iOS 绘制简单图形---UIBezierPath篇
2017-06-23 13:57
316 查看
****************贝塞尔篇****************
一、 关于UIBezierPath
UIBezierPath这个类在UIKit中, 是Core Graphics框架关于path的一个封装,使用此类可以定义简单的形状,比如我们常用到,矩形,圆形,椭圆,弧,或者不规则的多边形。
二、使用步骤
1、 重写View的drawRect方法
2、 创建UIBezierPath的对象
3、 使用方法moveToPoint: 设置初始点
4、 根据具体要求使用UIBezierPath类方法绘图(比如要画线、矩形、圆、弧等)
5、 设置UIBezierPath对象相关属性 (比如lineWidth、lineJoinStyle、aPath.lineCapStyle、color)
6、 使用stroke 或者 fill方法结束绘图
三、初始化
初始化bezierPath对象
快速获取一个 矩形 bezierPath对象
快速获取一个 椭圆 bezierPath对象
快速获取一个 圆角矩形 bezierPath对象
快速获取一个 圆角可选矩形 bezierPath对象
快速获取一个 圆弧 bezierPath对象
使用 CGPath 创建一个bezierPath对象
四、绘制路径
绘制直线
圆弧
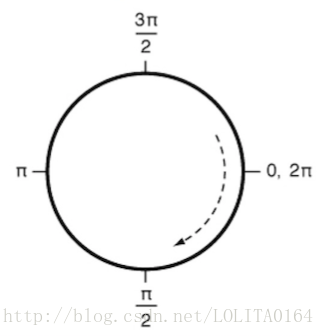
圆弧坐标系
二次贝塞尔曲线
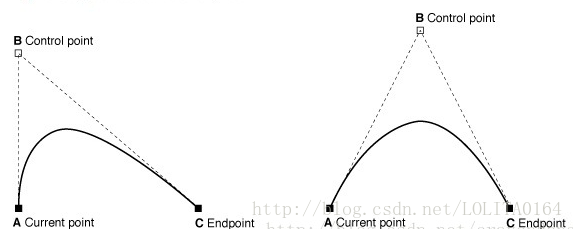
三次贝塞尔曲线
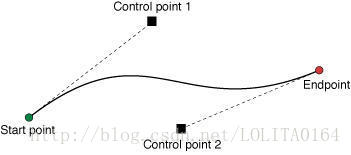
其他方法
五、path相关属性信息
路径的一些信息(readonly)
绘制中可以设置的相关属性
六、绘制方式
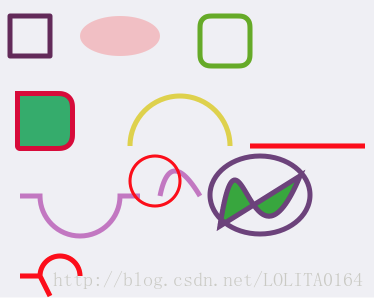
简单绘制效果
七、应用
Demo传送门
1、自定义弹框
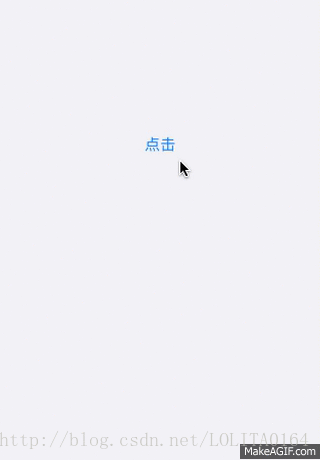
2、画板
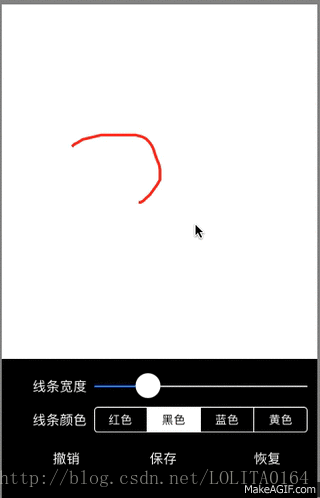
八、参考地址
1、iOS开发之画图板(贝塞尔曲线)
一、 关于UIBezierPath
UIBezierPath这个类在UIKit中, 是Core Graphics框架关于path的一个封装,使用此类可以定义简单的形状,比如我们常用到,矩形,圆形,椭圆,弧,或者不规则的多边形。
二、使用步骤
1、 重写View的drawRect方法
2、 创建UIBezierPath的对象
3、 使用方法moveToPoint: 设置初始点
4、 根据具体要求使用UIBezierPath类方法绘图(比如要画线、矩形、圆、弧等)
5、 设置UIBezierPath对象相关属性 (比如lineWidth、lineJoinStyle、aPath.lineCapStyle、color)
6、 使用stroke 或者 fill方法结束绘图
三、初始化
初始化bezierPath对象
UIBezierPath *path = [UIBezierPath bezierPath];
快速获取一个 矩形 bezierPath对象
// frame UIBezierPath *path = [UIBezierPath bezierPathWithRect:CGRectMake(10, 20, 40, 40)];
快速获取一个 椭圆 bezierPath对象
// 宽度相等则为圆形 UIBezierPath *path = [UIBezierPath bezierPathWithOvalInRect:CGRectMake(80, 20, 80, 40)];
快速获取一个 圆角矩形 bezierPath对象
// 参数一: 矩形范围 // 参数二: 圆角半径, 如果半径大于矩形短边的一半, 则按照一半处理 UIBezierPath *path = [UIBezierPath bezierPathWithRoundedRect:CGRectMake(200, 20, 50, 50) cornerRadius:10];
