利用构造函数和原型链做的简单的飞机大战游戏
2017-10-09 11:43
211 查看
思路:一个飞机大战游戏,应该有我方飞机,子弹,敌方飞机(分为大中小三等)还有分数的积分。
//步骤:
1.我方飞机的构造函数
创建我方飞机
我方飞机运动
我方飞机发射子弹
2.子弹的构造函数
子弹的创建
子弹的移动
子弹和飞机的碰撞检测
3.敌方飞机的构造函数
创建敌飞机
敌军移动
敌机碰撞
随机设置敌机大小
4.实例化。
以下是html部分代码
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>飞机大战</title>
<style>
*{
padding: 0;
margin: 0;
}
#plane{
width: 320px;
height: 568px;
background: url(img/background.png);
margin: 30px auto 0px;
position: relative;
cursor: none;
overflow: hidden;
}
#plane span{
position: absolute;
right: 10px;
top: 10px;
}
#plane span i{
font-style:normal;
}
</style>
</head>
<body>
<div id="plane">
<span>慧新小盆友的分数:<i>0</i>分</span>
</div>
<script src="plane.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
plane.js部分
var outBox=document.getElementById('plane');
var Ascore=document.getElementsByTagName('i')[0];
var sumScore=0;
/*/-------------------------------我放飞机---------------------------------------------/*/
function Myplane(w,h,x,y,iurl,burl){
this.w=w;
this.h=h;
this.x=x;
this.y=y;
this.iurl=iurl;
this.burl=burl;
this.createMyplane();
}
Myplane.prototype.createMyplane=function(){
this.imgmyplane=document.createElement('img');
this.imgmyplane.src=this.iurl;
this.imgmyplane.style.cssText='width:'+this.w+'px;height:'+this.h+'ps;position:absolute;left:'+this.x+'px;top:'+this.y+'px;';
outBox.appendChild(this.imgmyplane);
this.myplaneMove();
this.myplaneShoot();
};
Myplane.prototype.myplaneMove=function(){
var that=this;
document.onmousemove=function(ev){
var ev=ev||window.event;
that.ml=ev.clientX-outBox.offsetLeft-that.imgmyplane.offsetWidth/2;
that.mt=ev.clientY-outBox.offsetTop-that.imgmyplane.offsetHeight/2;
if(that.ml<0){
that.ml=0;
}else if(that.ml>outBox.offsetWidth-that.imgmyplane.offsetWidth){
that.ml=outBox.offsetWidth-that.imgmyplane.offsetWidth;
}
if(that.mt<0){
that.mt=0;
}else if(that.mt>outBox.offsetHeight-that.imgmyplane.offsetHeight){
that.mt=outBox.offsetHeight-that.imgmyplane.offsetHeight;
}
that.imgmyplane.style.left=that.ml+'px';
that.imgmyplane.style.top=that.mt+'px';
return false; //用来阻止默认事件即系统默认事件
}
}
Myplane.prototype.myplaneShoot=function(){
var that=this;
document.onmousedown=function(ev){
var ev=ev||window.event;
if(ev.which==1){//??????????????????????????????????????为啥等于一
shootbullet();
function shootbullet(){
new Bullet(6,14,that.imgmyplane.offsetLeft+that.imgmyplane.offsetWidth/2-3,that.imgmyplane.offsetTop-14,'img/bullet.png');//为啥减三,为啥减14
}
that.shoottimer=setInterval(shootbullet,400);
}
}
document.onmouseup=function(){
clearInterval(that.shoottimer);
}
document.oncontextmenu=function(){
return false;
}
}
/*/-------------------------------子弹----------------------------------------------/*/
function Bullet(w,h,x,y,iurl){
this.w=w;
this.h=h;
this.x=x;
this.y=y;
this.iurl=iurl;
this.createBullet();
}
Bullet.prototype.createBullet=function(){
this.imgbullet=document.createElement('img');
this.imgbullet.src=this.iurl;
this.imgbullet.style.cssText='width:'+this.w+'px;height:'+this.h+'px;position:absolute;left:'+this.x+'px;top:'+this.y+'px;';
outBox.appendChild(this.imgbullet);
this.bulletMove();
}
Bullet.prototype.bulletMove=function(){
var that=this;
this.bullettimer=setInterval(function(){
that.y-=5;
if(that.y<=-that.h){
clearInterval(that.bullettimer);
