N-Queens--LeetCode
2017-10-29 13:54
555 查看
1.题目
N-QueensThe n-queens puzzle is the problem of placing n queens on an n×n chessboard such that no two queens attack each other.
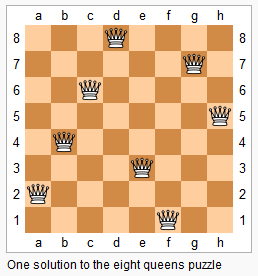
Given an integer n, return all distinct solutions to the n-queens puzzle.
Each solution contains a distinct board configuration of the n-queens’ placement, where ‘Q’ and ‘.’ both indicate a queen and an empty space respectively.
For example,
There exist two distinct solutions to the 4-queens puzzle:
[
[“.Q..”, // Solution 1
“…Q”,
“Q…”,
“..Q.”],
[“..Q.”, // Solution 2
“Q…”,
“…Q”,
“.Q..”]
]
2.题意
在一个N*N的棋盘上放置N个皇后,每行一个并使其不能互相攻击(同一行、同一列、同一斜线上的皇后都会自动攻击)3.分析
经典的N皇后问题经典解法为回溯递归,一层层向下扫描
使用pos数组,pos[i]表示第i行皇后所在的列,初始值为-1
从0开始递归,每一行都遍历一次各列,判断如果在该位置放置皇后会不会有冲突
当最后一行皇后放好后,得到一种可能的结果,存入res
4.代码
class Solution { public: vector<vector<string>> solveNQueens(int n) { vector<vector<string>> res; vector<int> pos(n, -1); solveNQueensDFS(pos, 0, res); return res; } private: void solveNQueensDFS(vector<int> &pos, int row, vector<vector<string>> &res) { int n = pos.size(); if(row == n) { vector<string> out(n, string(n, '.')); for(int i = 0; i < n; ++i) out[i][pos[i]] = 'Q'; res.push_back(out); } else { for(int col = 0; col < n; ++col) { if(isValid(pos, row, col)) { pos[row] = col; solveNQueensDFS(pos, row + 1, res); pos[row] = -1; } } } } bool isValid(vector<int> &pos, int row, int col) { for(int i = 0; i < row; ++i) { if(pos[i] == col || abs(row - i) == abs(col - pos[i])) return false; } return true; } };
相关文章推荐
- LeetCode-N-Queens-N皇后-回溯
- LeetCode 51. N-Queens
- leetcode 050 —— N-Queens
- LeetCode - N-Queens I && II
- LeetCode刷题笔录N-Queens
- [leetcode]N-Queens II @ Python
- leetcode 51. N-Queens
- 【leetcode】N-Queens
- leetcode - N-Queens
- 【leetcode】N-queens
- LeetCode N-Queens
- leetcode-51. N-Queens
- LeetCode 51.N-Queens
- leetcode - N-Queens
- [leetcode] N-Queens
- 2017.11.8 LeetCode N皇后问题 - 51. N-Queens - 52....
- LeetCode--51. N-Queens
- LeetCode 51. N-Queens
- LeetCode: N-Queens
- Leetcode_n-queens-ii