React-Native 之 GD (十六)首页筛选功能
2017-09-01 22:10
387 查看
1.首页筛选功能
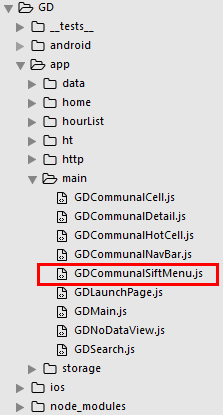
GDCommunalSiftMenu.js
/** * 筛选菜单 */ import React, { Component, PropTypes } from 'react'; import { StyleSheet, Text, View, Image, ListView, TouchableOpacity, Dimensions, Platform, } from 'react-native'; // 获取屏幕宽高 const {width, height} = Dimensions.get('window'); export default class GDCommunalSiftMenu extends Component { static defaultProps = { removeModal:{}, loadSiftData:{} }; static propTypes = { data:PropTypes.array, }; // 构造 constructor(props) { super(props); // 初始状态 this.state = { dataSource: new ListView.DataSource({rowHasChanged:(r1, r2) => r1 !== r2}) }; } // 退出 popToHome(data) { this.props.removeModal(data); } // 点击事件 siftData(mall, cate) { this.props.loadSiftData(mall, cate); this.popToHome(false); } // 处理数据 loadData() { let data = []; for (let i = 0; i<this.props.data.length; i++) { data.push(this.props.data[i]); } // 重新渲染 this.setState({ dataSource: this.state.dataSource.cloneWithRows(data), }) } renderRow(rowData) { return( <View style={styles.itemViewStyle}> <TouchableOpacity onPress={() => this.siftData(rowData.mall, rowData.cate)} > <View style={styles.itemViewStyle}> <Image source={{uri:rowData.image}} style={styles.itemImageStyle} /> <Text>{rowData.title}</Text> </View> </TouchableOpacity> </View> ) } componentDidMount() { this.loadData(); } render() { return( <TouchableOpacity onPress={() => this.popToHome(false)} activeOpacity={1} // 不透明 > <View style={styles.container}> {/* 菜单内容 */} <ListView dataSource={this.state.dataSource} renderRow={this.renderRow.bind(this)} contentContainerStyle={styles.contentViewStyle} // 内容样式 initialListSize={16} // 默认加载数据条数 /> </View> </TouchableOpacity> ) } } const styles = StyleSheet.create({ container: { width:width, height:height }, contentViewStyle: { flexDirection:'row', flexWrap:'wrap', width: width, top:Platform.OS === 'ios' ? 64 : 44, }, itemViewStyle: { width:width * 0.25, height:70, backgroundColor:'rgba(249,249,249,1.0)', justifyContent:'center', alignItems:'center' }, itemImageStyle: { width:40, height:40 } });
2.数据源:
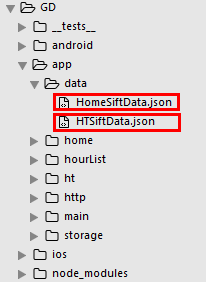
HomeSiftData.json
[ { "title" : "全部", "image" : "iconall_40x40", "mall" : "", "cate" : "" }, { "title" : "京东", "image" : "iconjd_40x40", "mall" : "京东商城", "cate" : "" }, { "title" : "亚马逊中国", "image" : "iconamazon_40x40", "mall" : "亚马逊中国", "cate" : "" }, { "title" : "天猫", "image" : "icontmall_40x40", "mall" : "天猫", "cate" : "" }, { "title" : "数码", "image" : "icondigital_40x40", "mall" : "", "cate" : "digital" }, { "title" : "母婴", "image" : "iconbaby_40x40", "mall" : "", "cate" : "baby" }, { "title" : "服装", "image" : "iconclothes_40x40", "mall" : "", "cate" : "clothes" }, { "title" : "食品", "image" : "iconfood_40x40", "mall" : "", "cate" : "food" }, { "title" : "旅行", "image" : "icontravel_40x40", "mall" : "", "cate" : "travel" }, { "title" : "家电", "image" : "iconelectrical_40x40", "mall" : "", "cate" : "electrical" }, { "title" : "日用", "image" : "icondaily_40x40", "mall" : "", "cate" : "daily" }, { "title" : "囤货", "image" : "iconstockup_40x40", "mall" : "", "cate" : "stockup" }, { "title" : "运动户外", "image" : "iconsport_40x40", "mall" : "", "cate" : "sport" }, { "title" : "美妆配饰", "image" : "iconmakeup_40x40", "mall" : "", "cate" : "makeup" }, { "title" : "汽车用品", "image" : "iconautomobile_40x40", "mall" : "", "cate" : "automobile" }, { "title" : "促销活动", "image" : "iconsale_40x40", "mall" : "", "cate" : "sale" } ]
HTSiftData.json
[ { "title" : "全部", "image" : "iconall_40x40", "mall" : "", "cate" : "" }, { "title" : "Amazon", "image" : "iconamazon_40x40", "mall" : "Amazon", "cate" : "" }, { "title" : "6pm", "image" : "icon6pm_40x40", "mall" : "6pm", "cate" : "" }, { "title" : "日本亚马逊", "image" : "iconamazon_40x40", "mall" : "日本亚马逊", "cate" : "" }, { "title" : "直邮中国", "image" : "iconzdirect_40x40", "mall" : "", "cate" : "zdirect" }, { "title" : "数码", "image" : "icondigital_40x40", "mall" : "", "cate" : "digital" }, { "title" : "母婴", "image" : "iconbaby_40x40", "mall" : "", "cate" : "baby" }, { "title" : "服装", "image" : "iconclothes_40x40", "mall" : "", "cate" : "clothes" }, { "title" : "食品", "image" : "iconfood_40x40", "mall" : "", "cate" : "food" }, { "title" : "家电", "image" : "iconelectrical_40x40", "mall" : "", "cate" : "electrical" }, { "title" : "日用", "image" : "icondaily_40x40", "mall" : "", "cate" : "daily" }, { "title" : "运动户外", "image" : "iconsport_40x40", "mall" : "", "cate" : "sport" } ]
3.调用
GDHome.js
/** * 首页 */ import React, { Component } from 'react'; import { StyleSheet, Text, View, TouchableOpacity, Image, ListView, Dimensions, ActivityIndicator, Modal, // 模态 AsyncStorage, // 缓存数据库(数据持久化) } from 'react-native'; // 引入 下拉刷新组件 import {PullList} from 'react-native-pull'; // 导航器 import CustomerComponents, { Navigator } from 'react-native-deprecated-custom-components'; // 获取屏幕宽高 const {width, height} = Dimensions.get('window'); // 引入自定义导航栏组件 import CommunalNavBar from '../main/GDCommunalNavBar'; // 引入 公共cell import CommunalCell from '../main/GDCommunalCell'; // 引入 详情页 组件 import CommunalDetail from '../main/GDCommunalDetail'; // 引入 筛选菜单组件 import CommunalSiftMenu from '../main/GDCommunalSiftMenu'; // 引入 近半小时热门组件 import HalfHourHot from './GDHalfHourHot'; // 引入 搜索页面组件 import Search from '../main/GDSearch'; // 引入 空白页组件 import NoDataView from '../main/GDNoDataView'; // 数据 筛选菜单 import HomeSiftData from '../data/HomeSiftData.json'; export default class GDHome extends Component { // 构造 constructor(props) { super(props); // 初始状态 this.state = { dataSource: new ListView.DataSource({rowHasChanged:(r1, r2) => r1 !