用C语言实现简单的停车场管理
2017-08-02 14:47
447 查看
这个程序是利用栈和循环队列实现的,自己得先处理好逻辑关系就好了。由于题目没有要求,这个程序就没加重复判断,比如一辆车已经停在车位上或者便道上,再来一辆就判断不了了。关于栈,就是先进后出的思想,队列就是先进先出的思想。这个程序自己没用链栈和链队列做,因为感觉比较耗时。不过栈和队列的运用大多数都是用数组,先掌握好数组的表示再用链表写上手也很快。
项目要求:停车场是一个能放 n 辆车的狭长通道,只有一个大门,汽车按到达的先后次序停放。若车场满了,车要在门外的便道上等候,一旦有车走,则便道上第一辆车进入。当停车场中的车离开时,由于通道窄,在它后面的车要先退出,待它走后依次进入。汽车离开时按停放时间收费。
基本功能要求:
1)建立三个数据结构分别是:停放队列,让路栈,等候队列
2)输入数据模拟管理过程,数据(入或出,车号)。
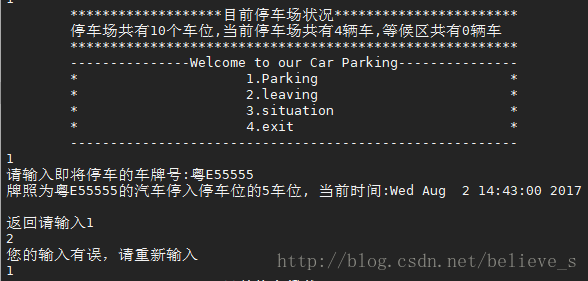
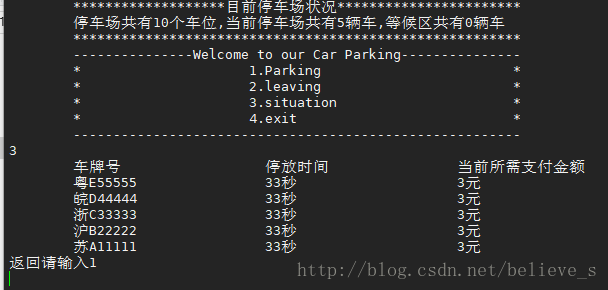
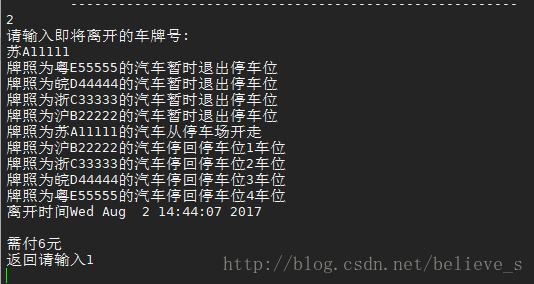
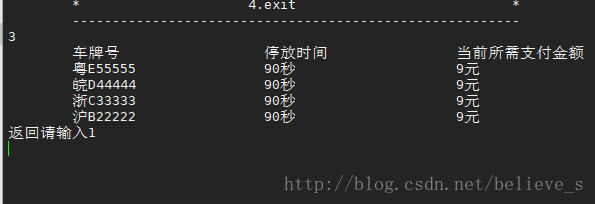
头文件: PLot.h
源文件 PLot.c
主函数 main.c
项目要求:停车场是一个能放 n 辆车的狭长通道,只有一个大门,汽车按到达的先后次序停放。若车场满了,车要在门外的便道上等候,一旦有车走,则便道上第一辆车进入。当停车场中的车离开时,由于通道窄,在它后面的车要先退出,待它走后依次进入。汽车离开时按停放时间收费。
基本功能要求:
1)建立三个数据结构分别是:停放队列,让路栈,等候队列
2)输入数据模拟管理过程,数据(入或出,车号)。
头文件: PLot.h
#ifndef __PLOT_H__ #define __PLOT_H__ #define FALSE 0 #define TRUE 1 #define MONEY 1 // 单价可以自己定义 #define MAX_STOP 10 #define MAX_PAVE 100 // 存放汽车牌号 typedef struct { int time1; // 进入停车场时间 int time2; // 离开停车场时间 char plate[10]; // 汽车牌照号码,定义一个字符指针类型 }Car; // 停放栈 typedef struct { Car Stop[MAX_STOP-1]; // 各汽车信息的存储空间 int top; // 用来指示栈顶位置的静态指针 }Stopping; // 等候队列 typedef struct { int count; // 用来指示队中的数据个数 Car Pave[MAX_PAVE-1]; // 各汽车信息的存储空间 int front, rear; // 用来指示队头和队尾位置的静态指针 }Pavement; // 让路栈 typedef struct { Car Help[MAX_STOP-1]; // 各汽车信息的存储空间 int top; // 用来指示栈顶位置的静态指针 }Buffer; Stopping s; Pavement p; Buffer b; Car c; char C[10]; void stop_pave (); // 车停入便道 void car_come (); // 车停入停车位 void stop_to_buff (); // 车进入让路栈 void car_leave (); // 车离开 void welcome (); // 主界面函数 void Display (); // 显示车辆信息 #endif
源文件 PLot.c
