java基础---->java注解的使用(一)
2017-10-17 11:48
441 查看
注解是众多引入到Java SE5中的重要的语言变化之一。它为我们在代码中添加信息提供了一种形式化的方法,使我们可以在稍后某个时刻非常方便的使用这些数据。今天我们就开始学习一下java中注解的知识。
标准注解:@Override,@Deprecated,@Suppresswarnings。
元注解:@Target,@Retention,@Documented,@Inherited。
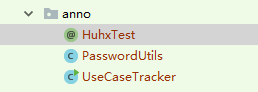
运行的结果如下:
java注解的介绍
java内置了三种标准注解,以及四种元注解。元注解专职负责注解其他的注解。标准注解:@Override,@Deprecated,@Suppresswarnings。
元注解:@Target,@Retention,@Documented,@Inherited。
java注解的使用案例
测试的项目结构如下: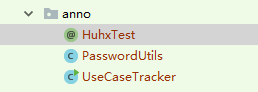
一、定义注解:
package com.linux.huhx.anno; import java.lang.annotation.ElementType; import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; import java.lang.annotation.Target; /** * @Author huhx * @Date: 2017/9/17 20:07 * 定义注解: */ @Target(ElementType.METHOD) @Retention(RetentionPolicy.RUNTIME) public @interface HuhxTest { public int id(); public String description() default "no description"; }
二、编写要被注解的测试类
package com.linux.huhx.anno; import java.util.List; /** * @Author huhx * @Date: 2017/9/17 20:13 */ public class PasswordUtils { @HuhxTest(id = 47, description = "password must contain at least one numeric") public boolean validatePassword(String password) { return password.matches("\\w*\\d\\w*"); } @HuhxTest(id = 48) public String encryptPassword(String password) { return new StringBuilder(password).reverse().toString(); } @HuhxTest(id = 49, description = "new passrod can't equal previously used ones") public boolean checkForNewPassword(List<String> prevPasswords, String password) { return !prevPasswords.contains(password); } }
三、编写注解处理器
package com.linux.huhx.anno; import java.lang.reflect.Method; import java.util.ArrayList; import java.util.Collections; import java.util.List; /** * @Author huhx * @Date: 2017/9/17 20:12 */ public class UseCaseTracker { public static void trackUseCases(List<Integer> useCases, Class<?> cl) { for (Method m : cl.getDeclaredMethods()) { HuhxTest test = m.getAnnotation(HuhxTest.class); if (test != null) { System.out.println("found use case: " + test.id() + ", " + test.description()); useCases.remove(new Integer(test.id())); } } for (int i : useCases) { System.out.println("warning: missing use case " + i); } } public static void main(String[] args) { List<Integer> useCases = new ArrayList<>(); Collections.addAll(useCases, 47, 48, 49, 50); trackUseCases(useCases, PasswordUtils.class); } }
运行的结果如下:
found use case: 49, new passrod can't equal previously used ones found use case: 48, no description found use case: 47, password must contain at least one numeric warning: missing use case 50
友情链接
相关文章推荐
- Java基础--->09.关于JDK使用旧方法编译出错、警告问题。
- Java基础-学习使用Annotation注解对象
- Java基础笔记 – Annotation注解的介绍和使用 自定义注解
- Java基础之集合框架——使用真的的链表LinkedList<>(TryPolyLine)
- java基础---->Zip压缩的使用(转)
- Java基础加强<二>_内省、注解、泛型
- Java基础笔记 – Annotation注解的介绍和使用 自定义注解
- java基础---->Comparable和Comparator的使用
- java基础---->Base64算法的使用
- java基础---->Base64算法的使用
- Java基础-学习使用Annotation注解对象
- Java基础笔记 – Annotation注解的介绍和使用 自定义注解
- Java基础之集合框架——使用堆栈Stack<>对象模拟发牌(TryDeal)
- Java基础—注解的使用
- <黑马程序员>java基础加强--eclipse的使用
- Java笔记7 Java基础加强<3>注解
- JAVA基础学习之IP简述使用、反射、正则表达式操作、网络爬虫、可变参数、了解和入门注解的应用、使用Eclipse的Debug功能(7)