collection集合的介绍
2017-06-22 00:00
337 查看
摘要: 总结collection集合常用的方法
1. 内容摘要
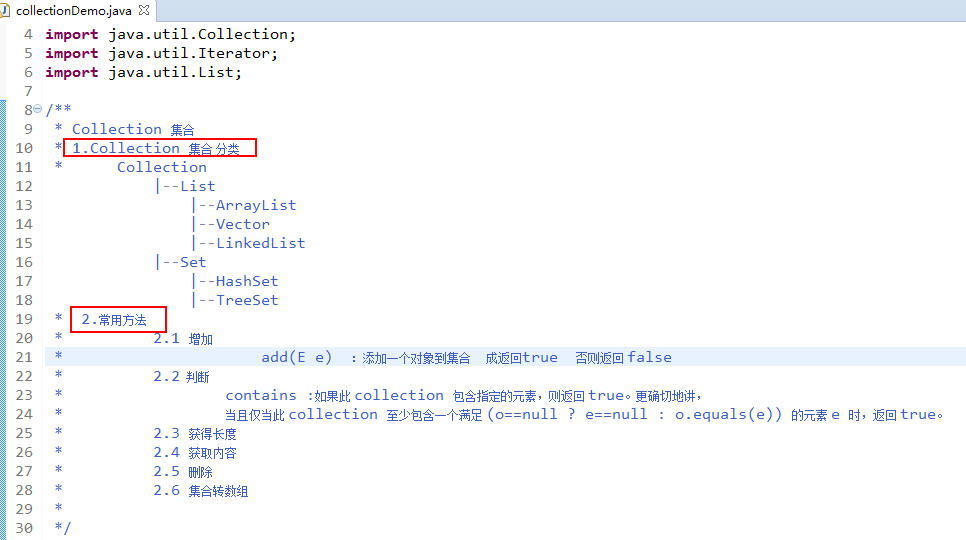
2. collection常用方法
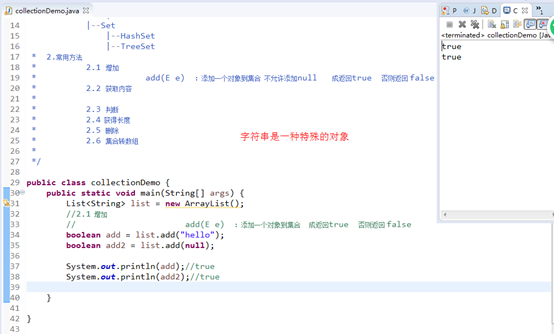
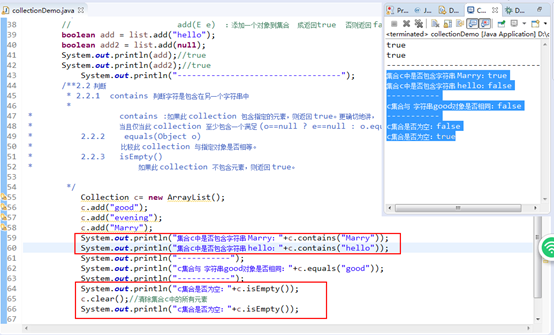
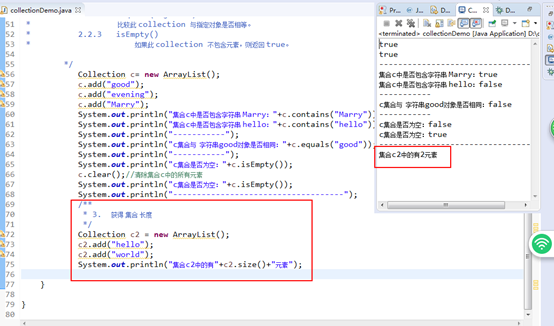
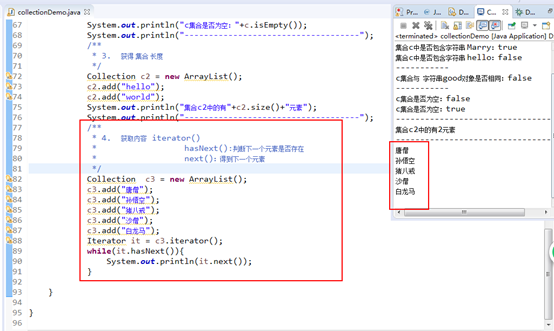
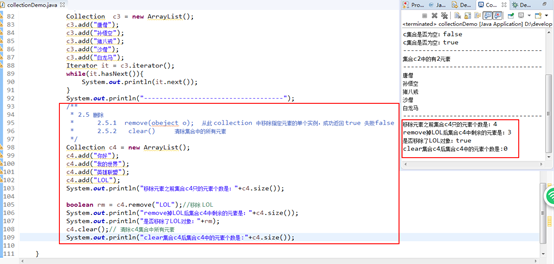
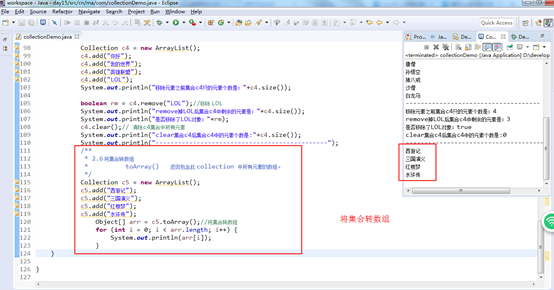
3.代码
package cn.ma.com;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
/**
* Collection 集合
* 1.Collection 集合 分类
* Collection
|--List
|--ArrayList
|--Vector
|--LinkedList
|--Set
|--HashSet
|--TreeSet
* 2.常用方法
* 2.1 增加
* add(E e) :添加一个对象到集合 成返回true 否则返回 false
* 2.2 判断
* contains :如果此 collection 包含指定的元素,则返回 true。更确切地讲,
* 当且仅当此 collection 至少包含一个满足 (o==null ? e==null : o.equals(e)) 的元素 e 时,返回 true。
* 2.3 获得长度
* 2.4 获取内容
* 2.5 删除
* 2.6 集合转数组
*
*/
public class collectionDemo {
public static void main(String[] args) {
List<String> list = new ArrayList();
/**
* 2.1 增加
*
*/
// add(E e) :添加一个对象到集合 成返回true 否则返回 false
boolean add = list.add("hello");
boolean add2 = list.add(null);
System.out.println(add);//true
System.out.println(add2);//true
System.out.println("----------------------------------");
/**2.2 判断
* 2.2.1 contains 判断字符是包含在另一个字符串中
*
* contains :如果此 collection 包含指定的元素,则返回 true。更确切地讲,
* 当且仅当此 collection 至少包含一个满足 (o==null ? e==null : o.equals(e)) 的元素 e 时,返回 true。
* 2.2.2 equals(Object o)
* 比较此 collection 与指定对象是否相等。
* 2.2.3 isEmpty()
