java tcp网络通信 传输文件
2017-08-31 23:27
686 查看
初学java 实现tcp网络通信 界面使用windowBuilder,界面如下:
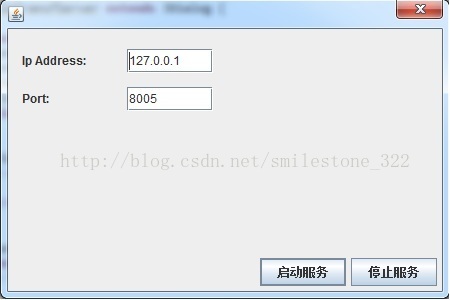
首先服务端监听端口号8005,启动服务后,等待客户端连接。
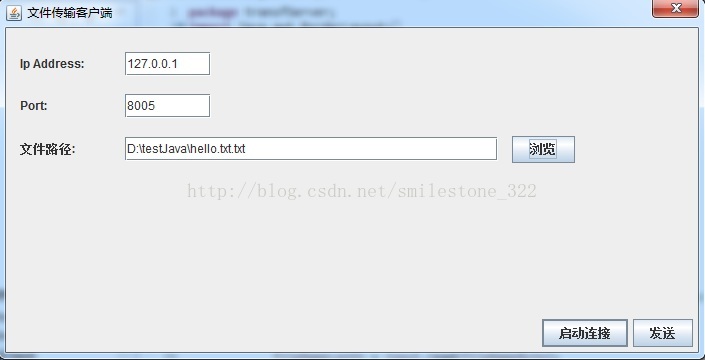
客户端首先选择需要传输的文件,然后启动连接,就开始传输文件了。
服务端的源码如下:
Thread.sleep(100);
客户端的源码如下:
首先服务端监听端口号8005,启动服务后,等待客户端连接。
客户端首先选择需要传输的文件,然后启动连接,就开始传输文件了。
服务端的源码如下:
package transfServer; import java.awt.BorderLayout; import java.awt.FlowLayout; import javax.swing.JButton; import javax.swing.JDialog; import javax.swing.JPanel; import javax.swing.border.EmptyBorder; import java.awt.event.ActionListener; import java.io.BufferedOutputStream; import java.io.File; import java.io.FileOutputStream; import java.io.InputStream; import java.net.*; import java.awt.event.ActionEvent; import javax.swing.JLabel; import javax.swing.JTextField; public class FileTransfServer extends JDialog { private final JPanel contentPanel = new JPanel(); private JTextField ipAddress; private JTextField Port; private Socket socket; //accept 后sock private String filePath="E:\\testJava"; private boolean bStartFlag=false; //IP地址 private String strIp=""; private int nPort=0; public void ReadData(Socket sock) { try{ InputStream input = sock.getInputStream(); //打开 inputStream资源 byte[] fileNameByte = new byte[1024]; //存放fileName int fileNameLenth; //读取的字符长度 fileNameLenth = input.read(fileNameByte); String fileName = new String(fileNameByte,0,fileNameLenth); //还原fileName System.out.println(Thread.currentThread().getName() + fileName); //下面是接受文件 BufferedOutputStream output = new BufferedOutputStream(new FileOutputStream(new File(filePath, fileName))); byte[] buff = new byte[1024*1024*5]; int len; while ( (len = input.read(buff))!=-1){ output.write(buff, 0, len); } System.out.println("receive finished!"); output.close(); sock.close(); }catch(Exception e){ System.out.println(e); } } /** * Launch the application. */ public static void main(String[] args) { try { FileTransfServer dialog = new FileTransfServer(); dialog.setDefaultCloseOperation(JDialog.DISPOSE_ON_CLOSE); dialog.setVisible(true); } catch (Exception e) { e.printStackTrace(); } } /** * Create the dialog. */ public FileTransfServer() { setBounds(100, 100, 450, 300); getContentPane().setLayout(new BorderLayout()); contentPanel.setBorder(new EmptyBorder(5, 5, 5, 5)); getContentPane().add(contentPanel, BorderLayout.CENTER); contentPanel.setLayout(null); JLabel lblIpAddress = new JLabel("Ip Address:"); lblIpAddress.setBounds(14, 23, 91, 18); contentPanel.add(lblIpAddress); { JLabel lblPort = new JLabel("Port:"); lblPort.setBounds(14, 61, 91, 18); contentPanel.add(lblPort); } //ip地址 ipAddress = new JTextField("127.0.0.1"); ipAddress.setBounds(119, 20, 86, 24); contentPanel.add(ipAddress); ipAddress.setColumns(10); //端口号 Port = new JTextField("8005"); Port.setColumns(10); Port.setBounds(119, 58, 86, 24); contentPanel.add(Port); { JPanel buttonPane = new JPanel(); buttonPane.setLayout(new FlowLayout(FlowLayout.RIGHT)); getContentPane().add(buttonPane, BorderLayout.SOUTH); { JButton btnStartServer = new JButton("启动服务"); btnStartServer.