== r2}), // 数据源 优化 loaded: false, // 用于判断是否显示空白页 isHalfHourHotModal: false, // 用于判断模态的可见性 isSiftModal: false, // 筛选功能 }; // 全局定义一个空数组用于存储列表数据 this.data = []; // 绑定 this.loadData = this.loadData.bind(this); this.loadMore = this.loadMore.bind(this); } // 网络请求 loadData(resolve) { let params = {"count" : 10 }; HTTPBase.get('https://guangdiu.com/api/getlist.php', params) .then((responseData) => { // 情况数组(刷新时) this.data = []; // 拼接数据 this.data = this.data.concat(responseData.data); // 重新渲染 this.setState({ dataSource: this.state.dataSource.cloneWithRows(this.data), loaded:true, }); // 关闭刷新动画 if (resolve !== undefined){ setTimeout(() => { resolve(); }, 1000); } // 存储数组中最后一个元素的id let cnlastID = responseData.data[responseData.data.length - 1].id; AsyncStorage.setItem('cnlastID', cnlastID.toString()); // 只能存储字符或字符串 // 首页存储数组中第一个元素的id let cnfirstID = responseData.data[0].id; AsyncStorage.setItem('cnfirstID', cnfirstID.toString()); // // 清除本地存储的数据 // RealmBase.removeAllData('HomeData'); // // 存储数据到本地 // RealmBase.create('HomeData', responseData.data); // 向数据表存储数据 }) .catch((error) => { // // 数据加载失败,拿到本地存储的数据,展示出来,如果没有存储,那就显示无数据页面 // this.data = RealmBase.loadAll('HomeData'); // 从数据表提取数据 // // 重新渲染 // this.setState({ // dataSource: this.state.dataSource.cloneWithRows(this.data), // loaded:true, // }); }) } // 接收 筛选菜单的参数,进行网络请求 loadSiftData(mall, cate) { let params = {}; if(mall === "" && cate === ""){ // 全部 this.loadData(undefined); return; } if(mall === ""){ // cate 有值 params = { "cate" : cate }; }else{ params = { "mall" : mall }; } HTTPBase.get('https://guangdiu.com/api/getlist.php', params) .then((responseData) => { // 情况数组(刷新时) this.data = []; // 拼接数据 this.data = this.data.concat(responseData.data); // 重新渲染 this.setState({ dataSource: this.state.dataSource.cloneWithRows(this.data), loaded:true, }); // 存储数组中最后一个元素的id let cnlastID = responseData.data[responseData.data.length - 1].id; AsyncStorage.setItem('cnlastID', cnlastID.toString()); // 只能存储字符或字符串 }) .catch((error) => { }) } // 加载更多数据的网络请求 loadMoreData(value) { let params = { "count" : 10, "sinceid" : value }; HTTPBase.get('https://guangdiu.com/api/getlist.php', params) .then((responseData) => { // 拼接数据 this.data = this.data.concat(responseData.data); // 重新渲染 this.setState({ dataSource: this.state.dataSource.cloneWithRows(this.data), loaded:true, }); // 存储数组中最后一个元素的id let cnlastID = responseData.data[responseData.data.length - 1].id; AsyncStorage.setItem('cnlastID', cnlastID.toString()); // 只能存储字符或字符串 }) .catch((error) => { }) } // 加载更多数据操作 loadMore() { // 读取存储的id AsyncStorage.getItem('cnlastID') .then((value) => { // 数据加载操作 this.loadMoreData(value); }) } // 模态到近半小时热门 pushToHalfHourHot() { this.setState({ isHalfHourHotModal: true }) } // 跳转到搜索页面 pushToSearch() { this.props.navigator.push({ component: Search, }) } // 安卓模态销毁模态 onRequestClose() { this.setState({ isHalfHourHotModal:false, isSiftModal:false, }) } // 关闭模态 closeModal(data) { this.setState({ isHalfHourHotModal:data, isSiftModal:data, }) } // 显示筛选菜单 showSiftMenu() { this.setState({ isSiftModal:true, }) } // 返回左边按钮 renderLeftItem() { // 将组件返回出去 return( <TouchableOpacity onPress={() => {this.pushToHalfHourHot()}} > <Image