Java基础3
2017-06-26 11:40
232 查看
Java形式参数问题-类型名、抽象类、接口
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
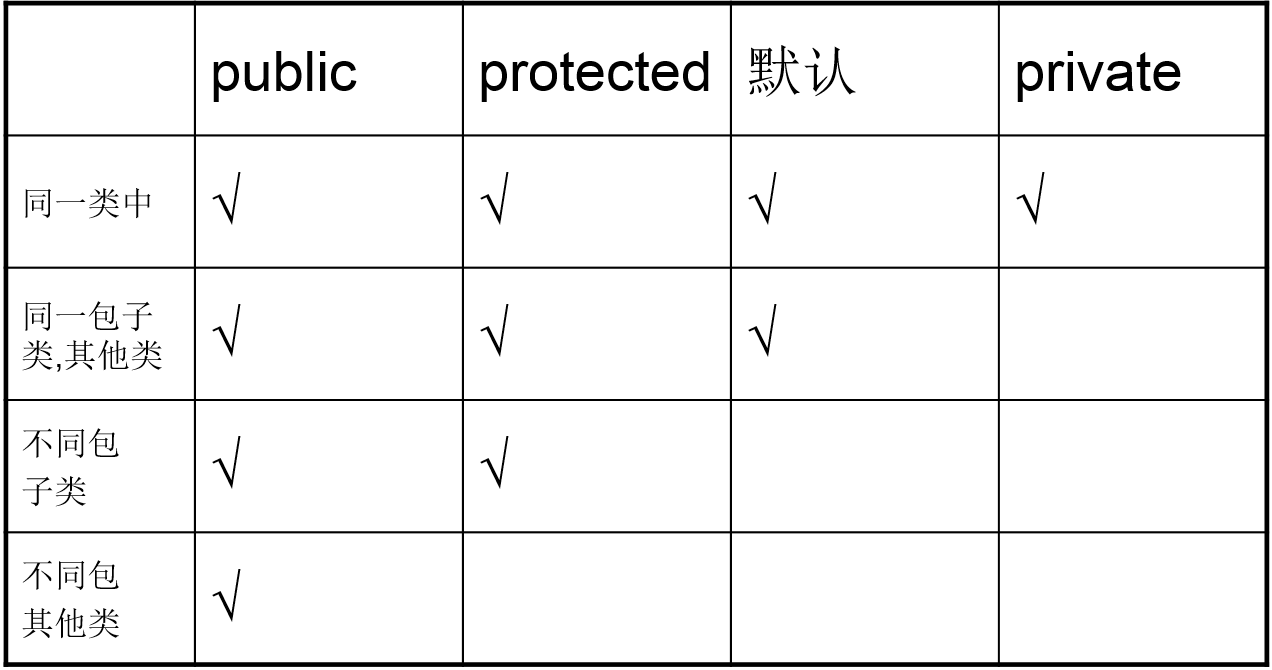
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
2种形式参数类型
形式参数:
引用类型:
形式参数: 基本类型(太简单) 引用类型 类名:(匿名对象的时候其实我们已经讲过了)需要的是该类的对象 <StudentTest > 抽象类:需要的是该抽象的类子类对象 <PersonTest> 接口:需要的是该接口实现类对象 <TeacherTest>
类名作为形式参数-代码块
// <1>类作为形式参数 class Student{ public void study(){ System.out.println("Good Good Study,Day Day Up!"); } } class StduentDemo{ public void method(Student s){ s.study(); } } public class StudentTest { public static void main(String[] args) { StduentDemo sd = new StduentDemo(); Student ss =new Student(); sd.method(ss); } }1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
抽象类作为形式参数-代码块
// <2>抽象类作为形式参数 //定义一个抽象类 abstract class Person { public abstract void study(); } class PersonDemo { public void method(Person p){ //真正需要的是该抽象类的子类对象,因为本身不能实例化 p.study(); } } //定义一个具体的学生类 class Student extends Person { public void study() { System.out.println("Good Good Study,Day Day Up"); } } class PersonTest{ public static void main(String[] args) { //需求:我要测试Person类中的study()方法 PersonDemo pd = new PersonDemo(); // Person p = new Student(); //Person p = new Student(); 多态 Person p = new Student(); //传入参数为Person类,因为是抽象类不可以直接实例化,需要间接实例化 pd.method(p); } }1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
接口作为形式参数-代码块
// <3>接口作为形式参数 interface Love{ public abstract void love(); } class LoveDemo{ public void method(Love l){ //Love l=new Teacher(); 多态 l.love(); } } class Teacher implements Love{ //接口的实现类对象 public void love(){ System.out.println("老师爱学生"); } } public class TeacherTest{ public static void main(String[] args) { // 测试LoveDemo类中的love()方法 LoveDemo ld=new LoveDemo(); Love l=new Teacher(); //接口:需要的是该接口的实现类对象 ld.method(l); } }-------------------------------------------------
Java返回值-类、抽象类、接口
返回值类型
返回值类型 基本类型:(基本类型太简单,我不准备讲解) 引用类型: 类:返回的是该类的对象 抽象类:返回的是该抽象类的子类对象 接口:返回的是该接口的实现类的对象
类名作为返回值-代码块
// <1>类作为返回值 class Student { public void study() { System.out.println("Good Good Study,Day Day Up"); } } class StudentDemo { public Student getStudent() { //Student s = new Student(); //return s; return new Student(); } } class StudentTest2 { public static void main(String[] args) { StudentDemo sd = new StudentDemo(); Student s = sd.getStudent(); //new Student(); Student s = new Student(); s.study(); } }1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
抽象类作为返回值-代码块
// <2> 抽象类作为返回值 abstract class Person { public abstract void study(); } class PersonDemo { public Person getPerson() { //Person p = new Student(); //return p; return new Student(); } } class Student extends Person { public void study() { System.out.println("Good Good Study,Day Day Up"); } } class PersonTest2 { public static void main(String[] args) { //需求:我要测试Person类中的study()方法 PersonDemo pd = new PersonDemo(); Person p = pd.getPerson(); //new Student(); Person p = new Student(); 多态 p.study(); } }1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
接口作为返回值-代码块
// <3> 接口作为返回值 //定义一个说的的接口 interface speak{ public abstract void speakeng(); } class teacher implements speak{ public void speakeng(){ System.out.println("I can speak!"); } } class teacherDemo{ public speak show(){ return new teacher(); //返回的是该接口的实现类的对象 } } public class TeacherTest4 { public static void main(String[] args) { teacherDemo td =new teacherDemo(); speak l= td.show(); //new teacher(); speak l=new teacher(); 多态 l.speakeng(); } }--------------------------------------------------------
链式编程。 每次调用完毕方法后,返回的是一个对象。
链式连接-代码块
