Professional JS(三.Language basic-syntax&keywords 4000 &reserved&variables&half data type)&黑画(5)
2017-07-01 16:55
357 查看
3.1-Syntax
1.case-sensitivity(variables,function names,operators).
2.Identifiers:
①An identifier is the name of a variable,function,property or function argument.
②Identifiers may be one or more characters in the following format:
a)The first character must be a letter,an underscore'_' or a dollar sign'$'.
b)All other characters may be letters,underscores,dollar signs or numbers.
③By convention(按照惯例),ECMAScript identifiers use camel case,meaning that the first letter is lowercase and each additional word is offset by a capital letter,like this:firstSecond,myCar,oneTwoThree.
④keywords,reserved words(保留字),true,false,null cannot be used as identifiers.
3.Comment:(single-line&block comments)
①A single-line comment begins with two forward-slash characters,such as this://single line comment.
②A block comment begins with a forward slash and asterisk'*' and ends with the opposite.
4.Strict Mode:
①A different parsing(解析) and execution model for JS,where some of the erratic(不稳定的) behavior of ECMAScript 3 is addressed(解决) and errors are thrown for unsafe activities.
②"use strict",Although this may look like a string that isn't assigned to a variable(一个没有被赋值的变量),this is a pragma(编译指示) that tells supporting JS engines to change into strict mode.
③You may also specify just a function to execute in strict mode by including the pragma at the top of the funciton body:
④Internet Explorer 10+,Firefox 4+,Safari 5.1+,Opera 12+ and Chrome support strict mode.
5.Statements:
①Statements in ECMAScript are terminated by a semicolon(分号),though omitting the semicolon make the parser(解析器) determine where the end of a statement occurs.
②var sum=a+b //valid even without a semicolon ---not recommended
var diff=a-b;//valid ---preferred
③Multiple statements can be combined into a code block by using C-style syntax,beginning with a left curly brace'{' and ending with a right curly brace'}'.
6.Keywords&reserved words:
①have some specific uses,such as indicating the beginning or end of control statements or performing(执行) specific operations(操作).
②reserved words:be reserved for future as keywords.
③Keywords:break/case/catch/continue/debugger/default/delete/do/else/finally/for/function/if/in/instanceof/new/return/switch/this/throw/try/typeof/var/void/while/with.
④Generally speaking,it's best to avoid using both keywords and reserved words as both identifiers and property names to ensure compatibility(兼容性) with past and future ECMAScript editions(note eval&arguments).
7.Variables:
①Every variables is simply a named placeholder(占位符) for a value.
var yyc; //without initialization it holds the special value undefined
②Though it's not recommended to switch data type that a variable contains,it is completely valid(合法的) in ECMAScript.
③Possible to define a variable globally by simply omitting the var opearator.
④As soon as the function test() is called,the variable is defined and becomes accessible outside of the function once it has been executed.
⑤Although it's possible to define global variables by omitting the var operator,this approach is not
recommended.Global variables defines locally hard to maintain and cause confusion,because it's not immediately apparent of the omission of var was intentional(有意的).Strict mode throws a ReferenceError when undeclared variable
is assigned a value.
⑥Because ECMAScript is loosely typed(松散类型的),variable initialization using different data type may be combined into a single statement,separating each variable with a comma','.
⑦Though inserting line breaks and indenting(缩进) the variables isn't necessary,it helps to improve readability.
8.Data Types:
①Five simple data type(also called primitive types[基本类型]):Undefined,Boolean,Null,Number,String.
*②There is also one complex data type called Object,which is an unordered list of name-value pairs.
③Because ECMAScript is loosely typed,there needs to be a way to determine the data type of a given variable(use typeof operator).
④Note that because typeof is an operator and not a function,no parentheses(圆括号) are required(although they can be used).
⑤Calling typeof null returns a value of "object",as the special value null is considered to be an empty object reference.
⑥Calling typeof RegExp returns a value of "function".(RegExp:Regular Expression)
⑦Technically,functions are considered objects in ECMAScript and don't represent another data type.However,they do have some special properties,which necessitates differentiating between functions and other objects via the typeof operator.(通过typeof操作符来区分函数和其他对象是有必要的)
9.The Undefined Type:
*①The Undefined type has only one value,which is the special value undefined.When a variable is declared using var but not initialization,it is assigned the value of undefined.
②By default,any uninitialized variable gets the value of undefined.
