cxf+spring发布RESTful服务
2017-08-21 15:11
429 查看
什么是rest服务
REST 是一种软件架构模式,只是一种风格,rest服务采用HTTP 做传输协议,REST 对于HTTP 的利用分为以下两种:资源定位和资源操作。
资源定位:
Rest要求对资源定位更加准确,如下:
非rest方式:http://ip:port/queryUser.action?userType=student&id=001
Rest方式:http://ip:port/user/student/001
Rest方式表示互联网上的资源更加准确,但是也有缺点,可能目录的层级较多不容易理解。
资源操作:
利用HTTP 的GET、POST、PUT、DELETE 四种操作来表示数据库操作的SELECT、UPDATE、INSERT、DELETE 操作。
比如:
查询学生方法:
设置Http的请求方法为GET,url如下:
http://ip:port/user/student/001
添加学生方法:
设置http的请求方法为PUT,url如下:
http://ip:port/user/student/001/张三/……
Rest常用于资源定位,资源操作方式较少使用。
REST 是一种软件架构理念,现在被移植到Web 服务上,那么在开发Web 服务上,偏于面向资源的服务适用于REST,REST 简单易用,效率高,SOAP 成熟度较高,安全性较好。直接上demo。
注意:REST 不等于WebService,JAX-RS 只是将REST 设计风格应用到Web 服务开发上。
pom.xml
SEI以及实现类
实现类,简单模拟的数据:
applicationContext.xml
web.xml
这里的配置和上篇发布普通服务是一样的,这里不做赘述。
部署访问,http://localhost:8080/ws_spring_cxf_rest/ws/student/query/001
这里简单访问以下,可以有多种访问方式。
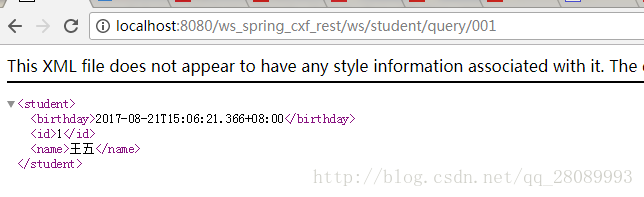
最后,这个cxf还挺不错的,这里做个记录,日后方便学习。
REST 是一种软件架构模式,只是一种风格,rest服务采用HTTP 做传输协议,REST 对于HTTP 的利用分为以下两种:资源定位和资源操作。
资源定位:
Rest要求对资源定位更加准确,如下:
非rest方式:http://ip:port/queryUser.action?userType=student&id=001
Rest方式:http://ip:port/user/student/001
Rest方式表示互联网上的资源更加准确,但是也有缺点,可能目录的层级较多不容易理解。
资源操作:
利用HTTP 的GET、POST、PUT、DELETE 四种操作来表示数据库操作的SELECT、UPDATE、INSERT、DELETE 操作。
比如:
查询学生方法:
设置Http的请求方法为GET,url如下:
http://ip:port/user/student/001
添加学生方法:
设置http的请求方法为PUT,url如下:
http://ip:port/user/student/001/张三/……
Rest常用于资源定位,资源操作方式较少使用。
REST 是一种软件架构理念,现在被移植到Web 服务上,那么在开发Web 服务上,偏于面向资源的服务适用于REST,REST 简单易用,效率高,SOAP 成熟度较高,安全性较好。直接上demo。
注意:REST 不等于WebService,JAX-RS 只是将REST 设计风格应用到Web 服务开发上。
pom.xml
<properties> <!-- CXF版本 --> <cxf.version>3.1.11</cxf.version> <spring.version>4.1.7.RELEASE</spring.version> </properties> <dependencies> <dependency> <groupId>org.apache.cxf</groupId> <artifactId>cxf-rt-frontend-jaxrs</artifactId> <version>${cxf.version}</version> </dependency> <dependency> <groupId>org.apache.cxf</groupId> <artifactId>cxf-rt-transports-http</artifactId> <version>${cxf.version}</version> </dependency> <dependency> <groupId>org.apache.cxf</groupId> <artifactId>cxf-rt-transports-http-jetty</artifactId> <version>${cxf.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-web</artifactId> <version>${spring.version}</version> </dependency> <!-- https://mvnrepository.com/artifact/org.eclipse.jetty/jetty-webapp --> <dependency> <groupId>org.eclipse.jetty</groupId> <artifactId>jetty-webapp</artifactId> <version>9.2.13.v20150730</version> </dependency> <!-- https://mvnrepository.com/artifact/javax.ws.rs/javax.ws.rs-api --> <dependency> <groupId>javax.ws.rs</g d83c roupId> <artifactId>javax.ws.rs-api</artifactId> <version>2.0.1</version> </dependency> </dependencies> <build> <finalName>ws_spring_cxf_rest</finalName> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <configuration> <source>1.8</source> <target>1.8</target> <encoding>UTF-8</encoding> </configuration> </plugin> </plugins> </build>
