使用java和Velocity创建html复杂的table
2017-10-14 00:00
381 查看
摘要: 使用Velocity创建html复杂的table
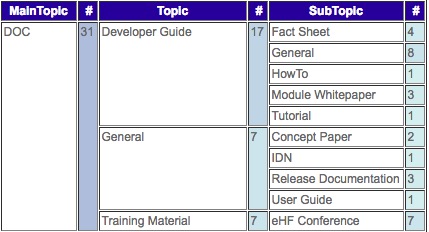
2、在标签
3、就像在(1)里面介绍的,为了细粒度的控制,html抽象出了表格,行,列的标签。基于java,我们也可以抽象出类Table表示表格,抽象出类Cell表示单元格。在类Table中用一个二维数据容纳类Cell,这样就可以完整的表示一个表格了。
4、类Cell中有四个基本的字段
message字段表示要显示的文本.
rowspan字段表示
colspan字段表示
visiable字段表示是否显示
4、类Table中有三个基本的字段
在创建Table类的时候要传行数rows、列数cols,用来初始化表格。
这个addCell方法是类Table的核心方法,处理添加的Cell。row和col参数(都从1开始),指定了Cell的起始位置。处理的逻辑:从Cell的起始位置开始,按照rowspan和colspan的值,分别向右和向下的Cell的visiable属性设置为false(不显示),那么在velocity渲染的时候就不会显示这些单元格。添加的Cell就能占据这些不会显示的单元格的位置。
2、Cell类
3、velocity
4、测试类
5、简单的效果图
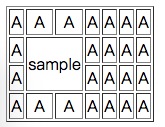
http://www.easyjf.com/blog/html/20080109/1015823.html
https://www.javaworld.com/article/2077810/open-source-tools/complex-html-tables-made-easy-with-apache-velocity.html
一、问题介绍
在工作中遇到过这样的的需求,要用java生成一个如下样式的html表格,这个样例只是一个简单的举例子,还可能更复杂。最后选择velocity模版引擎实现,表格最后是什么样子,只有获得数据之后才能确定,所以在代码中动态创建表格。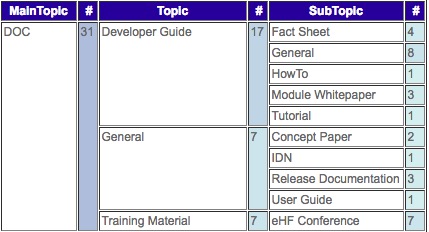
二、原理介绍
1、在html中,表格的标签是<table>,行的标签是
<tr>,列的标签是
<td>。从这些标签,可以看出,表格->行->列->单元格,是对表格的细粒度的控制。
2、在标签
<td>中,有rowspan和colspan两个属性,分别控制着单元格向右和向下占据几个单元格,对于默认值、0和1,效果都是一样的,都只是占据自己的单元格,不会占据其他的单元格。复杂表格的创建,主要就是细粒度的控制这个2属性。
3、就像在(1)里面介绍的,为了细粒度的控制,html抽象出了表格,行,列的标签。基于java,我们也可以抽象出类Table表示表格,抽象出类Cell表示单元格。在类Table中用一个二维数据容纳类Cell,这样就可以完整的表示一个表格了。
4、类Cell中有四个基本的字段
private String message = ""; private int rowspan = 0; private int colspan = 0; private boolean visiable = true; public Cell(String message, int rowspan, int colspan, boolean visiable) { this.message = message; this.rowspan = rowspan; this.colspan = colspan; this.visiable = visiable; }
message字段表示要显示的文本.
rowspan字段表示
<td>标签中rowspan属性.
colspan字段表示
<td>标签中colspan属性.