* 如果此 collection 不包含元素,则返回 true。
*/
Collection c= new ArrayList();
c.add("good");
c.add("evening");
c.add("Marry");
System.out.println("集合c中是否包含字符串 Marry:"+c.contains("Marry"));
System.out.println("集合c中是否包含字符串 hello:"+c.contains("hello"));
System.out.println("-----------");
System.out.println("c集合与 字符串good对象是否相同:"+c.equals("good"));
System.out.println("-----------");
System.out.println("c集合是否为空:"+c.isEmpty());
c.clear();//清除集合c中的所有元素
System.out.println("c集合是否为空:"+c.isEmpty());
System.out.println("------------------------------------");
/**
* 3. 获得 集合 长度
*/
Collection c2 = new ArrayList();
c2.add("hello");
c2.add("world");
System.out.println("集合c2中的有"+c2.size()+"元素");
System.out.println("------------------------------------");
/**
* 4. 获取内容 iterator()
* hasNext():判断下一个元素是否存在
* next():得到下一个元素
*/
Collection c3 = new ArrayList();
c3.add("唐僧");
c3.add("孙悟空");
c3.add("猪八戒");
c3.add("沙僧");
c3.add("白龙马");
Iterator it = c3.iterator();
while(it.hasNext()){
System.out.println(it.next());
}
System.out.println("------------------------------------");
/**
* 2.5 删除
* 2.5.1 remove(obeject o); 从此 collection 中移除指定元素的单个实例,成功返回 true 失败false
* 2.5.2 clear() 清除集合中的所有元素
*/
Collection c4 = new ArrayList();
c4.add("你好");
c4.add("我的世界");
c4.add("英雄联盟");
c4.add("LOL");
System.out.println("移除元素之前集合c4只的元素个数是:"+c4.size());
boolean rm = c4.remove("LOL");//移除 LOL
System.out.println("remove掉LOL后集合c4中剩余的元素是:"+c4.size());
