JAVA JBDC+开源工具连接MySql数据库示例心得二
2017-07-17 23:20
357 查看
使用数据库连接池连接数据库,还使用了一些开源工具简化上一次的JDBC开发,下面是代码示例:
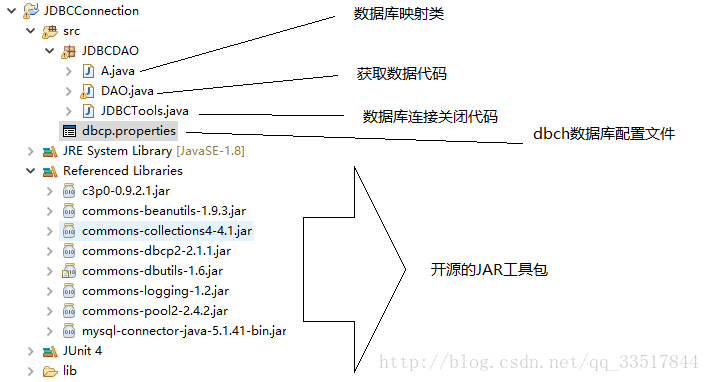
先看DBCH数据库连接池的配置文件的内容:
A.java的代码片,里面的属性与数据库的字段名一样,是数据库的映射对象类
然后先看JDBCTools.java数据库连接的代码操作:
在看DAO.java,最后写了几个查询数据的方式,还望提高哈。。。。。
JDBC的学习到这里就结束了,接下来学习Hibernate等框架的使用。。。。
先看DBCH数据库连接池的配置文件的内容:
driverClassName=com.mysql.jdbc.Driver url=jdbc:mysql://localhost:3306/userdb?useUnicode=true&characterEncoding=utf-8&useSSL=false username=root password=123456 dataSource.initialSize=20 dataSource.maxIdle=20 dataSource.minIdle=5 dataSource.maxActive=100 dataSource.logAbandoned=true dataSource.removeAbandoned=true dataSource.removeAbandonedTimeout=180 dataSource.maxWait=1000
A.java的代码片,里面的属性与数据库的字段名一样,是数据库的映射对象类
package JDBCDAO; public class A { private int id; private String name; private String password; private String email; public A() { } public A(int id, String name, String password, String email) { this.id = id; this.name = name; this.password = password; this.email = email; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } public String toString() { return "A [id=" + id + ", name=" + name + ", password=" + password + ", email=" + email + "]"; } }
然后先看JDBCTools.java数据库连接的代码操作:
package JDBCDAO; import java.io.InputStream; import java.sql.Connection; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import java.util.Properties; import org.apache.commons.dbcp2.BasicDataSource; import org.apache.commons.dbcp2.BasicDataSourceFactory; public class JDBCTools { /** * 使用DBCP连接池连接数据库 * @return Connection */ public static Connection GetdbcpConnection(){ Connection connection = null; try { Properties properties = new Properties(); //获取配置文件流 InputStream in = JDBCTools.class.getClassLoader().getResourceAsStream("dbcp.properties"); properties.load(in); BasicDataSource basicDataSource = BasicDataSourceFactory.createDataSource(properties);// 得到一个连接池对象 connection = basicDataSource.getConnection(); } catch (Exception e) { e.printStackTrace(); } return connection; } /** * 关闭connection连接,statement连接,resultSet连接 */ public static void CloseCSR(Connection connection,Statement statement,ResultSet resultSet){ if(connection!=null){ try { connection.close(); } catch (SQLException e) { e.printStackTrace(); } } if(statement!=null){ try { statement.close(); } catch (SQLException e) { e.printStackTrace(); } } if(resultSet!=null){ try { resultSet.close(); } catch (SQLException e) { e.printStackTrace(); } } } /** * 开始事务 */ public static void setAutoCommit(Connection connection){ try { connection.setAutoCommit(false); System.out.println("开始事务"); } catch (SQLException e) { e.printStackTrace(); } } /** * 提交事务 */ public static void commit(Connection connection){ try { connection.commit(); System.out.println("提交事务"); } catch (SQLException e) { e.printStackTrace(); } } /** * 回滚事务 */ public static void rollback(Connection connection){ try { connection.rollback(); System.out.println("回滚事务"); } catch (SQLException e) { e.printStackTrace(); } } }
在看DAO.java,最后写了几个查询数据的方式,还望提高哈。。。。。
package JDBCDAO; import java.lang.reflect.InvocationTargetException; import java.sql.Connection; import java.sql.ResultSet; import java.sql.ResultSetMetaData; import java.sql.SQLException; import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map; import org.apache.commons.beanutils.BeanUtils; import org.apache.commons.dbutils.QueryRunner; import org.apache.commons.dbutils.ResultSetHandler; import org.apache.commons.dbutils.handlers.MapHandler; import org.apache.commons.dbutils.handlers.MapListHandler; /** * 访问数据的DAO 定义好访问数据表的各种方法 * * @param T:DAO处理实体类的类型 */ public class DAO { private QueryRunner queryRunner = null; public