test01:YUV转RGB
2017-06-13 08:26
190 查看
YUV转RGB实验原理
由电视原理可知,亮度和色差信号的构成如下:
Y=0.2990R+0.5870G+0.1140B
R-Y=0.7010R-0.5870G-0.1140B
B-Y=-0.2990R-0.5870G+0.8860B
为了使色差信号的动态范围控制在0.5之间,需要进行归一化,对色差信号引入压缩系数。归一化后的色差信号为:
U=-0.1684R-0.3316G+0.5B
V=0.5R-0.4187G-0.0813B
易推得YUV到RGB空间的转换公式为:
R=Y+1.4075(V−128)
G=Y−0.3455(U−128)−0.7169(V−128)
B=Y+1.779(U−128)
色度格式
4:2:0格式是指色差信号U,V的取样频率为亮度信号取样频率的四分之一,在水平方向和垂直方向上的取样点数均为Y的一半。
RGB格式的视频按每个像素B、G、R的顺序储存数据;所占空间大小为Width*Height*Frame*3。
YUV格式的视频将整帧图像的Y打包存储,依次再存整帧的U、V,然后再是下一帧的数据;YUV格式按4:2:0取样的视频所占空间大小为Width*Height*Frame*1.5(Width*Height*Frame*+Width*Height*Frame*1/4+Width*Height*Frame*1/4)
将YUV文件转换为RGB文件后,由于RGB文件的颜色是8bit量化,则数值不在0-255范围内的颜色会出现溢出。因此需对R,G,B做是否溢出的判断,超过255的就令其显示为255,小于0的就令其显示为0.
实验流程
1为两个文件开辟缓冲区。
2读取yuv文件,并写入。
3通过调用函数YUV2RGB将YUV文件转化为RGB文件。
4将RGB缓存写入文件中。
5释放缓冲区。
关键代码及其分析
main函数
[html]
view plain
copy
#include <stdio.h>
#include <stdlib.h>
#include <malloc.h>
#include "yuv2rgb.h"
#define u_int8_t unsigned __int8 //一个字节
#define u_int unsigned __int32 //4个字节
#define u_int32_t unsigned __int32
#define FALSE false
#define TRUE true
//为了防止不同编译系统的int语法不同
int main(int argc, char* argv[])
{
/* variables controlable from command line */
u_int frameWidth = 352; /* --width=<uint> */
u_int frameHeight = 240; /* --height=<uint> */
bool flip = TRUE; /* --flip */
unsigned int i;
/* internal variables */
char* rgbFileName = NULL;
char* yuvFileName = NULL;
FILE* rgbFile = NULL;
FILE* yuvFile = NULL;
u_int8_t* rgbBuf = NULL;
u_int8_t* yBuf = NULL;
u_int8_t* uBuf = NULL;
u_int8_t* vBuf = NULL;
u_int32_t videoFramesWritten = 0;
/* begin process command line */
/* point to the specified file names */
yuvFileName = argv[1];
rgbFileName = argv[2];
frameWidth = atoi(argv[3]); //将字符型的256转换成整型
frameHeight = atoi(argv[4]);
/* open the YUV file */
yuvFile = fopen(yuvFileName, "rb");
if (yuvFile == NULL)
{
printf("cannot find yuv file\n");
exit(1);
}
else
{
printf("The input yuv file is %s\n", yuvFileName);