visiable字段表示是否显示
<td>标签
4、类Table中有三个基本的字段
// 创建表格的行数 private int rows = 0; // 创建表格的列数 private int cols = 0; // 二维数组 private Cell[][] cells = null; public Table(int rows, int cols) { this.rows = rows; this.cols = cols; this.cells = new Cell[rows][cols]; init(rows, cols); } private void init(int rows, int cols) { for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { cells[i][j] = new Cell("A", 1, 1, true); } } }
在创建Table类的时候要传行数rows、列数cols,用来初始化表格。
public void addCell(Cell cell, int row, int col) { int rowIndex = row - 1; int colIndex = col - 1; int rowSize = rowIndex + cell.getRowspan(); int colSize = colIndex + cell.getColspan(); for (int i = rowIndex; i < rowSize; i++) { for (int j = colIndex; j < colSize; j++) { cells[i][j].setVisiable(false); cells[i][j].setMessage("noVisiable"); } } cells[rowIndex][colIndex] = cell; }
这个addCell方法是类Table的核心方法,处理添加的Cell。row和col参数(都从1开始),指定了Cell的起始位置。处理的逻辑:从Cell的起始位置开始,按照rowspan和colspan的值,分别向右和向下的Cell的visiable属性设置为false(不显示),那么在velocity渲染的时候就不会显示这些单元格。添加的Cell就能占据这些不会显示的单元格的位置。
三、完整代码
1、Table类public class Table {
// 创建表格的行数 private int rows = 0; // 创建表格的列数 private int cols = 0; // 二维数组 private Cell[][] cells = null; public Table(int rows, int cols) { this.rows = rows; this.cols = cols; this.cells = new Cell[rows][cols]; init(rows, cols); } private void init(int rows, int cols) { for (int i = 0; i < rows; i++) { for (int j = 0; j < cols; j++) { cells[i][j] = new Cell("A", 1, 1, true); } } }
public void addCell(Cell cell, int row, int col) { int rowIndex = row - 1; int colIndex = col - 1; int rowSize = rowIndex + cell.getRowspan(); int colSize = colIndex + cell.getColspan(); for (int i = rowIndex; i < rowSize; i++) { for (int j = colIndex; j < colSize; j++) { cells[i][j].setVisiable(false); cells[i][j].setMessage("noVisiable"); } } cells[rowIndex][colIndex] = cell; }
public int getRows() {
return rows;
}
public void setRows(int rows) {
this.rows = rows;
}
public int getCols() {
return cols;
}
public void setCols(int cols) {
this.cols = cols;
}
public Cell getCell(int row, int col) {
return cells[row - 1][col - 1];
}
public void setCells(Cell[][] cells) {
this.cells = cells;
}
}
2、Cell类
public class Cell { private String message = ""; private int rowspan = 0; private int colspan = 0; private boolean visiable = true; public Cell(String message, int rowspan, int colspan, boolean visiable) { this.message = message; this.rowspan = rowspan; this.colspan = colspan; this.visiable = visiable; } public String getMessage() { return message; } public void setMessage(String message) { this.message = message; } public int getRowspan() { return rowspan; } public void setRowspan(int rowspan) { this.rowspan = rowspan; } public int getColspan() { return colspan; } public void setColspan(int colspan) { this.colspan = colspan; } public boolean isVisiable() { return visiable; } public void setVisiable(boolean visiable) { this.visiable = visiable; } }
3、velocity
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>table</title> </head> <body> <table border="1"> #foreach($row in [1..$table.rows]) <tr> #foreach($col in [1..$table.cols]) #set ($cell = $table.getCell($row, $col)) #if($cell.visiable) <td rowspan="$cell.rowspan" colspan="$cell.colspan" align="center">$cell.message</td> #end #end </tr> #end </table> </body> </html>
4、测试类
public static void main(String[] args) { Table table = new Table(4, 7); table.addCell(new Cell("sample", 2, 2, true), 2, 2); VelocityEngine ve = new VelocityEngine(); ve.setProperty(RuntimeConstants.RESOURCE_LOADER, "classpath"); ve.setProperty("classpath.resource.loader.class", ClasspathResourceLoader.class.getName()); ve.init(); Template t = ve.getTemplate("example.vm"); VelocityContext ctx = new VelocityContext(); ctx.put("table", table); StringWriter sw = new StringWriter(); t.merge(ctx, sw); System.out.println(sw.toString()); }
5、简单的效果图
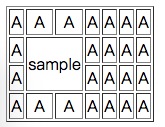
四、后记
这里只是简单的介绍了一种实现复杂表格的思路,举例子中用的java和velocity,其实不限于这2者,单元格的属性也可以扩展。还可以看其他的文章:http://www.easyjf.com/blog/html/20080109/1015823.html
https://www.javaworld.com/article/2077810/open-source-tools/complex-html-tables-made-easy-with-apache-velocity.html
相关文章推荐
- 创建智能网络蜘蛛——如何使用Java网络对象和HTML对象(翻译)
- JavaMaill:使用MIME协议创建内含图片的复杂邮件
- html的table使用div创建
- 使用Java将PDF解析成HTML页面进行展示并从页面中提取Json数据设置到Table中
- 如何使用Java中HttpClient解析Html中的table
- spring boot 使用velocity、freeMarker模板创建html页面返回给前端
- Java中JTbale使用1—创建一个table
- JavaMaill:使用MIME协议创建内含图片的复杂邮件
- Java使用Velocity模板发送HTML格式邮件并解决中文乱码问题
- spring boot 使用velocity、freeMarker模板创建html页面返回给前端
- jsp使用自定义标签taglib分页系列——TableTag.java
- jsp使用自定义标签taglib分页系列——TableBase.java
- 使用 on prebuilt table 创建物化视图
- HTML中使用JavaScript创建TR和TD
- 使用iframe和table模拟frameset的resize功能.html
- win32下使用gcc编译供java 调用的dll须知(jni) http://www.blogjava.net/lixf/archive/2005/12/23/25177.html
- HTML中div标签与table标签组合使用问题
- 计算Java日期--学习怎样创建和使用日期(1)
- 计算Java日期--学习怎样创建和使用日期
- 在table中插入多行,能使用与insertAdjacentHTML相似的功能吗?