商品展示案例
2017-04-24 19:44
441 查看
在实际开发中往往避免不了在界面上操作。在商品展示案例中我们可以在界面中对商品进行增、删、改、查。要实现这些功能需要使用ListView 和 SQLine数据库。
运行效果图:
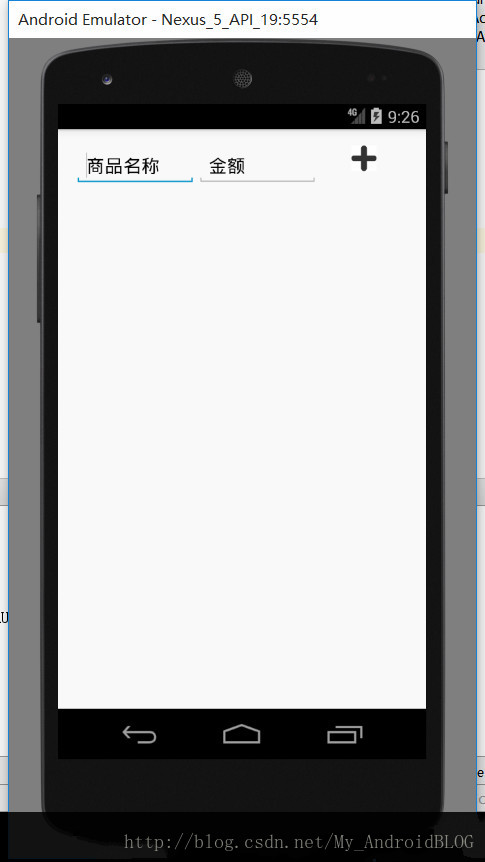
1.创建程序:首先创建一个“商品展示”的应用。设计用户交互界面。
代码如下:
创建数据库:DBHelper.xml
Goods类:Goods.Java
GoodsDao.java,用于操作数据
界面交互:MainActivity.java
2 、清单文件:
运行效果图:
1.创建程序:首先创建一个“商品展示”的应用。设计用户交互界面。
代码如下:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout android:orientation="vertical" xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.example.aaa.myapplication.MainActivity" > <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> <EditText android:id="@+id/nameET" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="1" android:hint="商品名称" android:inputType= "textPersonName" /> <EditText android:id="@+id/balanceET" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="1" android:hint="金额" android:inputType="number"/> <ImageView android:onClick="add" android:id="@+id/addIV" android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@android:drawable/ic_input_add"/> </LinearLayout> <ListView android:id="@+id/accountLV" android:layout_width="match_parent" android:layout_height="match_parent"> </ListView> </LinearLayout>
创建数据库:DBHelper.xml
package cn.edu.bzu.test02.db; import android.content.Context; import android.database.sqlite.SQLiteDatabase; import android.database.sqlite.SQLiteOpenHelper; import android.provider.Settings; public class DBHelper extends SQLiteOpenHelper { private Context context; public static final String DB_NAME = "GoodsStore.db"; public static final String CREATE_GOODS = "create table goods(id integer primary key autoincrement,name varchar(20),balance integer)";//商品名称列和金额列 public DBHelper(Context context) { super(context, DB_NAME, null, 2); } @Override public void onCreate(SQLiteDatabase db) { System.out.println("create succeed"); db.execSQL(CREATE_GOODS); } @Override public void onUpgrade(SQLiteDatabase sqLiteDatabase, int i, int i1) { System.out.println("onUpgrade succeed"); } }
Goods类:Goods.Java
public class Goods { private long id; private String name; private Integer balance; public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Integer getBalance() { return balance; } public void setBalance(Integer balance) { this.balance = balance; } public Goods(Long id, String name, Integer balance) { super(); this.id=id; this.balance = balance; this.name = name; } public Goods(String name,Integer balance){ super(); this.name=name; this.balance=balance; } public Goods(){ super(); } public String toString() { return "名称" + name + "价格" + balance; } }
GoodsDao.java,用于操作数据
package cn.edu.bzu.test02.dao; import android.content.ContentValues; import android.content.Context; import android.database.Cursor; import android.database.sqlite.SQLiteDatabase; import java.util.ArrayList; import java.util.List; import cn.edu.bzu.test02.bean.Goods; import cn.edu.bzu.test02.db.DBHelper; public class GoodsDao { private DBHelper helper; public GoodsDao(Context context) { //创建Dao时,创建Helper helper = new DBHelper(context); } public void insert(Goods goods) { //获取数据库对象 SQLiteDatabase db = helper.getWritableDatabase(); ContentValues values = new ContentValues(); values.put("name", goods.getName()); values.put("balance", goods.getBalance()); long id = db.insert("goods", null, values); goods.setId(id); db.close();//关闭数据库 } //根据id删除数据 public int detele(long id) { SQLiteDatabase db = helper.getWritableDatabase(); int count = db.delete("goods", "id=?", new String[]{id + ""}); db.close(); return count; } //更新数据 public int update(Goods goods) { SQLiteDatabase DB = helper.getWritableDatabase(); ContentValues values = new ContentValues(); values.put("name", goods.getName()); values.put("balance", goods.getBalance()); int count = DB.update("goods", values, "id=?", new String[]{goods.getId() + ""}); DB.close(); return count; } //查询所有数据倒序排列 public List<Goods> queryAll() { SQLiteDatabase db = helper.getReadableDatabase(); Cursor curor = db.query("goods", null, null, null, null, null, "balance DESC"); List<Goods> list = new ArrayList<Goods>(); while (curor.moveToNext()) { long id = curor.getLong(curor.getColumnIndex("id")); String name = curor.getString(1); int balance = curor.getInt(2); list.add(new Goods(id, name, balance)); } db.close(); curor.close(); return list; } }
界面交互:MainActivity.java
