SpringBoot使用WEB进阶
2017-07-23 00:00
225 查看
摘要: SpringBoot使用WEB进阶
1.使用aop处理请求get,post
2.统一异常检查
3.单元测试
4效果预览:
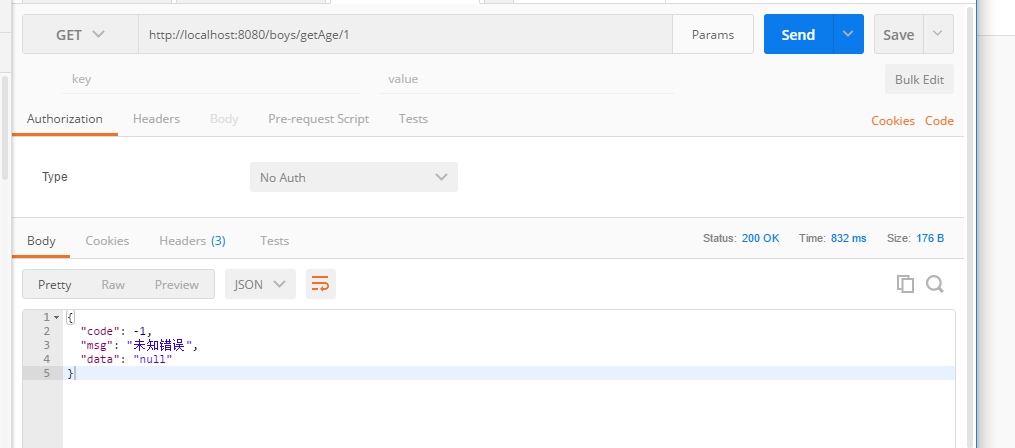
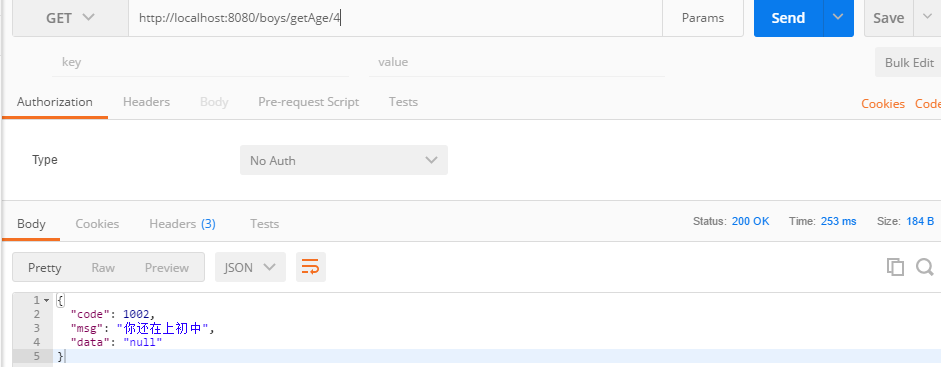
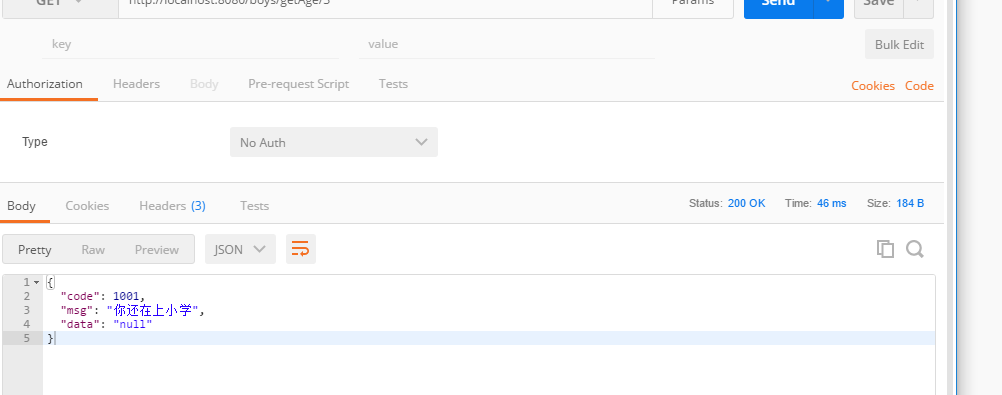
5.开发环境
maven开发环境:3.3.9
Itellij IDEA旗舰版
java开发环境java version "1.8.0_91"
6.完成的 完整的代码实例
HttpAspect
BoyController
HelloController
Boy
Result
ResultEnum
BoyException
ExceptionHandle
Boyproperties
BoyRepository
BoyService
ResultUtil
HlsApplication
1.使用aop处理请求get,post
2.统一异常检查
3.单元测试
4效果预览:
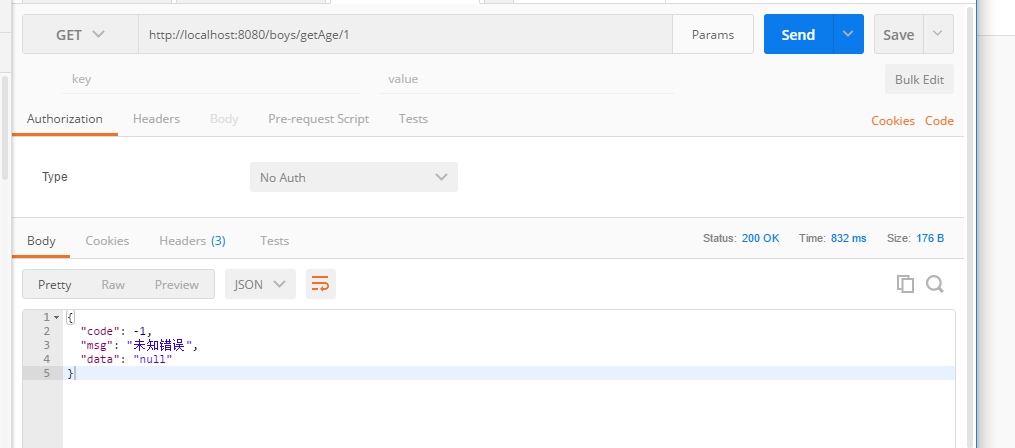
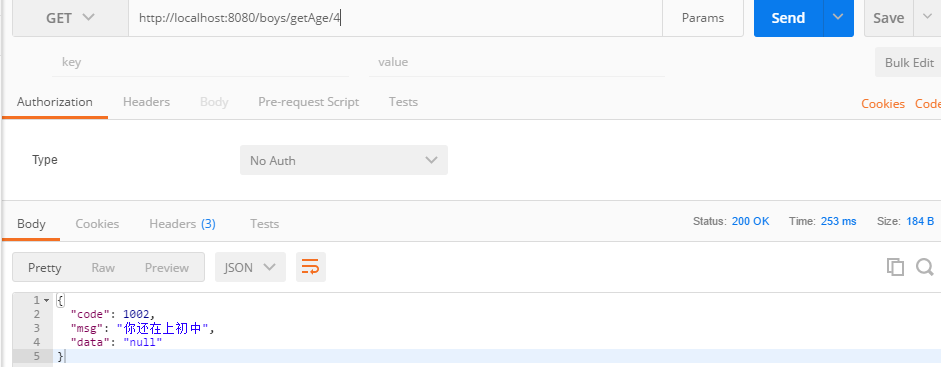
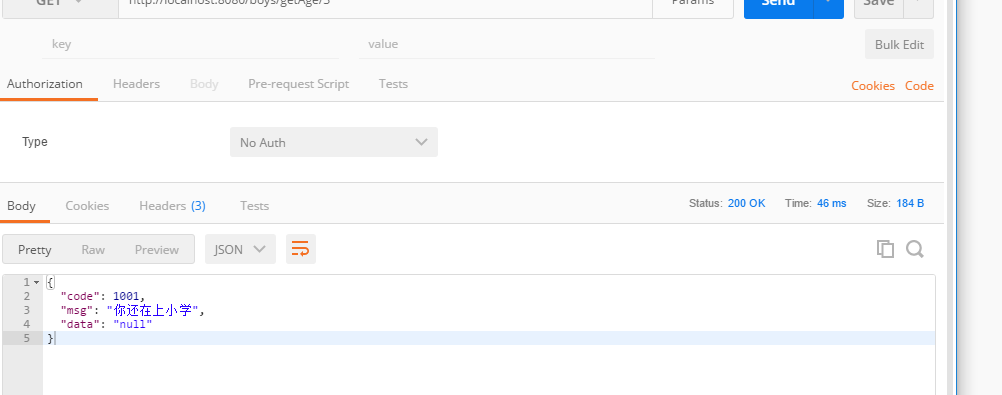
5.开发环境
maven开发环境:3.3.9
Itellij IDEA旗舰版
java开发环境java version "1.8.0_91"
6.完成的 完整的代码实例
HttpAspect
package com.hls; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class HlsApplication { public static void main(String[] args) { SpringApplication.run(HlsApplication.class, args); } }
BoyController
package com.hls.controller; import com.hls.domain.Boy; import com.hls.domain.Result; import com.hls.repository.BoyRepository; import com.hls.service.BoyService; import com.hls.util.ResultUtil; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Value; import org.springframework.validation.BindingResult; import org.springframework.web.bind.annotation.*; import javax.validation.Valid; import java.util.List; /** * Created by huangliusong on 2017/7/19. * 查询男生列表 */ @RestController public class BoyController { @Autowired private BoyRepository boyRepository; @Autowired private BoyService boyService; @GetMapping(value = "/boys") public List<Boy> boylist(){ return boyRepository.findAll(); } @PostMapping(value="/boys") public Result<Boy> boyadd(@Valid Boy boy, BindingResult bindingResult){ if(bindingResult.hasErrors()){ return ResultUtil.error(0,bindingResult.getFieldError().getDefaultMessage()); } boy.setAge(boy.getAge()); boy.setBirth(boy.getBirth()); boy.setId(1001); return ResultUtil.success(boyRepository.save(boy)); } //查询 @GetMapping(value = "/boys/{id}") public Boy findBoy(@PathVariable("id")Integer id){ return boyRepository.findOne(id); } //更新 @PostMapping(value = "/boys/{id}") public Boy updateBoy(@PathVariable("id")Integer id, @RequestParam("age")String age, @RequestParam("birth")String birth){ Boy boy=new Boy(); boy.setId(id); boy.setBirth(birth); boy.setAge(age); return boyRepository.save(boy); } //删除 @DeleteMapping(value = "/boys/{id}") public void