}
/* open the RGB file */
rgbFile = fopen(rgbFileName, "wb");
if (rgbFile == NULL)
{
printf("cannot find rgb file\n");
exit(1);
}
else
{
printf("The output rgb file is %s\n", rgbFileName);
}
/* get an input buffer for a frame */
rgbBuf = (u_int8_t*)malloc(frameWidth * frameHeight * 3); // 向系统申请分配指定(frameWidth * frameHeight * 3)个字节的内存空间,并用指针rgbBuf指向它
/* get the output buffers for a frame */
yBuf = (u_int8_t*)malloc(frameWidth * frameHeight);
uBuf = (u_int8_t*)malloc((frameWidth * frameHeight) / 4);
vBuf = (u_int8_t*)malloc((frameWidth * frameHeight) / 4);
if (rgbBuf == NULL || yBuf == NULL || uBuf == NULL || vBuf == NULL) //判断buffer是否已满
{
printf("no enought memory\n");
exit(1);
}
while (fread(yBuf, 1, frameWidth * frameHeight, yuvFile)
&& fread(uBuf, 1, frameWidth * frameHeight / 4, yuvFile)
&& fread(vBuf, 1, frameWidth * frameHeight / 4, yuvFile))
{
//若fread读入成功,则返回十几读取的数据量个数。若视频结束,fread读取失败则返回0,循环结束。
if (YUV2RGB(frameWidth, frameHeight, rgbBuf, yBuf, uBuf, vBuf, flip))
{
printf("error");
return 0;
}
fwrite(rgbBuf, 1, frameWidth * frameHeight * 3, rgbFile);
printf("\r...%d", ++videoFramesWritten);
}
printf("\n%u %ux%u video frames written\n",
videoFramesWritten, frameWidth, frameHeight);
/* cleanup */
fclose(rgbFile);
fclose(yuvFile);
if(rgbBuf!=NULL) free(rgbBuf);
if (yBuf != NULL)free(yBuf);
if (uBuf != NULL)free(uBuf);
if (vBuf != NULL)free(vBuf);
return(0);
}
yuv2rgb.cpp
[csharp]
view plain
copy
/**************************************************************************
* *
* This code is developed by Adam Li. This software is an *
* implementation of a part of one or more MPEG-4 Video tools as *
* specified in ISO/IEC 14496-2 standard. Those intending to use this *
* software module in hardware or software products are advised that its *
* use may infringe existing patents or copyrights, and any such use *
* would be at such party's own risk. The original developer of this *