SEI以及实现类
@WebService //@Path("/student") public interface StudentService { //查询学生信息 @GET //http的get方法 @Path("/query/{id}")//id参数通过url传递 @Produces(MediaType.APPLICATION_XML)//设置媒体类型xml格式 public Student queryStudent(@PathParam("id")long id); //查询学生列表 @GET //http的get方法 @Path("/querylist/{type}") @Produces({"application/json;charset=utf-8",MediaType.APPLICATION_XML})//设置媒体类型xml格式和json格式 //如果想让rest返回xml需要在rest的url后边添加?_type=xml,默认为xml //如果想让rest返回json需要在rest的url后边添加?_type=json public List<Student> queryStudentList(@PathParam("type") String type); }
实现类,简单模拟的数据:
public class StudentServiceImpl implements StudentService{ @Override public Student queryStudent(long id) { //模拟数据 Student student=new Student(); student.setBirthday(new Date()); student.setId(id); student.setName("王五"); return student; } @Override public List<Student> queryStudentList(String type) { // 使用静态数据 List<Student> list = new ArrayList<Student>(); Student student1 = new Student(); student1.setId(1000l); student1.setName("张三"); student1.setBirthday(new Date()); Student student2 = new Student(); student2.setId(1000l); student2.setName("张三"); student2.setBirthday(new Date()); list.add(student1); list.add(student2); return list; } }
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:jaxws="http://cxf.apache.org/jaxws" xmlns:jaxrs="http://cxf.apache.org/jaxrs" xmlns:cxf="http://cxf.apache.org/core" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://cxf.apache.org/jaxrs http://cxf.apache.org/schemas/jaxrs.xsd http://cxf.apache.org/jaxws http://cxf.apache.org/schemas/jaxws.xsd http://cxf.apache.org/core http://cxf.apache.org/schemas/core.xsd"> <!-- service --> <bean id="studentService" class="com.kongl.service.StudentServiceImpl"/> <jaxrs:server address="/student"> <jaxrs:serviceBeans> <ref bean="studentService"/> </jaxrs:serviceBeans> </jaxrs:server> </beans>
web.xml
这里的配置和上篇发布普通服务是一样的,这里不做赘述。
部署访问,http://localhost:8080/ws_spring_cxf_rest/ws/student/query/001
这里简单访问以下,可以有多种访问方式。
最后,这个cxf还挺不错的,这里做个记录,日后方便学习。
相关文章推荐
- Spring整合cxf发布restful服务
- (五)CXF整合Spring发布RESTful风格的Web服务
- 使用cxf-rs发布服务启动报错:org.springframework.beans.factory.NoSuchBeanDefinitionException
- (七) CXF 整合Spring--发布WS服务
- webservice--CXF+Spring整合发布SOAP协议的服务
- 使用Spring + CXF 发布WebService服务
- WebService(CXF发布WebService服务与Spring整合)(2)
- cxf集成到spring中发布restful webservice
- CXF+Spring搭建Restful接口服务
- 使用CXF和spring发布rest服务
- (七)CXF之与spring整合发布web服务
- cxf+spring发布webservice服务(服务器端)
- webservice--CXF+Spring整合发布REST的服务
- cxf+spring发布webservice服务(服务器端)
- Spring 集成CXF框架发布Webservice服务 和 使用jdk生成Webservice clinet
- 使用cxf发布rest服务接口,和spring的整合
- CXF 开发RESTful WebService服务(Spring+JPA服务端,JAXRS2.0+WebClient客户端)
- 【webservice】CXF结合spring发布简单的webservice服务
- cxf+spring发布webservice服务(客户端)
- CXF系列之JAX-RS:CXF与spring集成发布REST服务