用单链表对学生成绩插入删除查找
2017-10-10 20:40
330 查看
头文件
#ifndef LinkList_H
#define LinkList_H //if not defined,防止头文件的重复包含和编译
template <class student> //定义单链表的结点
struct Node
{
student data;
Node <student>*next;
};
//以下是类LinkList的声明
template <class student>
class LinkList
{
public:
LinkList();
LinkList(student a[],int n);
~LinkList();
int Locate(student x);
void Insert(int i,student x);
student Delete(int i);
void PrintList();
private:
Node<student>*first;
};
#endif
源文件
#include <iostream>
using namespace std;
#include "LinkList.h"
template<class student>
LinkList<student>::LinkList()
{
first=new Node<student>;
first->next=NULL;
}
template<class student>
LinkList<student>::LinkList (student a[],int n)
{
Node<student>*r,*s;
first=new Node<student>;
r=first;
for(int i=0;i<n;i++)
{
s=new Node<student>;
s->data=a[i];
r->next=s;
r=s;
}
r->next=NULL;
}
template<class student>
LinkList<student>::~LinkList()
{
Node<student>*q=NULL;
while(first!=NULL)
{
q=first;
first=first->next;
delete q;
}
}
template<class student>
void LinkList<student>::Insert(int i,student x)
{
Node<student>*p=first,*s=NULL;
int count=0;
while(p!=NULL&&count<i-1)
{
p=p->next;
count++;
}
if(p==NULL) throw"位置";
else{
s=new Node<student>;s->data=x;
s->next=p->next;p->next=s;
}
}
template<class student>
student LinkList<student>::Delete(int i)
{
Node<student>*p=first,*q=NULL;
student x;
int count=0;
while(p!=NULL&&count<i-1)
{
p=p->next;
count++;
}
if(p==NULL||p->next==NULL)
throw"位置";
else{
q=p->next;x=q->data;
p->next=q->next;
delete q;
return x;
}
}
template<class student>
int LinkList<student>::Locate(student x)
{
Node<student>*p=first->next;
int count=1;
while(p!=NULL)
{
if(p->data==x) return count;
p=p->next;
count++;
}
return 0;
}
template<class student>
void LinkList<student>::PrintList()
{
Node <student>*p=first->next;
while(p!=NULL)
{
cout<<p->data<<"";
p=p->next;
}
cout<<endl;
}
源文件主函数
#include <iostream>
using namespace std;
#include "LinkList.cpp"
void main()
{
int r[5]={95,68,75,98,79};
LinkList<int>L(r,5);
cout<<"5个学生的成绩分别是:"<<endl;
L.PrintList();
cout<<"李华成绩为85"<<endl;
try
{
L.Insert(2,85);
}
catch (char *s)
{
cout<<s<<endl;
}<
8baa
br />cout<<"将李华的成绩插入到第二个后成绩为;"<<endl;
L.PrintList();
cout<<"成绩为85的学生排在:";
cout<<L.Locate(85)<<endl;
cout<<"删除第二个学生的成绩前,所有学生成绩分别为:"<<endl;
L.PrintList();
try
{
L.Delete(2);
}
catch (char *s)
{
cout<<s<<endl;
}
cout<<"删除后所有的学生成绩分别为:"<<endl;
L.PrintList();
system("pause");
}
调试后结果
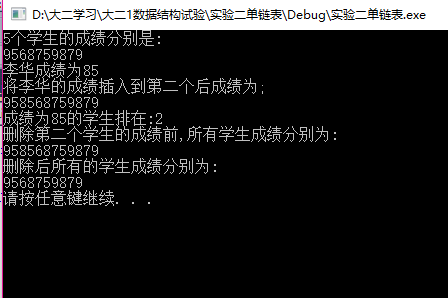
不明白为什么输出的分数之间没有空格,求解求解求解。
#ifndef LinkList_H
#define LinkList_H //if not defined,防止头文件的重复包含和编译
template <class student> //定义单链表的结点
struct Node
{
student data;
Node <student>*next;
};
//以下是类LinkList的声明
template <class student>
class LinkList
{
public:
LinkList();
LinkList(student a[],int n);
~LinkList();
