java使用FileUpload实现文件的上传和下载
2017-10-10 11:11
771 查看
文件的上传和下载在平时的生活中是用的也挺广泛,例如qq,qq邮箱,csdn登都会用到这一技术,可以说,上传和下载在生活中的使用和频繁也很常见。
在这里先总结下文件上传的要点:
1)提交方式必须为POST提交
2)表单中有文件上传类型的表单项,例如<input type="file"/>
3)指定表单类型必须为:enctype="multipart/form-data",
而默认的表单类型为:enctype="application/x-www-form-urlencoded"
在这里我们使用Apache提供的文件上传组件:FileUpload组件
我们首先引入相应的jar包:
commons-fileupload-1.2.1.jar 【文件上传组件核心jar包】
commons-io-1.4.jar 【封装了对文件处理的相关工具类】
接下来我们就来实现一下文件和上传的一个小案例
首先我们写一个fileindex.jsp页面,用于给定相应的链接
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>文件上传和下载案例</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
</head>
<body>
<a href="${pageContext.request.contextPath}/upload.jsp">文件上传</a>
<a href="${pageContext.request.contextPath}/fileServlet?method=downlist">下载列表</a>
</body>
</html>
然后对应写好文件上传的前台upload.jsp
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>文件上传</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
</head>
<body>
<fieldset>
<legend>文件上传表单</legend>
<form action="${pageContext.
e6bb
request.contextPath }/fileServlet?method=upload" enctype="multipart/form-data" method="POST">
文件名:<input type="text" name="username"/><br/><br/>
文件:<input type="file" name="file_img"/><br/><br/>
<input type="submit" value="提交">
</form>
</fieldset>
</body>
</html>
然后在FileServlet写一个处理上传的表单的方法upload
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
String method = req.getParameter("method");
if ("upload".equals(method)) {
//文件上传
upload(req, resp);
}else if("downlist".equals(method)){
//下载列表
downList(req, resp);
}else if ("download".equals(method)) {
//下载
download(req, resp);
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
doGet(req, resp);
}
/**
* 文件上传
* @param req
* @param resp
* @throws ServletException
* @throws IOException
*/
@SuppressWarnings("static-access")
protected void upload(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
try {
//创建上传文件工厂类
DiskFileItemFactory factory = new DiskFileItemFactory();
//创建FileItem对象
ServletFileUpload upload = new ServletFileUpload(factory);
//对文件进行限制
//设置单个文件最大大小
upload.setFileSizeMax(30*1024*1024);
//设置总大小
upload.setSizeMax(100*1024*1024);
//设置编码
upload.setHeaderEncoding("utf-8");
//判断是否为上传表单
if (upload.isMultipartContent(req)) {
//将请求的数据封装到FileItem集合中
List<FileItem> items = upload.parseRequest(req);
//遍历各个文件项
for (FileItem item : items) {
//获取元素名
String fieldName = item.getFieldName();
//普通文本数据
if (item.isFormField()) {
//获取值
String fieldValue = item.getString("utf-8");
System.out.println(fieldValue);
}else{
//文件表单项
//使用uuid给文件命名防止重复
String id = UUID.randomUUID().toString();
//获取原本的文件名
String fileName = item.getName();
//拼接上传的文件名
fileName = id + "#" + fileName;
//得到上传目录
String path = getServletContext().getRealPath("/upload");
File file = new File(path,fileName);
//上传
item.write(file);
//删除临时文件
item.delete();
//提交成功后跳转到下载列表页面
req.getRequestDispatcher("/fileServlet?method=downlist").forward(req, resp);
}
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
当上传成功后跳通过传入参数method=downlist跳转到下载列表的Servlet,于是我们在FileServlet里面再写一个方法downList方法
/**
* 进入下载列表
* @param req
* @param resp
* @throws ServletException
* @throws IOException
*/
protected void downList(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
// 实现思路:先获取upload目录下所有文件的文件名,再保存;跳转到down.jsp列表展示
HashMap<String, String> fileNames = new HashMap<>();
//获取上传文件的路径以及文件名
String path = getServletContext().getRealPath("/upload");
File file = new File(path);
String[] list = file.list();
if (list != null && list.length > 0) {
for (int i = 0; i < list.length; i++) {
//获取全名
String fileName = list[i];
//获取短名
String shortName = fileName.substring(fileName.lastIndexOf("#")+1);
//封装
fileNames.put(fileName, shortName);
}
//保存到request域中
req.setAttribute("fileNames", fileNames);
//转发
req.getRequestDispatcher("/downlist.jsp").forward(req, resp);