③Generally speaking,you should never explicitly set a variable to be undefined.The literal undefined value is provided mainly for comparison and was't added until ECMA-262,third edition to help formalize the difference between an empty object pointer(null)
and an uninitialized variable.
④Note that a variable containing the value of undefined is different from a variable that hasn't been defined at all.
⑤Only one useful operation can be performed on an undeclared variable,you can call typeof on it(calling delete on an undeclared variable won't cause an error,but this isn't very useful and in fact throws an error in strict mode.
*⑥The typeof operator returns "undefined" when called on an uninitialized variable,but it also returns "undefined" when called on an undeclared variable.(typeof未初始化和未声明的变量,结果都是"undefined").Logically,this make sense(说得通) because no real operations can be performed
with either variable even though they are technically very different.
⑦Even though uninitialized variables are automatically assigned a value of "undefined",it is advisable to always initialize variables.That way,when typeof returns "undefined",you'll know that it's because a given variable hasn't been declared rather than simply
not initialized.(即使为初始化的变量会被自动赋值为undefined,但养成初始化变量的习惯仍是个明智的选择。这样以来,当一个变量的类型返回为undefined时,你就知道该变量是未被声明的,而不是为初始化的)
10.The Null Type:
①The Null type is the second data type that has only one value:the special value null.
②Logically,a null value is an empty object pointer,which is why typeof returns "object" when it's passed a null value in the following example.
③When defining a variable that is meant to later hold an object,it is advisable to initialize the variable to null as opposed to anything else.
④That way,you can explicitly check for the value null to determine if the variable has been filled with an object reference at a later time.
⑤The value undefined is a derivative(派生) of null,so ECMA-262 defines them to be superficially(表面地) equal.
11.The Boolean Type:
①The Boolean type has only two literal values:true and false.
②
③It's important to understand what variable you're using in a flow-control statement because of this automatic conversion(转换).
④Mistakenly using an object instead of a Boolean can drastically(彻底地) alter the flow of your application.
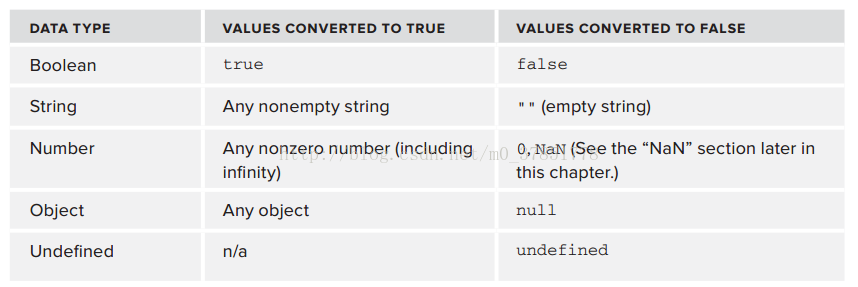
黑客与画家(5.another road)
1.目标客户:
①对于公司哪些事情可以外包,哪些不行?
公司内部所有不直接接受到竞争压力的部门都应外包出去,聘请专门的公司来打理。
②索要高价的人将更多的钱投入推销。
③我们的宣传费每月只有300美元,无法派遣一个衣冠楚楚、言之凿凿的团队到客户公司做演示。
2.桌面电脑:
①桌面电脑当做一台服务器。
②BSD:Berkeley Software Distribution.
单词整理:
define/valid有效的,合法的/implement实现/call out说明/looser syntax/built-in functionality/pseudolanguage伪语言/adhere依附,追随/forward-slash'/'/readability/enterprise/erratic不稳定的/entire/specifically/specify/intent意图/indent缩进/semicolon分号/parser/omission遗漏,省略/compress压缩/white
space/performance性能/error-prone显示错误/set/denoted标记/asterisk星号/specification规则,说明书/represent表示/numeric/identical完全相同的/satellite卫星
1.case-sensitivity(variables,function names,operators).
2.Identifiers:
①An identifier is the name of a variable,function,property or function argument.
②Identifiers may be one or more characters in the following format:
a)The first character must be a letter,an underscore'_' or a dollar sign'$'.
b)All other characters may be letters,underscores,dollar signs or numbers.
③By convention(按照惯例),ECMAScript identifiers use camel case,meaning that the first letter is lowercase and each additional word is offset by a capital letter,like this:firstSecond,myCar,oneTwoThree.
④keywords,reserved words(保留字),true,false,null cannot be used as identifiers.
