Spring 简单的IOC和AOP
2017-06-21 10:53
555 查看
像前面博客中提到struts框架,Hibernate框架似的,spring同样也是一个开源的框架。使用框架的的优势在于分层结构,每层有相应的框架,减少开发工作量,减少组件之间的耦合。struts框架应用web层,Hibernate框架应用持久层,spring应用两者之间。
我觉得,框架是出于聪明的赖人之手。聪明是说他们开发封装每层框架,把复杂的操作全部封装在框架中。而赖人是说他所有复杂的操作实现全部交给计算机来实现,减少人们的开发工作量,把工作的注意力集中在业务逻辑上。
那我们来介绍一下spring框架。
spring框架是一个开源的轻量级的基于IOC与AOP核心技术的容器框架,主要是解决企业的复杂操作实现。
那IOC与AOP,到底如何解释呢,在看spring视频中,两个专业术语一定必须要懂得。
IOC:inverse of Control:控制反转。意思是程序中的之间的关系,不用代码控制,而完全是由容器来控制。在运行阶段,容器会根据配置信息直接把他们的关系注入到组件中。控制反转和依赖注入的区别,依赖注入强调关系的注入是由容器在运行时完成,而控制反转强调关系是由容器控制。其实本质是一样的。
AOP
AOP是动态代理的应用,将具体业务和相应的其它方面(比如日志,权限之类的)划分开来,业务不会知道还有没有其它的功能来辅助,需要的话我就给他加上一个配置就可以,而不用去修改业务代码。
1.新建一个普通的Java项目
2.引入相应的jar包
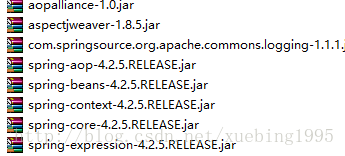
3.提供配置文件ApplicationContext.xml文件
4.开始写代码
配置文件:
applicationContext.xml
测试类:
TestMain.java
运行结果:
复杂Bean类:
UserFather.java
配置文件多加一句:
运行结果:
2.@Component 公共组建
3.@Controller 控制用户请求 在 springMVC中用
4.@Repository 在SSM基本不用
基于上边的代码
接口:
UserServiceImp.java
配置文件:
测试类:
运行结果:
测试类:
运行结果:
运行结果
我觉得,框架是出于聪明的赖人之手。聪明是说他们开发封装每层框架,把复杂的操作全部封装在框架中。而赖人是说他所有复杂的操作实现全部交给计算机来实现,减少人们的开发工作量,把工作的注意力集中在业务逻辑上。
那我们来介绍一下spring框架。
spring框架是一个开源的轻量级的基于IOC与AOP核心技术的容器框架,主要是解决企业的复杂操作实现。
那IOC与AOP,到底如何解释呢,在看spring视频中,两个专业术语一定必须要懂得。
IOC:inverse of Control:控制反转。意思是程序中的之间的关系,不用代码控制,而完全是由容器来控制。在运行阶段,容器会根据配置信息直接把他们的关系注入到组件中。控制反转和依赖注入的区别,依赖注入强调关系的注入是由容器在运行时完成,而控制反转强调关系是由容器控制。其实本质是一样的。
控制反转和依赖注入区别具体代码链接:
http://www.cnblogs.com/xxzhuang/p/5948902.htmlAOP
AOP是动态代理的应用,将具体业务和相应的其它方面(比如日志,权限之类的)划分开来,业务不会知道还有没有其它的功能来辅助,需要的话我就给他加上一个配置就可以,而不用去修改业务代码。
一、IOC
用代码演示一下控制反转是如何实现的。1.新建一个普通的Java项目
2.引入相应的jar包
3.提供配置文件ApplicationContext.xml文件
4.开始写代码
GetBean方式:
简单Bean类:package com.entity; import java.util.List; public class User { private int id; private String name; private List<String> list; 4000 public List<String> getList() { return list; } public void setList(List<String> list) { this.list = list; } public User() { // TODO Auto-generated constructor stub } public User(int id,String name) { // TODO Auto-generated constructor stub this.id=id; this.name=name; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } }
注意:一定要有无参构造函数
否则会出现这样的错误Error creating bean with name 'user' defined in class path resource [applicationContext.xml]: Instantiation of bean failed;
配置文件:
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?><!-- 当前的XML文档版本与字符编码 --> <!-- xmlns:自定义标记及XML编写规范的命名空间。 --> <!-- xmlns:xsi:指当前XML文档应遵守的W3C国际组织定义的XML编写规范 --> <!-- xsi:schemaLocation: 指定某命名空间所使用的schema约束文件路径。 --> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/jdbc http://www.springframework.org/schema/jdbc/spring-jdbc.xsd http://www.springframework.org/schema/task http://www.springframework.org/schema/task/spring-task.xsd http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/cache http://www.springframework.org/schema/cache/spring-cache.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.0.xsd "> <bean id="user" class="com.entity.User"> <!-- 构造初始化 --> <!-- <constructor-arg type="int" value="12121"></constructor-arg> <constructor-arg type="java.lang.String" value="老王八"></constructor-arg> --> <property name="id" value="5454" /> <property name="name" value="沈雪冰" /> <property name="list"> <list> <value>aaaaa</value> <value>bbbbb</value> <value>ccccc</value> </list> </property> </bean> </beans>
测试类:
TestMain.java
package com.entity; import java.lang.annotation.Retention; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Required; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import org.springframework.stereotype.Repository; import com.controller.UserController; import com.service.UserService; public class TestMain { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml"); User user = context.getBean(User.class); // 不用强转 // User user= (User) context.getBean("user"); System.out.println(user.getId()); System.out.println(user.getName()); } }
运行结果:
5454 沈雪冰 [aaaaa, bbbbb, ccccc]
复杂Bean类:
UserFather.java
package com.entity; public class UserFather { private String fathername; private User user; public String getFathername() { return fathername; } public void setFathername(String fathername) { this.fathername = fathername; } public User getUser() { return user; } public void setUser(User user) { this.user = user; } }
配置文件多加一句:
<bean id="fatherUser" class="com.entity.UserFather"> <property name="fathername" value="老爹" /> <property name="user" ref="user" /> </bean>
