BottomSheetBehavior底部弹出的用法
2017-11-15 15:38
417 查看
需要的依赖:
compile ‘com.android.support:appcompat-v7:23.2.1’
compile ‘com.android.support:design:23.2.1’
效果图如下:
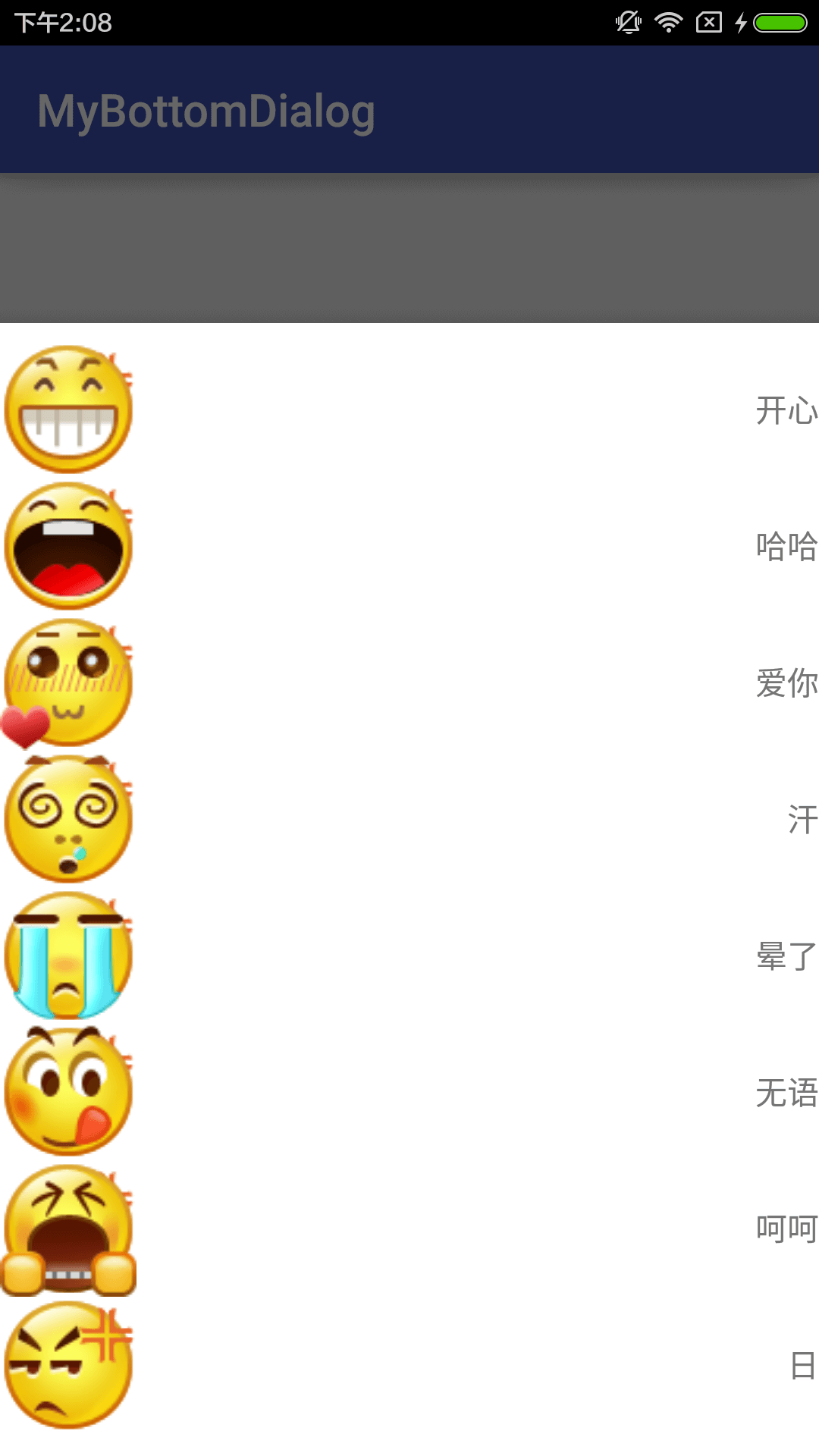
一直出现的问题是弹出窗口只显示部分,很烦人,浪费半个下午终于解决了
下面看代码:
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
activity_main.xml布局文件
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
相关Adpter
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
styles.xml格式文件,这个很有用
2
3
4
5
6
7
8
9
10
11
12
13
14
15
item.xml文件
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
哈哈哈,搞定了,懒得废话!代码是学coding的最好老师!