* software module and his/her company, and subsequent editors and their *
* companies (including Project Mayo), will have no liability for use of *
* this software or modifications or derivatives thereof. *
* *
* Project Mayo gives users of the Codec a license to this software *
* module or modifications thereof for use in hardware or software *
* products claiming conformance to the MPEG-4 Video Standard as *
* described in the Open DivX license. *
* *
* The complete Open DivX license can be found at *
* http://www.projectmayo.com/opendivx/license.php . *
* *
**************************************************************************/
/**************************************************************************
*
* rgb2yuv.c, 24-bit RGB bitmap to YUV converter
*
* Copyright (C) 2001 Project Mayo
*
* Adam Li
*
* DivX Advance Research Center <darc@projectmayo.com>
*
**************************************************************************/
/* This file contains RGB to YUV transformation functions. */
#include "stdlib.h"
#include "yuv2rgb.h"
static float YUVRGB14075[256];
static float YUVRGB03455[256], YUVRGB07169[256];
static float YUVRGB1779[256];
int YUV2RGB(int width, int height, void *rgb_out, void *y_in, void *u_in, void *v_in, int flip)
{
static int init_done = 0;
long i, j, size;
float r1, g1, b1;
unsigned char *r, *g, *b;
unsigned char *y, *u, *v;
unsigned char *y_buffer, *u_buffer, *v_buffer, *rgb_buffer;
unsigned char *sub_u_buf, *sub_v_buf;
if (init_done == 0)
{
InitLookupTable();
init_done = 1;
}
// 检查width和height能否被2除尽
if ((width % 2) || (height % 2)) return 1;
size = width*height;
// allocate memory分配内存
y_buffer = (unsigned char *)y_in;
u_buffer = (unsigned char *)u_in;
v_buffer = (unsigned char *)v_in;
rgb_buffer = (unsigned char *)rgb_out;
sub_u_buf = (unsigned char *)malloc(size);
sub_v_buf = (unsigned char *)malloc(size);
for (i = 0; i<height; i++)
{