3.Comment:(single-line&block comments)
①A single-line comment begins with two forward-slash characters,such as this://single line comment.
②A block comment begins with a forward slash and asterisk'*' and ends with the opposite.
4.Strict Mode:
①A different parsing(解析) and execution model for JS,where some of the erratic(不稳定的) behavior of ECMAScript 3 is addressed(解决) and errors are thrown for unsafe activities.
②"use strict",Although this may look like a string that isn't assigned to a variable(一个没有被赋值的变量),this is a pragma(编译指示) that tells supporting JS engines to change into strict mode.
③You may also specify just a function to execute in strict mode by including the pragma at the top of the funciton body:
function doSomething(){ "use strict" //function body }
④Internet Explorer 10+,Firefox 4+,Safari 5.1+,Opera 12+ and Chrome support strict mode.
5.Statements:
①Statements in ECMAScript are terminated by a semicolon(分号),though omitting the semicolon make the parser(解析器) determine where the end of a statement occurs.
②var sum=a+b //valid even without a semicolon ---not recommended
var diff=a-b;//valid ---preferred
③Multiple statements can be combined into a code block by using C-style syntax,beginning with a left curly brace'{' and ending with a right curly brace'}'.
6.Keywords&reserved words:
①have some specific uses,such as indicating the beginning or end of control statements or performing(执行) specific operations(操作).
②reserved words:be reserved for future as keywords.
③Keywords:break/case/catch/continue/debugger/default/delete/do/else/finally/for/function/if/in/instanceof/new/return/switch/this/throw/try/typeof/var/void/while/with.
④Generally speaking,it's best to avoid using both keywords and reserved words as both identifiers and property names to ensure compatibility(兼容性) with past and future ECMAScript editions(note eval&arguments).
7.Variables:
①Every variables is simply a named placeholder(占位符) for a value.
var yyc; //without initialization it holds the special value undefined
②Though it's not recommended to switch data type that a variable contains,it is completely valid(合法的) in ECMAScript.
③Possible to define a variable globally by simply omitting the var opearator.
④As soon as the function test() is called,the variable is defined and becomes accessible outside of the function once it has been executed.
function test(){ message="hi";//global variable } test();
⑤Although it's possible to define global variables by omitting the var operator,this approach is not
recommended.Global variables defines locally hard to maintain and cause confusion,because it's not immediately apparent of the omission of var was intentional(有意的).Strict mode throws a ReferenceError when undeclared variable
is assigned a value.
⑥Because ECMAScript is loosely typed(松散类型的),variable initialization using different data type may be combined into a single statement,separating each variable with a comma','.
⑦Though inserting line breaks and indenting(缩进) the variables isn't necessary,it helps to improve readability.
8.Data Types:
①Five simple data type(also called primitive types[基本类型]):Undefined,Boolean,Null,Number,String.
*②There is also one complex data type called Object,which is an unordered list of name-value pairs.
③Because ECMAScript is loosely typed,there needs to be a way to determine the data type of a given variable(use typeof operator).
④Note that because typeof is an operator and not a function,no parentheses(圆括号) are required(although they can be used).
⑤Calling typeof null returns a value of "object",as the special value null is considered to be an empty object reference.
⑥Calling typeof RegExp returns a value of "function".(RegExp:Regular Expression)
⑦Technically,functions are considered objects in ECMAScript and don't represent another data type.However,they do have some special properties,which necessitates differentiating between functions and other objects via the typeof operator.(通过typeof操作符来区分函数和其他对象是有必要的)
9.The Undefined Type:
*①The Undefined type has only one value,which is the special value undefined.When a variable is declared using var but not initialization,it is assigned the value of undefined.
②By default,any uninitialized variable gets the value of undefined.
③Generally speaking,you should never explicitly set a variable to be undefined.The literal undefined value is provided mainly for comparison and was't added until ECMA-262,third edition to help formalize the difference between an empty object pointer(null)
and an uninitialized variable.
④Note that a variable containing the value of undefined is different from a variable that hasn't been defined at all.
var message; console.log(message);//undefined console.log(message1); //error
⑤Only one useful operation can be performed on an undeclared variable,you can call typeof on it(calling delete on an undeclared variable won't cause an error,but this isn't very useful and in fact throws an error in strict mode.
