meteor方法
2017-09-07 00:00
183 查看
1.Account
login(phoneNumber: string, code: string): Promise<void> { return new Promise<void>((resolve, reject) => { Accounts.verifyPhone(phoneNumber, code, (e: Error) => { if (e) { return reject(e); } resolve(); }); }); }
2.发布和订阅
1.在server端发布Meteor.publish('time', function() { ... });
2.在client端订阅
后面可以带参数或者控制器函数
Meteor.subscribe('time', id)
Meteor.subscribe('time', { onReady: function() { console.log('the server called this.ready()') }, onError: function() { console.log('error on the server') } });
客户端也要创建新的mongo实例,然后通过订阅后的服务器的数据会推送到该实例上,但是只存在于内存中,所有对数据库的操作必须通过服务器的mongo实例进行操作!
3.配合react事件函数取消订阅
componentWillUnmount() { this.props.handle.stop(); } export default createContainer(() => { return { time: Time.find().fetch(), handle: handle }; }, Timer)
const handle = Meteor.subscribe('time', { onReady: function() { console.log('the server called this.ready()') }, onError: function() { console.log('error on the server') }}); handle.stop() // will call onStop(callback) on the server. handle.ready() // returns true if the server called ready()
4.在服务器端写就绪和取消订阅的处理
利用this.ready() this.stop() this.onStop()
Meteor.publish('time', function() { let self = this; self.added('time', id, time); // notify if record is added to the collection time this.ready(); // notify that the initial dataset was sent self.onStop(function () { self.stop() console.log('stopped called') Meteor.clearInterval(interval); // clear the interval if the the client unsubscribed }); });
3.延迟函数
Meteor.setInterval(function() { newTime(); }, 1000); Meteor.clearInterval(interval);
4.数据库操作
默认可以直接在客户端对数据库进行操作,删除secure包后,只能调用服务器的方法对数据进行操作,而如果直接在客户端调用collection.insert()之类的方法,最终的结果只会存储在客户端的minimogo中1.定义方法
import { Meteor } from 'meteor/meteor'; Meteor.methods({ cartInsert: function(product) { CartCollection.insert({ 'title' : product.title, 'price' : product.price, 'inventory' : product.inventory, 'quantity': 1 });
2.然后在客户端使用
import { Meteor } from 'meteor/meteor'; export const addToCart = (product) => { Meteor.call('cartInsert', product); };
对于返回数值的可采用promise
cartTotal: function() { let total = CartCollection.aggregate([ { $project: {"priceByquantity":{ $multiply: [ "$price", "$quantity" ] } }}, { $group: { "_id": "null", "totalPrice": { $sum: "$priceByquantity" } } } ]); return total; }
用aggregate计算总价钱,$project来产生一个"priceByquantity"的新键, "_id": "null"表示把所有的加起来,"totalPrice"为赋值的对象,最终得到的值包裹在data中
export const getCartTotal = () => { return new Promise((resolve, reject) => { Meteor.call('cartTotal', (error, data) => { if (error) { reject(error) } if(!data[0]){ resolve(0) } resolve(data[0].totalPrice) }) }); };
使用promise来包裹,利用then还有catch来对其中的数据进行操作
getCartTotal().then(result => { self.setState({ totalPrice: result }) }).catch(error => { alert('error') });
由于promise是异步的,而container本身是异步的,其内部只能使用同步的方法,所以把getCartTotal()放在组件事件周期中使用
componentDidMount() componentWillReceiveProps()
只有初始化和props改变的时候
5.验证和模型
import { check } from 'meteor/check'; check('title' : product.title,string) check( product, CartCollection.simpleSchema())
不常用!!
meteor add aldeed:simple-schema
let CartSchema = new SimpleSchema({ _id: { type: String, optional: false }, price: { type: Number, decimal: true 设为false则为整数 }, quantity: { type: Number, defaultValue: 1, optional: true }, department: { type: String, optional: false } });
department是必须的,验证完模型后再insert
直接使用collection2会自动每次校正格式(meteor add aldeed:collection2)
CartCollection.attachSchema(CartSchema) check( product, CartCollection.simpleSchema());
//弃用 CartCollection.schema.validate(product); CartCollection.schema.clean(product); check( product, CartCollection.schema); //在每次插入前去掉product里面不必要的部分,使其规范化 CartCollection.insert({....})
6.用户登录
meteor add accounts-password allanning:roles
Accounts.creatUser({email:444,password:6665}) Meteor.loginWithPassword(email,password) Meteor.logout(fuc(err){}) Meteor.users.findOne() Meteor.user()--获取当前用户
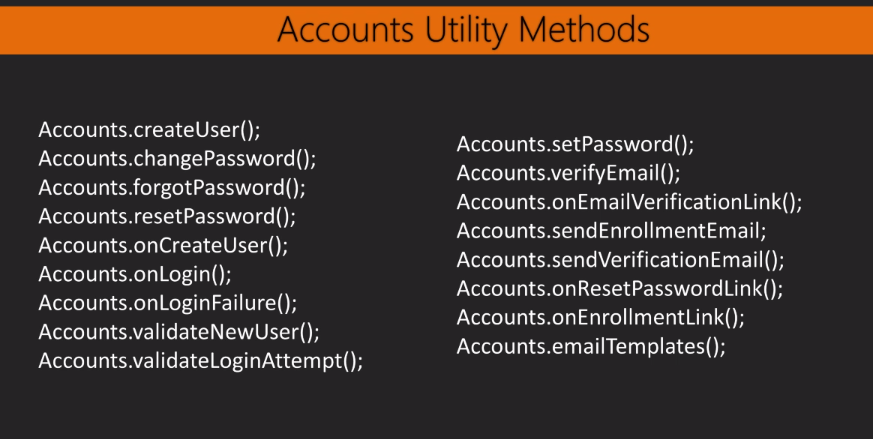
相关文章推荐
- meteor中异步回调的处理的方法
- meteor ---快速启动meteor和 mongodb 方法--MAC
- 手工给Meteor增加smart package的方法
- 将meteor部署在自己服务器上的简易方法
- Android or iOS 运行 meteor App 屏幕一片空白 White screen的解决方法
- 解决 meteor 频繁安装失败的方法
- 关于Meteor的microscope教程中《用户系统》遇到问题解决方法
- UVA 1398 - Meteor(排序+扫描方法+几何)
- PHP 学习笔记 - - - 简单方法的使用 (数组)
- maven国内镜像(maven下载慢的解决方法)
- Hibernate (七) session常用方法、懒加载、一级缓存
- 利用反射调用方法
- Java中常用的加密方法(JDK)
- c语言程序的存储区域与const关键字的使用方法
- 简单测试OPA627运放真伪的方法
- mysql5.5免安装版的配置方法
- viewpager中图片不能全屏显示的解决方法
- qt中域名解析的方法
- extjs中的JS代码在firefox可以正常运行,在IE中无法运行的方法。