deleteBoy(@PathVariable("id")Integer id){ boyRepository.delete(id); } //通过年龄查询 @GetMapping(value = "boys/age/{age}") public List<Boy> boylistByAge(@PathVariable("age") String age){ return boyRepository.findByAge(age); } @PostMapping(value = "/boys/two") public void boyTwo(){ boyService.insertBoyTow(); } /** * 对年龄进行判断 */ @GetMapping(value = "/boys/getAge/{id}") public void getAge(@PathVariable("id")Integer id)throws Exception{ boyService.getAge(id); } }
HelloController
package com.hls.controller; import com.hls.repository.Boyproperties; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.*; /** * Created by huangliusong on 2017/7/18. */ @RestController @RequestMapping("/huang") public class HelloController { @Autowired private Boyproperties boyproperties; @GetMapping("/liu1") public String say (@RequestParam(value="id",required = false, defaultValue ="0") Integer id){ return "index.html?"+id; } }
Boy
package com.hls.domain; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.Id; import javax.validation.constraints.Min; /** * Created by huangliusong on 2017/7/19. */ @Entity public class Boy { @Id @GeneratedValue private Integer id; @Min(value=18,message = "不是18,不能录入进去") private String age; private String birth; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getAge() { return age; } public void setAge(String age) { this.age = age; } public String getBirth() { return birth; } public void setBirth(String birth) { this.birth = birth; } public Boy() { } @Override public String toString() { return "Boy{" + "id=" + id + ", age='" + age + '\'' + ", birth='" + birth + '\'' + '}'; } }
Result
package com.hls.domain; /** * http请求返回的最外层对象 * Created by huangliusong on 2017/7/22. */ public class Result<T> { //错误码 private Integer code; //提示信息 private String msg; //具体的内容 private T data; public Integer getCode() { return code; } public void setCode(Integer code) { this.code = code; } public String getMsg() { return msg; } public void setMsg(String msg) { this.msg = msg; } public T getData() { return data; } public void setData(T data) { this.data = data; } }
ResultEnum
package com.hls.enmus; /** * Created by huangliusong on 2017/7/22. */ public enum ResultEnum { UNKNOW_ERROR(-1,"未知错误"), SUCCESS(0,"成功"), PRIMARY_SCHOOL(1001,"你还在上小学"), MIDDLE_SCHOOL(1002,"你还在上初中") ; public Integer getCode() { return code; } public void setCode(Integer code) { this.code = code; } public String getMsg() { return msg; } public void setMsg(String msg) { this.msg = msg; } private Integer code; private String msg; ResultEnum(Integer code, String msg) { this.code = code; this.msg = msg; } }