System.out.println("是否移除了LOL对象:"+rm);
c4.clear();// 清除c4集合中所有元素
System.out.println("clear集合c4后集合c4中的元素个数是:"+c4.size());
System.out.println("----------------------------------------------");
/**
* 2.6 将集合转数组
* toArray() 返回包含此 collection 中所有元素的数组。
*/
Collection c5 = new ArrayList();
c5.add("西游记");
c5.add("三国演义");
c5.add("红楼梦");
c5.add("水浒传");
Object[] arr = c5.toArray();//将集合转数组
for (int i = 0; i < arr.length; i++) {
System.out.println(arr[i]);
}
}
}
4.案列
4.1 学生类
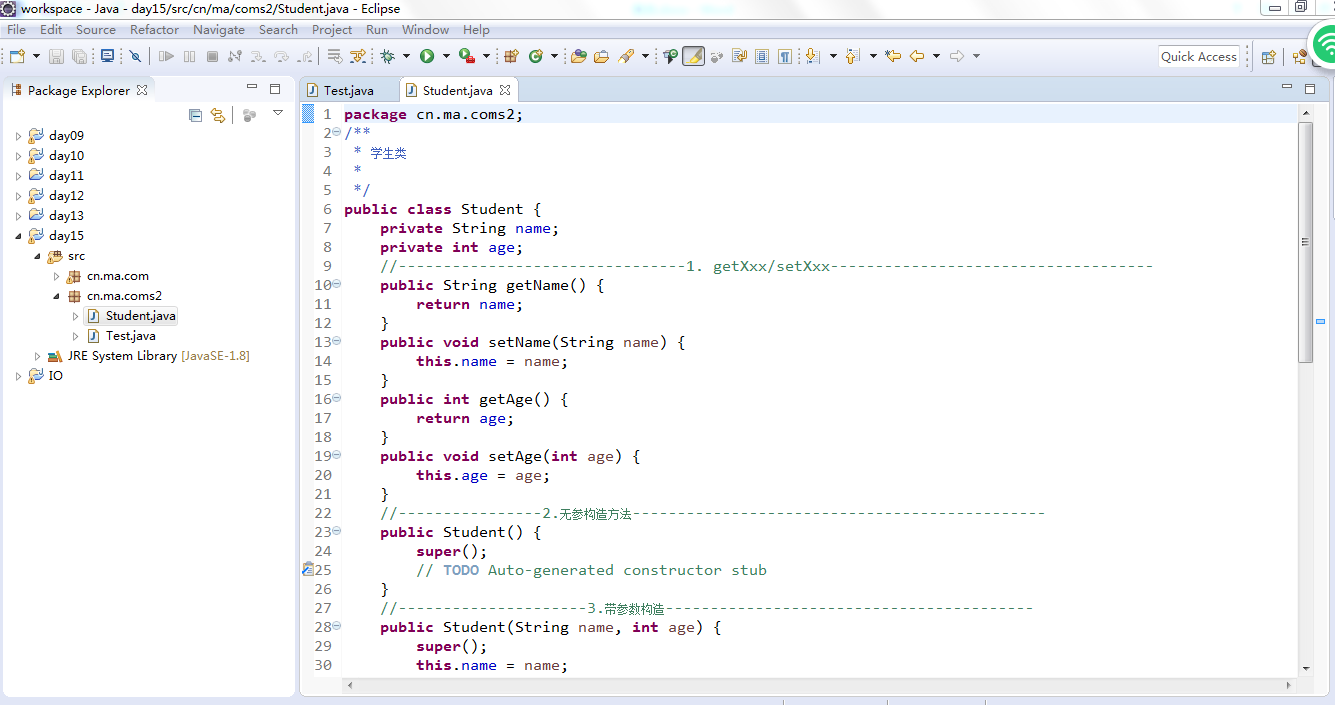
4.2 测试类
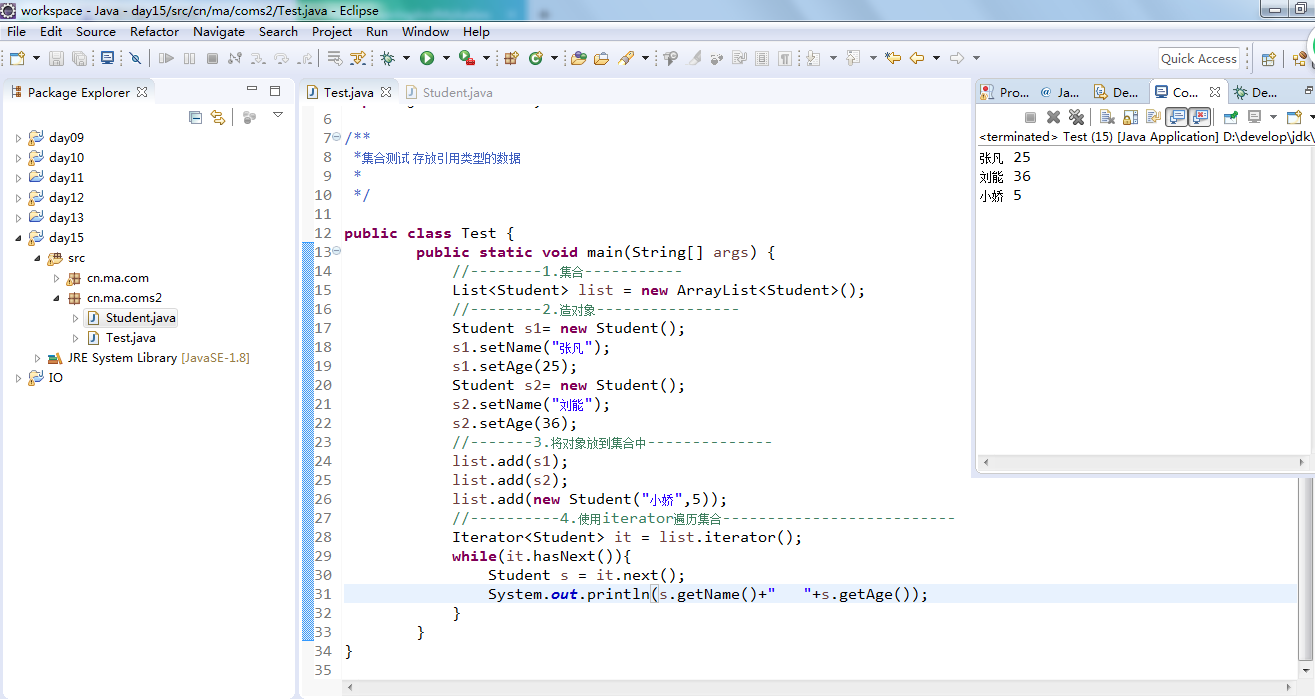
4.3 代码
4.3.1 学生类 代码
package cn.ma.coms2;
/**
* 学生类
*
*/
public class Student {
private String name;
private int age;
//--------------------------------1. getXxx/setXxx------------------------------------
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
//----------------2.无参构造方法----------------------------------------------
public Student() {
super();
// TODO Auto-generated constructor stub
}
//---------------------3.带参数构造-----------------------------------------
public Student(String name, int age) {
super();
this.name = name;
this.age = age;
}
//-------------------4.toString方法------------------------------------------
@Override
public String toString() {
return "Student [name=" + name + ", age=" + age + "]";
}
//----------------------5.hashCode--------------------------------------
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + age;