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent arg0) { //启动服务添加的代码 //获取ip地址和端口号 strIp=ipAddress.getText(); //服务器IP,此处没有用到 String strPort=Port.getText(); //String to int nPort=Integer.parseInt(strPort); //服务器端口号 //启动服务函数 try{ ServerSocket server = new ServerSocket(nPort); bStartFlag=true; while(bStartFlag) { Socket socket = server.accept(); ReadData(socket);server.close();
Thread.sleep(100);
} }catch(Exception e) { System.out.println(e); } } }); btnStartServer.setActionCommand("StartServer"); buttonPane.add(btnStartServer); getRootPane().setDefaultButton(btnStartServer); } { JButton cancelButton = new JButton("停止服务"); cancelButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { //添加取消命令的代码 bStartFlag=false; } }); cancelButton.setActionCommand("Cancel"); buttonPane.add(cancelButton); } } } }
客户端的源码如下:
package transfClient; import java.awt.BorderLayout; import java.awt.FlowLayout; import javax.swing.JButton; import javax.swing.JDialog; import javax.swing.JPanel; import javax.swing.border.EmptyBorder; import javax.swing.JLabel; import javax.swing.JTextField; import java.awt.event.ActionListener; import java.io.BufferedInputStream; import java.io.File; import java.io.FileInputStream; import java.io.IOException; import java.io.OutputStream; import java.awt.event.ActionEvent; import javax.swing.JFileChooser; import javax.swing.filechooser.FileNameExtensionFilter; import java.io.File; import java.net.*; public class FileTransfClient extends JDialog { private final JPanel contentPanel = new JPanel(); private JTextField IpAddress; private JTextField Port; private JTextField FileDir; private String filePath=""; private String strIp=""; private int nPort=0; //发送文件代码 public void SendFile(String strIp,int nPort) { // try { //不处理异常编译不过 InetAddress address=InetAddress.getByName(strIp); Socket servSock=new Socket(address,nPort); File file = new File (filePath); String fileName = file.getName(); OutputStream out = servSock.getOutputStream(); //传输文件名 out.write(fileName.getBytes()); BufferedInputStream input = new BufferedInputStream(new FileInputStream(file)); byte[] buff = new byte[1024*1024]; int len; while (( len=input.read(buff) )!=-1){ out.write(buff,0,len); } servSock.close(); }catch(Exception e) { System.out.println(e); } } /** * Launch the application. */ public static void main(String[] args) { try { FileTransfClient dialog = new FileTransfClient(); dialog.setDefaultCloseOperation(JDialog.DISPOSE_ON_CLOSE); dialog.setTitle("文件传输客户端"); dialog.setVisible(true); } catch (Exception e) { e.printStackTrace(); } } /** * Create the dialog. */ public FileTransfClient() { setBounds(100, 100, 708, 362); getContentPane().setLayout(new