BoyException
package com.hls.exception; import com.hls.domain.Result; import com.hls.enmus.ResultEnum; /** * Created by huangliusong on 2017/7/22. */ public class BoyException extends RuntimeException{ private Integer code; public BoyException(ResultEnum resultEnum) { super(resultEnum.getMsg()); this.code = resultEnum.getCode(); } public Integer getCode() { return code; } public void setCode(Integer code) { this.code = code; } }
ExceptionHandle
package com.hls.handler; import com.hls.domain.Result; import com.hls.exception.BoyException; import com.hls.util.ResultUtil; import org.springframework.web.bind.annotation.ControllerAdvice; import org.springframework.web.bind.annotation.ExceptionHandler; import org.springframework.web.bind.annotation.ResponseBody; /** * Created by huangliusong on 2017/7/22. */ @ControllerAdvice public class ExceptionHandle { @ExceptionHandler(value = Exception.class) @ResponseBody public Result handler(Exception e){ if(e instanceof BoyException){ BoyException boyException=(BoyException)e; return ResultUtil.error(boyException.getCode(),boyException.getMessage()); }else{ return ResultUtil.error(-1,"未知错误"); } } }
Boyproperties
package com.hls.repository; import org.springframework.boot.context.properties.ConfigurationProperties; import org.springframework.context.annotation.Configuration; import org.springframework.stereotype.Component; /** * Created by huangliusong on 2017/7/18. */ @Component @ConfigurationProperties(prefix = "boy") public class Boyproperties { private String hlsBirth; private Integer hlsAge; public String getHlsBirth() { return hlsBirth; } public void setHlsBirth(String hlsBirth) { this.hlsBirth = hlsBirth; } public void setHlsAge(Integer hlsAge) { this.hlsAge = hlsAge; } public Integer getHlsAge() { return hlsAge; } }
BoyRepository
package com.hls.repository; import com.hls.domain.Boy; import org.springframework.data.jpa.repository.JpaRepository; import java.util.List; /** * Created by huangliusong on 2017/7/19. */ public interface BoyRepository extends JpaRepository<Boy,Integer>{ //通过年龄查询 public List<Boy> findByAge(String age); }
BoyService