result = prime * result + ((name == null) ? 0 : name.hashCode());
return result;
}
//--------------------6.equals----------------------------------------------------
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
Student other = (Student) obj;
if (age != other.age)
return false;
if (name == null) {
if (other.name != null)
return false;
} else if (!name.equals(other.name))
return false;
return true;
}
}
4.3.2 测试类 代码
package cn.ma.coms2;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
/**
*集合测试 存放引用类型的数据
*
*/
public class Test {
public static void main(String[] args) {
//--------1.集合-----------
List<Student> list = new ArrayList<Student>();
//--------2.造对象----------------
Student s1= new Student();
s1.setName("张凡");
s1.setAge(25);
Student s2= new Student();
s2.setName("刘能");
s2.setAge(36);
//-------3.将对象放到集合中--------------
list.add(s1);
list.add(s2);
list.add(new Student("小娇",5));
//----------4.使用iterator遍历集合--------------------------
Iterator<Student> it = list.iterator();
while(it.hasNext()){
Student s = it.next();
System.out.println(s.getName()+" "+s.getAge());
}
}
}
1. 内容摘要
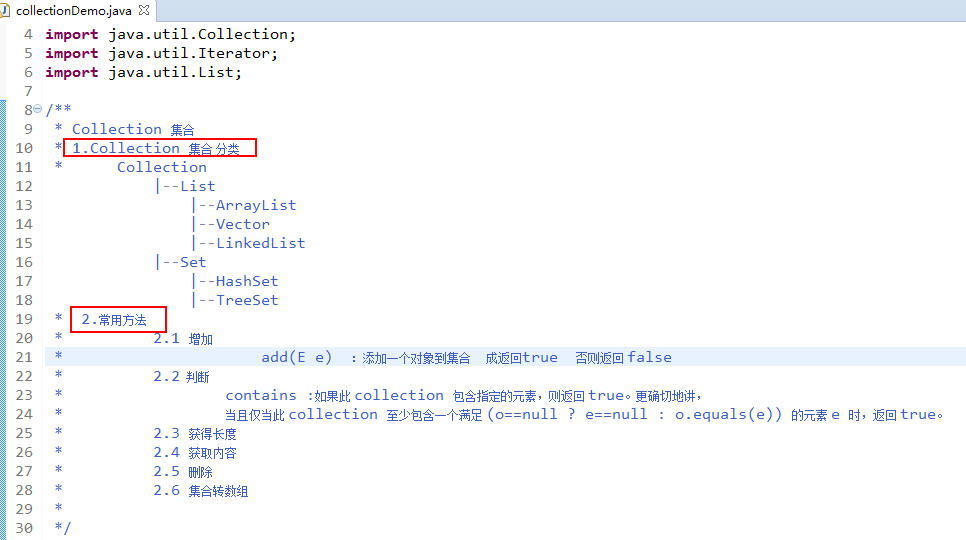
2. collection常用方法
1 Collection集合常用方法
1.1 添加
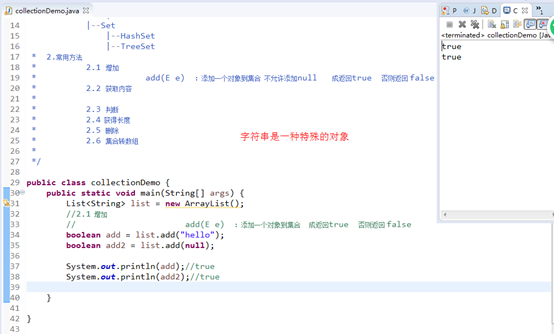
1.2 判断功能
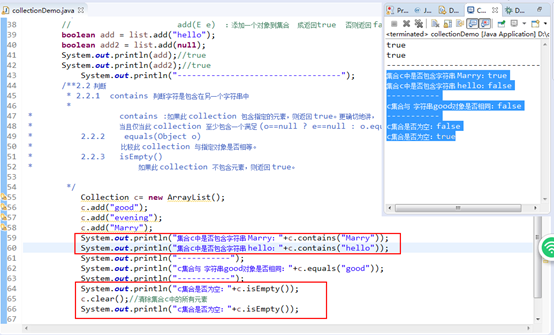
1.3 获得集合中元素长度
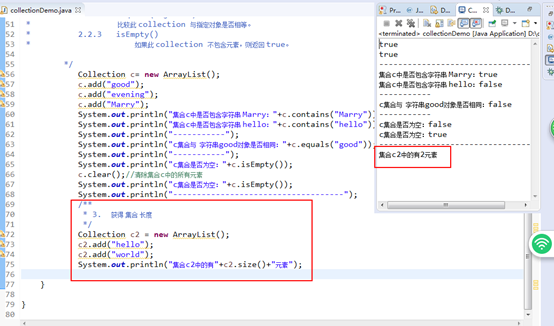
1.4 获得集合中的元素
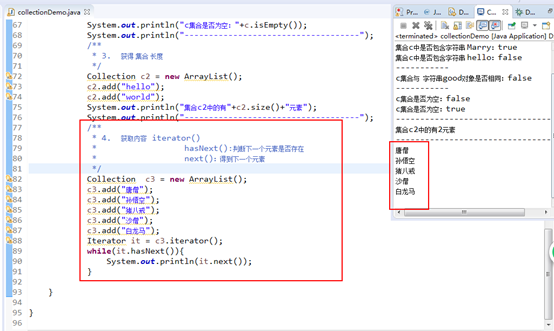
1.5 删除功能
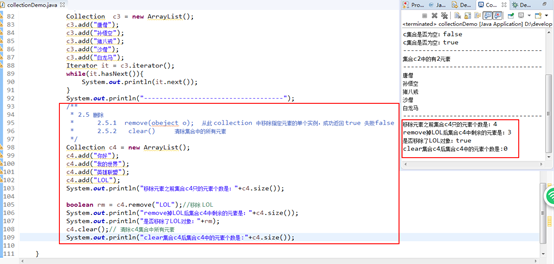
