设计模式系列(9)简单工厂模式
2017-06-08 11:11
267 查看
1.什么是简单工厂模式
简单工厂模式属于类的创建型模式,又叫做静态工厂方法模式。通过专门定义一个类来负责创建其它类的实例,被创建的实例通常都具有共同的父类。
2.模式中包含的角色及其职责
1) 工厂(Creator)角色
简单工厂模式的核心,它负责实现创建所有实例的内部逻辑。工厂类可以被外外界直接调用,创建所需要的产品对象。
1) 抽象(Product)角色
简单工厂模式所创建的所有对象的父类,它负责描述所共有的公共接口。
2) 具体产品(Concrete Product)角色
简单工厂模式所创建的具体实例对象。
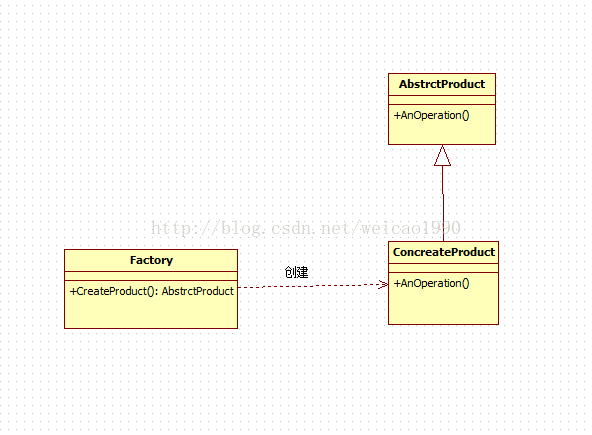
简单工厂模式 UML类图
3.简单工厂模式的优缺点
在这个模式中,工厂类是整个模式的关键所在。它包含必要的判断逻辑,能够根据外界给定的信息,决定应该创建哪个具体类的对象。用户在使用时可以直接根据工厂类去创建所需要的实例,而无需了解这些对象是如何创建以及如何组织。有利于整个软件体系结构的优化。不难发现,简单工厂的缺点也正体现在工厂类上,由于工厂类集中体现了所有实例的创建逻辑,所有高内聚方面做的并不好。另外,当系统中的具体产品类不断增多时,可能出现要求工厂类要做相应的修改,扩展性并不好。
4.示例代码, 通过工厂类创建不同的水果:
simple_factory.cpp
simple_factory.cpp
main.cpp
简单工厂模式属于类的创建型模式,又叫做静态工厂方法模式。通过专门定义一个类来负责创建其它类的实例,被创建的实例通常都具有共同的父类。
2.模式中包含的角色及其职责
1) 工厂(Creator)角色
简单工厂模式的核心,它负责实现创建所有实例的内部逻辑。工厂类可以被外外界直接调用,创建所需要的产品对象。
1) 抽象(Product)角色
简单工厂模式所创建的所有对象的父类,它负责描述所共有的公共接口。
2) 具体产品(Concrete Product)角色
简单工厂模式所创建的具体实例对象。
简单工厂模式 UML类图
3.简单工厂模式的优缺点
在这个模式中,工厂类是整个模式的关键所在。它包含必要的判断逻辑,能够根据外界给定的信息,决定应该创建哪个具体类的对象。用户在使用时可以直接根据工厂类去创建所需要的实例,而无需了解这些对象是如何创建以及如何组织。有利于整个软件体系结构的优化。不难发现,简单工厂的缺点也正体现在工厂类上,由于工厂类集中体现了所有实例的创建逻辑,所有高内聚方面做的并不好。另外,当系统中的具体产品类不断增多时,可能出现要求工厂类要做相应的修改,扩展性并不好。
4.示例代码, 通过工厂类创建不同的水果:
simple_factory.cpp
#ifndef SIMPLE_FACTORY_H #define SIMPLE_FACTORY_H class Fruit { public: virtual void getFruit() = 0; protected: private: }; class Banana : public Fruit { public: virtual void getFruit(); protected: private: }; class Apple : public Fruit { public: virtual void getFruit(); protected: private: }; class Factory { public: Fruit *create(char *p); }; #endif // SIMPLE_FACTORY_H
simple_factory.cpp
#ifndef SIMPLE_FACTORY_CPP #define SIMPLE_FACTORY_CPP #include <iostream> #include <simple_factory.h> #include <string.h> using namespace std; void Banana::getFruit() { cout << "banana" << endl; } void Apple::getFruit() { cout << "Apple" << endl; } Fruit* Factory::create(char *p) { if(0 == strcmp(p, "banana")) { return new Banana; } else if(0 == strcmp(p, "apple")) { return new Apple; } else { return NULL; cout << "no support ..." << endl; } } #endif // SIMPLE_FACTORY_CPP
main.cpp
#include <iostream> #include "simple_factory.h" using namespace std; int main() { Factory *f = new Factory; Fruit *fruit = NULL; //工厂生产香蕉 fruit = f->create("banana"); fruit->getFruit(); delete fruit; //工厂生产苹果 fruit = f->create("apple"); fruit->getFruit(); delete fruit; delete f; return 0; }
相关文章推荐
- 设计模式系列--工厂模式(简单工厂模式、抽象工厂模式)
- 设计模式系列之一:简单工厂模式
- 设计模式系列——三个工厂模式(简单工厂模式,工厂方法模式,抽象工厂模式)
- 设计模式系列(四)简单工厂模式(Simple Factory Pattern/Static Factory Method)
- 设计模式系列——三个工厂模式(简单工厂模式,工厂方法模式,抽象工厂模式)
- 设计模式系列(十一)外观模式(Facade Pattern)
- PHP设计模式-工厂系列(一)-简单工厂模式(静态工厂模式)
- 设计模式系列:里氏替换原则
- 设计模式系列——三个工厂模式(简单工厂模式,工厂方法模式,抽象工厂模式)
- 《设计模式 系列》- 结构型模式 - 外观模式
- 设计模式系列之热身
- 设计模式系列二 观察者模式介绍
- 设计模式系列之七适配器模式
- 设计模式(二) : 创建型模式--简单工厂模式
- 设计模式(02) 工厂方法模式+简单工厂模式(上)
- 设计模式系列-观察者模式 推荐
- 设计模式(一) 简单工厂模式
- 设计模式系列-命令模式 推荐
- 设计模式系列-工厂方法模式
- 设计模式(一)——简单工厂模式