BorderLayout()); contentPanel.setBorder(new EmptyBorder(5, 5, 5, 5)); getContentPane().add(contentPanel, BorderLayout.CENTER); contentPanel.setLayout(null); { JLabel lblNewLabel = new JLabel("Ip Address:"); lblNewLabel.setBounds(14, 27, 91, 18); contentPanel.add(lblNewLabel); } { JLabel lblPort = new JLabel("Port:"); lblPort.setBounds(14, 69, 91, 18); contentPanel.add(lblPort); } { //IP 地址 IpAddress = new JTextField("127.0.0.1"); IpAddress.setBounds(119, 24, 86, 24); contentPanel.add(IpAddress); IpAddress.setColumns(10); } { //端口号 Port = new JTextField("8005"); Port.setColumns(10); Port.setBounds(119, 66, 86, 24); contentPanel.add(Port); } { JLabel label = new JLabel("文件路径:"); label.setBounds(14, 112, 91, 18); contentPanel.add(label); } { FileDir = new JTextField(""); FileDir.setColumns(10); FileDir.setBounds(119, 109, 373, 24); contentPanel.add(FileDir); } JButton browseBtn = new JButton("浏览"); browseBtn.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { //浏览Btn消息相应函数 JFileChooser jf=new JFileChooser(); jf.setDialogTitle("请选择需要发送的文件"); FileNameExtensionFilter filter=new FileNameExtensionFilter("文本文件(*.txt;*.kcd)","txt","kcd"); jf.setFileFilter(filter); int returnVal=jf.showOpenDialog(null); if(returnVal ==JFileChooser.APPROVE_OPTION) { filePath=jf.getSelectedFile().getPath(); FileDir.setText(filePath); } } }); browseBtn.setActionCommand("Browse"); browseBtn.setBounds(506, 108, 63, 27); contentPanel.add(browseBtn); { JPanel buttonPane = new JPanel(); buttonPane.setLayout(new FlowLayout(FlowLayout.RIGHT)); getContentPane().add(buttonPane, BorderLayout.SOUTH); { JButton StartConnectBtn = new JButton("启动连接"); StartConnectBtn.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { //连接服务器所需代码 //获取ip地址和端口号 strIp=IpAddress.getText(); //服务器IP String strPort=Port.getText(); //String to int nPort=Integer.parseInt(strPort); //服务器端口号 //传输文件 SendFile(strIp,nPort); } }); StartConnectBtn.setActionCommand("StartConnect"); buttonPane.add(StartConnectBtn); getRootPane().setDefaultButton(StartConnectBtn); } { JButton SendBtn = new JButton("发送"); SendBtn.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { //往服务器发送文件所需代码 } }); SendBtn.setActionCommand("Send"); buttonPane.add(SendBtn); } } } }
相关文章推荐
- java tcp 网络通信--使用多线程传输文件
- Java基础—网络编程【OSI/RM TCP/IP】【网络通信三要素】【UDP传输 & TCP传输】【DNS域名解析】
- Java基础—网络编程【OSI/RM TCP/IP】【网络通信三要素】【UDP传输 & TCP传输】【DNS域名解析】
- Java基础—网络编程【OSI/RM TCP/IP】【网络通信三要素】【UDP传输 & TCP传输】【DNS域名解析】
- java 网络编程之TCP通信和简单的文件上传功能
- java 网络编程之TCP通信和简单的文件上传功能实例
- Java 使用 TCP 和 UDP 传输文件
- JAVA 使用网络传输文件 并且使用原来的文件名称(服务端改进版)
- win32 TCP网络文件传输服务器端1.23
- win32 TCP网络文件传输服务器端1.23
- 黑马程序员 Java网络传输UDP和TCP协议
- (转)Java 网络文件传输
- Android学习笔记---28_网络通信之通过HTTP协议实现文件上传,组拼http 的post方法,传输内容
- Java中的TCP/UDP网络通信编程
- Java 使用 TCP 和 UDP 传输文件
- java 网络编程 [网络传输] [协议] [UDP与TCP] [套接字] [URL与URI]
- Qt与Java实现Tcp网络通信,收发简单字符串。
- Java网络编程之TCP协议下—上传文件到服务器程序
- java socket通信-传输文件图片
- Java 网络文件传输