103. Binary Tree Zigzag Level Order Traversal
2017-05-14 18:09
323 查看
Given a binary tree, return the zigzag level order traversal of its nodes’ values. (ie, from left to right, then right to left for the next level and alternate between).
For example:
Given binary tree [3,9,20,null,null,15,7],
return its zigzag level order traversal as:
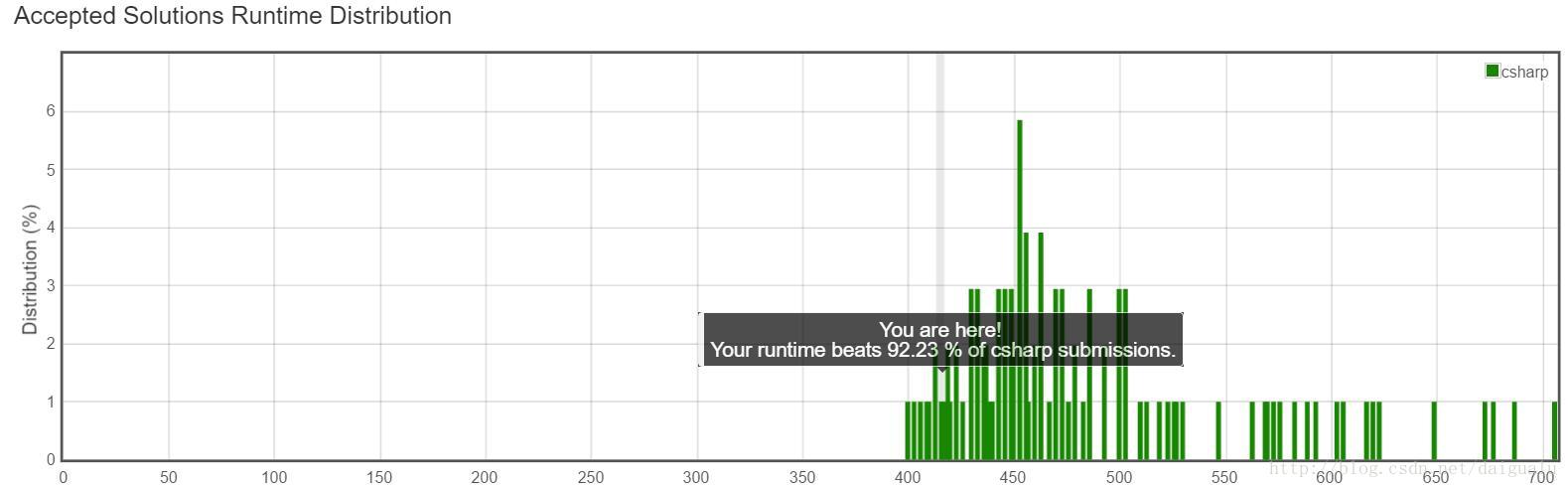
For example:
Given binary tree [3,9,20,null,null,15,7],
3 / \ 9 20 / \ 15 7
return its zigzag level order traversal as:
[ [3], [20,9], [15,7] ]
代码实现
public class Solution { private int lineno; //从二层开始按行号编号 public IList<IList<int>> ZigzagLevelOrder(TreeNode root) { if (root == null) return new List<IList<int>>(); IList<IList<int>> rtn = new List<IList<int>>{new List<int> {root.val}}; //第一层 var tmp = zigzagLevelOrder(new List<TreeNode> { root }); //tmp: 第二层~叶子层 if (tmp != null && tmp.Count > 0) { foreach (var item in tmp) rtn.Add(item); } return rtn; } //第二层到叶子层: right to left; left to right; ... private IList<IList<int>> zigzagLevelOrder(IList<TreeNode> curLevel) { if (curLevel == null || curLevel.Count == 0) return null; IList<IList<int>> rtn= new List<IList<int>>(); List<TreeNode> nextn = new List<TreeNode>(); foreach (var item in curLevel) { if (item.left != null) nextn.Add(item.left); if (item.right != null) nextn.Add(item.right); } if (nextn.Count == 0) return null; if(lineno%2==0) //反转本层 { List<int> tmp = nextn.Select(r => r.val).ToList(); tmp.Reverse(); rtn.Add(tmp); } else rtn.Add(nextn.Select(r=>r.val).ToList()); //正序 ++lineno; var children = zigzagLevelOrder(nextn); if (children != null && children.Count > 0) { foreach (var item in children) rtn.Add(item); } return rtn; } }
结果分析
leetcode-solution库
leetcode算法题目解决方案每天更新在github库中,欢迎感兴趣的朋友加入进来,也欢迎star,或pull request。https://github.com/jackzhenguo/leetcode-csharp相关文章推荐
- LeetCode103 Binary Tree Zigzag Level Order Traversal
- LeetCode(103) Binary Tree Zigzag Level Order Traversal
- LeetCode103 Binary Tree Zigzag Level Order Traversal
- 103. Binary Tree Zigzag Level Order Traversal
- 103. Binary Tree Zigzag Level Order Traversal
- Leetcode[103]-Binary Tree Zigzag Level Order Traversal
- leetcode_103 Binary Tree Zigzag Level Order Traversal
- LeetCode题解-103-Binary Tree Zigzag Level Order Traversal
- leetcode_103题——Binary Tree Zigzag Level Order Traversal(广度优先搜索,队列queue,栈stack)
- 103 Binary Tree Zigzag Level Order Traversal
- 103. Binary Tree Zigzag Level Order Traversal
- Leetcode-103(Java) Binary Tree Zigzag Level Order Traversal
- leetcode 103 —— Binary Tree Zigzag Level Order Traversal
- FTPrep, 103 Binary Tree Zigzag Level Order Traversal
- [Leetcode 95] 103 Binary Tree Zigzag Level Order Traversal
- 103. Binary Tree Zigzag Level Order Traversal
- LeetCode 103 Binary Tree Zigzag Level Order Traversal(二叉树层序遍历)
- 103. Binary Tree Zigzag Level Order Traversal【M】
- 103. Binary Tree Zigzag Level Order Traversal
- 103. Binary Tree Zigzag Level Order Traversal