outBox.removeChild(that.imgbullet);
}
that.imgbullet.style.top=that.y+'px';
that.bulletHit();
},20)
}
Bullet.prototype.bulletHit=function(){
var army=document.querySelectorAll('.army');
for(var i=0;i<army.length;i++){
if((this.x+this.w)>=army[i].offsetLeft&&this.x<=(army[i].offsetLeft+army[i].offsetWidth)&&(this.y+this.h)>=army[i].offsetTop&&this.y<=(army[i].offsetTop+army[i].offsetHeight)){
clearInterval(this.bullettimer);
try{
outBox.removeChild(this.imgbullet);
}catch(e){
return;
}
army[i].blood--;
army[i].jiancha();
}
}
}
function Army(w,h,x,y,iurl,burl,speed,blood,score){
this.w=w;
this.h=h;
this.x=x;
this.y=y;
this.iurl=iurl;
this.burl=burl;
this.speed=speed;
this.blood=blood;
this.score=score;
this.createArmy();
}
Army.prototype.createArmy=function(){
var that=this;
this.imgarmy=document.createElement('img');
this.imgarmy.src=this.iurl;
this.imgarmy.style.cssText='width:'+this.w+'px;height:'+this.h+'px;position:absolute;left:'+this.x+'px;top:'+this.y+'px;';
this.imgarmy.className='army';
outBox.appendChild(this.imgarmy);
this.imgarmy.blood=this.blood;
this.imgarmy.score=this.score;
this.imgarmy.jiancha=function(){
if(this.blood<=0){
this.className='';
clearInterval(that.imgarmy.timer);
this.src=that.burl;
setTimeout(function(){
outBox.removeChild(that.imgarmy);
},500);
sumScore+=this.score;
Ascore.innerHTML=sumScore;
}
}
this.armyMove();
}
Army.prototype.armyMove=function(){
var that=this;
this.imgarmy.timer=setInterval(function(){
that.y+=that.speed;
if(that.y>=outBox.offsetHeight){
clearInterval(that.imgarmy.timer);
outBox.removeChild(that.imgarmy);
}
that.imgarmy.style.top=that.y+'px';
that.armyHit();
},20)
}
Army.prototype.armyHit=function(){
if((this.x+this.w)>=ourplane.ml&& this.x<=(ourplane.ml+ourplane.w) && (this.y+this.h)>=ourplane.mt && this.y<=(ourplane.mt+ourplane.h)){
var army=document.querySelectorAll('.army');
for(var i=0;i<army.length;i++){
clearInterval(army[i].timer);
}
clearInterval(onearmytimer);
document.onmousemove=null;
document.onmousedown=null;
ourplane.imgmyplane.src=ourplane.burl;
setTimeout(function(){
alert('哈哈哈哈,死翘翘了吧!');
window.location.reload();
},1000);
}
}
var onearmytimer=setInterval(function(){
for(var i=0;i<ranNum(1,5);i++){
var num=ranNum(1,20);
if(num<15){
new Army(34,24,ranNum(0,outBox.offsetWidth-34),-24,'img/smallplane.png','img/smallplaneboom.gif',ranNum(1,3),1,1);
}else if(num>=15&&num<=20){
new Army(46,60,ranNum(0,outBox.offsetWidth-46),-60,'img/midplane.png','img/midplaneboom.gif',ranNum(1,2),3,3);
}else if(num==20){
new Army(110,164,ranNum(0,outBox.offsetWidth-110),-164,'img/bigplane.png','img/bigplaneboom.gif',ranNum(1,1),10,10);
}
}
},2000);
var ourplane=new Myplane(66,80,(outBox.offsetWidth-66)/2,outBox.offsetHeight-80,'img/myplane.gif','img/myplaneBoom.gif');
function ranNum(min,max){
return Math.floor(Math.random()*(max-min+1)+min);
}
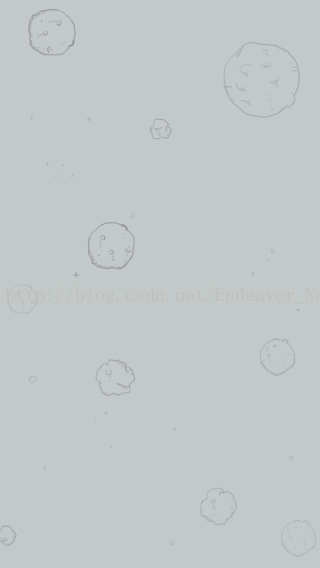
背景图
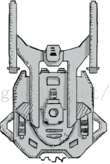
敌方大飞机
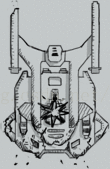
敌方被毁飞机

子弹





//步骤:
1.我方飞机的构造函数
创建我方飞机
我方飞机运动
我方飞机发射子弹
2.子弹的构造函数
子弹的创建
子弹的移动
子弹和飞机的碰撞检测
3.敌方飞机的构造函数
创建敌飞机
敌军移动
敌机碰撞
随机设置敌机大小
4.实例化。
以下是html部分代码
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>飞机大战</title>
<style>
*{
padding: 0;
margin: 0;
}
#plane{
width: 320px;