for (j = 0; j<width; j++)
{
*(sub_u_buf + i*width + j) = *(u_buffer + (i / 2)*(width / 2) + j / 2);
*(sub_v_buf + i*width + j) = *(v_buffer + (i / 2)*(width / 2) + j / 2);
}
}
b = rgb_buffer;//RGB格式文件储存时顺序为倒序
y = y_buffer;
u = sub_u_buf;
v = sub_v_buf;
// convert YUV to RGB
for (i = 0; i<height; i++)
{
for (j = 0; j<width; j++)
{
g = b + 1;
r = b + 2;
r1 = *y + YUVRGB14075[*v];
g1 = *y - YUVRGB03455[*u] - YUVRGB07169[*v];
b1 = *y + YUVRGB1779[*u];
*r = (r1>0 ? (r1>255 ? 255 : (unsigned char)r1) : 0);
*g = (g1>0 ? (g1>255 ? 255 : (unsigned char)g1) : 0);
*b = (b1>0 ? (b1>255 ? 255 : (unsigned char)b1) : 0);
b = b + 3;
y++;
u++;
v++;
}
}
if (sub_u_buf!=NULL) free(sub_u_buf);
if (sub_v_buf!=NULL) free(sub_v_buf);
return 0;
}
void InitLookupTable()
{
int i;
for (i = 0; i < 256; i++) YUVRGB14075[i] = (float)1.4075 * (i - 128);
for (i = 0; i < 256; i++) YUVRGB03455[i] = (float)0.3455 * (i - 128);
for (i = 0; i < 256; i++) YUVRGB07169[i] = (float)0.7169 * (i - 128);
for (i = 0; i < 256; i++) YUVRGB1779[i] = (float)1.779 * (i - 128);
}
打开项目属性窗口,通过设置工作目录、命令参数完成主函数的参数输入。

原YUV文件和生成的RGB重新转化成的YUV文件进行对比
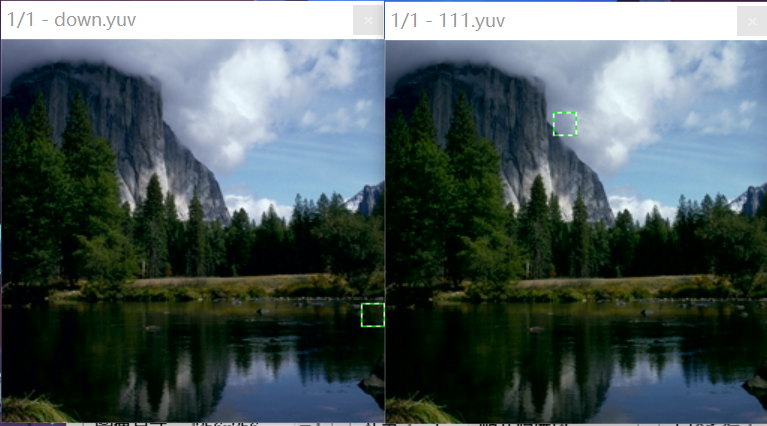
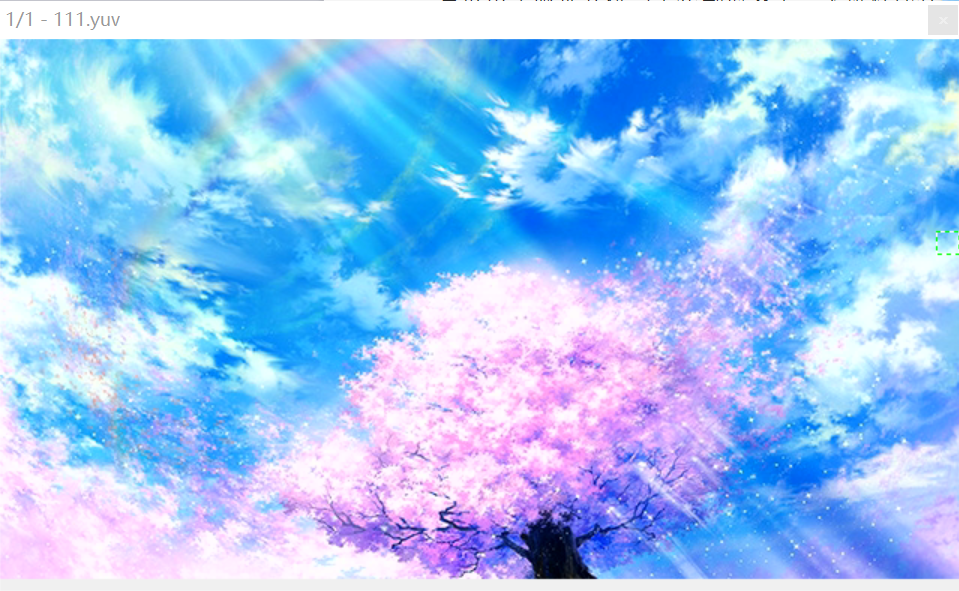
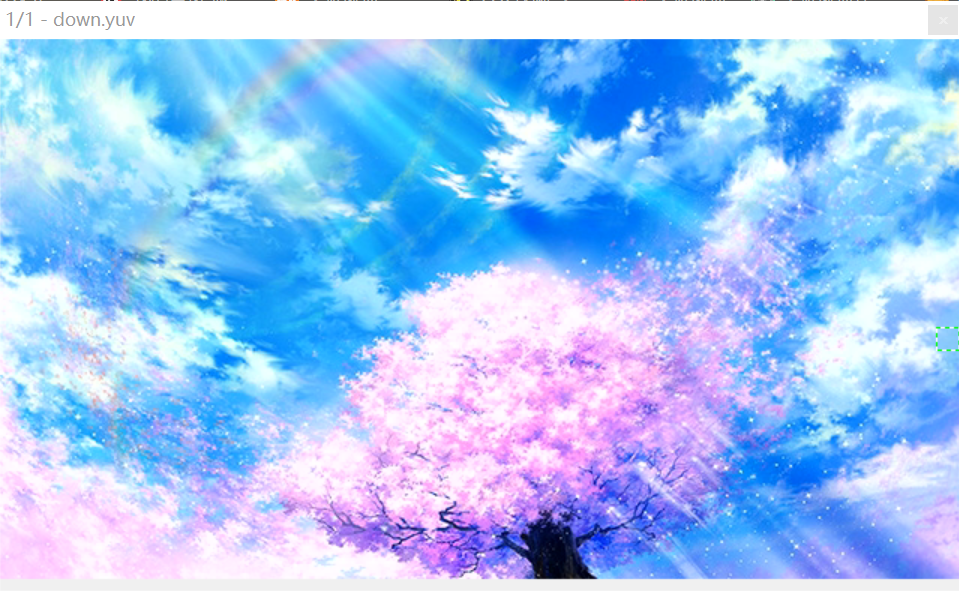
实验结果
经过两次转化后的YUV文件与原YUV文件基本一致。
由电视原理可知,亮度和色差信号的构成如下:
Y=0.2990R+0.5870G+0.1140B
R-Y=0.7010R-0.5870G-0.1140B
B-Y=-0.2990R-0.5870G+0.8860B
为了使色差信号的动态范围控制在0.5之间,需要进行归一化,对色差信号引入压缩系数。归一化后的色差信号为:
U=-0.1684R-0.3316G+0.5B
V=0.5R-0.4187G-0.0813B
易推得YUV到RGB空间的转换公式为:
R=Y+1.4075(V−128)
G=Y−0.3455(U−128)−0.7169(V−128)
B=Y+1.779(U−128)
色度格式
4:2:0格式是指色差信号U,V的取样频率为亮度信号取样频率的四分之一,在水平方向和垂直方向上的取样点数均为Y的一半。
RGB格式的视频按每个像素B、G、R的顺序储存数据;所占空间大小为Width*Height*Frame*3。
YUV格式的视频将整帧图像的Y打包存储,依次再存整帧的U、V,然后再是下一帧的数据;YUV格式按4:2:0取样的视频所占空间大小为Width*Height*Frame*1.5(Width*Height*Frame*+Width*Height*Frame*1/4+Width*Height*Frame*1/4)
将YUV文件转换为RGB文件后,由于RGB文件的颜色是8bit量化,则数值不在0-255范围内的颜色会出现溢出。因此需对R,G,B做是否溢出的判断,超过255的就令其显示为255,小于0的就令其显示为0.
