C#工大租车系统(包含异常处理)
2017-10-11 22:12
218 查看
一、 实验目的
1、熟悉Visual Studio.Net集成开发环境。
2、掌握c#的基本语法。
3、理解并掌握面向对象三要素:封装、继承、多态。
4、培养动手能力与解决实际问题的能力。
二、 实验内容及要求
根据所学面向对象知识,编写一个控制面板的“工大汽车租赁系统”,实现以下功能:
展示所有可租车辆
选择车型、租车量
展示租车清单,包括:总金额、总载货量以及车型、总载人量及其车型。
用户输入错误时,加入异常处理:当询问用户是否租车,用户输入是字母时,用try - catch 语句处理异常,并提示重新输入;当用户输入的车辆序号不存在时,抛出“车辆
不存在”异常,并提示重新输入
程序源代码:
运行结果分析:
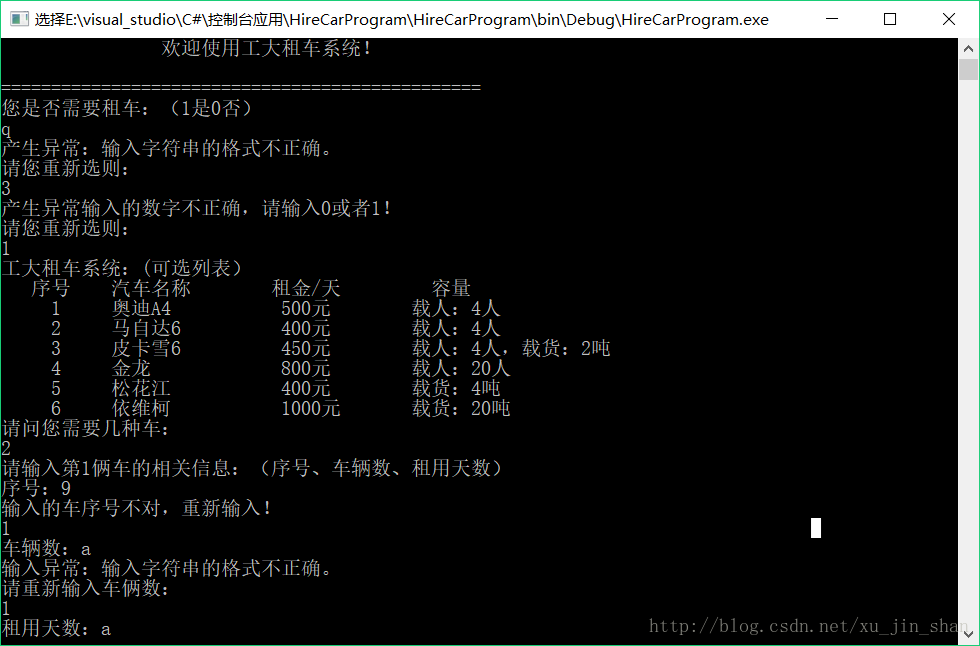
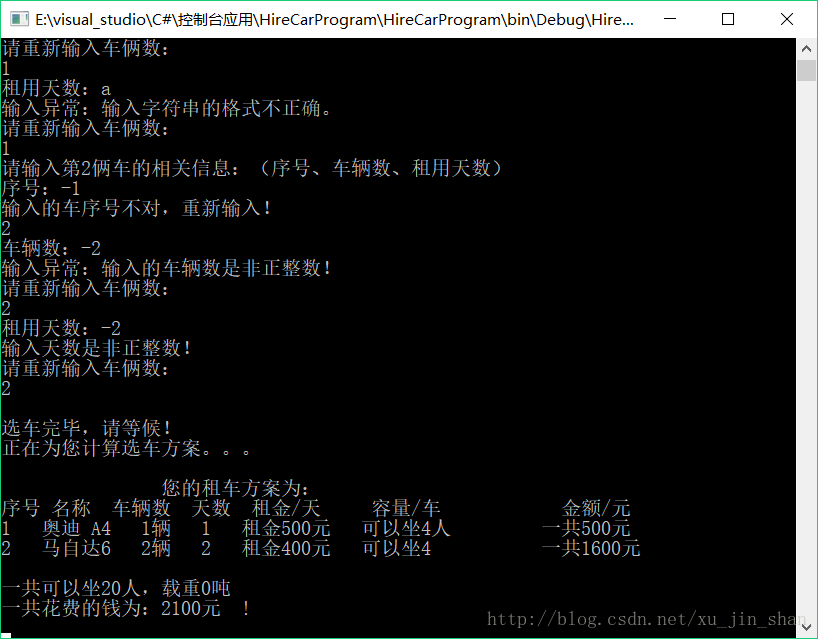
分析:
1. 在选车的时候,输入如果是字母则抛出异常,异常信息为:产生异常:输入的字符串的格式不正确。如果输入的是非0或非1 的数字,则抛出异常,异常信息为:产生异常输入的数字不正确,请输入0或者1!。如果输入是0,则推出系统,提示信息为:欢迎光顾工大租车系统!欢迎下次再来!。如果是输入1,则进入工大租车系统,显示可以租用的车辆。该位置使用了循环,如果发生异常,则可以一直输入,直到正确为止。
2. 选则车辆的种类,输入数字,表示的是选用的车辆的种类;
3. 提醒输入第一俩车的信息,后面的车辆提示信息以此类推,如果输入的序号不是1~6,则抛出异常,异常信息为:输入的车序号不对;
4. 提示输入车辆数,如果输入的是字母,则引发异常,异常信息为:输入异常:输入字符串的格式不正确。如果输入的是非正数,则引发异常,异常信息为:输入的车辆数是非正数!提示重新输入,该异常的信息只能输错一次,因为是该处没有使用循环,故不能连续输入错误信息。
5. 提示输入天数,异常信息类似于车俩数。
6. 当输入完成时,提供租车方案,格式如图,同时计算总载货量和总载人量,给予输出。
运行结构图:
1、熟悉Visual Studio.Net集成开发环境。
2、掌握c#的基本语法。
3、理解并掌握面向对象三要素:封装、继承、多态。
4、培养动手能力与解决实际问题的能力。
二、 实验内容及要求
根据所学面向对象知识,编写一个控制面板的“工大汽车租赁系统”,实现以下功能:
展示所有可租车辆
选择车型、租车量
展示租车清单,包括:总金额、总载货量以及车型、总载人量及其车型。
用户输入错误时,加入异常处理:当询问用户是否租车,用户输入是字母时,用try - catch 语句处理异常,并提示重新输入;当用户输入的车辆序号不存在时,抛出“车辆
不存在”异常,并提示重新输入
程序源代码:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace HireCarProgram { class Car //车主类,作为基类 { private string name; //车名字 public string Name //属性设置 { set { name = value; } get { return name; } } private int xuhao; //车序号 public int Xuhao { set { xuhao = value; } get { return xuhao; } } private double price; //车每辆每天的价格 public double Price { set { price = value; } get { return price; } } private int days; //租车天数,默认为0 public int Days { set { days = value; } get { return days; } } private int number; //租车数量,默认为0 public int Number { set { number = value; } get { return number; } } public Car() { this.days = 0; this.number = 0; } public Car(int days,int number) { this.days = days; this.number = number; } public double zj() //计算一种车的总价格 { return (this.price * this.number * this.days); } } class GoodsCar : Car //载货车,继承Car { private int weight; //每辆车每次载重 public int Weight { set { weight = value; } get { return weight; } } public GoodsCar():base() { } public GoodsCar(int days,int number):base(days,number) { } public int zz() //计算一种车总载重 { return (this.Number * this.weight * this.Days); } } class PeopleCar : Car //客车,继承Car { private int people; //每辆车每次载人数 public int People { set { people = value; } get { return people; } } public PeopleCar():base() { } public PeopleCar(int days,int number):base(days,number) { } public int zr() //计算一种车的载人 { return (this.Number * this.people * this.Days); } } class GoodsAndPeopleCar : Car //皮卡雪客车货车 { private int weight; public int Weight { get { return weight; } set { weight = value; } } 4000 private int people; public int People { set { people = value; } get { return people; } } public GoodsAndPeopleCar():base() { this.Xuhao = 3; this.Name = "皮 卡雪"; this.Price = 450; this.weight = 2; this.people = 4; } public GoodsAndPeopleCar(int days, int number):base(days,number) //构造参数为选车天数和选车数 { this.Xuhao = 3; this.Name = "皮 卡雪"; this.Price = 450; this.weight = 2; this.people = 4; } public void toString() //输出信息 { Console.WriteLine("{0} {1} {2}辆 {3} 租金{4}元 {5}坐,{6}吨 一共{7}元", this.Xuhao, this.Name, this.Number, this.Days, this.Price, this.People, this.Weight, (this.Days * this.Price * this.Number)); } public int zz() //计算总重量 { return (this.Number * this.Weight * this.Days); } public int zr() //计算总人数 { return (this.Number * this.People * this.Days); } } class SongHuaJiangGoodsCar : GoodsCar //货车松花江 { public SongHuaJiangGoodsCar():base()//无参构造,默认天数和车辆数为0 { this.Xuhao = 5; this.Name = "松 花江"; this.Price = 400; this.Weight = 4; } public SongHuaJiangGoodsCar(int days, int number):base(days,number) { this.Xuhao = 5; this.Name = "松 花江"; this.Price = 400; this.Weight = 4; } public void toString() { Console.WriteLine("{0} {1} {2}辆 {3} 租金{4}元 装载货物{5}吨 一共{6}元", this.Xuhao, this.Name, this.Number, this.Days, this.Price, this.Weight, (this.Days * this.Price * this.Number)); } } class YiWeiKeGoodsCar : GoodsCar//货车依维柯 { public YiWeiKeGoodsCar():base() { this.Xuhao = 6; this.Name = "依 维柯"; this.Price = 1000; this.Weight = 20; } public YiWeiKeGoodsCar(int days, int number):base(days,number) { this.Xuhao = 6; this.Name = "依 维柯"; this.Price = 1000; this.Weight = 20; } public void toString() { Console.WriteLine("{0} {1} {2}辆 {3} 租金{4}元 装载货物{5}吨 一共{6}元", this.Xuhao, this.Name, this.Number, this.Days, this.Price, this.Weight, (this.Days * this.Price * this.Number)); } } class AoDiA4PeopleCar : PeopleCar //客车奥迪A4 { public AoDiA4PeopleCar():base() { this.Xuhao = 1; this.Name = "奥迪 A4"; this.Price = 500; this.People = 