CasperJS API 第一篇
2017-09-11 18:13
211 查看
Casperjs 官方API
获得Casper实例两种方式
var casper = require(‘casper’).create();
var casper = new require(‘casper’).Casper();
create()函数传参数
var casper = require(‘casper’).create({
clientScripts: [
‘includes/jquery.js’, // These two scripts will be injected in remote
‘includes/underscore.js’ // DOM on every request
],
pageSettings: {
loadImages: false, // The WebPage instance used by Casper will
loadPlugins: false // use these settings
},
logLevel: “info”, // Only “info” level messages will be logged
verbose: true // log messages will be printed out to the console
});
或者在运行时传递参数
var casper = require(‘casper’).create();
casper.options.waitTimeout = 1000;
更多参数请查看官方文档
back()
casper.start(‘http://foo.bar/1‘);
casper.thenOpen(‘http://foo.bar/2‘);
casper.thenOpen(‘http://foo.bar/3‘);
casper.back(); //回退页面函数
casper.run(function() {
console.log(this.getCurrentUrl()); // ‘http://foo.bar/2’ });
base64encode()
post请求encode
Selectors
检查

是否存在
Selectors-CSS3
使用测试框架
Selectors-Xpath
建议下面的用法
Warning
The only limitation of XPath use in CasperJS is in the casper.fill() method when you want to fill file fields; PhantomJS natively only allows the use of CSS3 selectors in its uploadFile method, hence this limitation.
click()
Signature: click(String selector, [Number|String X, Number|String Y]) //selector支持CSS3和XPath
clickLabel()
…
capture()
captureBase64()
captureSelector()
clear()
clearCache()
clearMemoryCache()
debugHTML()
debugPage()
die()
…
download()
each()
eachThen()
echo()
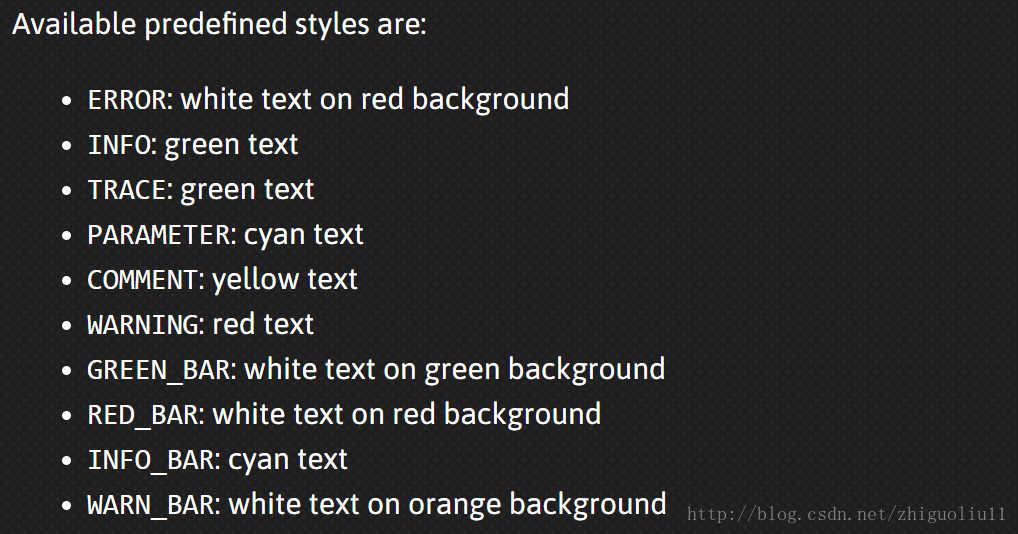
evaluate()
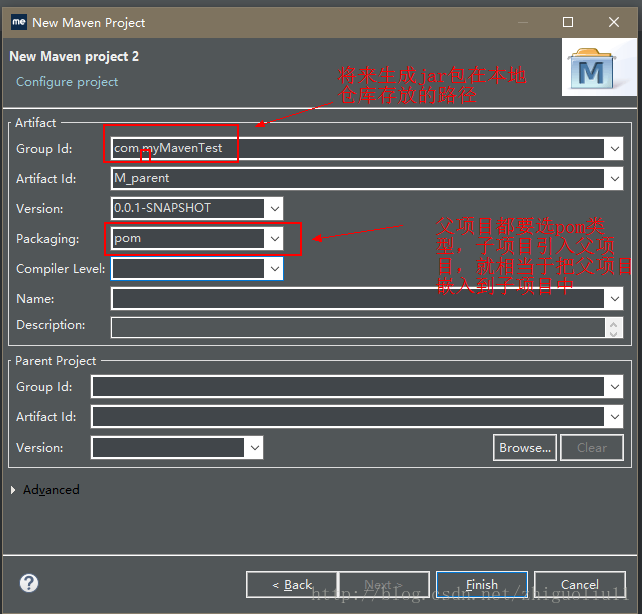
evaluateOrDie()
exit()
Exits PhantomJS with an optional exit status code.
