Thinking in java-39 序列化 Serialization
2017-07-17 10:38
330 查看
1. 对象序列化的意义
本文详细内容参考自java_T_point && tutorialspoint.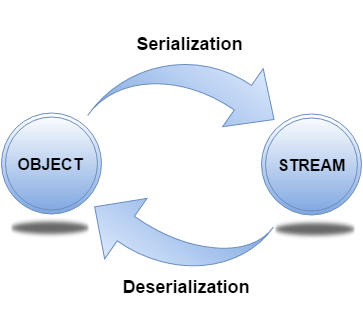
Java语言中的序列化:将对象状态写入字节流中的机制。
对象可以用一组包含该对象数据和信息(包含对象的类型、存储在对象中的数据)的字节所表示。在序列化对象写入到文件之后,该对象可以从文件中读出,并进行反向的解序列化过程。也就是说,类型信息、代表该对象的字节信息及相关数据可以用来在内存中重建该对象数据。
2. 对象序列化常用的类和方法
//Methods used in Serialization java.io.FileOutputStream.FileOutputStream(String name) throws FileNotFoundException java.io.ObjectOutputStream.ObjectOutputStream(OutputStream out) throws IOException void java.io.ObjectOutputStream.writeObject(Object obj) throws IOException //Methods used in Deserialization java.io.FileInputStream.FileInputStream(String name) throws FileNotFoundException java.io.ObjectInputStream.ObjectInputStream(InputStream in) throws IOException Object java.io.ObjectInputStream.readObject() throws IOException, ClassNotFoundException
3. 序列化和解序列化Demo
对于要持久化的对象,必须实现Serializable interface.package com.fqy.serial; import java.io.Serializable; public class Student implements Serializable { private static final long serialVersionUID = 1L; private int id; private transient double weight; private String name; public Student(int id, String name, double weight) { this.id = id; this.name = name; this.weight = weight; } public int getId() { return id; } public String getName() { return name; } public double getWeight() { return weight; } } package com.fqy.serial; import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectOutputStream; public class Persist { public static void main(String[] args) { Student stu = new Student(985, "fqy", 62.0); // Create a fileOutputStream to write to the file with a specified name try { FileOutputStream fout = new FileOutputStream("fout.txt"); // Create an ObjectOutstream that writes to the specified // OutputStream ObjectOutputStream oos = new ObjectOutputStream(fout); // Write the specified object to the ObjectOutputStream oos.writeObject(stu); oos.flush(); oos.close(); System.out.println("success"); } catch (IOException ex) { ex.printStackTrace(); } } } package com.fqy.serial; import java.io.FileInputStream; import java.io.IOException; import java.io.ObjectInputStream; public class Depersist { public static void main(String[] args) { Student stu = null; try { FileInputStream fis = new FileInputStream("fout.txt"); ObjectInputStream ois = new ObjectInputStream(fis); stu = (Student) ois.readObject(); ois.close(); } catch (IOException i) { i.printStackTrace(); return; } catch (ClassNotFoundException c) { System.out.println("Student class not found."); c.printStackTrace(); return; } /* * The value of the weight field was 62.0 when the object was * serialized, but because the field is transient, this value was not * sent to the output stream. The weight field of the deserialized * Student object is 0. */ System.out.println(stu.getName() + " " + stu.getId() + " " + stu.getWeight()); } } //Running result: fqy 985 0.0
相关文章推荐
- java之序列化Serialization 机制
- Java序列化(Serialization)
- Java Serialization 序列化
- Thinking in java 之'数据初始化'
- java中对象序列化(Serialization)的注意事项
- 浅析Java Object Serialization与 Hadoop 序列化
- Java Serialization序列化与反序列化
- JAVA-基础(六) Java.serialization 序列化
- 怎样做才能让Java 序列化机制 更安全 ? Security principles we follow to make Java Serialization safe.
- Java Serialization/序列化/反序列化
- Java源码——对象序列化(对象的存储及读取)(Object Serialization)
- 回首Java——Java序列化机制(Serialization,Deserialization)
- 深入分析Java的序列化(Serialization)
- java serialization/deserialization (序列化对象自描述)
- Java Serialization序列化机制-学习笔记
- java中的序列化 serialization
- Java 序列化综述-由《Efficient Java serialization》说起
- Java Serialization/序列化/反序列化
- 探讨和比较Java和_NET的序列化_Serialization_框架
- Java 网络编程 之 传输对象 Serialization 序列化