4; } public AoDiA4PeopleCar(int days, int number) : base(days,number) { this.Xuhao = 1; this.Name = "奥迪 A4"; this.Price = 500; this.People = 4; } public void toString() { Console.WriteLine("{0} {1} {2}辆 {3} 租金{4}元 可以坐{5}人 一共{6}元", this.Xuhao, this.Name, this.Number, this.Days, this.Price, this.People, (this.Days * this.Price * this.Number)); } } class MaZiDa6PeopleCar : PeopleCar //客车马自达6 { public MaZiDa6PeopleCar():base() { this.Xuhao = 2; this.Name = "马自达6"; this.Price = 400; this.People = 4; } public MaZiDa6PeopleCar(int days, int number):base(days,number) { this.Xuhao = 2; this.Name = "马自达6"; this.Price = 400; this.People = 4; } public void toString() { Console.WriteLine("{0} {1} {2}辆 {3} 租金{4}元 可以坐{5} 一共{6}元", this.Xuhao, this.Name, this.Number, this.Days, this.Price, this.People, (this.Days * this.Price * this.Number)); } } class JinLongPeopleCar : PeopleCar //客车金龙 { public JinLongPeopleCar():base() { this.Xuhao = 4; this.Name = "金 龙"; this.Price = 800; } public JinLongPeopleCar(int days, int number):base(days,number) { this.Xuhao = 4; this.Name = "金 龙"; this.Price = 800; this.People = 20; } public void toString() { Console.WriteLine("{0} {1} {2}辆 {3} 租金{4}元 可以坐{5} 一共{6}元", this.Xuhao, this.Name, this.Number, this.Days, this.Price, this.People, (this.Days * this.Price * this.Number)); } } class UserException : Exception //自定义异常类 { //重载Excetption类的构造函数 public UserException() { } public UserException(string ms) : base(ms) { } //public UserException(string ms,Exception inner) : base(ms, inner) { } } class Program { static void Main(string[] args) { AoDiA4PeopleCar aodi = new AoDiA4PeopleCar(); //建立六种车的类型 MaZiDa6PeopleCar mazi = new MaZiDa6PeopleCar(); GoodsAndPeopleCar gope = new GoodsAndPeopleCar(); JinLongPeopleCar jinl = new JinLongPeopleCar(); SongHuaJiangGoodsCar songh = new SongHuaJiangGoodsCar(); YiWeiKeGoodsCar yiwe = new YiWeiKeGoodsCar(); Console.WriteLine("\t\t欢迎使用工大租车系统!\n"); Console.WriteLine("================================================"); Console.WriteLine("您是否需要租车:(1是0否)"); //判断是否租车 int a = -1; //提示选车,当类容为输入字母时候提示异, //输入非0和非1提示异常 do { try { a = Convert.ToInt32(Console.ReadLine()); if(a!=0&&a!