package com.hls.service; import com.hls.enmus.ResultEnum; import com.hls.exception.BoyException; import com.hls.repository.BoyRepository; import com.hls.domain.Boy; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import javax.transaction.Transactional; /** * Created by huangliusong on 2017/7/21. */ @Service public class BoyService { @Autowired private BoyRepository boyRepository; //添加事物注解 @Transactional public void insertBoyTow(){ Boy boy1=new Boy(); boy1.setAge("67"); boy1.setBirth("1967"); Boy boy2=new Boy(); boy2.setAge("688"); boy2.setBirth("1968"); boyRepository.save(boy1); boyRepository.save(boy2); } public void getAge(Integer id) throws Exception{ Boy boy=boyRepository.findOne(id); Integer age=Integer.parseInt(boy.getAge()); System.out.println(age); if(age<10){ //返回你还在上小学 throw new BoyException(ResultEnum.PRIMARY_SCHOOL); }else if(age>10&&age<16){ //返回 你还在上初中 throw new BoyException(ResultEnum.MIDDLE_SCHOOL); } } /** * 通过id查询详细信息 * @param id * @return */ public Boy findOneBoy(Integer id){ return boyRepository.findOne(id); } }
ResultUtil
package com.hls.util; import com.hls.domain.Result; import com.sun.org.apache.regexp.internal.RE; /** * Created by huangliusong on 2017/7/22. */ public class ResultUtil { public static Result success(Object object){ Result result=new Result(); result.setCode(0); result.setData(object); result.setMsg("成功"); return result; } public static Result success(){ return success(null); } public static Result error(Integer code,String msg){ Result result=new Result(); result.setMsg(msg); result.setCode(code); result.setData("null"); return result; } }
HlsApplication
package com.hls; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class HlsApplication { public static void main(String[] args) { SpringApplication.run(HlsApplication.class, args); } }
相关文章推荐
- 【SpringBoot】Spring Boot进阶之Web进阶( 第2章 Web进阶-使用AOP处理请求 )
- 使用Spring Boot开发Web项目
- 使用Spring Boot开发Web项目(二)之添加HTTPS支持
- 使用Spring Boot开发Web项目(二)之添加HTTPS支持
- 爬虫框架webmagic与spring boot的结合使用
- 使用Spring Boot开发Web项目
- springboot使用webmagic框架来抓取自己的博客信息
- Spring Boot 之web Filter --不支持排序的使用
- Spring Boot中使用AOP统一处理Web请求日志
- 使用idea+springboot+Mybatis搭建web项目
- 使用Spring Boot开发Web项目
- 46. Spring Boot中使用AOP统一处理Web请求日志【从零开始学Spring Boot】
- Spring Boot 之FilterRegistrationBean --支持web Filter 排序的使用
- • Spring Boot - 进阶 数 据 访 问 -使用jdbcTemplate访问数据库
- 使用spring boot和thymeleaf提供web内容
- 使用Spring Boot开发Web项目(二)之添加HTTPS支持
- Spring Boot 之web Filter --支持排序的使用扩展
- 使用Spring Boot开发Web项目
- springboot学习1-使用 Spring Starter Project快速创建基于spring-boot的web项目
- 使用Spring Boot开发Web项目