}
}然后跳转到downlist.jsp页面
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>下载列表</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<style>
table{
margin: 100px auto;
width:350px;
height:150px;
}
</style>
</head>
<body>
<table border="1" align="center">
<tr>
<th>序号</th>
<th>文件名</th>
<th>操作</th>
</tr>
<c:forEach varStatus="nums" var="en" items="${requestScope.fileNames }">
<tr>
<td>${nums.count }</td>
<td>${en.value }</td>
<td>
<!-- 构建一个地址 -->
<c:url var="url" value="fileServlet">
<c:param name="method" value="download"></c:param>
<c:param name="fileName" value="${en.key }"></c:param>
</c:url>
<a href="${url }">下载</a>
</td>
</tr>
</c:forEach>
</table>
</body>
</html>
当点击下载后,跳回FileServlet页面,并传入一个参数method=download,进入到下载的方法中
/**
* 下载文件
* @param req
* @param resp
* @throws ServletException
* @throws IOException
*/
protected void download(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
//获取用户下载的文件名称
String fileName = req.getParameter("fileName");
//对get请求进行转码
fileName = new String(fileName.getBytes("ISO8859-1"), "UTF-8");
// 先获取上传目录路径
String path = getServletContext().getRealPath("/upload");
// 获取一个文件流
FileInputStream in = new FileInputStream(new File(path,fileName));
// 如果文件名是中文,需要进行url编码
fileName = URLEncoder.encode(fileName,"UTF-8");
// 设置下载的响应头
resp.setHeader("content-desposition", "attachment;fileName=" + fileName);
// 获取字节输出流 输出文件
OutputStream out = resp.getOutputStream();
byte[] buf = new byte[1024];
int len = -1;
while((len = in.read(buf)) != -1){
out.write(buf,0,len);
}
//关闭流
out.close();
in.close();
}
这样一个简单的上传和下载的案例就完成了
运行结果如下:
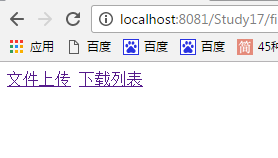
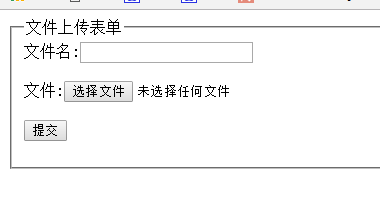
在这里先总结下文件上传的要点:
1)提交方式必须为POST提交
2)表单中有文件上传类型的表单项,例如<input type="file"/>
3)指定表单类型必须为:enctype="multipart/form-data",
而默认的表单类型为:enctype="application/x-www-form-urlencoded"
在这里我们使用Apache提供的文件上传组件:FileUpload组件
我们首先引入相应的jar包:
commons-fileupload-1.2.1.jar 【文件上传组件核心jar包】
commons-io-1.4.jar 【封装了对文件处理的相关工具类】
接下来我们就来实现一下文件和上传的一个小案例
首先我们写一个fileindex.jsp页面,用于给定相应的链接
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>文件上传和下载案例</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
</head>
<body>
<a href="${pageContext.request.contextPath}/upload.jsp">文件上传</a>
<a href="${pageContext.request.contextPath}/fileServlet?method=downlist">下载列表</a>
</body>
</html>
然后对应写好文件上传的前台upload.jsp
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>文件上传</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
</head>
<body>
<fieldset>
<legend>文件上传表单</legend>
<form action="${pageContext.
e6bb
request.contextPath }/fileServlet?method=upload" enctype="multipart/form-data" method="POST">
文件名:<input type="text" name="username"/><br/><br/>
文件:<input type="file" name="file_img"/><br/><br/>
<input type="submit" value="提交">
</form>
</fieldset>
</body>
</html>
然后在FileServlet写一个处理上传的表单的方法upload
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
String method = req.getParameter("method");
if ("upload".equals(method)) {
//文件上传
upload(req, resp);
}else if("downlist".equals(method)){
//下载列表
downList(req, resp);
}else if ("download".equals(method)) {
//下载
download(req, resp);
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
doGet(req, resp);
}
/**
* 文件上传
* @param req
* @param resp
* @throws ServletException
* @throws IOException
*/
@SuppressWarnings("static-access")
protected void upload(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
try {
//创建上传文件工厂类
DiskFileItemFactory factory = new DiskFileItemFactory();
//创建FileItem对象
ServletFileUpload upload = new ServletFileUpload(factory);
//对文件进行限制
//设置单个文件最大大小
upload.setFileSizeMax(30*1024*1024);
//设置总大小
upload.setSizeMax(100*1024*1024);
//设置编码
upload.setHeaderEncoding("utf-8");
//判断是否为上传表单
if (upload.isMultipartContent(req)) {
//将请求的数据封装到FileItem集合中