1.6 将集合转数组
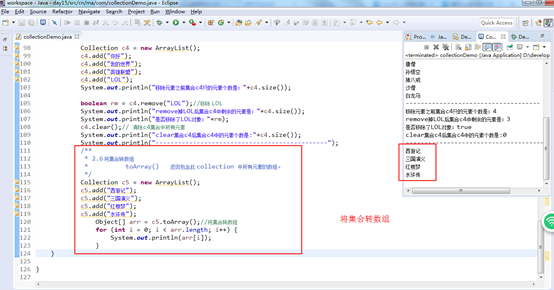
3.代码
package cn.ma.com;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
/**
* Collection 集合
* 1.Collection 集合 分类
* Collection
|--List
|--ArrayList
|--Vector
|--LinkedList
|--Set
|--HashSet
|--TreeSet
* 2.常用方法
* 2.1 增加
* add(E e) :添加一个对象到集合 成返回true 否则返回 false
* 2.2 判断
* contains :如果此 collection 包含指定的元素,则返回 true。更确切地讲,
* 当且仅当此 collection 至少包含一个满足 (o==null ? e==null : o.equals(e)) 的元素 e 时,返回 true。
* 2.3 获得长度
* 2.4 获取内容
* 2.5 删除
* 2.6 集合转数组
*
*/
public class collectionDemo {
public static void main(String[] args) {
List<String> list = new ArrayList();
/**
* 2.1 增加
*
*/
// add(E e) :添加一个对象到集合 成返回true 否则返回 false
boolean add = list.add("hello");
boolean add2 = list.add(null);
System.out.println(add);//true
System.out.println(add2);//true
System.out.println("----------------------------------");
/**2.2 判断
* 2.2.1 contains 判断字符是包含在另一个字符串中
*
* contains :如果此 collection 包含指定的元素,则返回 true。更确切地讲,
* 当且仅当此 collection 至少包含一个满足 (o==null ? e==null : o.equals(e)) 的元素 e 时,返回 true。
* 2.2.2 equals(Object o)
* 比较此 collection 与指定对象是否相等。
* 2.2.3 isEmpty()
* 如果此 collection 不包含元素,则返回 true。
*/
Collection c= new ArrayList();
c.add("good");
c.add("evening");
c.add("Marry");
System.out.println("集合c中是否包含字符串 Marry:"+c.contains("Marry"));
System.out.println("集合c中是否包含字符串 hello:"+c.contains("hello"));
System.out.println("-----------");
System.out.println("c集合与 字符串good对象是否相同:"+c.equals("good"));