快速获取一个 圆角可选矩形 bezierPath对象
// 参数一: 矩形范围 // 参数二: 圆弧位置, 可以多选 // 参数三: 圆弧半径 取最小 UIBezierPath *path = [UIBezierPath bezierPathWithRoundedRect:CGRectMake(20, 100, 50, 50) byRoundingCorners:UIRectCornerTopRight|UIRectCornerBottomRight cornerRadii:CGSizeMake(10, 20)];
快速获取一个 圆弧 bezierPath对象
// 参数一: 圆弧圆心 // 参数二: 圆弧半径 // 参数三: 开始弧度 // 参数四: 结束弧度 // 参数五: 是否为顺时针 UIBezierPath *path = [UIBezierPath bezierPathWithArcCenter:CGPointMake(180, 150) radius:50 startAngle:M_PI endAngle:0 clockwise:YES];
使用 CGPath 创建一个bezierPath对象
// + (instancetype)bezierPathWithCGPath:(CGPathRef)CGPath;
四、绘制路径
绘制直线
// 1、初始化 UIBezierPath *path = [UIBezierPath bezierPath]; // 2、设置路径 [path moveToPoint:CGPointMake(250, 150)]; //起点 [path addLineToPoint:CGPointMake(kScreenWidth-10, 150)]; //移动到下一个点 // 3、设置属性 // 4、渲染绘图 [path stroke];
圆弧
圆弧坐标系
// 1、初始化 UIBezierPath *path = [UIBezierPath bezierPath]; // 2、设置路径 [path moveToPoint:CGPointMake(20, 200)]; //起点 // 参数一: 圆弧圆心 // 参数二: 圆弧半径 // 参数三: 开始弧度 // 参数四: 结束弧度 // 参数五: 是否为顺时针 [path addArcWithCenter:CGPointMake(80, 200) radius:40 startAngle:M_PI endAngle:0 clockwise:NO]; // 3、设置属性 // 4、渲染绘图 [path stroke];
二次贝塞尔曲线
// 1、初始化 UIBezierPath *path = [UIBezierPath bezierPath]; // 2、设置路径 [path moveToPoint:CGPointMake(160, 200)]; //起点 // 参数一: 曲线的终点位置 // 参数二: 控制点 [path addQuadCurveToPoint:CGPointMake(200, 200) controlPoint:CGPointMake(170, 150)]; // 3、设置属性 // 4、渲染绘图 [path stroke];
三次贝塞尔曲线
// 1、初始化 UIBezierPath *path = [UIBezierPath bezierPath]; // 2、设置路径 [path moveToPoint:CGPointMake(220, 230)]; //起点 // 参数一: 曲线的终点位置 // 参数二: 第一控制点 // 参数三: 第二控制点 [path addCurveToPoint:CGPointMake(300, 180) controlPoint1:CGPointMake(240, 100) controlPoint2:CGPointMake(250, 300)]; // 3、设置属性 // 4、渲染绘图 [path stroke];