实验流程
1为两个文件开辟缓冲区。
2读取yuv文件,并写入。
3通过调用函数YUV2RGB将YUV文件转化为RGB文件。
4将RGB缓存写入文件中。
5释放缓冲区。
关键代码及其分析
main函数
[html]
view plain
copy
#include <stdio.h>
#include <stdlib.h>
#include <malloc.h>
#include "yuv2rgb.h"
#define u_int8_t unsigned __int8 //一个字节
#define u_int unsigned __int32 //4个字节
#define u_int32_t unsigned __int32
#define FALSE false
#define TRUE true
//为了防止不同编译系统的int语法不同
int main(int argc, char* argv[])
{
/* variables controlable from command line */
u_int frameWidth = 352; /* --width=<uint> */
u_int frameHeight = 240; /* --height=<uint> */
bool flip = TRUE; /* --flip */
unsigned int i;
/* internal variables */
char* rgbFileName = NULL;
char* yuvFileName = NULL;
FILE* rgbFile = NULL;
FILE* yuvFile = NULL;
u_int8_t* rgbBuf = NULL;
u_int8_t* yBuf = NULL;
u_int8_t* uBuf = NULL;
u_int8_t* vBuf = NULL;
u_int32_t videoFramesWritten = 0;
/* begin process command line */
/* point to the specified file names */
yuvFileName = argv[1];
rgbFileName = argv[2];
frameWidth = atoi(argv[3]); //将字符型的256转换成整型
frameHeight = atoi(argv[4]);
/* open the YUV file */
yuvFile = fopen(yuvFileName, "rb");
if (yuvFile == NULL)
{
printf("cannot find yuv file\n");
exit(1);
}
else
{
printf("The input yuv file is %s\n", yuvFileName);
}
/* open the RGB file */
rgbFile = fopen(rgbFileName, "wb");
if (rgbFile == NULL)
{
printf("cannot find rgb file\n");
exit(1);
}
else
{
printf("The output rgb file is %s\n", rgbFileName);
}
/* get an input buffer for a frame */
rgbBuf = (u_int8_t*)malloc(frameWidth * frameHeight * 3); // 向系统申请分配指定(frameWidth * frameHeight * 3)个字节的内存空间,并用指针rgbBuf指向它
/* get the output buffers for a frame */
yBuf = (u_int8_t*)malloc(frameWidth * frameHeight);
uBuf = (u_int8_t*)malloc((frameWidth * frameHeight) / 4);
vBuf = (u_int8_t*)malloc((frameWidth * frameHeight) / 4);
if (rgbBuf == NULL || yBuf == NULL || uBuf == NULL || vBuf == NULL) //判断buffer是否已满
{
printf("no enought memory\n");
exit(1);
}
while (fread(yBuf, 1, frameWidth * frameHeight, yuvFile)
&& fread(uBuf, 1, frameWidth * frameHeight / 4, yuvFile)
&& fread(vBuf, 1, frameWidth * frameHeight / 4, yuvFile))
{
//若fread读入成功,则返回十几读取的数据量个数。若视频结束,fread读取失败则返回0,循环结束。
if (YUV2RGB(frameWidth, frameHeight, rgbBuf, yBuf, uBuf, vBuf, flip))
{
printf("error");
return 0;
}
fwrite(rgbBuf, 1, frameWidth * frameHeight * 3, rgbFile);
printf("\r...%d", ++videoFramesWritten);
}
printf("\n%u %ux%u video frames written\n",
videoFramesWritten, frameWidth, frameHeight);
/* cleanup */
fclose(rgbFile);
fclose(yuvFile);
if(rgbBuf!=NULL) free(rgbBuf);
if (yBuf != NULL)free(yBuf);
if (uBuf != NULL)free(uBuf);
if (vBuf != NULL)free(vBuf);
return(0);
}
yuv2rgb.cpp
[csharp]
view plain
copy
/**************************************************************************
* *
* This code is developed by Adam Li. This software is an *
* implementation of a part of one or more MPEG-4 Video tools as *
* specified in ISO/IEC 14496-2 standard. Those intending to use this *
* software module in hardware or software products are advised that its *
* use may infringe existing patents or copyrights, and any such use *
* would be at such party's own risk. The original developer of this *
* software module and his/her company, and subsequent editors and their *
* companies (including Project Mayo), will have no liability for use of *
* this software or modifications or derivatives thereof. *