System.out.println("-----------");
System.out.println("c集合是否为空:"+c.isEmpty());
c.clear();//清除集合c中的所有元素
System.out.println("c集合是否为空:"+c.isEmpty());
System.out.println("------------------------------------");
/**
* 3. 获得 集合 长度
*/
Collection c2 = new ArrayList();
c2.add("hello");
c2.add("world");
System.out.println("集合c2中的有"+c2.size()+"元素");
System.out.println("------------------------------------");
/**
* 4. 获取内容 iterator()
* hasNext():判断下一个元素是否存在
* next():得到下一个元素
*/
Collection c3 = new ArrayList();
c3.add("唐僧");
c3.add("孙悟空");
c3.add("猪八戒");
c3.add("沙僧");
c3.add("白龙马");
Iterator it = c3.iterator();
while(it.hasNext()){
System.out.println(it.next());
}
System.out.println("------------------------------------");
/**
* 2.5 删除
* 2.5.1 remove(obeject o); 从此 collection 中移除指定元素的单个实例,成功返回 true 失败false
* 2.5.2 clear() 清除集合中的所有元素
*/
Collection c4 = new ArrayList();
c4.add("你好");
c4.add("我的世界");
c4.add("英雄联盟");
c4.add("LOL");
System.out.println("移除元素之前集合c4只的元素个数是:"+c4.size());
boolean rm = c4.remove("LOL");//移除 LOL
System.out.println("remove掉LOL后集合c4中剩余的元素是:"+c4.size());
System.out.println("是否移除了LOL对象:"+rm);
c4.clear();// 清除c4集合中所有元素
System.out.println("clear集合c4后集合c4中的元素个数是:"+c4.size());
System.out.println("----------------------------------------------");
/**
* 2.6 将集合转数组
* toArray() 返回包含此 collection 中所有元素的数组。
*/
Collection c5 = new ArrayList();
c5.add("西游记");
c5.add("三国演义");