height: 568px;
background: url(img/background.png);
margin: 30px auto 0px;
position: relative;
cursor: none;
overflow: hidden;
}
#plane span{
position: absolute;
right: 10px;
top: 10px;
}
#plane span i{
font-style:normal;
}
</style>
</head>
<body>
<div id="plane">
<span>慧新小盆友的分数:<i>0</i>分</span>
</div>
<script src="plane.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
plane.js部分
var outBox=document.getElementById('plane');
var Ascore=document.getElementsByTagName('i')[0];
var sumScore=0;
/*/-------------------------------我放飞机---------------------------------------------/*/
function Myplane(w,h,x,y,iurl,burl){
this.w=w;
this.h=h;
this.x=x;
this.y=y;
this.iurl=iurl;
this.burl=burl;
this.createMyplane();
}
Myplane.prototype.createMyplane=function(){
this.imgmyplane=document.createElement('img');
this.imgmyplane.src=this.iurl;
this.imgmyplane.style.cssText='width:'+this.w+'px;height:'+this.h+'ps;position:absolute;left:'+this.x+'px;top:'+this.y+'px;';
outBox.appendChild(this.imgmyplane);
this.myplaneMove();
this.myplaneShoot();
};
Myplane.prototype.myplaneMove=function(){
var that=this;
document.onmousemove=function(ev){
var ev=ev||window.event;
that.ml=ev.clientX-outBox.offsetLeft-that.imgmyplane.offsetWidth/2;
that.mt=ev.clientY-outBox.offsetTop-that.imgmyplane.offsetHeight/2;
if(that.ml<0){
that.ml=0;
}else if(that.ml>outBox.offsetWidth-that.imgmyplane.offsetWidth){
that.ml=outBox.offsetWidth-that.imgmyplane.offsetWidth;
}
if(that.mt<0){
that.mt=0;
}else if(that.mt>outBox.offsetHeight-that.imgmyplane.offsetHeight){
that.mt=outBox.offsetHeight-that.imgmyplane.offsetHeight;
}
that.imgmyplane.style.left=that.ml+'px';
that.imgmyplane.style.top=that.mt+'px';
return false; //用来阻止默认事件即系统默认事件
}
}
Myplane.prototype.myplaneShoot=function(){
var that=this;
document.onmousedown=function(ev){
var ev=ev||window.event;
if(ev.which==1){//??????????????????????????????????????为啥等于一
shootbullet();
function shootbullet(){
new Bullet(6,14,that.imgmyplane.offsetLeft+that.imgmyplane.offsetWidth/2-3,that.imgmyplane.offsetTop-14,'img/bullet.png');//为啥减三,为啥减14
}
that.shoottimer=setInterval(shootbullet,400);
}
}
document.onmouseup=function(){
clearInterval(that.shoottimer);
}
document.oncontextmenu=function(){
return false;
}
}
/*/-------------------------------子弹----------------------------------------------/*/
function Bullet(w,h,x,y,iurl){
this.w=w;
this.h=h;
this.x=x;
this.y=y;
this.iurl=iurl;
this.createBullet();
}
Bullet.prototype.createBullet=function(){
this.imgbullet=document.createElement('img');
this.imgbullet.src=this.iurl;
this.imgbullet.style.cssText='width:'+this.w+'px;height:'+this.h+'px;position:absolute;left:'+this.x+'px;top:'+this.y+'px;';
outBox.appendChild(this.imgbullet);
this.bulletMove();
}
Bullet.prototype.bulletMove=function(){
var that=this;
this.bullettimer=setInterval(function(){
that.y-=5;
if(that.y<=-that.h){
clearInterval(that.bullettimer);
outBox.removeChild(that.imgbullet);
}
that.imgbullet.style.top=that.y+'px';
that.bulletHit();
},20)
}
Bullet.prototype.bulletHit=function(){
var army=document.querySelectorAll('.army');
for(var i=0;i<army.length;i++){
if((this.x+this.w)>=army[i].offsetLeft&&this.x<=(army[i].offsetLeft+army[i].offsetWidth)&&(this.y+this.h)>=army[i].offsetTop&&this.y<=(army[i].offsetTop+army[i].offsetHeight)){
