Linux 线程概念与基础
2017-06-08 17:41
190 查看
一、什么是线程?
线程是在进程内部运行的控制流程。多线程的控制流程可以长期共存,操作系统会在各线程之间调度和切换,就像在多个进程之间调度和切换一样。
由于同一个进程的多个线程共享同一地址空间地址空间,因此代码段和数据段都是共享的,如果定义一个函数,在各线程之中都可以调用,如果定义一个全局变量,在各线程中都可以访问到的,除此之外,线程还共享以下资源:
1>文件描述符表
2>每种信号的处理方式
3>当前工作目录
4>用户id和组id
5>堆空间
但是有些资源是每个线程独自有一份的
1>线程id
2>上下文,包括各种寄存器的值、程序计数器和栈指针
3>栈空间
4>errno变量
5>信号屏蔽字
6>调度优先级
二、线程与进程的区别
进程是程序执行时的一个实例,即它是程序已经执行到课中程度的数据结构的汇集。从内核的观点看,进程的目的就是担当分配系统资源(CPU时间、内存等)的基本单位。
线程是进程的一个执行流,是CPU调度和分派的基本单位,它是比进程更小的能独立运行的基本单位。一个进程由几个线程组成(拥有很多相对独立的执行流的用户程序共享应用程序的大部分数据结构),线程与同属一个进程的其他的线程共享进程所拥有的全部资源。
进程——资源分配的最小单位,线程——程序执行的最小单位
进程有独立的地址空间,一个进程崩溃后,在保护模式下不会对其它进程产生影响,而线程只是一个进程中的不同执行路径。线程有自己的堆栈和局部变量,但线程没有单独的地址空间,一个线程死掉就等于整个进程死掉,所以多进程的程序要比多线程的程序健壮,但在进程切换时,耗费资源较大,效率要差一些。
总的来说就是:进程有独立的地址空间,线程没有单独的地址空间(同一进程内的线程共享进程的地址空间)。
三、下面是线程的简单代码
线程创建
程序运行如下
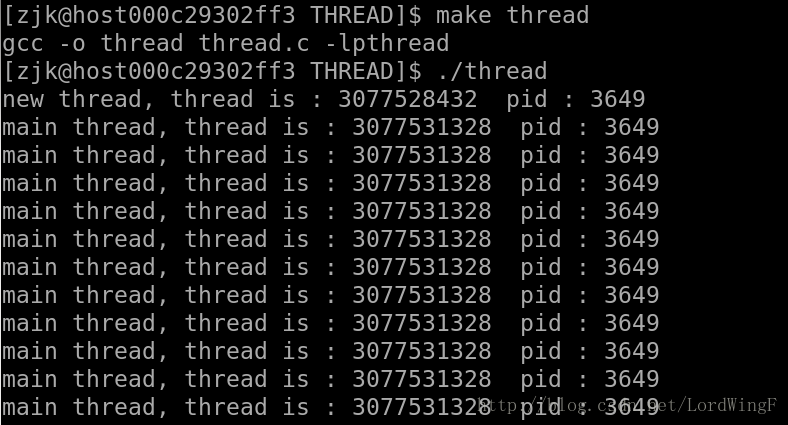
再调用ps -ef -f | grep proftpd 来查看创建的线程

线程的等待释放
程序运行如下
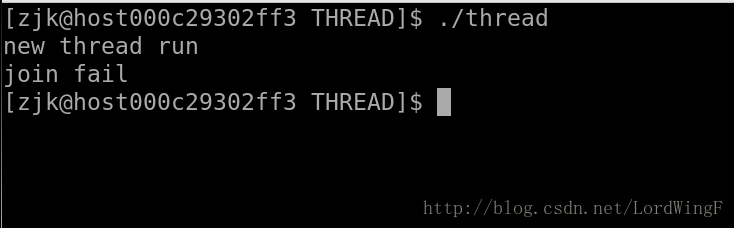
这就是线程的简单创建和退出等待
a446
线程是在进程内部运行的控制流程。多线程的控制流程可以长期共存,操作系统会在各线程之间调度和切换,就像在多个进程之间调度和切换一样。
由于同一个进程的多个线程共享同一地址空间地址空间,因此代码段和数据段都是共享的,如果定义一个函数,在各线程之中都可以调用,如果定义一个全局变量,在各线程中都可以访问到的,除此之外,线程还共享以下资源:
1>文件描述符表
2>每种信号的处理方式
3>当前工作目录
4>用户id和组id
5>堆空间
但是有些资源是每个线程独自有一份的
1>线程id
2>上下文,包括各种寄存器的值、程序计数器和栈指针
3>栈空间
4>errno变量
5>信号屏蔽字
6>调度优先级
二、线程与进程的区别
进程是程序执行时的一个实例,即它是程序已经执行到课中程度的数据结构的汇集。从内核的观点看,进程的目的就是担当分配系统资源(CPU时间、内存等)的基本单位。
线程是进程的一个执行流,是CPU调度和分派的基本单位,它是比进程更小的能独立运行的基本单位。一个进程由几个线程组成(拥有很多相对独立的执行流的用户程序共享应用程序的大部分数据结构),线程与同属一个进程的其他的线程共享进程所拥有的全部资源。
进程——资源分配的最小单位,线程——程序执行的最小单位
进程有独立的地址空间,一个进程崩溃后,在保护模式下不会对其它进程产生影响,而线程只是一个进程中的不同执行路径。线程有自己的堆栈和局部变量,但线程没有单独的地址空间,一个线程死掉就等于整个进程死掉,所以多进程的程序要比多线程的程序健壮,但在进程切换时,耗费资源较大,效率要差一些。
总的来说就是:进程有独立的地址空间,线程没有单独的地址空间(同一进程内的线程共享进程的地址空间)。
三、下面是线程的简单代码
线程创建
/*Makefile*/ thread:thread.c gcc -o $@ $^ -lpthread .PHONY:clean clean: rm -f thread /*thread.c*/ /************************************************************************* > File Name: thread.c > Author:LordWingF > Mail: NULL > Created Time: Sun 04 Jun 2017 04:10:21 AM PDT ************************************************************************/ #include<stdio.h> #include<stdlib.h> #include<pthread.h> //创建新线程 void* thread_run(void* arg) { printf("new thread, thread is : %u pid : %d\n", pthread_self(), getpid()); sleep(5); /*退出方式*/ pthread_exit((void*)123); //finish thread 线程专用 //exit(123); //finish process, not thread 进程专用 //return (void*)1; //could finish process, thread too 线程进程都可以使用 } int main() { pthread_t tid; pthread_create(&tid, NULL, thread_run, NULL); sleep(1); /*pthread_cancel(pthread_t thread)发送终止信号给thread线程,如果成功则返回0,否则为非0值*/ pthread_cancel(tid); while(1){ printf("main thread, thread is : %u pid : %d\n", pthread_self(), getpid()); sleep(1); } return 0; }
程序运行如下
再调用ps -ef -f | grep proftpd 来查看创建的线程
线程的等待释放
/*Makefile*/ thread:thread.c gcc -o $@ $^ -lpthread .PHONY:clean clean: rm -f thread /*thread.c*/ /************************************************************************* > File Name: thread.c > Author:LordWingF > Mail: NULL > Created Time: Sun 04 Jun 2017 04:10:21 AM PDT ************************************************************************/ #include<stdio.h> #include<stdlib.h> #include<pthread.h> //创建新线程 void* thread_run(void* _val) { /*如果一个线程结束运行但没有被join,则它的状态类似于进程中的僵尸进程,这时可以在子线程中加入代码pthread_detach(pthread_self())或者父线程调用pthread_detach(thread_id)*/ pthread_detach(pthread_self()); printf("%s\n",(char*)_val); return NULL; } /*主线程*/ int main() { //创建新线程 pthread_t tid; int tret = pthread_create(&tid, NULL, thread_run, "new thread run"); //定义ret接受新线程的返回值,如果ret不是0的话,则说明等待失败,反之,等待成功。在这里因为子线程中调用了pthread_detach(pthread_self()),所以一定会返回失败 sleep(1); void* ret; if(pthread_join(tid, &ret) != 0){ printf("join fail\n"); } }
程序运行如下
这就是线程的简单创建和退出等待
a446
相关文章推荐
- Linux环境下编程(二)——线程基础概念
- Linux线程技术:概念与技术发展
- Linux线程技术的概念与技术发展
- (转)linux下bluetooth编程(一)基础概念
- 【Linux基础】线程发展历程
- 【Linux基础】线程概述
- 理解Linux中进程,线程等概念
- Unix/Linux C++应用开发-C++基础概念"数组、指针和字符串"
- JAVA线程之一----基础概念
- 线程的基础概念
- 【Linux基础】TCP/IP基本概念
- 了解Linux线程技术的概念与技术发展
- 介绍NPTL (经典--诠释了线程的概念,以及linux调度单位)
- Linux 线程基础 1
- linux操作系统基础概念(二) 操作系统总体介绍
- Diy My Own Linux (1 基础概念) 连载3
- LINUX主要记忆点(1):基础概念--2011-08-07
- Linux 线程 多线程 基础知识 (转)
- [linux 基础] 线程之间的通信