source={{uri:'hot_icon_20x20'}} style={styles.navbarLeftItemStyle} /> </TouchableOpacity> ); } // 返回中间按钮 renderTitleItem() { return( <TouchableOpacity // 显示或隐藏筛选菜单 onPress={() => {this.showSiftMenu()}} > <Image source={{uri:'navtitle_home_down_66x20'}} style={styles.navbarTitleItemStyle} /> </TouchableOpacity> ); } // 返回右边按钮 renderRightItem() { return( <TouchableOpacity // 跳转搜索页面 onPress={() => {this.pushToSearch()}} > <Image source={{uri:'search_icon_20x20'}} style={styles.navbarRightItemStyle} /> </TouchableOpacity> ); } // ListView尾部 renderFooter() { return ( <View style={{height: 100}}> <ActivityIndicator /> </View> ); } // 根据网络状态决定是否渲染 listView renderListView() { if(this.state.loaded === false) { // 显示空白页 return( <NoDataView /> ); }else{ return( <PullList // 将ListView 改为 PullList // 下拉刷新 onPullRelease={(resolve) => this.loadData(resolve)} // 数据源 通过判断dataSource是否有变化,来判断是否要重新渲染 dataSource={this.state.dataSource} renderRow={this.renderRow.bind(this)} // 隐藏水平线 showsHorizontalScrollIndicator={false} style={styles.listViewStyle} initialListSize={7} // 默认渲染数据条数 // 返回 listView 头部 renderHeader={this.renderHeader} // 上拉加载更多 onEndReached={this.loadMore} onEndReachedThreshold={60} renderFooter={this.renderFooter} /> ); } } // 通过id 跳转详情页 pushToDetail(value) { this.props.navigator.push({ component:CommunalDetail, params: { url: 'https://guangdiu.com/api/showdetail.php' + '?' + 'id=' + value } }) } // 返回每一行cell的样式 renderRow(rowData) { // 使用cell组件 return( <TouchableOpacity // 给每一个cell添加点击事件 onPress={() => this.pushToDetail(rowData.id)} > <CommunalCell image={rowData.image} title={rowData.title} mall={rowData.mall} // 平台 pubTime={rowData.pubtime} // 时间 fromSite={rowData.fromsite} // 来源 /> </TouchableOpacity> ); } // 生命周期 组件渲染完成 已经出现在dom文档里 componentDidMount() { // 请求数据 this.loadData(); } render() { return ( <View style={styles.container}> {/* 初始化近半小时热门模态 */} <Modal animationType='slide' // 动画 底部弹窗 transparent={false} // 透明度 visible={this.state.isHalfHourHotModal} // 可见性 onRequestClose={() => this.onRequestClose()} // 销毁 > <Navigator initialRoute={{ name:'halfHourHot', component:HalfHourHot }} renderScene={(route, navigator) => { let Component = route.component; return <Component removeModal={(data) => this.closeModal(data)} {...route.params} navigator={navigator} /> }} /> </Modal> {/* 初始化筛选菜单模态 */} <Modal animationType='none' // 无动画 transparent={true} // 为透明状态 visible={this.state.isSiftModal} // 可见性 onRequestClose={() => this.onRequestClose()} // 销毁 > <CommunalSiftMenu removeModal={(data) => this.closeModal(data)} data={HomeSiftData} loadSiftData={(mall, cate) => this.loadSiftData(mall, cate)} /> </Modal> {/* 导航栏样式 */} <CommunalNavBar leftItem = {() => this.renderLeftItem()} titleItem = {() => this.renderTitleItem()} rightItem = {() => this.renderRightItem()} /> {/* 根据网络状态决定是否渲染 listView */} {this.renderListView()} </View> ); } } const styles = StyleSheet.create({ container: { flex: 1, alignItems: 'center', }, navbarLeftItemStyle: { width:20, height:20, marginLeft:15, }, navbarTitleItemStyle: { width:66, height:20, }, navbarRightItemStyle: { width:20, height:20, marginRight:15, }, listViewStyle: { width:width, }, });