c5.add("红楼梦");
c5.add("水浒传");
Object[] arr = c5.toArray();//将集合转数组
for (int i = 0; i < arr.length; i++) {
System.out.println(arr[i]);
}
}
}
4.案列
4.1 学生类
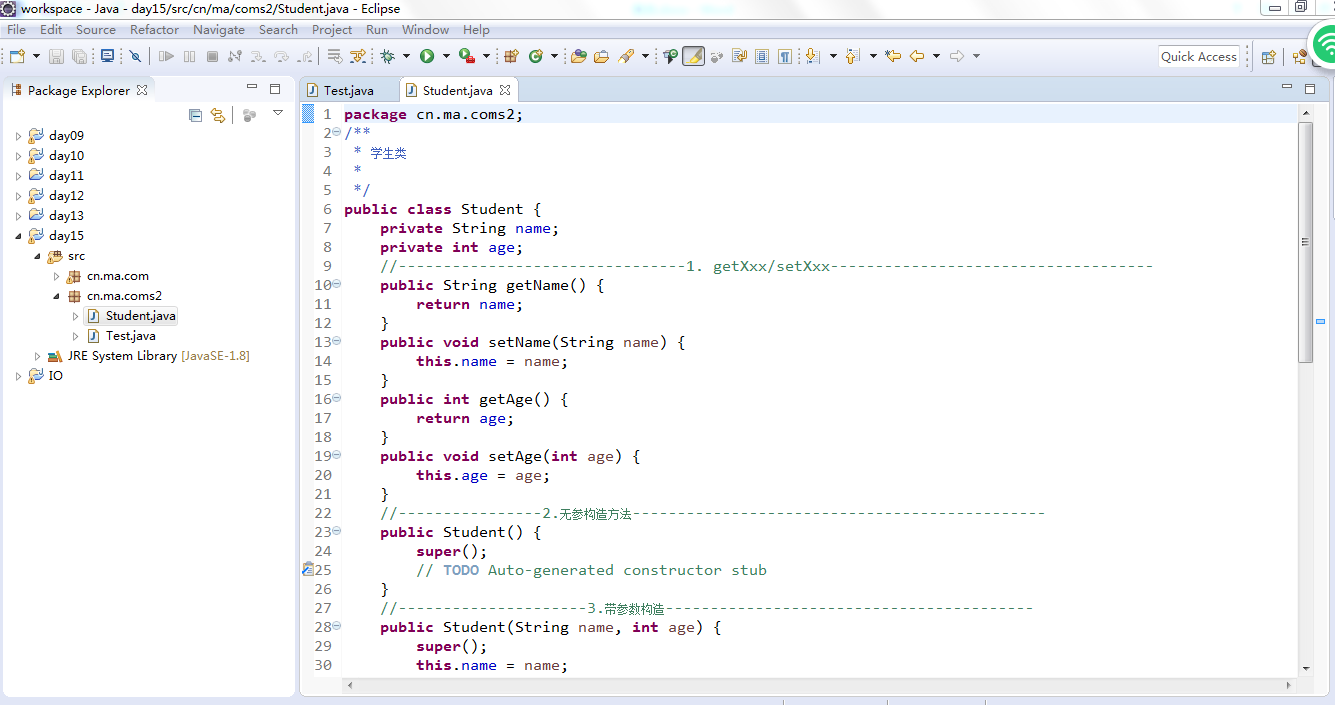
4.2 测试类
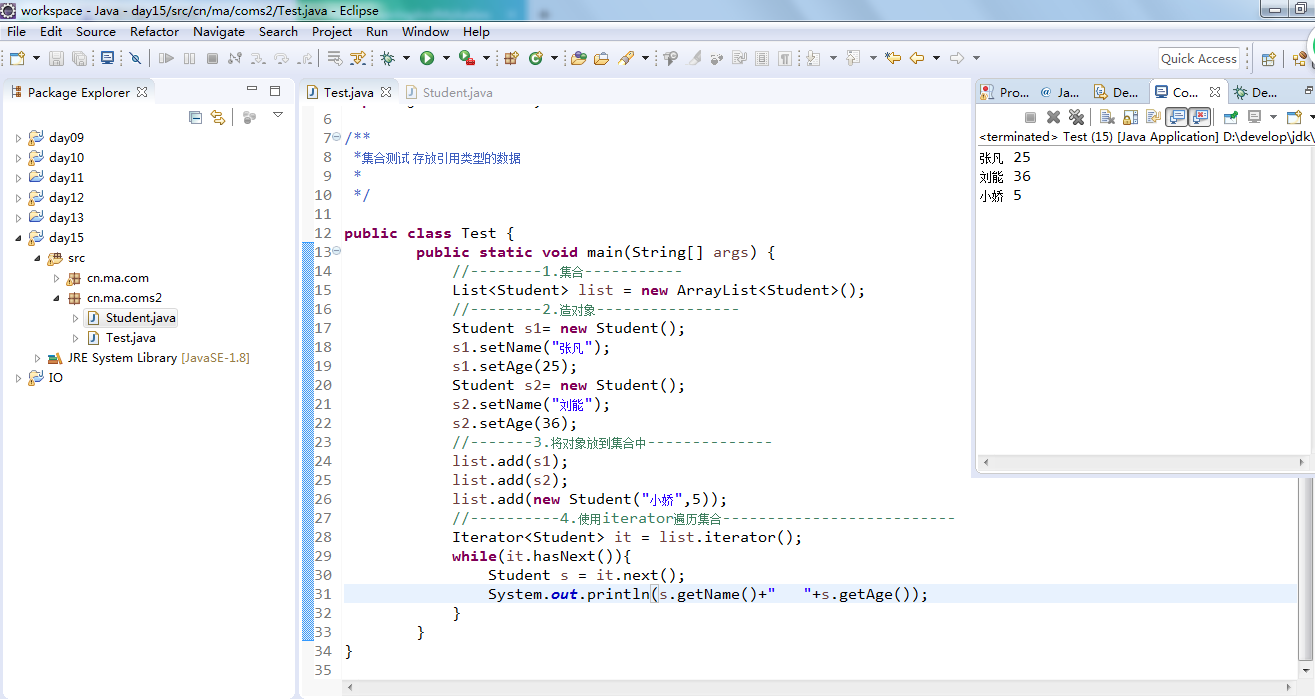
4.3 代码
4.3.1 学生类 代码
package cn.ma.coms2;
/**
* 学生类
*
*/
public class Student {
private String name;
private int age;
//--------------------------------1. getXxx/setXxx------------------------------------
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
//----------------2.无参构造方法----------------------------------------------
public Student() {
super();
// TODO Auto-generated constructor stub
}
//---------------------3.带参数构造-----------------------------------------
public Student(String name, int age) {
super();
this.name = name;
this.age = age;
}
//-------------------4.toString方法------------------------------------------
@Override
public String toString() {
return "Student [name=" + name + ", age=" + age + "]";
}
//----------------------5.hashCode--------------------------------------
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + age;
result = prime * result + ((name == null) ? 0 : name.hashCode());
return result;
}
//--------------------6.equals----------------------------------------------------
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
Student other = (Student) obj;
if (age != other.age)
return false;
if (name == null) {