退出PhantomJS时有一个可选退出状态码。
Note: You can not rely on the fact that your script will be turned off immediately, because this method works asynchronously. It means that your script may continue to be executed after the call of this method. More info here.
注意:你不能依赖你的脚步会被立即关闭,因为这个方式异步工作。这意味着exit()被调用时,你的脚步会继续执行。
获得Casper实例两种方式
var casper = require(‘casper’).create();
var casper = new require(‘casper’).Casper();
create()函数传参数
var casper = require(‘casper’).create({
clientScripts: [
‘includes/jquery.js’, // These two scripts will be injected in remote
‘includes/underscore.js’ // DOM on every request
],
pageSettings: {
loadImages: false, // The WebPage instance used by Casper will
loadPlugins: false // use these settings
},
logLevel: “info”, // Only “info” level messages will be logged
verbose: true // log messages will be printed out to the console
});
或者在运行时传递参数
var casper = require(‘casper’).create();
casper.options.waitTimeout = 1000;
更多参数请查看官方文档
back()
casper.start(‘http://foo.bar/1‘);
casper.thenOpen(‘http://foo.bar/2‘);
casper.thenOpen(‘http://foo.bar/3‘);
casper.back(); //回退页面函数
casper.run(function() {
console.log(this.getCurrentUrl()); // ‘http://foo.bar/2’ });
base64encode()
var base64logo = null; casper.start('http://www.google.fr/', function() { base64logo = this.base64encode('http://www.google.fr/images/srpr/logo3w.png'); }); casper.run(function() { this.echo(base64logo).exit(); });
post请求encode
var base64contents = null; casper.start('http://domain.tld/download.html', function() { base64contents = this.base64encode('http://domain.tld/', 'POST', { param1: 'foo', param2: 'bar' }); }); casper.run(function() { this.echo(base64contents).exit(); });
Selectors
<!doctype html> <html> <head> <meta charset="utf-8"> <title>My page</title> </head> <body> <h1 class="page-title">Hello</h1> <ul> <li>one</li> <li>two</li> <li>three</li> </ul> <footer><p>©2012 myself</p></footer> </body> </html>
检查
是否存在
Selectors-CSS3
var casper = require('casper').create(); casper.start('http://domain.tld/page.html', function() { if (this.exists('h1.page-title')) { this.echo('the heading exists'); } }); casper.run();
使用测试框架
casper.test.begin('The heading exists', 1, function suite(test) { casper.start('http://domain.tld/page.html', function() { test.assertExists('h1.page-title'); }).run(function() { test.done(); }); });
Selectors-Xpath
casper.start('http://domain.tld/page.html', function() { this.test.assertExists({ type: 'xpath', path: '//h1[@class="page-title"]' }, 'the element exists'); });
建议下面的用法
var x = require('casper').selectXPath; casper.start('http://domain.tld/page.html', function() { this.test.assertExists(x('//h1[@class="page-title"]'), 'the element exists'); });
Warning
The only limitation of XPath use in CasperJS is in the casper.fill() method when you want to fill file fields; PhantomJS natively only allows the use of CSS3 selectors in its uploadFile method, hence this limitation.