// <1>类作为返回值 class Student { public void study() { System.out.println("Good Good Study,Day Day Up"); } } class StudentDemo { public Student getStudent() { return new Student(); } } class StudentTest3 { public static void main(String[] args) { //如何调用的呢? StudentDemo sd = new StudentDemo(); //Student s = sd.getStudent(); //s.study(); //大家注意了 sd.getStudent().study(); //返回的是对象,所以可以再次调用方法 } }-----------------
类及其组成可以用的修饰符
权限修饰符
Java 类及其组成可以用的修饰符
修饰符: 权限修饰符:private,默认的,protected,public 状态修饰符:static,final 抽象修饰符:abstract 类: 权限修饰符:默认修饰符,public 状态修饰符:final 抽象修饰符:abstract 用的最多的就是:public 成员变量: 权限修饰符:private,默认的,protected,public 状态修饰符:static,final 用的最多的就是:private 构造方法: 权限修饰符:private,默认的,protected,public 用的最多的就是:public 成员方法: 权限修饰符:private,默认的,protected,public 状态修饰符:static,final 抽象修饰符:abstract 用的最多的就是:public 除此以外的组合规则: 成员变量:public static final 成员方法:public static public abstract public final
代码块
public class Demo { //成员变量 private int x = 10; int y = 20; protected int z = 30; public int a = 40; public final int b = 50; public static int c = 60; public static final int d = 70; //此处不允许使用修饰符abstract //abstract int e = 80; //构造方法 private Demo(){} Demo(String name){} protected Demo(String name,int age) {} public Demo(String name,int age,String address) {} //此处不允许使用修饰符static //public static Demo(){} //此处不允许使用修饰符final //public final Demo() {} //此处不允许使用修饰符abstract //public abstract Demo(){} //成员方法 //static void show() {} //abstract void show(); //final void show(){} }---------------------------------------------------------------------
成员内部类
成员内部类: <1>外部类名.内部类名 对象名 = 外部类对象.内部类对象; Outer.Inner oi = new Outer().new Inner(); <2>成员内部类被静态修饰后的访问方式是: //格式:外部类名.内部类名 对象名 = new 外部类名.内部类名(); Outer.Inner oi = new Outer.Inner();
成员内部类的代码块1
//访问内部类成员 class Outer { private int num = 10; class Inner { public void show() { System.out.println(num); } } } class InnerClassDemo3 { public static void main(String[] args) { //需求:我要访问Inner类的show()方法 //格式:外部类名.内部类名 对象名 = 外部类对象.内部类对象; Outer.Inner oi = new Outer().new Inner(); oi.show(); } }1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
成员内部类(被静态修饰)的代码块2
//成员内部类(静态类型) class Outer { private int num = 10; private static int num2 = 100; //内部类用静态修饰是因为内部类可以看出是外部类的成员 public static class Inner { public void show() { //System.out.println(num); System.out.println(num2); } public static void show2() { //System.out.println(num); System.out.println(num2); } } } class InnerClassDemo4 { public static void main(String[] args) { //使用内部类 // 限定的新静态类 //成员内部类被静态修饰后的访问方式是: //格式:外部类名.内部类名 对象名 = new 外部类名.内部类名(); // Outer.Inner oi = new Outer().new Inner(); Outer.Inner oi = new Outer.Inner(); // oi.show(); oi.show2(); //show2()的另一种调用方式 Outer.Inner.show2(); } }1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
练习题
//访问内部类成员 class Outer{ public int num=10; class Inner{ public int num=20; public void show(){ int num=30; System.out.println(num); System.out.println(this.num); System.out.println(new Outer().num); //通过对象去访问 System.out.println(Outer.this.num); //通过外部类限制this } } } public class InnerClassTest { public static void main(String[] args) { Outer.Inner oi = new Outer().new Inner(); //访问Inner类的show()方法 oi.show(); } }-------------------------------------------------------------------------------
相关文章推荐
- Java(JVM)虚拟机结构基础,和JAR文件包及jar命令详解
- Java动画编程基础第二部分
- 回复:学习java必须有c++基础么?
- (原创) 脚踏实地学Java之:基础篇
- Java 应了解的基础知识(转载)
- 基于Java的动画编程基础
- Java 101之线程基础
- Java Swing入门基础
- Java Swing入门基础 (转)
- Java动画编程基础第一部分
- Java基础之关键字
- 学java也快半年了,一直在看基础的东西,终于决定开始转J2ee了……
- Eclipse基础--java环境变量设置
- 第二讲 Java语言基础知识
- Java动画编程基础第四部分
- JAVA调试技术--基础
- Java初学及基础问题
- JAVA面试基础试题[转贴]
- Java 应了解的基础知识(转载)
- JAVA相关基础知识(1)