以上代码全部直接复制粘贴就可以使用,下面再给出我写的一个Demo,包含头部和底部的,加深使用的理解:
http://download.csdn.net/download/wanxuedong/10119837
compile ‘com.android.support:appcompat-v7:23.2.1’
compile ‘com.android.support:design:23.2.1’
效果图如下:
一直出现的问题是弹出窗口只显示部分,很烦人,浪费半个下午终于解决了
下面看代码:
package com.example.mybottomdialog; import android.os.Bundle; import android.support.annotation.NonNull; import android.support.design.widget.BottomSheetBehavior; import android.support.v7.app.AppCompatActivity; import android.support.v7.widget.LinearLayoutManager; import android.support.v7.widget.RecyclerView; import android.view.View; import android.widget.Button; import com.example.mybottomdialog.adapter.Item; import com.example.mybottomdialog.adapter.ItemAdapter; import java.util.ArrayList; import java.util.List; public class MainActivity extends AppCompatActivity { private Button btn_window; View mBottomSheet; BottomSheetBehavior mBehavior; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); btn_window = (Button) findViewById(R.id.btn_window); mBottomSheet = findViewById(R.id.bottomSheet); RecyclerView recyclerView = (RecyclerView) mBottomSheet.findViewById(R.id.recyclerview); mBehavior = BottomSheetBehavior.from(mBottomSheet); mBehavior.setBottomSheetCallback(new BottomSheetBehavior.BottomSheetCallback() { @Override public void onStateChanged(@NonNull View bottomSheet, int newState) { } @Override public void onSlide(@NonNull View bottomSheet, float slideOffset) { } }); recyclerView.setLayoutManager(new LinearLayoutManager(this)); recyclerView.setHasFixedSize(true); recyclerView.setAdapter(new ItemAdapter(createItems())); btn_window.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if (mBehavior.getState() == BottomSheetBehavior.STATE_COLLAPSED) { mBehavior.setState(BottomSheetBehavior.STATE_EXPANDED); } else { mBehavior.setState(BottomSheetBehavior.STATE_COLLAPSED); } } }); } public List<Item> createItems() { ArrayList<Item> items = new ArrayList<>(); items.add(new Item(R.drawable.f001, "开心")); items.add(new Item(R.drawable.f002, "哈哈")); items.add(new Item(R.drawable.f003, "爱你")); items.add(new Item(R.drawable.f004, "汗")); items.add(new Item(R.drawable.f005, "晕了")); items.add(new Item(R.drawable.f006, "无语")); items.add(new Item(R.drawable.f007, "呵呵")); items.add(new Item(R.drawable.f008, "日")); return items; } /* private void showBottomSheetDialog() { BottomSheetDialog bottomSheetDialog = new BottomSheetDialog(MainActivity.this); View view = getLayoutInflater().inflate(R.layout.sheet, null); RecyclerView recyclerView = (RecyclerView) view.findViewById(R.id.recyclerView); recyclerView.setAdapter(new ItemAdapter(createItems())); recyclerView.setHasFixedSize(true); recyclerView.setLayoutManager(new LinearLayoutManager(getParent())); bottomSheetDialog.setContentView(view); bottomSheetDialog.show(); }*/ }1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
activity_main.xml布局文件
<?xml version="1.0" encoding="utf-8"?> <android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:fitsSystemWindows="true" tools:context=".MainActivity"> <LinearLayout android:layout_width="match_parent" android:layout_height="200dp" android:gravity="center" android:orientation="vertical"> <Button android:id="@+id/btn_window" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="显示底部弹出窗口" /> </LinearLayout> <LinearLayout android:id="@+id/bottomSheet" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_gravity="bottom" android:background="@android:color/white" android:elevation="4dp" android:orientation="vertical" app:behavior_hideable="true" app:behavior_peekHeight="0dp" app:layout_behavior="@string/bottom_sheet_behavior" tools:ignore="UnusedAttribute"> <android.support.v7.widget.RecyclerView android:id="@+id/recyclerview" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#fff" /> </LinearLayout> </android.support.design.widget.CoordinatorLayout>1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
