自定义View 购物车加减数量
2017-10-14 00:00
405 查看
首先我们需要的是 加(botton) 减 (botton) 输入数量(editText) 【这里我还设置了最大值】
然后
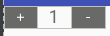
对 加减按钮设置监听,点击对editText的内容进行修改。
布局代码:
再写我们需要在调用这个自定义View的时候设置它的属性【res -> values -> attrs.xml】写入
最后我们写自定义的LinearLayout,代码如下:
最后在MainActivity布局中调用:
然后
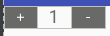
对 加减按钮设置监听,点击对editText的内容进行修改。
布局代码:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="horizontal" android:focusable="true" android:showDividers="middle"> //android:showDividers="middle"在每个子View间加入分隔线 <TextView android:id="@+id/add" android:layout_width="0dp" android:layout_weight="1" android:layout_height="match_parent" android:background="@color/gray" android:gravity="center" android:minWidth="30dp" android:text="+" android:textColor="@color/write" /> <EditText android:id="@+id/count" android:layout_width="0dp" android:layout_weight="1" android:layout_height="match_parent" android:background="@null" android:gravity="center" android:inputType="number" android:minWidth="40dp" android:text="1" android:textColor="@color/gray" /> <TextView android:id="@+id/delete" android:layout_width="0dp" android:layout_weight="1" android:layout_height="match_parent" android:background="@color/gray" android:gravity="center" android:minWidth="30dp" android:text="-" android:textColor="@color/write" /> </LinearLayout>
再写我们需要在调用这个自定义View的时候设置它的属性【res -> values -> attrs.xml】写入
<?xml version="1.0" encoding="utf-8"?> <resources> <declare-styleable name="AddDeleteCount"> <attr name="maxCount" format="integer"/> <attr name="btnWidth" format="dimension"/> <attr name="editWidth" format="dimension"/> <attr name="AddDeleteBackgroungColor" format="color"/> <attr name="isEditCount" format="boolean"/> <attr name="textSize" format="dimension"/> </declare-styleable> </resources>
最后我们写自定义的LinearLayout,代码如下:
import android.content.Context; import android.content.res.TypedArray; import android.graphics.Color; import android.support.annotation.Nullable; import android.text.Editable; import android.text.TextWatcher; import android.util.AttributeSet; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.EditText; import android.widget.LinearLayout; import android.widget.TextView; import cn.jhc.startdemo.R; /** * Created by Administrator on 2017/10/14. */ public class AddDeleteCount extends LinearLayout implements View.OnClickListener, TextWatcher { private int max, btnWidth, edtWidth, addOrDeleteBg; private EditText edt_count; //edit控件是否可以修改 private boolean IsEdtCountEdited = false; public AddDeleteCount(Context context) { this(context, null); } public AddDeleteCount(Context context, @Nullable AttributeSet attrs) { super(context, attrs); LayoutInflater.from(context).inflate(R.layout.add_or_delete, this); TextView add = findViewById(R.id.add); add.setOnClickListener(this); TextView delete = findViewById(R.id.delete); delete.setOnClickListener(this); edt_count = findViewById(R.id.count); edt_count.addTextChangedListener(this); if (attrs != null) { TypedArray array = context.obtainStyledAttributes(attrs, R.styleable.AddDeleteCount); max = array.getInt(R.styleable.AddDeleteCount_maxCount, -1); btnWidth = array.getDimensionPixelOffset(R.styleable.AddDeleteCount_btnWidth, 100); edtWidth = array.getDimensionPixelOffset(R.styleable.AddDeleteCount_editWidth, 80); addOrDeleteBg = array.getColor(R.styleable.AddDeleteCount_AddDeleteBackgroungColor, Color.GRAY); IsEdtCountEdited = array.getBoolean(R.styleable.AddDeleteCount_isEditCount, false); array.recycle(); } edt_count.setEnabled(IsEdtCountEdited); LayoutParams params = new LayoutParams(btnWidth, ViewGroup.LayoutParams.MATCH_PARENT); add.setLayoutParams(params); delete.setLayoutParams(params); LayoutParams params1 = new LayoutParams(edtWidth, ViewGroup.LayoutParams.MATCH_PARENT); edt_count.setLayoutParams(params1); add.setBackgroundColor(addOrDeleteBg); delete.setBackgroundColor(addOrDeleteBg); } @Override public void onClick(View view) { int currentCount = Integer.parseInt(edt_count.getText().toString()); switch (view.getId()) { case R.id.add: if (currentCount < max) { edt_count.setText(String.valueOf(currentCount + 1)); } break; case R.id.delete: if (currentCount > 1) { edt_count.setText(String.valueOf(currentCount - 1)); } break; } } @Override public void beforeTextChanged(CharSequence charSequence, int i, int i1, int i2) { } @Override public void onTextChanged(CharSequence charSequence, int i, int i1, int i2) { } @Override public void afterTextChanged(Editable editable) { if (edt_count.getText().toString().isEmpty()) { return; } else { if (Integer.parseInt(edt_count.getText().toString()) > max) { edt_count.setText(String.valueOf(max)); } } } public void setEdtCountEdited(boolean edtCountEdited) { edt_count.setEnabled(edtCountEdited); } public int getCount() { if (!edt_count.getText().toString().isEmpty()) { return Integer.parseInt(edt_count.getText().toString()); } return 1; } }
最后在MainActivity布局中调用:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="cn.jhc.startdemo.duty.DutyActivity" android:orientation="vertical"> <cn.jhc.startdemo.common.view.AddDeleteCount android:id="@+id/add_delete" android:layout_width="300dp" android:layout_height="40dp" app:maxCount="5" app:btnWidth="100dp" app:editWidth="100dp" app:isEditCount="false" app:AddDeleteBackgroungColor="@color/gray" app:textSize="14dp"/> </LinearLayout>
相关文章推荐
- 自定义view继承LinearLayout实现购物车数量的加减
- 仿京东购物车加减数量的自定义View
- Android——自定义View之购物车的加减
- Android——自定义View之购物车的加减
- 自定义view之路--数量加减view--适用电商类
- 自定义购物车加减view
- 自定义View实现东购物车加减控件
- 购物车数量加减器(自定义之初窥门径)
- Android自定义控件之购物车数量加减器
- Android使用自定义View实现购物车的加减
- 购物车加减器--自定义view
- 购物车商品数量增减输入自定义View
- Android——自定义View之购物车的加减
- 购物车自定义加减控件
- jQuery实现购物车物品数量的加减
- Android中自定义ViewGroup使每行组件数量不确定,并拿到选中数据
- JQuery购物车多物品数量的加减+总价计算
- 简单购物车数量加减
- jQuery实现购物车物品数量的加减
- 实现购物车多物品数量的加减+总价计算