#include "PLot.h" #include <stdio.h> #include <time.h> // 包含时间函数的头文件 #include <string.h> #include <stdlib.h> void stop_to_pave() // 车停入便道 { // 判断队满 if (p.count > 0 && (p.front == (p.rear + 1) % MAX_PAVE)) { printf ("便道已满,请下次再来\n"); } else { strcpy(p.Pave[p.rear].plate, C); p.rear = (p.rear + 1) % MAX_PAVE; // 队尾指示器加1 p.count++; // 计数器加1 printf ("牌照为%s的汽车停入便道上的%d的位置\n", C, p.rear); } } void car_come() // 车停入停车位 { printf ("请输入即将停车的车牌号:"); // 输入车牌号 scanf ("%s", &C); if (s.top >= MAX_STOP - 1) // 如果停车位已满,停入便道 { stop_to_pave(); // 停车位->便道 函数 } else { s.top++; // 停车位栈顶指针加1 time_t t1; long int t = time (&t1); // 标记进入停车场的时间 char* t2; t2 = ctime (&t1); // 获取当前时间 c.time1 = t; strcpy(s.Stop[s.top].plate, C); // 将车牌号登记 printf ("牌照为%s的汽车停入停车位的%d车位, 当前时间:%s\n", C, s.top + 1, t2); } return ; } void stop_to_buff() // 车进入让路栈 { // 停车位栈压入临时栈,为需要出栈的车辆让出道 while (s.top >= 0) { if (0 == strcmp(s.Stop[s.top--].plate, C)) { break; } // 让出的车进入让路栈 strcpy(b.Help[b.top++].plate, s.Stop[s.top + 1].plate); printf ("牌照为%s的汽车暂时退出停车位\n", s.Stop[s.top + 1].plate); } b.top --; // 如果停车位中的车都让了道,说明停车位中无车辆需要出行 if (s.top < -1) { printf ("停车位上无此车消息\n"); } else { printf ("牌照为%s的汽车从停车场开走\n", s.Stop[s.top + 1].plate); } // 将让路栈中的车辆信息压入停车位栈 while (b.top >= 0) { strcpy(s.Stop[++s.top].plate, b.Help[b.top--].plate); printf ("牌照为%s的汽车停回停车位%d车位\n", b.Help[b.top + 1].plate, s.top + 1); } // 从便道中 -> 停车位 while (s.top < MAX_STOP-1) { if (0 == p.count) // 判断队列是否为空 { break; } // 不为空,将便道中优先级高的车停入停车位 else { strcpy(s.Stop[++s.top].plate, p.Pave[p.front].plate); printf ("牌照为%s的汽车从便道中进入停车位的%d车位\n", p.Pave[p.front].plate, s.top+1); p.front = (p.front + 1) % MAX_PAVE; p.count--; } } } void car_leave() // 车离开 { printf ("请输入即将离开的车牌号:\n"); scanf ("%s", &C); if (s.top < 0) // 判断停车位是否有车辆信息 { printf ("车位已空,无车辆信息!\n"); } else { stop_to_buff(); } time_t t1; long int t = time (&t1); c.time2 = t; // 标记离开停车场的时间 char* t2; t2 = ctime (&t1); // 获取当前时间 printf ("离开时间%s\n需付%ld元\n", t2, MONEY * (c.time2 - c.time1) / 10); } void Display() { int i = s.top; if (-1 == i) { printf ("停车场为空\n"); } time_t t1; long int t = time(&t1); // 标记显示时的时间 printf ("\t车牌号\t\t\t停放时间\t\t当前所需支付金额\n"); while (i != -1) { printf ("\t%s\t\t%d秒\t\t\t%d元\n", s.Stop[i].plate, t - c.time1, MONEY * (t - c.time1) / 10); i--; } } void welcome() { printf ("\t*******************目前停车场状况***********************\n"); printf ("\t停车场共有%d个车位,当前停车场共有%d辆车,等候区共有%d辆车\n", MAX_STOP, s.top+1, (p.rear + MAX_PAVE - p.front) % MAX_PAVE); printf ("\t********************************************************\n"); printf ("\t---------------Welcome to our Car Parking---------------\n"); printf ("\t* 1.Parking *\n"); printf ("\t* 2.leaving *\n"); printf ("\t* 3.situation *\n"); printf ("\t* 4.exit *\n"); printf ("\t--------------------------------------------------------\n"); }
主函数 main.c
/********************************************************** 问题描述:停车场是一个能放 n 辆车的狭长通道,只有一个大门, 汽车按到达的先后次序停放。若车场满了,车要在门外的便道上等候 ,一旦有车走,则便道上第一辆车进入。当停车场中的车离开时,由 于通道窄,在它后面的车要先退出,待它走后依次进入。汽车离开 时按停放时间收费。 基本功能要求: 1)建立三个数据结构分别是:停放队列,让路栈,等候队列 2)输入数据模拟管理过程,数据(入或出,车号)。 ***********************************************************/ #include "PLot.h" int main() { // 初始化 s.top = -1; b.top = 0; p.rear = 0; p.count = 0; p.front = 0; while(1) { system("clear"); welcome(); int i, cho; scanf ("%d", &i); if (1 == i) car_come(); if (2 == i) car_leave(); if (3 == i) Display(); if (4 == i) break; printf ("返回请输入1\n"); scanf ("%d", &cho); if (1 == cho) { continue; } else { printf ("您的输入有误,请重新输入\n"); scanf ("%d", &cho); continue; } } return 0; }
相关文章推荐
- 一个大学C语言试题的简单实现--员工信息管理程序
- C语言-实现简单的车辆管理系统-课程设计
- 单链表的应用:用C语言实现简单的员工管理系统(新建、增、删、改、查、排序)
- java栈+队列实现简单的停车场管理问题
- c语言实现的简单的员工工资管理系统
- C语言实现简单学籍管理系统
- C语言实现简单学生管理系统
- C语言实现简单学生成绩管理系统
- C语言简单用链表实现学生管理系统
- 【C语言】模拟狭窄停车场管理,使用栈和队列实现
- C语言停车场模拟管理程序的设计与实现
- Linux环境下C语言实现简单的基于文件的学生信息管理系统
- 学生信息管理系统简单模拟(C语言实现)
- 用C语言实现一个简单的HTTP客户端(HTTP Client)
- 用简单的C语言实现多任务轮流切换(模拟操作系统线程机制)
- 用C语言实现一个简单的HTTP客户端(HTTP Client)
- C语言实现简单的停车场管理系统
- 用链表实现一个简单的学生操作管理系统C语言版
- C语言的HashTable简单实现
- 简单的Linux扫描仪应用:C语言实现