package cn.edu.bzu.test02; import android.app.Activity; import android.content.DialogInterface; import android.support.v7.app.AlertDialog; import android.os.Bundle; import android.view.View; import android.view.ViewGroup; import android.widget.AdapterView; import android.widget.BaseAdapter; import android.widget.ArrayAdapter; import android.widget.EditText; import android.widget.ImageView; import android.widget.ListView; import android.widget.TextView; import android.widget.Toast; import java.util.List; import cn.edu.bzu.test02.bean.Goods; import cn.edu.bzu.test02.dao.GoodsDao; public class MainActivity extends Activity { private ListView goodsLV; private GoodsDao goodsdao; private EditText nameET; private EditText balanceET; private MyAdapter adapter; private List<Goods> list; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); initView(); goodsdao = new GoodsDao(this); list = goodsdao.queryAll(); adapter = new MyAdapter(); goodsLV.setAdapter(adapter); } //初始化控件 private void initView() { goodsLV = (ListView) findViewById(R.id.listView); nameET = (EditText) findViewById(R.id.nameET); balanceET = (EditText) findViewById(R.id.balanceET); goodsLV.setOnItemClickListener(new MyOnItemClickListener()); } //点击事件触发 public void add(View view) { String name = nameET.getText().toString().trim(); String balance = balanceET.getText().toString().trim(); Goods goods = new Goods(name, balance.equals("") ? 0 : Integer.parseInt(balance)); goodsdao.insert(goods); list.add(goods); adapter.notifyDataSetChanged(); goodsLV.setSelection(goodsLV.getCount() - 1); nameET.setText(""); balanceET.setText(""); } /自定义一个适配器 private class MyAdapter extends BaseAdapter { @Override public int getCount() { return list.size(); } @Override public Object getItem(int position) { return list.get(position); } @Override public long getItemId(int position) { return position; } @Override public View getView(int position, View convertView, ViewGroup parent) { View item = convertView != null ? convertView : View.inflate(getApplicationContext(), R.layout.item, null); TextView idTV = (TextView) item.findViewById(R.id.idTV); TextView nameTV = (TextView) item.findViewById(R.id.nameTV); TextView balanceTV = (TextView) item.findViewById(R.id.balanceTV); final Goods g = list.get(position); idTV.setText(g.getId() + ""); nameTV.setText(g.getName()); balanceTV.setText(g.getBalance() + ""); ImageView upIV = (ImageView) item.findViewById(R.id.upIV); ImageView downIV = (ImageView) item.findViewById(R.id.downIV); ImageView deleteIV = (ImageView) item.findViewById(R.id.deleteIV); //向上箭头的点击事件 upIV.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { g.setBalance(g.getBalance() + 1); notifyDataSetChanged(); goodsdao.update(g); } }); //向下箭头的点击事件 downIV.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { g.setBalance(g.getBalance() - 1); notifyDataSetChanged(); goodsdao.update(g); } }); //删除图片的点击事件 deleteIV.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { android.content.DialogInterface.OnClickListener listener = new android.content.DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int i) { list.remove(g); goodsdao.detele(g.getId()); notifyDataSetChanged(); } }; //创建对话框 AlertDialog.Builder builder = new AlertDialog.Builder(MainActivity.this); builder.setTitle("确定要删除吗?"); builder.setPositiveButton("确定", listener); builder.setNegativeButton("取消", null); builder.show(); } }); return item; } } //ListView的点击事件 private class MyOnItemClickListener implements AdapterView.OnItemClickListener { @Override public void onItemClick(AdapterView<?> adapterView, View view, int i, long l) { Goods goods = (Goods) adapterView.getItemAtPosition(i); Toast.makeText(getApplicationContext(), goods.toString(), Toast.LENGTH_SHORT).show(); } } }
2 、清单文件:
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="cn.edu.bzu.test02"> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
相关文章推荐
- 商品展示案例
- 商品展示案例
- 商品展示案例
- SQLite数据库——案例:商品展示
- ListView和SQLite数据库案例--商品展示
- Android案例-商品展示
- 数据库的增删改查案例----商品展示
- Android 案例:商品展示
- 商品展示案例ShopShowDemo
- Android中商品展示案例
- 商品展示案例
- ListView控件以及常用数据适配器Adapter的使用+商品展示案例
- android商品展示案例FruitStore
- 商品展示案例 (数据存储和访问
- 商品展示案例
- 商品展示案例
- 简单商品展示案例(ListView)
- 案例————商品展示(SQLite数据库存储)
- SQLite数据库---ListView控件之商品展示案例
- 商品展示案例(SQLite数据库存储和ListView的使用)