效果图:
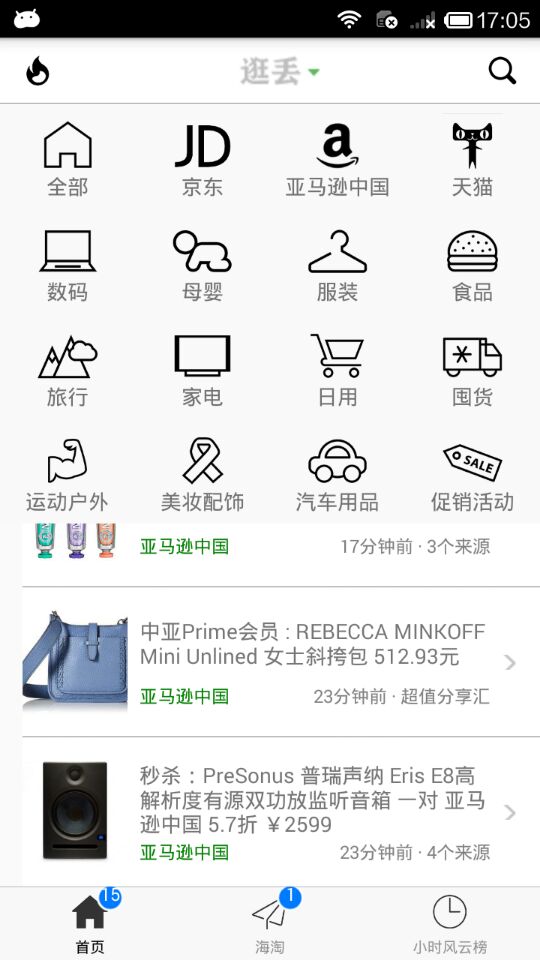
GDHt.js
/** * 海淘折扣 */ import React, { Component } from 'react'; import { StyleSheet, Text, View, TouchableOpacity, Image, ListView, Dimensions, ActivityIndicator, Modal, // 模态 AsyncStorage, // 缓存数据库(数据持久化) } from 'react-native'; // 引入 下拉刷新组件 import {PullList} from 'react-native-pull'; // 导航器 import CustomerComponents, { Navigator } from 'react-native-deprecated-custom-components'; // 获取屏幕宽高 const {width, height} = Dimensions.get('window'); // 引入自定义导航栏组件 import CommunalNavBar from '../main/GDCommunalNavBar'; // 引入 cell import CommunalCell from '../main/GDCommunalCell'; // 引入 详情页 组件 import CommunalDetail from '../main/GDCommunalDetail'; // 引入 筛选菜单组件 import CommunalSiftMenu from '../main/GDCommunalSiftMenu'; // 引入 近半小时热门组件 import USHalfHourHot from './GDUSHalfHourHot'; // 引入 搜索页面组件 import Search from '../main/GDSearch'; // 引入 空白页组件 import NoDataView from '../main/GDNoDataView'; // 数据 筛选菜单 import HTSiftData from '../data/HTSiftData.json'; export default class GDHome extends Component { // 构造 constructor(props) { super(props); // 初始状态 this.state = { dataSource: new ListView.DataSource({rowHasChanged:(r1, r2) => r1 !== r2}), // 数据源 优化 loaded: false, // 用于判断是否显示空白页 isUSHalfHourHotModal: false, // 用于判断模态的可见性 isSiftModal: false, // 筛选功能 }; // 全局定义一个空数组用于存储列表数据 this.data = []; // 绑定 this.loadData = this.loadData.bind(this); this.loadMore = this.loadMore.bind(this); } // 加载最新数据网络请求 loadData(resolve) { let params = { "count" : 10, "country" : "us" }; HTTPBase.get('https://guangdiu.com/api/getlist.php', params) .then((responseData) => { // 清空数组(刷新时) this.data = []; // 拼接数据 this.data = this.data.concat(responseData.data); // 重新渲染 this.setState({ dataSource: this.state.dataSource.cloneWithRows(this.data), loaded:true, }); // 关闭刷新动画 if (resolve !== undefined){ setTimeout(() => { resolve(); }, 1000); } // 存储数组中最后一个元素的id let uslastID = responseData.data[responseData.data.length - 1].id; AsyncStorage.setItem('uslastID', uslastID.toString()); // 只能存储字符或字符串 // 首页存储数组中第一个元素的id let usfirstID = responseData.data[0].id; AsyncStorage.setItem('usfirstID', usfirstID.toString()); // // 清除本地存储的数据 // RealmBase.removeAllData('HomeData'); // // 存储数据到本地 // RealmBase.create('HomeData', responseData.data); // 向数据表存储数据 }) .catch((error) => { // // 数据加载失败,拿到本地存储的数据,展示出来,如果没有存储,那就显示无数据页面 // this.data = RealmBase.loadAll('HomeData'); // 从数据表提取数据 // // 重新渲染 // this.setState({ // dataSource: this.state.dataSource.cloneWithRows(this.data), // loaded:true, // }); }) } // 接收 筛选菜单的参数,进行网络请求 loadSiftData(mall, cate) { let params = {}; if(mall === "" && cate === ""){ // 全部 this.loadData(undefined); return; } if(mall === ""){ // cate 有值 params = { "cate" : cate, "country" : "us" }; }else{ params = { "mall" : mall, "country" : "us" }; } HTTPBase.get('https://guangdiu.com/api/getlist.php', params) .then((responseData) => { // 情况数组(刷新时) this.data = []; // 拼接数据 this.data = this.data.concat(responseData.data); // 重新渲染 this.setState({ dataSource: this.state.dataSource.cloneWithRows(this.data), loaded:true, }); // 存储数组中最后一个元素的id let cnlastID = responseData.data[responseData.data.length - 1].id; AsyncStorage.setItem('cnlastID', cnlastID.toString()); // 只能存储字符或字符串 }) .catch((error) => { }) } // 加载更多数据的网络请求 loadMoreData(value) { let params = { "count" : 10, "country" : "us", "sinceid" : value }; HTTPBase.get('https://guangdiu.com/api/getlist.php', params) .then((responseData) => { // 拼接数据 this.data = this.data.concat(responseData.data); // 重新渲染 this.setState({ dataSource: this.state.dataSource.cloneWithRows(this.data), loaded:true, }); // 存储数组中最后一个元素的id let uslastID = responseData.data[responseData.data.length - 1].id; AsyncStorage.setItem('uslastID', uslastID.toString()); // 只能存储字符或字符串 }) .catch((error) => { }) } // 加载更多数据操作 loadMore() { // 读取存储的id AsyncStorage.getItem('uslastID') .then((value) => { // 数据加载操作 this.loadMoreData(value); }) } // 模态到近半小时热门 pushToHalfHourHot() { this.setState({ isUSHalfHourHotModal: true }) } // 显示筛选菜单 showSiftMenu() { this.setState({ isSiftModal:true, }) } // 跳转到搜索页面 pushToSearch() { this.props.navigator.push({ component: Search, }) } // 安卓模态销毁模态 onRequestClose() { this.setState({ isUSHalfHourHotModal:false, isSiftModal:false }) } // 关闭模态 closeModal(data) { this.setState({ isUSHalfHourHotModal:data, isSiftModal:data }) } // 返回左边按钮 renderLeftItem() { // 将组件返回出去 return( <TouchableOpacity onPress={() => {this.pushToHalfHourHot()}} > <Image source={{uri:'hot_icon_20x20'}} style={styles.navbarLeftItemStyle} /> </TouchableOpacity> ); } // 返回中间按钮 renderTitleItem() { return( <TouchableOpacity // 显示或隐藏筛选菜单 onPress={() => {this.showSiftMenu()}} > <Image source={{uri:'navtitle_home_down_66x20'}} style={styles.navbarTitleItemStyle} /> </TouchableOpacity> ); } // 返回右边按钮 renderRightItem() { return( <TouchableOpacity // 跳转搜索页面 onPress={() => {this.pushToSearch()}} > <Image source={{uri:'search_icon_20x20'}} style={styles.navbarRightItemStyle} /> </TouchableOpacity> ); } // ListView尾部 renderFooter() { return ( <View style={{height: 100}}> <ActivityIndicator /> </View> ); } // 根据网络状态决定是否渲染 listView renderListView() { if(this.state.loaded === false) { // 显示空白页 return( <NoDataView /> ); }else{ return( <PullList // 将ListView 改为 PullList // 下拉刷新 onPullRelease={(resolve) => this.loadData(resolve)} // 数据源 通过判断dataSource是否有变化,来判断是否要重新渲染 dataSource={this.state.dataSource} renderRow={this.renderRow.bind(this)} // 隐藏水平线 showsHorizontalScrollIndicator={false} style={styles.listViewStyle} initialListSize={7} // 返回 listView 头部 renderHeader={this.renderHeader} // 上拉加载更多 