List<FileItem> items = upload.parseRequest(req);
//遍历各个文件项
for (FileItem item : items) {
//获取元素名
String fieldName = item.getFieldName();
//普通文本数据
if (item.isFormField()) {
//获取值
String fieldValue = item.getString("utf-8");
System.out.println(fieldValue);
}else{
//文件表单项
//使用uuid给文件命名防止重复
String id = UUID.randomUUID().toString();
//获取原本的文件名
String fileName = item.getName();
//拼接上传的文件名
fileName = id + "#" + fileName;
//得到上传目录
String path = getServletContext().getRealPath("/upload");
File file = new File(path,fileName);
//上传
item.write(file);
//删除临时文件
item.delete();
//提交成功后跳转到下载列表页面
req.getRequestDispatcher("/fileServlet?method=downlist").forward(req, resp);
}
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
当上传成功后跳通过传入参数method=downlist跳转到下载列表的Servlet,于是我们在FileServlet里面再写一个方法downList方法
/**
* 进入下载列表
* @param req
* @param resp
* @throws ServletException
* @throws IOException
*/
protected void downList(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
// 实现思路:先获取upload目录下所有文件的文件名,再保存;跳转到down.jsp列表展示
HashMap<String, String> fileNames = new HashMap<>();
//获取上传文件的路径以及文件名
String path = getServletContext().getRealPath("/upload");
File file = new File(path);
String[] list = file.list();
if (list != null && list.length > 0) {
for (int i = 0; i < list.length; i++) {
//获取全名
String fileName = list[i];
//获取短名
String shortName = fileName.substring(fileName.lastIndexOf("#")+1);
//封装
fileNames.put(fileName, shortName);
}
//保存到request域中
req.setAttribute("fileNames", fileNames);
//转发
req.getRequestDispatcher("/downlist.jsp").forward(req, resp);
}
}然后跳转到downlist.jsp页面
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>下载列表</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<style>
table{
margin: 100px auto;
width:350px;
height:150px;
}
</style>
</head>
<body>
<table border="1" align="center">
<tr>
<th>序号</th>
<th>文件名</th>
<th>操作</th>
</tr>
<c:forEach varStatus="nums" var="en" items="${requestScope.fileNames }">
<tr>
<td>${nums.count }</td>
<td>${en.value }</td>
<td>
<!-- 构建一个地址 -->
<c:url var="url" value="fileServlet">
<c:param name="method" value="download"></c:param>
<c:param name="fileName" value="${en.key }"></c:param>
</c:url>
<a href="${url }">下载</a>
</td>
</tr>
</c:forEach>
</table>
</body>
</html>
当点击下载后,跳回FileServlet页面,并传入一个参数method=download,进入到下载的方法中
/**
* 下载文件
* @param req
* @param resp
* @throws ServletException
* @throws IOException
*/
protected void download(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
//获取用户下载的文件名称
String fileName = req.getParameter("fileName");
//对get请求进行转码
fileName = new String(fileName.getBytes("ISO8859-1"), "UTF-8");
// 先获取上传目录路径
String path = getServletContext().getRealPath("/upload");
// 获取一个文件流
FileInputStream in = new FileInputStream(new File(path,fileName));
// 如果文件名是中文,需要进行url编码
fileName = URLEncoder.encode(fileName,"UTF-8");
// 设置下载的响应头
resp.setHeader("content-desposition", "attachment;fileName=" + fileName);
// 获取字节输出流 输出文件
OutputStream out = resp.getOutputStream();
byte[] buf = new byte[1024];
int len = -1;
while((len = in.read(buf)) != -1){
out.write(buf,0,len);
}
//关闭流
out.close();
in.close();
}
这样一个简单的上传和下载的案例就完成了
运行结果如下:
相关文章推荐
- JAVA-使用commos-fileupload实现文件上传与下载
- JAVA使用commos-fileupload实现文件上传与下载实例解析
- JAVA中使用FTPClient实现文件上传下载(键人岐)
- JAVA中使用FTPClient实现文件上传下载
- JAVA中使用FTPClient实现文件上传下载
- JAVA中使用FTPClient实现文件上传下载
- Java 使用 SFTP 实现文件上传下载(一)
- Java 使用Socket实现文件上传与下载
- Java使用servlet实现文件上传至数据库和从数据库下载文件
- JAVA中使用FTPClient实现文件上传下载
- 使用ajaxFileUpload与SpringMVC实现异步上传下载文件并返回json数据
- java利用commons-fileupload组件实现文件的上传与下载
- Java中使用fileupload组件实现文件上传功能的实例代码
- java使用common-fileupload实现文件上传
- JAVA中使用FTPClient实现文件上传下载
- JAVA中使用FTPClient实现文件上传下载
- JAVA中使用FTPClient实现文件上传下载实例代码
- JAVA中使用FTPClient实现文件上传下载
- JAVAWEB开发之文件的上传与下载(开源组件commons-fileupload的详细使用)
- 使用commos-fileupload实现文件上传与下载