int Locate(student x);
void Insert(int i,student x);
student Delete(int i);
void PrintList();
private:
Node<student>*first;
};
#endif
源文件
#include <iostream>
using namespace std;
#include "LinkList.h"
template<class student>
LinkList<student>::LinkList()
{
first=new Node<student>;
first->next=NULL;
}
template<class student>
LinkList<student>::LinkList (student a[],int n)
{
Node<student>*r,*s;
first=new Node<student>;
r=first;
for(int i=0;i<n;i++)
{
s=new Node<student>;
s->data=a[i];
r->next=s;
r=s;
}
r->next=NULL;
}
template<class student>
LinkList<student>::~LinkList()
{
Node<student>*q=NULL;
while(first!=NULL)
{
q=first;
first=first->next;
delete q;
}
}
template<class student>
void LinkList<student>::Insert(int i,student x)
{
Node<student>*p=first,*s=NULL;
int count=0;
while(p!=NULL&&count<i-1)
{
p=p->next;
count++;
}
if(p==NULL) throw"位置";
else{
s=new Node<student>;s->data=x;
s->next=p->next;p->next=s;
}
}
template<class student>
student LinkList<student>::Delete(int i)
{
Node<student>*p=first,*q=NULL;
student x;
int count=0;
while(p!=NULL&&count<i-1)
{
p=p->next;
count++;
}
if(p==NULL||p->next==NULL)
throw"位置";
else{
q=p->next;x=q->data;
p->next=q->next;
delete q;
return x;
}
}
template<class student>
int LinkList<student>::Locate(student x)
{
Node<student>*p=first->next;
int count=1;
while(p!=NULL)
{
if(p->data==x) return count;
p=p->next;
count++;
}
return 0;
}
template<class student>
void LinkList<student>::PrintList()
{
Node <student>*p=first->next;
while(p!=NULL)
{
cout<<p->data<<"";
p=p->next;
}
cout<<endl;
}
源文件主函数
#include <iostream>
using namespace std;
#include "LinkList.cpp"
void main()
{
int r[5]={95,68,75,98,79};
LinkList<int>L(r,5);
cout<<"5个学生的成绩分别是:"<<endl;
L.PrintList();
cout<<"李华成绩为85"<<endl;
try
{
L.Insert(2,85);
}
catch (char *s)
{
cout<<s<<endl;
}<
8baa
br />cout<<"将李华的成绩插入到第二个后成绩为;"<<endl;
L.PrintList();
cout<<"成绩为85的学生排在:";
cout<<L.Locate(85)<<endl;
cout<<"删除第二个学生的成绩前,所有学生成绩分别为:"<<endl;
L.PrintList();
try
{
L.Delete(2);
}
catch (char *s)
{
cout<<s<<endl;
}
cout<<"删除后所有的学生成绩分别为:"<<endl;
L.PrintList();
system("pause");
}
调试后结果
不明白为什么输出的分数之间没有空格,求解求解求解。
相关文章推荐
- 用顺序表插入删除查找学生成绩
- 设计一个学生成绩管理系统,实现对学生成绩的动态管理,实现对学生成绩的输入,显示,删除,查找,插入,最佳,保存,计算,排序等主要功能
- 单链表的初始化,建立,插入,查找,删除
- c语言实现单链表建立,插入,删除,查找,循环链表,静态链表
- 链表的删除,插入,查找,排序
- 链表的实现(查找,插入,删除)
- 单链表的初始化 建立 插入 查找 删除(转自newwy)
- 单链表操作(建表、插入、删除、查找、求表长),
- 一个链表程序,支持建立,插入,删除,输出;学生的学号和分数
- 链表之节点插入、查找删除、遍历打印、遍历释放
- 单链表建立,插入,删除,查找,遍历操作
- 利用模板类编写一个程序,实现双向链表的插入、删除、查找、显示的功能。
- 链表(单双链表)用法与基本操作(构建、查找、插入、删除)实现
- 关于链表结构的基本操作 c 实现 (创建,插入删除,反转,合并链表,查找,是否有环,链表相交情况)
- 用JAVA语言,编写一个链表类(双向链表),实现插入,删除,查找操作
- 单链表很全的例子,插入,删除,,查找,排序
- 单链表的游标实现:插入、删除、查找、打印等功能
- 【数据结构作业二】写出单链表结点的结构体类型定义及查找、插入、删除算法,并以单链表作存储结构,实现有序表的合并
- 单链表 初始化 创建 头插法 尾插法 插入 删除 查找 合并 长度
- 单链表的初始化,建立,插入,查找,删除