*⑥The typeof operator returns "undefined" when called on an uninitialized variable,but it also returns "undefined" when called on an undeclared variable.(typeof未初始化和未声明的变量,结果都是"undefined").Logically,this make sense(说得通) because no real operations can be performed
with either variable even though they are technically very different.
⑦Even though uninitialized variables are automatically assigned a value of "undefined",it is advisable to always initialize variables.That way,when typeof returns "undefined",you'll know that it's because a given variable hasn't been declared rather than simply
not initialized.(即使为初始化的变量会被自动赋值为undefined,但养成初始化变量的习惯仍是个明智的选择。这样以来,当一个变量的类型返回为undefined时,你就知道该变量是未被声明的,而不是为初始化的)
10.The Null Type:
①The Null type is the second data type that has only one value:the special value null.
②Logically,a null value is an empty object pointer,which is why typeof returns "object" when it's passed a null value in the following example.
var yyc=null; typeof yyc;//"object"
③When defining a variable that is meant to later hold an object,it is advisable to initialize the variable to null as opposed to anything else.
④That way,you can explicitly check for the value null to determine if the variable has been filled with an object reference at a later time.
if(yyc!=null){ //do something with yyc. }
⑤The value undefined is a derivative(派生) of null,so ECMA-262 defines them to be superficially(表面地) equal.
null==undefined;//true⑥Any time an object is expected but is not available,null should be used in its place.(只要意在保存对象的变量,即使现在还没真正保存对象,也应让该变量保存null值)
11.The Boolean Type:
①The Boolean type has only two literal values:true and false.
②
var message="Hello world!"; if(message){ alert("value is true"); }In this example,the alert will be displayed because the string message is automatically converted into its Boolean equivalent(true).
③It's important to understand what variable you're using in a flow-control statement because of this automatic conversion(转换).
④Mistakenly using an object instead of a Boolean can drastically(彻底地) alter the flow of your application.
黑客与画家(5.another road)
1.目标客户:
①对于公司哪些事情可以外包,哪些不行?
公司内部所有不直接接受到竞争压力的部门都应外包出去,聘请专门的公司来打理。
②索要高价的人将更多的钱投入推销。
③我们的宣传费每月只有300美元,无法派遣一个衣冠楚楚、言之凿凿的团队到客户公司做演示。
2.桌面电脑:
①桌面电脑当做一台服务器。
②BSD:Berkeley Software Distribution.
单词整理:
define/valid有效的,合法的/implement实现/call out说明/looser syntax/built-in functionality/pseudolanguage伪语言/adhere依附,追随/forward-slash'/'/readability/enterprise/erratic不稳定的/entire/specifically/specify/intent意图/indent缩进/semicolon分号/parser/omission遗漏,省略/compress压缩/white
space/performance性能/error-prone显示错误/set/denoted标记/asterisk星号/specification规则,说明书/represent表示/numeric/identical完全相同的/satellite卫星
相关文章推荐
- long long data type in GCC and what's the meaning of 1LL
- struts中post方式上传文件,enctype="multipart/form-data",request.getParameter("file") 是null的问题分析
- _BLOCK_TYPE_VALID(pHead->nBlockUse),_CrtIsValidHeapPointer(pUserData),动态链接库内存分配
- FORM的ENCTYPE="multipart/form-data" 时request.getParameter()值为null问题的解决
- enctype="multipart/form-data" 作用
- 解决当FORM的ENCTYPE="multipart/form-data" 时request.getParameter()获取不到值的方法
- vc下error C2011: 'DataTypeEnum' : 'enum' type redefinition解决方法
- 2 Datatype and variables in VBScript
- php在form表单ENCTYPE="multipart/form-data" 不用转换编码
- ENCTYPE="multipart/form-data"用于表单里有图片上传
- 解决当FORM的ENCTYPE="multipart/form-data" 时request.getParameter()获取不到值的方法
- struts中enctype="multipart/form-data",request.getParameter("file") 是null的问题分
- enctype="multipart/form-data"
- 当form为enctype="multipart/form-data"时的代码
- 文件上传时异常Invalid field value for field "**"与enctype="multipart/form-data"
- 解决当FORM的ENCTYPE="multipart/form-data" 时request.getParameter()获取不到值的方法
- form表单中的enctype="multipart/form-data"
- PL/SQL教程(二)Data&Variables
- The content of element type "struts-config" must match "(data-sources?,form-
- ADO.NET Entity Framework: The version of SQL Server in use does not support datatype 'datetime2'