其他方法
-(void)closePath; // 封闭路径,可以自动连接起点和终点 - (void)removeAllPoints; // 移除路径中所有点 - (void)appendPath:(UIBezierPath *)bezierPath; //添加一条路径 - (UIBezierPath *)bezierPathByReversingPath; // 路径反转 当前路径的起点作为当前点 - (void)applyTransform:(CGAffineTransform)transform; // 使用CGAffineTransform
五、path相关属性信息
路径的一些信息(readonly)
// 路径是否为空 @property(readonly,getter=isEmpty) BOOL empty; // 封闭所有路径点的最小矩形范围, 不包括控制点 @property(nonatomic,readonly) CGRect bounds; // 当前的点 @property(nonatomic,readonly) CGPoint currentPoint; // 路径区域中是否包含某个点 - (BOOL)containsPoint:(CGPoint)point;
绘制中可以设置的相关属性
// 线条宽度 @property(nonatomic) CGFloat lineWidth; // 起点终点的样式 /* kCGLineCapButt, // 默认 (方形结束, 结束位置正好为精确位置),如果线条太宽,看起来不完全封闭 kCGLineCapRound, // (圆形结束, 结束位置超过精确位置半个线宽) kCGLineCapSquare // (方形结束, 结束位置超过精确位置半个线宽) */ @property(nonatomic) CGLineCap lineCapStyle; // 线条连接点样式 /* kCGLineJoinMiter, // 默认 kCGLineJoinRound, // 圆形 kCGLineJoinBevel // */ @property(nonatomic) CGLineJoin lineJoinStyle;
六、绘制方式
- (void)fill; // 填充绘图 [[UIColor redColor] setFill]; //设置填充色 - (void)stroke; // 绘制路径 [[UIColor redColor] setStroke]; //设置线条颜色
简单绘制效果
七、应用
Demo传送门
1、自定义弹框
2、画板
八、参考地址
1、iOS开发之画图板(贝塞尔曲线)
相关文章推荐
- iOS --- OpenGLES之简单的图形绘制
- MAC IOS 用openCV 绘制简单图形
- iOS Quartz2D绘制简单图形
- iOS Quartz2D 绘制简单图形--线,圆,弧线,贝塞尔曲线,文字
- IOS之Quartz2D绘图1.简单几何图形绘制
- iOS上使用Quartz 2D绘制简单图形
- iOS开发UI篇—Quartz2D简单图形绘制(二)直线,三角形,矩形,扇形,弧,圆
- iOS上使用Quartz 2D绘制简单图形
- iOS图形绘制UIBezierPath篇
- iOS开发的2D绘制--CoreGraphics的简单使用二(画图形)
- 创建视图并绘制简单图形
- FxCAD实验一 简单图形的绘制
- HTML5 Canvas简单图形绘制[矩形、圆形、线]
- D3D编程之绘制简单图形
- Silverlight 5 3d游戏开发(1)绘制简单图形
- OpenGL_Qt学习笔记之_02(绘制简单平面几何图形)
- OpenPlug Studio应用在iOS的图形绘制效能是Adobe Flash Builder 4.5.1应用的4倍!
- 【Iphone 游戏开发之一】创建视图并绘制简单图形 推荐
- Android中简单图形绘制,及全屏设置
- Windows控制台下绘制简单图形