinding;
public class MainActivity extends AppCompatActivity {
ActivityMainBinding mainBinding;
IMyAidlInterface mInterface;
ServiceConnection mConn = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName componentName, IBinder iBinder) {
mInterface = IMyAidlInterface.Stub.asInterface(iBinder);
}
@Override
public void onServiceDisconnected(ComponentName componentName) {
mInterface = null;
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
mainBinding = DataBindingUtil.setContentView(this, R.layout.activity_main);
Intent intent = new Intent();
//输入包名,第二个输入包名.服务端那边的服务名
intent.setComponent(new ComponentName("com.bwei.czx.service","com.bwei.czx.service.CalculatorService"));
bindService(intent,mConn, Service.BIND_AUTO_CREATE);
mainBinding.calculatorButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
int num1 = TypeCast.toInt(mainBinding.etNum1.getText().toString(),0);
int num2 = TypeCast.toInt(mainBinding.etNum2.getText().toString(),0);
Log.i("=======","num1="+num1+"num2="+num2);
try {
//防御性判断
if(mInterface != null){
int result = mInterface.add(num1,num2);
mainBinding.tvResult.setText(String.valueOf(result));
}
} catch (RemoteException e) {
e.printStackTrace();
}
}
});
}
@Override
protected void onDestroy() {
if(mInterface != null){
unbindService(mConn);
}
super.onDestroy();
}
}
服务端:
CalculatorService
import android.app.Service;
import android.content.Intent;
import android.os.IBinder;
import android.os.RemoteException;
public class CalculatorService extends Service {
private IBinder mBinder = new IMyAidlInterface.Stub() {
@Override
public int add(int num1, int num2) throws RemoteException {
return num1 + num2;
}
};
@Override
public IBinder onBind(Intent intent) {
return mBinder;
}
}
然后在服务端创建一个aidl
interface IMyAidlInterface {
int add(int num1,int num2);
}
之后把服务端创建的aidl复制,粘贴到客户端里面。
写完就可以运行了。
相关文章推荐
- java两个进程之间通信
- 直接读取进程内存实现两个应用程序之间的通信
- 通过共享内存,利用循环队列实现两个进程A,B之间的通信
- Android AIDL 实现两个APP之间的跨进程通信实例
- Delphi 两个应用程序(进程)之间的通信
- 使用管道实现两个进程之间的通信
- Delphi 两个应用程序(进程)之间的通信
- Linux进程间通信-----使用数据报套接字实现两个进程之间的通信
- Linux下的有名管道(05)---使用两个管道实现两个进程之间的通信(对讲机模式)
- 两个进程之间的通信方式有哪几种?
- LINUX 实现两个进程之间的通信
- VC运用命名管道实现两个进程之间通信的流程
- 通过共享内存,实现两个进程A,B之间的通信
- SpringCloud : 两个微服务进程之间通信(远程调用)
- 实现了本机两个进程之间的通信 c#.net socket
- Linux下的有名管道(06)---使用两个管道实现两个进程之间的通信(手机模式)
- Delphi 两个应用程序(进程)之间的通信
- 两个进程之间通信
- 进程之间的8种通信方式
- 进程,线程之间的通信方式