【java】java swing初识:事件触发&登陆界面
2017-05-03 16:31
405 查看
欢迎大家到我的个人主页点击打开链接,一起交流、学习,一起进步~
1.界面输出字符:hello world!
[align=justify]效果截图:[/align]
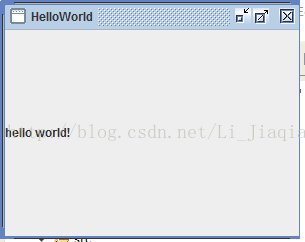
[align=justify]
[/align]
2.界面由按钮触发,输出字符:hello world!!
效果截图:
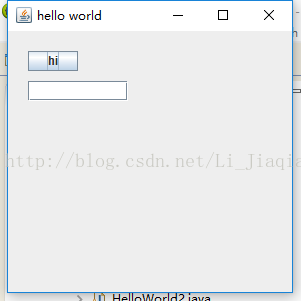
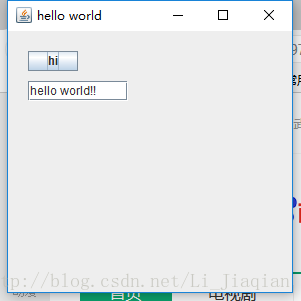
3.用户登录界面,输入用户名及密码
效果截图:
1.界面输出字符:hello world!
package vaniot.com; import javax.swing.*; public class HelloWorld { public static void createAndShow(){ //好看的界面风格 JFrame.setDefaultLookAndFeelDecorated(true); //创建及设置窗口 JFrame frame=new JFrame("HelloWorld"); //使JTable中关闭窗口后让后台程序也一起关闭,若缺少内存将会越占越满 frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //窗口显示的内容 JLabel label=new JLabel("hello world!"); frame.getContentPane().add(label); //显示窗口 frame.pack(); frame.setVisible(true); } /** * @param args */ public static void main(String[] args) { // TODO Auto-generated method stub //显示窗口 javax.swing.SwingUtilities.invokeLater(new Runnable(){ public void run(){ createAndShow(); } }); } }
[align=justify]效果截图:[/align]
[align=justify]
[/align]
2.界面由按钮触发,输出字符:hello world!!
package vaniot.com; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JTextField; public class HelloWorld2 { public static void main(String[] args)throws Exception{ NewFrame frame=new NewFrame(); frame.setDefaultCloseOperation(frame.EXIT_ON_CLOSE); frame.setVisible(true); } } class NewFrame extends JFrame{ private JButton button; private JTextField text; public NewFrame(){ super(); this.setSize(300,300); //布局 this.getContentPane().setLayout(null); //添加按钮 this.add(this.getButton(),null); this.add(this.getText(),null); this.setTitle("hello world"); } private JButton getButton() { // TODO Auto-generated method stub if(button==null){ button=new JButton(); button.setBounds(20,20,50,20); button.setText("hi"); //添加监听类 button.addActionListener(new HelloButton()); } return button; } private JTextField getText(){ if(text==null){ text=new JTextField(); text.setBounds(20,50,100,20); } return text; } private class HelloButton implements ActionListener{ public void actionPerformed(ActionEvent e){ //System.out.println("hello world!!"); text.setText("hello world!!"); } } }
效果截图:
3.用户登录界面,输入用户名及密码
package vaniot.com; import javax.swing.*; public class LoginExample { public static void placeComponents(JPanel panel){ //布局管理器 panel.setLayout(null); //user: JLabel userlabel=new JLabel("User:"); //设置组件位置,setBounds(x,y,width,height) userlabel.setBounds(10,20,80,25); panel.add(userlabel); //创建文本域用于用户输入 JTextField usertext=new JTextField(20); usertext.setBounds(100,20,165,25); panel.add(usertext); //password: JLabel pwlabel=new JLabel("password:"); pwlabel.setBounds(10,60,80,25); panel.add(pwlabel); //password输入域,输入以点号代替 JPasswordField pwtext=new JPasswordField(20); pwtext.setBounds(100,60,165,25); panel.add(pwtext); //登录按钮 JButton loginButton=new JButton("login"); loginButton.setBounds(10,100,80,25); panel.add(loginButton); } /** * @param args */ public static void main(String[] args) { // TODO Auto-generated method stub JFrame frame=new JFrame("login example"); //设置窗口大小 frame.setSize(400,400); frame.setDefaultCloseOperation(frame.EXIT_ON_CLOSE); //创建面板 JPanel panel=new JPanel(); frame.add(panel); placeComponents(panel); //界面可见 frame.setVisible(true); } }
效果截图:
相关文章推荐
- Java Swing界面编程(21)---事件处理:窗体事件
- Java Swing界面编程(29)---JCheckBox事件处理
- javaSE Swing 按钮文本框触发事件
- Java Swing界面编程(23)---事件处理:编写用户验证登录用例
- java swing中实现列表中加入单选按钮,单选按钮发生变化时能触发事件
- Java——Swing界面,接口和事件
- Java Swing界面编程(25)---事件处理:鼠标事件及监听处理
- JAVA swing之用户登陆界面
- java界面编程(4) ------ Swing事件模型
- java Swing QQ登陆界面
- Java Swing界面编程(27)---JRadioButton事件处理
- atitit.D&D drag&drop拖拽文件到界面功能 html5 web 跟个java swing c#.net c++ 的总结
- Java Swing界面编程(29)---JCheckBox事件处理
- Java Swing界面编程(21)---事件处理:窗口事件
- Java Swing界面编程(22)---事件处理:动作事件及监听处理
- atitit.D&D drag&drop拖拽文件到界面功能 html5 web 跟个java swing c#.net c++ 的总结
- JAVA-Swing图形化界面之事件监听1
- Java Swing  获取当前系统默认界面
- Java Swing界面编程(24)---事件处理:键盘事件及监听处理
- Java Swing用户登陆界面