click()
casper.start('http://google.fr/'); casper.thenEvaluate(function(term) { document.querySelector('input[name="q"]').setAttribute('value', term); document.querySelector('form[name="f"]').submit(); }, 'CasperJS'); casper.then(function() { // Click on 1st result link this.click('h3.r a'); }); casper.then(function() { // Click on 1st result link this.click('h3.r a',10,10); }); casper.then(function() { // Click on 1st result link this.click('h3.r a',"50%","50%"); }); casper.then(function() { console.log('clicked ok, new location is ' + this.getCurrentUrl()); }); casper.run();
Signature: click(String selector, [Number|String X, Number|String Y]) //selector支持CSS3和XPath
clickLabel()
…
capture()
casper.start('http://www.google.fr/', function() { this.capture('google.png', { top: 100, left: 100, width: 500, height: 400 }); }); casper.run();
casper.start('http://foo', function() { this.capture('foo', undefined, { format: 'jpg', quality: 75 }); });
captureBase64()
casper.start('http://google.com', function() { // selector capture console.log(this.captureBase64('png', '#lga')); // clipRect capture console.log(this.captureBase64('png', { top: 0, left: 0, width: 320, height: 200 })); // whole page capture console.log(this.captureBase64('png')); }); casper.run();
captureSelector()
casper.start('http://www.weather.com/', function() { this.captureSelector('weather.png', '#LookingAhead'); }); casper.run();
clear()
casper.start('http://www.google.fr/', function() { this.clear(); // javascript execution in this page has been stopped }); casper.then(function() { // ... }); casper.run();
clearCache()
casper.start('http://www.google.fr/', function() { this.clearCache(); // cleared the memory cache and replaced page object with newPage(). }); casper.then(function() { // ... }); casper.run();
clearMemoryCache()
casper.start('http://www.google.fr/', function() { this.clearMemoryCache(); // cleared the memory cache. }); casper.then(function() { // ... }); casper.run();
debugHTML()
var casper = require('casper').create(); casper.start('http://www.migelab.com/', function() { this.debugHTML();//输出html内容代码到console }); casper.run();
debugPage()
casper.start('http://www.migelab.com/', function() { this.debugPage();//输出文本内容到console }); casper.run();
die()
…
download()
casper.start('http://www.google.fr/', function() { var url = 'http://www.google.fr/intl/fr/about/corporate/company/'; this.download(url, 'google_company.html'); }); casper.run(function() { this.echo('Done.').exit(); });
each()
var links = [ 'http://google.com/', 'http://yahoo.com/', 'http://bing.com/' ]; casper.start().each(links, function(self, link) { self.thenOpen(link, function() { this.echo(this.getTitle()); }); }); casper.run();
eachThen()
var casper = require('casper').create(); var urls = ['http://google.com/', 'http://yahoo.com/']; casper.start().eachThen(urls, function(response) { this.thenOpen(response.data, function(response) { console.log('Opened', response.url); }); }); casper.run();
echo()
casper.start('http://www.google.fr/', function() { this.echo('Page title is: ' + this.evaluate(function() { return document.title; }), 'INFO'); // Will be printed in green on the console }); casper.run();
evaluate()
casper.evaluate(function(username, password) { document.querySelector('#username').value = username; document.querySelector('#password').value = password; document.querySelector('#submit').click(); }, 'sheldon.cooper', 'b4z1ng4');
evaluateOrDie()
casper.start('http://foo.bar/home', function() { this.evaluateOrDie(function() { return /logged in/.match(document.title); }, 'not authenticated'); }); casper.run();
exit()
Exits PhantomJS with an optional exit status code.
退出PhantomJS时有一个可选退出状态码。
Note: You can not rely on the fact that your script will be turned off immediately, because this method works asynchronously. It means that your script may continue to be executed after the call of this method. More info here.
注意:你不能依赖你的脚步会被立即关闭,因为这个方式异步工作。这意味着exit()被调用时,你的脚步会继续执行。
相关文章推荐
- [译文]casperjs的API-colorizer模块
- 第一篇 arcgis api for javascript 简单地图
- [译文]casperjs的API-mouse模块
- CasperJS API 第二篇
- Android设计和开发系列第一篇:Notifications通知(Develop—API Guides)
- API开发第一篇:关于session的APP服务端API开发
- 深入理解javascript选择器API系列第一篇——4种元素选择器
- 我翻译的第一篇ArcGIS JavaScript API帮助文档
- casperjs 的API-casper模块
- ElasticSearch查询 第一篇:搜索API
- [译文]casperjs 的API-casper模块
- JavaMail邮件简介及API概述第一篇
- thinkphp api入门,开发,第一篇
- CasperJS API 第三篇
- [译文]casperjs的API-clientutils模块
- 我的第一篇博客随笔:关于angularjs API的理解心得。angular.bind() angular.boostrap()
- 第一篇 - dingo/api - 3.路由设置
- [CasperJS] API--The casper module(译)
- 微信支付之JSAPI开发第一篇-基本概念
- API解读第一篇——建立连接的核心对象(JDBC)