=1) { //自定义异常,显示输入数字非1和非0的异常 UserException user = new UserException("输入的数字不正确,请输入0或者1!"); throw us da17 er; } } catch (UserException e) //输入数字非1和非0异常信息 { Console.WriteLine("产生异常{0}",e.Message); Console.WriteLine("请您重新选则:"); a = -1; } catch (Exception e) //输入字母时候抛出异常 { Console.WriteLine("产生异常:{0}", e.Message); Console.WriteLine("请您重新选则:"); a = -1; } } while (a == -1); if (a == 1) { //显示租车清单 Console.WriteLine("工大租车系统:(可选列表)"); Console.WriteLine(" 序号\t 汽车名称\t 租金/天 \t 容量"); Console.WriteLine(" 1 \t 奥迪A4 \t 500元 \t 载人:4人"); Console.WriteLine(" 2 \t 马自达6 \t 400元 \t 载人:4人"); Console.WriteLine(" 3 \t 皮卡雪6 \t 450元 \t 载人:4人,载货:2吨"); Console.WriteLine(" 4 \t 金龙 \t 800元 \t 载人:20人"); Console.WriteLine(" 5 \t 松花江 \t 400元 \t 载货:4吨"); Console.WriteLine(" 6 \t 依维柯 \t 1000元 \t 载货:20吨"); Console.WriteLine("请问您需要几种车:"); int m = Convert.ToInt32(Console.ReadLine()); int[,] xingxi = new int[m, 3]; //保存租车信息(序号,车辆数,天数) for (int i = 0; i < m; i++) { Console.WriteLine("请输入第{0}俩车的相关信息:(序号、车辆数、租用天数)", i + 1); Console.Write("序号:"); try //输入序号,如果输入的不是1,2,3,4,5,6,则抛出异常 { xingxi[i, 0] = Convert.ToInt32(Console.ReadLine()); if (xingxi[i, 0]<=0||xingxi[i, 0]>=7) { UserException user = new UserException("输入的车序号不对,重新输入!"); throw user; } } catch(UserException ex) //输入序号不对,显示异常 { Console.WriteLine(ex.Message); xingxi[i, 0] = Convert.ToInt32(Console.ReadLine()); } catch(Exception ex) //输入字母异常 { Console.WriteLine(ex.Message); xingxi[i, 0] = Convert.ToInt32(Console.ReadLine()); } Console.Write("车辆数:"); try //输入车辆数,如果是字母或者是非负数则显示异常,提示重新输入 { xingxi[i, 1] = Convert.ToInt32(Console.ReadLine()); if(xingxi[i,1]<=0) { UserException user = new UserException("输入的车辆数是非正整数!"); throw user; } } catch(UserException ex) { Console.WriteLine("输入异常:{0}", ex.Message); Console.WriteLine("请重新输入车俩数:"); xingxi[i, 1] = Convert.ToInt32(Console.ReadLine()); } catch(Exception ex) { Console.WriteLine("输入异常:{0}", ex.Message); Console.WriteLine("请重新输入车俩数:"); xingxi[i, 1] = Convert.ToInt32(Console.ReadLine()); } Console.Write("租用天数:"); try //输入租车天数,如果是字字母则显示异常,提示重新输入 { xingxi[i, 2] = Convert.ToInt32(Console.ReadLine()); if(xingxi[i,2]<=0) { UserException user = new UserException("输入的车辆数是非正整数!"); throw user; } } catch(UserException ex) { Console.WriteLine(ex.Message); Console.WriteLine("请重新输入车俩数:"); xingxi[i, 2] = Convert.ToInt32(Console.ReadLine()); } catch (Exception ex) { Console.WriteLine("输入异常:{0}", ex.Message); Console.WriteLine("请重新输入车俩数:"); xingxi[i, 2] = Convert.ToInt32(Console.ReadLine()); } } for (int i = 0; i < m; i++) { switch (xingxi[i, 0]) //构造六种车的类型 { case 1: aodi = new AoDiA4PeopleCar(xingxi[i, 2], xingxi[i, 1]); break; case 2: mazi = new MaZiDa6PeopleCar(xingxi[i, 2], xingxi[i, 1]); break; case 3: gope = new GoodsAndPeopleCar(xingxi[i, 2], xingxi[i, 1]); break; case 4: jinl = new JinLongPeopleCar(xingxi[i, 2], xingxi[i, 1]); break; case 5: songh = new SongHuaJiangGoodsCar(xingxi[i, 2], xingxi[i, 1]); break; case 6: yiwe = new YiWeiKeGoodsCar(xingxi[i, 2], xingxi[i, 1]); break; default: Console.WriteLine("选车序号有错误!"); break; } } Console.WriteLine("\n选车完毕,请等候!\n正在为您计算选车方案。。。"); Console.WriteLine("\n\t\t您的租车方案为:"); Console.WriteLine("序号 名称 车辆数 天数 租金/天 容量/车 金额/元"); //通过判断,输出租车清单 if(aodi.Days!=0&&aodi.Number!=0) aodi.toString(); if (mazi.Days != 0 && mazi.Number != 0) mazi.toString(); if (gope.Days!=0&& gope.Number!=0) gope.toString(); if(jinl.Days != 0&&jinl.Number != 0) jinl.toString(); if(songh.Days != 0&&songh.Number != 0) songh.toString(); if(yiwe.Days != 0&&yiwe.Number != 0) yiwe.toString(); Console.WriteLine("\n一共可以坐{0}人,载重{1}吨", (aodi.zr() + mazi.zr() + gope.zr() + jinl.zr()), (gope.zz() + songh.zz() + yiwe.zz())); Console.WriteLine("一共花费的钱为:{0}元 !", aodi.zj() + mazi.zj() + gope.zj() + jinl.zj() + songh.zj() + yiwe.zj()); } else { Console.WriteLine("欢迎光顾工大租车系统!欢迎下次再来!"); } Console.Read(); } } }
运行结果分析:
分析:
1. 在选车的时候,输入如果是字母则抛出异常,异常信息为:产生异常:输入的字符串的格式不正确。如果输入的是非0或非1 的数字,则抛出异常,异常信息为:产生异常输入的数字不正确,请输入0或者1!。如果输入是0,则推出系统,提示信息为:欢迎光顾工大租车系统!欢迎下次再来!。如果是输入1,则进入工大租车系统,显示可以租用的车辆。该位置使用了循环,如果发生异常,则可以一直输入,直到正确为止。
2. 选则车辆的种类,输入数字,表示的是选用的车辆的种类;
3. 提醒输入第一俩车的信息,后面的车辆提示信息以此类推,如果输入的序号不是1~6,则抛出异常,异常信息为:输入的车序号不对;
4. 提示输入车辆数,如果输入的是字母,则引发异常,异常信息为:输入异常:输入字符串的格式不正确。如果输入的是非正数,则引发异常,异常信息为:输入的车辆数是非正数!提示重新输入,该异常的信息只能输错一次,因为是该处没有使用循环,故不能连续输入错误信息。
5. 提示输入天数,异常信息类似于车俩数。
6. 当输入完成时,提供租车方案,格式如图,同时计算总载货量和总载人量,给予输出。
运行结构图:
相关文章推荐
- C#之系统异常处理机制
- C#之系统异常处理机制
- C#.Net开发基于Oracle数据库系统常见异常处理
- C#之工大租车系统
- C#之系统异常处理机制
- 天轰穿C# -vs2010 - 03C#的异常处理之异常处理的系统流程【原创】
- C#之系统异常处理机制
- 初探C# 异常处理
- Head First C# 中文版 第10章 异常处理 page458
- C# 采用系统委托的方式处理线程内操作窗体控件(转载)
- C#以逗号拆分字符串,若字段中包含逗号(备注:包含逗号的字段必须有双引号引用)则对其进行拼接处理
- 编写高质量代码改善C#程序的157个建议——建议65:总是处理未捕获的异常
- C#中unhandled异常处理的问题
- Head First C# 中文版 第10章 异常处理 page478
- C#以逗号拆分字符串,若字段中包含逗号(备注:包含逗号的字段必须有双引号引用)则对其进行拼接处理
- C#异常处理经验(原则与方法)
- C#获取系统相关信息_详细异常信息
- C#中异常处理和Java的区别
- C#调用参数为函数指针的API函数 - 以SetUnhandledExceptionFilter为例编写一个全局异常处理程序
- C# 系统日志处理-生产者与消费者模式