* *
* Project Mayo gives users of the Codec a license to this software *
* module or modifications thereof for use in hardware or software *
* products claiming conformance to the MPEG-4 Video Standard as *
* described in the Open DivX license. *
* *
* The complete Open DivX license can be found at *
* http://www.projectmayo.com/opendivx/license.php . *
* *
**************************************************************************/
/**************************************************************************
*
* rgb2yuv.c, 24-bit RGB bitmap to YUV converter
*
* Copyright (C) 2001 Project Mayo
*
* Adam Li
*
* DivX Advance Research Center <darc@projectmayo.com>
*
**************************************************************************/
/* This file contains RGB to YUV transformation functions. */
#include "stdlib.h"
#include "yuv2rgb.h"
static float YUVRGB14075[256];
static float YUVRGB03455[256], YUVRGB07169[256];
static float YUVRGB1779[256];
int YUV2RGB(int width, int height, void *rgb_out, void *y_in, void *u_in, void *v_in, int flip)
{
static int init_done = 0;
long i, j, size;
float r1, g1, b1;
unsigned char *r, *g, *b;
unsigned char *y, *u, *v;
unsigned char *y_buffer, *u_buffer, *v_buffer, *rgb_buffer;
unsigned char *sub_u_buf, *sub_v_buf;
if (init_done == 0)
{
InitLookupTable();
init_done = 1;
}
// 检查width和height能否被2除尽
if ((width % 2) || (height % 2)) return 1;
size = width*height;
// allocate memory分配内存
y_buffer = (unsigned char *)y_in;
u_buffer = (unsigned char *)u_in;
v_buffer = (unsigned char *)v_in;
rgb_buffer = (unsigned char *)rgb_out;
sub_u_buf = (unsigned char *)malloc(size);
sub_v_buf = (unsigned char *)malloc(size);
for (i = 0; i<height; i++)
{
for (j = 0; j<width; j++)
{
*(sub_u_buf + i*width + j) = *(u_buffer + (i / 2)*(width / 2) + j / 2);
*(sub_v_buf + i*width + j) = *(v_buffer + (i / 2)*(width / 2) + j / 2);
}
}
b = rgb_buffer;//RGB格式文件储存时顺序为倒序
y = y_buffer;
u = sub_u_buf;
v = sub_v_buf;
// convert YUV to RGB
for (i = 0; i<height; i++)
{
for (j = 0; j<width; j++)
{
g = b + 1;
r = b + 2;
r1 = *y + YUVRGB14075[*v];
g1 = *y - YUVRGB03455[*u] - YUVRGB07169[*v];
b1 = *y + YUVRGB1779[*u];
*r = (r1>0 ? (r1>255 ? 255 : (unsigned char)r1) : 0);
*g = (g1>0 ? (g1>255 ? 255 : (unsigned char)g1) : 0);
*b = (b1>0 ? (b1>255 ? 255 : (unsigned char)b1) : 0);
b = b + 3;
y++;
u++;
v++;
}
}
if (sub_u_buf!=NULL) free(sub_u_buf);
if (sub_v_buf!=NULL) free(sub_v_buf);
return 0;
}
void InitLookupTable()
{
int i;
for (i = 0; i < 256; i++) YUVRGB14075[i] = (float)1.4075 * (i - 128);
for (i = 0; i < 256; i++) YUVRGB03455[i] = (float)0.3455 * (i - 128);
for (i = 0; i < 256; i++) YUVRGB07169[i] = (float)0.7169 * (i - 128);
for (i = 0; i < 256; i++) YUVRGB1779[i] = (float)1.779 * (i - 128);
}
打开项目属性窗口,通过设置工作目录、命令参数完成主函数的参数输入。
原YUV文件和生成的RGB重新转化成的YUV文件进行对比
实验结果
经过两次转化后的YUV文件与原YUV文件基本一致。
相关文章推荐
- YUV和RGB格式
- RGB YUV IOS
- YUV转换成RGB需要注意的问题 Matlab/C++
- 转:YUV RGB 常见视频格式解析
- 视频与图像RGB/YUV格式详解
- ffmpeg之yuv2rgb_c_24_rgb
- RGB、YUV和YCbCr介绍【转】
- 最简单的基于FFmpeg的libswscale的示例(YUV转RGB)
- YUV格式、RGB格式、JPEG格式、MJPEG格式之间的转换(C程序)之一
- 视音频数据处理入门:RGB、YUV像素数据处理
- sw_scale中实现yuv420转rgb888——neon汇编优化
- RGB、YUV和YCbCr 转换
- YUV 和RGB的相互转换
- RGB / YUV
- 高效率视频播放: GPU支持的YUV RGB 转化例子(2)
- RGB & YUV
- test-01
- YUV与RGB的理解
- neon内嵌汇编实现一个yuv转rgb的功能stopped原因
- Sensor信号输出YUV、RGB、RAW DATA、JPEG 4种方式区别