DAO() { queryRunner = new QueryRunner(); } /** * 返回一个List<A>的列表里面存储了与数据库映射的数据 * 就是将数据库所有结果集与A类映射,List装下所有A对象,一个A类对应一条字段 */ @SuppressWarnings("unchecked") public List get(Class clazz, Connection connection, String sql, Object... objects) throws SQLException, InstantiationException, IllegalAccessException { List<A> as = null; if (objects == null){ as = (List<A>) queryRunner.query(connection, sql, new MyResultsethandler(clazz)); } else { as = (List<A>) queryRunner.query(connection, sql, new MyResultsethandler(clazz), objects); } return as; } /** * 返回查询到的所有数据Map集合的结果集 返回所有查询到的列 * */ public List getListMapValues(Connection connection, String sql, Object... objects) throws SQLException { List<Map<String, Object>> values = null; if (objects == null) { values = queryRunner.query(connection, sql, new MapListHandler()); } else { values = queryRunner.query(connection, sql, new MapListHandler(), objects); } return values; } /** * 返回查询到的一条数据或第一条数据的Map集合的结果集; 只返回一条数据 * */ public Map<String, Object> getMapValue(Connection connection, String sql, Object... objects) throws SQLException, IllegalAccessException, InvocationTargetException, InstantiationException, NoSuchMethodException, SecurityException { Map<String, Object> values = null; if (objects == null) { values = queryRunner.query(connection, sql, new MapHandler()); } else { values = queryRunner.query(connection, sql, new MapHandler(), objects); } return values; } /** * 更新数据操作 INSERT,UPDATE,DELETE */ public void Update(Connection connection, String sql, Object... objects) throws SQLException { if (objects == null) {// 不带占位符的SQL语句 queryRunner.update(connection, sql); } else {// 带占位符的SQL语句 queryRunner.update(connection, sql, objects); } } } /** * 实现ResultSetHandler接口,返回List数据给上面用 * 数据库映射对象 */ class MyResultsethandler implements ResultSetHandler { Class clazz = null; public MyResultsethandler(Class clazz) throws InstantiationException, IllegalAccessException { this.clazz = clazz; } @Override public Object handle(ResultSet rs) throws SQLException { List<Object> liClazz = new ArrayList();//List存储对象 Map<String, Object> map = null;//存储一条字段 Object object = null;//用于反射实例化 List<Map<String, Object>> listMap = new ArrayList();//将所有Map集合存于List里面 ResultSetMetaData data = rs.getMetaData(); while (rs.next()) { map = new HashMap<String, Object>(); for (int i = 0; i < data.getColumnCount(); i++) { String columnLabel = data.getColumnLabel(i + 1);// 得到列名 Object columnValue = rs.getObject(i + 1);// 得到值 map.put(columnLabel, columnValue); } listMap.add(map); } if (listMap.size() > 0) { for (int i = 0; i < listMap.size(); i++) { try { object = clazz.newInstance(); for (Map.Entry<String, Object> entry : listMap.get(i).entrySet()) { String fieldName = entry.getKey(); Object value = entry.getValue(); BeanUtils.setProperty(object, fieldName, value); } liClazz.add(object); } catch (InstantiationException e) { e.printStackTrace(); } catch (IllegalAccessException e) { e.printStackTrace(); } catch (InvocationTargetException e) { e.printStackTrace(); } } } return liClazz; } }
JDBC的学习到这里就结束了,接下来学习Hibernate等框架的使用。。。。
相关文章推荐
- JDBC java连接mysql数据库示例
- JDBC中 mysql数据库的连接工具类 Java登录 及增删改查 整理 附带:Navicat Premium 11.0.12中文破解版.zip(下载)mysql数据库工具
- jdbc:java连接mysql数据库示例
- java实现的连接oracle/mysql数据库功能简单示例【附oracle+mysql数据库驱动包】
- Java连接MYSQL数据库的示例代码
- java 连接MySQL数据库提取信息示例
- Java基于jdbc连接mysql数据库操作示例
- Android 4.1:UI开源,连接,服务和工具 全面升级
- JAVA连接MySQL数据库----(常用数据库连接二)
- .net连接MYSQL数据库的方法及示例!
- java使用jdbc连接数据库工具类和jdbc连接mysql数据示例
- Android开源插件工具DL(dynamic-load-apk)学习心得
- Java连接MySql数据库的方法
- 7 款开源 Java 反编译工具
- JDBC简单介绍以及Java连接MySQL数据库
- java实现连接mysql数据库单元测试查询数据项目分享
- JDBC连接MySQL数据库及示例
- java连接MySQL数据库
- Java对MySQL数据库进行连接、查询和修改(转)