value只能放简单类型,ref是参考哪个Bean
测试:UserFather userFather=context.getBean(UserFather.class); System.out.println(userFather.getFathername());
运行结果:
老爹
注解方式:
1.@Service 业务层2.@Component 公共组建
3.@Controller 控制用户请求 在 springMVC中用
4.@Repository 在SSM基本不用
基于上边的代码
接口:
package com.service; public interface UserService { public void say(String value); }
UserServiceImp.java
package com.service; import org.springframework.stereotype.Service; @Service //注解 public class UserServiceImp implements UserService { @Override public void say(String value) { // TODO Auto-generated method stub System.out.println("hahahahah"); } }
配置文件:
<?xml version="1.0" encoding="UTF-8"?><!-- 当前的XML文档版本与字符编码 -->
<!-- xmlns:自定义标记及XML编写规范的命名空间。 -->
<!-- xmlns:xsi:指当前XML文档应遵守的W3C国际组织定义的XML编写规范 -->
<!-- xsi:schemaLocation: 指定某命名空间所使用的schema约束文件路径。 -->
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/jdbc http://www.springframework.org/schema/jdbc/spring-jdbc.xsd http://www.springframework.org/schema/task http://www.springframework.org/schema/task/spring-task.xsd http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/cache http://www.springframework.org/schema/cache/spring-cache.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.0.xsd ">
<!-- <context:component-scan base-package="com.service" /> -->
<context:component-scan base-package="com.*" /> <!--包扫描 com.所有包添加注解的地方-->
<bean id="user" class="com.entity.User">
<!-- 构造初始化 -->
<!-- <constructor-arg type="int" value="12121"></constructor-arg>
<constructor-arg type="java.lang.String" value="老王八"></constructor-arg> -->
<property name="id" value="5454" />
<property name="name" value="沈雪冰" />
<property name="list">
<list>
<value>aaaaa</value>
<value>bbbbb</value>
<value>ccccc</value>
</list>
</property>
</bean>
<bean id="fatherUser" class="com.entity.UserFather"> <property name="fathername" value="老爹" /> <property name="user" ref="user" /> </bean>
</beans>
测试类:
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml"); //在访问配置文件的时候自动加载
运行结果:
hahahahahah
依赖注入:
package com.controller; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Component; import org.springframework.stereotype.Controller; import org.springframework.stereotype.Service; import com.service.UserService; /** *********************************** * *@类名 UserController *@时间 2017年6月17日下午11:09:44 *@作者 沈雪冰 *@描述 * *********************************** */ @Controller public class UserController { @Autowired //注入 private UserService uservice; public UserService getUservice() { return uservice; } }
测试类:
UserController controller=context.getBean(UserController.class); UserService userService=controller.getUservice(); userService.say("我是沈雪冰");
运行结果:
我是沈雪冰
二、AOP
配置文件:<!-- 这头文件一定要加上--> xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation=" http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.0.xsd" <!--自动代理 --> <aop:aspectj-autoproxy />
package com.aop; import org.aspectj.lang.JoinPoint; import org.aspectj.lang.annotation.After; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Before; import org.aspectj.lang.annotation.Pointcut; import org.springframework.stereotype.Component; @Component @Aspect // 声明切面 public class UserAspect { @Pointcut("execution(* com.service.UserServiceImp.*(..))") public void Pointcut() { } //advice 我要干的事情 什么时候干 @Before(value="Pointcut()") public void doBefore(JoinPoint joinpoint){//连接点 程序执行的点 多指的是程序的方法 String name= joinpoint.getSignature().getName();//方法的名称 Object [] args =joinpoint.getArgs(); //拿到方法的参数 System.out.println(name+" "+args.toString()); } @After(value="Pointcut()") public void doAfter(JoinPoint joinpoint){ String name= joinpoint.getSignature().getName(); System.out.println(name); } }
注意 :Pointcut区分大小写
测试:userService.say("laowangab");
运行结果
say [Ljava.lang.Object;@34123d65 laowangab say
相关链接:
Spring实现AOP的多种方式相关文章推荐
- 简单理解Spring之IOC和AOP及代码示例
- 手写Spring基本体系IOC+AOP+MVC+事物管理等简单实现。
- 仿写Spring实现简单IOC与AOP
- 简单谈谈Spring的IOC与AOP
- AOP与IOC,简单理解spring
- 理解Spring的AOP和Ioc/DI就这么简单
- Spring中AOP和IOC的简单作用
- spring.net、castle windsor、unity实现aop、ioc的方式和简单区别
- spring中aop、ioc简单理解
- 用代码学习Spring:IoC、AOP(上)
- 为什么说Spring是一个轻量级的控制反转(IoC)和面向切面(AOP)的容器框架?
- 用代码学习Spring:IoC、AOP(下)
- 一个简单的Spring的AOP例子
- AOP在spring中的简单实现
- 简单至及的AOP和IOC
- 深入挖掘IOC、AOP以及Spring中的实现
- 简单至及的AOP和IOC
- 用代码学习Spring:IoC、AOP
- Spring的IOC简单实例
- 使用AOP,在spring中实现简单的性能测试