相关Adpter
package com.example.mybottomdialog.adapter; import android.support.annotation.DrawableRes; public class Item { private int mDrawableRes; private String mTitle; public Item(@DrawableRes int drawable, String title) { mDrawableRes = drawable; mTitle = title; } public int getDrawableResource() { return mDrawableRes; } public String getTitle() { return mTitle; } }1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
package com.example.mybottomdialog.adapter; import android.support.v7.widget.RecyclerView; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.ImageView; import android.widget.TextView; import com.example.mybottomdialog.R; import java.util.List; public class ItemAdapter extends RecyclerView.Adapter<ItemAdapter.ViewHolder> { private List<Item> mItems; public ItemAdapter(List<Item> items) { mItems = items; } @Override public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) { return new ViewHolder(LayoutInflater.from(parent.getContext()) .inflate(R.layout.item, parent, false)); } @Override public void onBindViewHolder(ViewHolder holder, int position) { holder.setData(mItems.get(position)); } @Override public int getItemCount() { return mItems.size(); } public class ViewHolder extends RecyclerView.ViewHolder { public ImageView imageView; public TextView textView; public Item item; public ViewHolder(View itemView) { super(itemView); imageView = (ImageView) itemView.findViewById(R.id.imageView); textView = (TextView) itemView.findViewById(R.id.textView); } public void setData(Item item) { this.item = item; imageView.setImageResource(item.getDrawableResource()); textView.setText(item.getTitle()); } } }1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
styles.xml格式文件,这个很有用
<resources> <!-- Base application theme. --> <style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar"> <!-- Customize your theme here. --> <item name="colorPrimary">@color/colorPrimary</item> <item name="colorPrimaryDark">@color/colorPrimaryDark</item> <item name="colorAccent">@color/colorAccent</item> </style> <style name="AppTheme.AppBarOverlay" parent="ThemeOverlay.AppCompat.Dark.ActionBar" /> <style name="AppTheme.PopupOverlay" parent="ThemeOverlay.AppCompat.Light" /> </resources>1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
item.xml文件
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:clickable="true"> <android.support.v7.widget.AppCompatImageView android:id="@+id/imageView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:background="@drawable/f008" /> <TextView android:id="@+id/textView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentRight="true" android:layout_centerVertical="true" android:text="xx" android:textSize="20dp" /> </RelativeLayout>1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
哈哈哈,搞定了,懒得废话!代码是学coding的最好老师!
以上代码全部直接复制粘贴就可以使用,下面再给出我写的一个Demo,包含头部和底部的,加深使用的理解:
http://download.csdn.net/download/wanxuedong/10119837
相关文章推荐
- BottomSheetBehavior底部弹出窗口的用法
- Android使用BottomSheetBehavior 和 BottomSheetDialog实现底部弹窗
- BottomSheetBehavior、BottomSheetDialog和BottomSheetDialogFragment的用法
- 使用 DialogFragment 和 BottomSheet 实现底部弹出框
- 底部弹窗:BottomSheetBehavior使用
- BottomSheetDialog底部弹出框、butterkoife使用
- Android使用CoordinatorLayout和BottomSheetBehavior实现滑动效果(底部抽屉)
- AndroidSweetSheet:从底部弹出面板(1)
- BottomDialog 是一个通过 DialogFragment 实现的底部弹窗布局,并且支持弹出动画,支持任意布局http://shaohui.me
- IOS UIActionSheet(底部 弹出框的使用)
- Android Bottom Sheet详解之BottomSheetBehavior与BottomSheetDialog
- Android BottomSheetDialog实现底部对话框的示例
- Android的Design库---BottomSheetBehavior和BottomSheetDialog
- BottomSheetDialog——仿知乎分享弹出框
- BottomSheetBehavior 之 java.lang.IllegalArgumentException: The view is not associated with BottomSheetBehavior
- PopupWindowFromBottom 从底部弹出popupwindow
- 开源项目:底部动作条(BottomSheet)
- Android 日常封装之暴力CustomActionSheet自定义Fragment从底部弹出界面
- BottomSheetDialog获得BottomSheetBehavior的方法
- Android Support 23.2 BottomSheetBehavior的使用