clearInterval(this.bullettimer);
try{
outBox.removeChild(this.imgbullet);
}catch(e){
return;
}
army[i].blood--;
army[i].jiancha();
}
}
}
function Army(w,h,x,y,iurl,burl,speed,blood,score){
this.w=w;
this.h=h;
this.x=x;
this.y=y;
this.iurl=iurl;
this.burl=burl;
this.speed=speed;
this.blood=blood;
this.score=score;
this.createArmy();
}
Army.prototype.createArmy=function(){
var that=this;
this.imgarmy=document.createElement('img');
this.imgarmy.src=this.iurl;
this.imgarmy.style.cssText='width:'+this.w+'px;height:'+this.h+'px;position:absolute;left:'+this.x+'px;top:'+this.y+'px;';
this.imgarmy.className='army';
outBox.appendChild(this.imgarmy);
this.imgarmy.blood=this.blood;
this.imgarmy.score=this.score;
this.imgarmy.jiancha=function(){
if(this.blood<=0){
this.className='';
clearInterval(that.imgarmy.timer);
this.src=that.burl;
setTimeout(function(){
outBox.removeChild(that.imgarmy);
},500);
sumScore+=this.score;
Ascore.innerHTML=sumScore;
}
}
this.armyMove();
}
Army.prototype.armyMove=function(){
var that=this;
this.imgarmy.timer=setInterval(function(){
that.y+=that.speed;
if(that.y>=outBox.offsetHeight){
clearInterval(that.imgarmy.timer);
outBox.removeChild(that.imgarmy);
}
that.imgarmy.style.top=that.y+'px';
that.armyHit();
},20)
}
Army.prototype.armyHit=function(){
if((this.x+this.w)>=ourplane.ml&& this.x<=(ourplane.ml+ourplane.w) && (this.y+this.h)>=ourplane.mt && this.y<=(ourplane.mt+ourplane.h)){
var army=document.querySelectorAll('.army');
for(var i=0;i<army.length;i++){
clearInterval(army[i].timer);
}
clearInterval(onearmytimer);
document.onmousemove=null;
document.onmousedown=null;
ourplane.imgmyplane.src=ourplane.burl;
setTimeout(function(){
alert('哈哈哈哈,死翘翘了吧!');
window.location.reload();
},1000);
}
}
var onearmytimer=setInterval(function(){
for(var i=0;i<ranNum(1,5);i++){
var num=ranNum(1,20);
if(num<15){
new Army(34,24,ranNum(0,outBox.offsetWidth-34),-24,'img/smallplane.png','img/smallplaneboom.gif',ranNum(1,3),1,1);
}else if(num>=15&&num<=20){
new Army(46,60,ranNum(0,outBox.offsetWidth-46),-60,'img/midplane.png','img/midplaneboom.gif',ranNum(1,2),3,3);
}else if(num==20){
new Army(110,164,ranNum(0,outBox.offsetWidth-110),-164,'img/bigplane.png','img/bigplaneboom.gif',ranNum(1,1),10,10);
}
}
},2000);
var ourplane=new Myplane(66,80,(outBox.offsetWidth-66)/2,outBox.offsetHeight-80,'img/myplane.gif','img/myplaneBoom.gif');
function ranNum(min,max){
return Math.floor(Math.random()*(max-min+1)+min);
}
背景图
敌方大飞机
敌方被毁飞机
子弹
相关文章推荐
- jQuery轮播图(二)利用构造函数和原型创建对象以实现继承
- jQuery图片轮播(二)利用构造函数和原型创建对象以实现继承
- JavaScript利用构造函数和原型的方式模拟C#类的功能
- JavaScript利用构造函数和原型的方式模拟C#类的功能
- 利用Java编写简单的WebService实例
- Effective TensorFlow Chapter 9: TensorFlow模型原型的设计和利用python ops的高级可视化
- 利用OC类和可变数组制作简单的通讯录(基于控制台)
- VMware的简单网络配置及利用Putty远程登陆RHEL
- C#利用反射动态创建对象 带参数的构造函数和String类型
- PHP on Windows Azure 入门教学系列(2) ——利用SQL Azure做一个简单的访问计数器
- Objective-C 原型模式 -- 简单介绍和使用
- 利用MFC简单的显示一个bmp图像
- iBATIS中1:N查询结果利用groupBy功能实现的简单配置
- 用UDP实现可靠文件传输,如何利用UDX创建一个简单的WIN32程序
- C#--第四周实验--任务2--定义一个描述坐标点的CPoint类,利用(默认参数值)构造函数传递参数。(控制台应用)
- javascript简单原型
- 构建简单原型展示系统,使用git钩子(hooks)自动同步仓库
- php中利用memcache/memecached构造简单消息队列
- ASP利用Stream方式生成静态页 [函数可重复调用,简单易用]
- javascript继承,原型继承,借用构造函数继承,混合继承