onEndReached={this.loadMore} onEndReachedThreshold={60} renderFooter={this.renderFooter} /> ); } } // 通过id 跳转详情页 pushToDetail(value) { this.props.navigator.push({ component:CommunalDetail, params: { url: 'https://guangdiu.com/api/showdetail.php' + '?' + 'id=' + value } }) } // 返回每一行cell的样式 renderRow(rowData) { // 使用cell组件 return( <TouchableOpacity // 给每一个cell添加点击事件 onPress={() => this.pushToDetail(rowData.id)} > <CommunalCell image={rowData.image} title={rowData.title} mall={rowData.mall} // 平台 pubTime={rowData.pubtime} // 时间 fromSite={rowData.fromsite} // 来源 /> </TouchableOpacity> ); } // 生命周期 组件渲染完成 已经出现在dom文档里 componentDidMount() { // 请求数据 this.loadData(); } render() { return ( <View style={styles.container}> {/* 初始化近半小时热门模态 */} <Modal animationType='slide' // 动画 底部弹窗 transparent={false} // 透明度 visible={this.state.isUSHalfHourHotModal} // 可见性 onRequestClose={() => this.onRequestClose()} // 销毁 > <Navigator initialRoute={{ name:'ushalfHourHot', component:USHalfHourHot }} renderScene={(route, navigator) => { let Component = route.component; return <Component removeModal={(data) => this.closeModal(data)} {...route.params} navigator={navigator} /> }} /> </Modal> {/* 初始化筛选菜单模态 */} <Modal animationType='none' // 无动画 transparent={true} // 为透明状态 visible={this.state.isSiftModal} // 可见性 onRequestClose={() => this.onRequestClose()} // 销毁 > <CommunalSiftMenu removeModal={(data) => this.closeModal(data)} data={HTSiftData} loadSiftData={(mall, cate) => this.loadSiftData(mall, cate)} /> </Modal> {/* 导航栏样式 */} <CommunalNavBar leftItem = {() => this.renderLeftItem()} titleItem = {() => this.renderTitleItem()} rightItem = {() => this.renderRightItem()} /> {/* 根据网络状态决定是否渲染 listView */} {this.renderListView()} </View> ); } } const styles = StyleSheet.create({ container: { flex: 1, alignItems: 'center', }, navbarLeftItemStyle: { width:20, height:20, marginLeft:15, }, navbarTitleItemStyle: { width:66, height:20, }, navbarRightItemStyle: { width:20, height:20, marginRight:15, }, listViewStyle: { width:width, }, });
效果图:
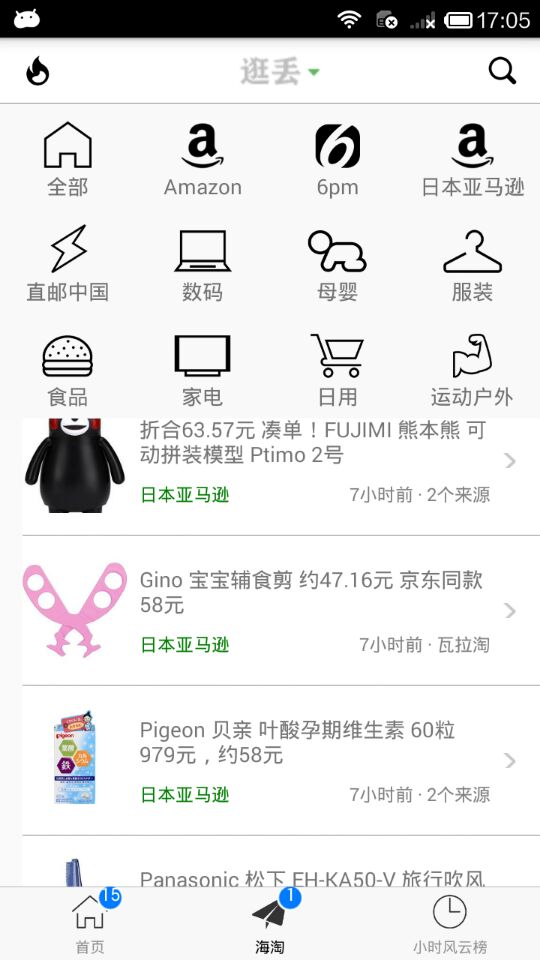
.
相关文章推荐
- React-Native 之 GD (十九)TabBarItem 逻辑完善 / 关闭筛选菜单滑动手势 / Navigator 掉帧卡顿问题处理
- React-Native 之 GD (十一)加载更多功能完善 及 跳转详情页
- React-Native 之 GD (十二)海淘半小时热门 及 获取最新数据个数功能 (角标)
- React-Native 之 GD (十八)监听 TabBarItem 点击与传值实现 点击 Item 进行刷新功能
- React-Native 之 GD (十四)小时风云榜 及 当前时间操作 及 上一小时、下一小时功能实现
- React Native布局实践:开发京东客户端首页(四)——首页功能按钮及控件封装
- (十六)ReactNative 中获取当前文件的相对路径和相对路径
- ReactNative ListView基础功能
- 用 `react native` 模仿做的一个美团客户端首页
- ReactNative系列之十六listview中子元素变更checkbox
- React Native Android 使用友盟SDK功能
- [置顶] 简析 React Native 用户反馈功能实现
- 小码哥-React-Native初体验三(window下搭建应用的首页)
- React-Native 之 GD (九)POST 网络请求封装
- React-Native 之 GD (二十)removeClippedSubviews / modal放置的顺序 / Android 加载git图\动图 / 去除 Android 中输入框的下划线 / navigationBar
- react-native 常用功能库几 4000 个人总结比较好的网站
- React Native商城项目实战13 - 首页中间上部分内容
- React-native 中的触摸响应功能
- React-Native 工程添加推送功能 (iOS 篇)
- react-native 新闻标签排序编辑功能