Android初级开发(九)——网络交互—HttpURLConnection
2017-08-28 11:11
281 查看
一、使用HttpURLConnection的步骤
1、获取到HttpURLConnection的实例,并传入目标的网络地址,然后调用openConnection()方法
2、设置HTTP请求所使用的方法GET和POST
GET:从服务器那里获取数据
之后再调用getInputStream()方法获取到服务器返回的输入流
POST:提交数据给服务器
每条数据都要以键值对的形式存在,数据与数据之间用“&”符号隔开
3、调用disconnect()方法将HTTP连接关闭掉
二、实例
需求:一个按钮用于发送HTTP请求,一个文本框用于将服务器返回的数据显示出来
1、效果图
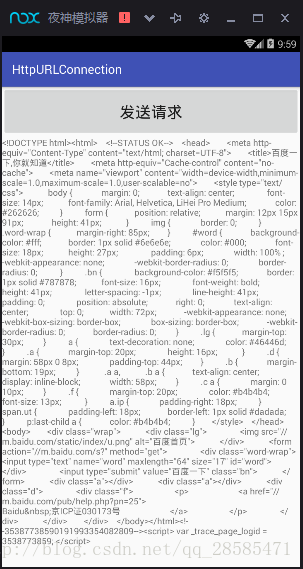
2、布局文件
3、MainActivity.java
4、声明网络权限
1、获取到HttpURLConnection的实例,并传入目标的网络地址,然后调用openConnection()方法
URL url = new URL("http://www.baidu.com"); HttpURLConnection connection = (HttpURLConnection)url.openConnection();
2、设置HTTP请求所使用的方法GET和POST
GET:从服务器那里获取数据
之后再调用getInputStream()方法获取到服务器返回的输入流
connection.setRequestMethod("GET"); InputStream in = connection.getInputStream();
POST:提交数据给服务器
每条数据都要以键值对的形式存在,数据与数据之间用“&”符号隔开
connection.setRequestMethod("POST"); DataOutputStream out = new DataOutputStream(connection.getOutputStream()); out.writeBytes("username=admin&password=123456");
3、调用disconnect()方法将HTTP连接关闭掉
connection.disconnect();
二、实例
需求:一个按钮用于发送HTTP请求,一个文本框用于将服务器返回的数据显示出来
1、效果图
2、布局文件
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <Button android:id="@+id/send_request" android:layout_width="match_parent" android:layout_height="80dp" android:text="发送请求" android:textSize="25dp"/> <ScrollView android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:id="@+id/response_text" android:layout_width="match_parent" android:layout_height="wrap_content" /> </ScrollView> </LinearLayout>
3、MainActivity.java
public class MainActivity extends AppCompatActivity implements View.OnClickListener { TextView responseText; Button sendRequset; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); sendRequset = (Button) findViewById(R.id.send_request); responseText = (TextView) findViewById(R.id.response_text); sendRequset.setOnClickListener(this); } @Override public void onClick(View view) { if (view.getId() == R.id.send_request){ sendRequsetWithHttpURLConnection(); } } private void sendRequsetWithHttpURLConnection() { //开启线程来发起网络请求 new Thread(new Runnable() { @Override public void run() { HttpURLConnection connection = null; BufferedReader reader = null; try { URL url = new URL("http://www.baidu.com"); connection = (HttpURLConnection) url.openConnection(); //提交数据给服务器的步骤 // connection.setRequestMethod("POST"); // DataOutputStream out = new DataOutputStream(connection.getOutputStream()); // out.writeBytes("username=admin&password=123456"); connection.setRequestMethod("GET"); //设置链接超时的毫秒数 connection.setConnectTimeout(8000); //设置读取超时的毫秒数 connection.setReadTimeout(8000); InputStream in = connection.getInputStream(); //下面对获取到的输入流进行读取 reader = new BufferedReader(new InputStreamReader(in)); StringBuilder response = new StringBuilder(); String line; while ((line = reader.readLine())!=null){ response.append(line); } showResponse(response.toString()); }catch (Exception e){ e.printStackTrace(); }finally { if(reader != null){ try { reader.close(); }catch (IOException e){ e.printStackTrace(); } } if (connection != null){ connection.disconnect(); } } } }).start(); } //结果传入showResponse方法中 private void showResponse(final String s) { runOnUiThread(new Runnable() { @Override public void run() { //在这里进行UI操作,将结果显示到界面上 responseText.setText(s); } }); } }
4、声明网络权限
<uses-permission android:name="android.permission.INTERNET"/>
相关文章推荐
- Android开发使用HttpURLConnection进行网络编程详解【附源码下载】
- Android开发-基础网络组件(1)使用HttpURLConnection登陆-注意打开网络需要在线程中执行-主线程不支持
- Android网络开发之HttpURLConnection
- Android开发中,HttpURLConnection与HttpClient哪个用来访问网络更好?
- Android应用开发网络请求 HttpClient和HttpURLConnection的区别
- Android开发-基础网络组件(1)使用HttpURLConnection登陆-AndroidStudio
- android开发-通过HttpURLConnection获取网络内容,Bitmap把二进制格式的图片转为位图
- Android开发之网络通信(HttpURLConnection,HttpClient,HttpGet,HttpPost)
- android 基础 网络交互 HttpClient HttpUrlConnection
- Android开发之网络请求通信专题(一):基于HttpURLConnection的请求通信
- Android开发与服务交互过程中,HTTPURLconnection 连接失败
- Android初级开发(九)——网络交互—OkHttp
- Android开发之网络请求HttpURLConnection
- Android网络连接之HttpURLConnection和HttpClient
- Android的三种网络联接方式(URL / HttpURLConnection | HttpClient | InetAddress )
- Android HttpURLConnection和HttpClient获取网络内容
- android HttpURLConnection 连接网络 读取返回数据
- Android网络连接之HttpURLConnection和HttpClient
- Android网络连接之HttpURLConnection和HttpClient
- Android 网络编程之HttpURLConnection