二叉树面试题(二)---求一颗二叉树的镜像
2017-04-20 11:24
232 查看
一、二叉树的镜像就是一个树在镜子里的成像;好吧!初中的物理知识来了,哈哈,其实很简单,采用递归的方法求二叉树的镜像:
(1)如果树为空,直接返回NULL;
(2)如果树不为空,求其左子树和右子树的镜像,递归完成后,将左子树的镜像放在根结点的右边,将右子树的镜像放在根结点的左边;
二、图说
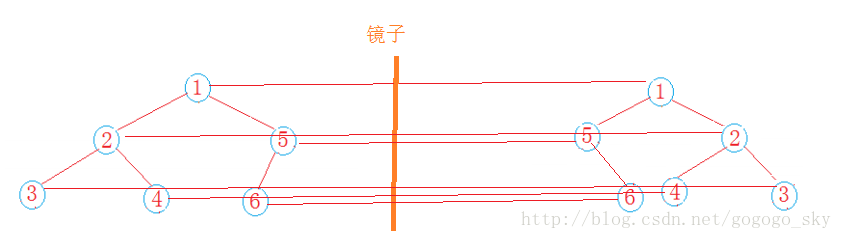
三:代码实现
测试代码:
四、运行结果
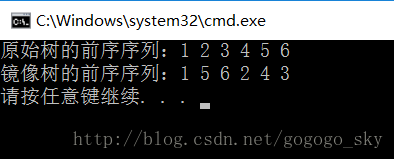
O(∩_∩)O
(1)如果树为空,直接返回NULL;
(2)如果树不为空,求其左子树和右子树的镜像,递归完成后,将左子树的镜像放在根结点的右边,将右子树的镜像放在根结点的左边;
二、图说
三:代码实现
#include<iostream> using namespace std; #include<assert.h> template<class T> struct TreeNode { TreeNode( const T& data=T()) :_data(data) ,_left(NULL) ,_right(NULL) {} int _data; TreeNode<T>* _left; TreeNode<T>* _right; }; template<class T> class BinaryTree { typedef TreeNode<T> Node; public: BinaryTree()//无参构造函数 :_root(NULL) {} BinaryTree(const T* arr,int sz,const T invalid)//有参构造函数 { assert(arr); int index=0;//数组中的位置 _root=BuildTree(arr,sz,invalid,index); } void Printf()//前序打印 { _Printf(_root); } void MirrorPrintf()//打印二叉树镜像 { _Printf(_MirrorImage(_root)); } protected: Node* BuildTree(const T* arr,int sz,const T invalid,int& index)//前序建树(别忘了index为引用) { if (index<sz && arr[index]!=invalid)//没有走完数组,且不是非法值 { Node* root=new Node(arr[index]); root->_left=BuildTree(arr,sz,invalid,++index); root->_right=BuildTree(arr,sz,invalid,++index); return root; } return NULL; } void _Printf(Node* root) { if (root) { cout<<root->_data<<" "; _Printf(root->_left); _Printf(root->_right); } } Node* _MirrorImage(Node* root)//求一颗二叉树的镜像 { if (NULL==root)//树为空 { return NULL; } else//树不为空 { Node* pleft=_MirrorImage(root->_left); Node* pright=_MirrorImage(root->_right); root->_left=pright; root->_right=pleft; return root; } } private: Node* _root; };
测试代码:
void Test() { int arr1[] = {1, 2, 3, '#', '#', 4, '#' , '#', 5, 6}; int sz1=sizeof(arr1)/sizeof(arr1[0]); BinaryTree<int> bt1(arr1,sz1,'#');//调用带有参数的构造函数 cout<<"原始树的前序序列:"; bt1.Printf(); cout<<endl; cout<<"镜像树的前序序列:"; bt1.MirrorPrintf(); cout<<endl; }
四、运行结果
O(∩_∩)O
相关文章推荐
- 剑指offer面试题19——二叉树的镜像
- 剑指offer面试题27:二叉树的镜像
- 求一颗二叉树的镜像
- 面试题19 二叉树的镜像
- 剑指offer阅读笔记 之面试题19 实现二叉树的镜像 之 又复习一遍二叉树
- [剑指offer][面试题19]二叉树的镜像
- 剑指offer--面试题19:二叉树的镜像
- 剑指offer面试题19:二叉树的镜像
- 面试题19:二叉树的镜像
- 【面试题十九】二叉树的镜像
- 《剑指Offer》学习笔记--面试题19:二叉树的镜像
- Java 剑指offer_面试题19_二叉树的镜像
- 面试题19:二叉树的镜像
- 判断一棵二叉树是否是平衡二叉树/求一颗二叉树的镜像
- 面试题19二叉树的镜像
- 面试题19 二叉树的镜像
- 剑指offer——面试题19:二叉树的镜像
- 面试题19_二叉树的镜像——剑指offer系列
- 【剑指Offer面试题】 九度OJ1521:二叉树的镜像