if (other.name != null)
return false;
} else if (!name.equals(other.name))
return false;
return true;
}
}
4.3.2 测试类 代码
package cn.ma.coms2;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
/**
*集合测试 存放引用类型的数据
*
*/
public class Test {
public static void main(String[] args) {
//--------1.集合-----------
List<Student> list = new ArrayList<Student>();
//--------2.造对象----------------
Student s1= new Student();
s1.setName("张凡");
s1.setAge(25);
Student s2= new Student();
s2.setName("刘能");
s2.setAge(36);
//-------3.将对象放到集合中--------------
list.add(s1);
list.add(s2);
list.add(new Student("小娇",5));
//----------4.使用iterator遍历集合--------------------------
Iterator<Student> it = list.iterator();
while(it.hasNext()){
Student s = it.next();
System.out.println(s.getName()+" "+s.getAge());
}
}
}
相关文章推荐
- java集合:Collection和Map的介绍
- 黑马程序员——Collection集合的一点介绍
- 黑马程序员__集合Collection__List及三个子类的方法介绍
- 1、001 集合总介绍和Collection介绍
- Collection:集合介绍
- Java学习笔记 13 Java 集合 Collection 基本介绍
- 黑马程序员-集合框架Collection List Set 接口的简单介绍
- Java集合框架介绍。Java Collection Frameworks = JCF
- Java collection 的一些介绍 集合
- Java集合Collection介绍
- Guava库介绍之集合(Collection)相关的API
- Guava库介绍之集合(Collection)相关的API
- Collection-集合的介绍
- 集合入门介绍第四章——Map集合
- 15. 集合类(Collection、List)
- Collection集合之六大接口(Collection、Set、List、Map、Iterator和Comparable)
- 黑马程序员_集合Collection
- 用collection.sort()方法对list集合排序
- java基础学习_集合类03_用户登录注册案例(集合版)、Set集合、Collection集合总结_day17总结
- Collection之List集合