图的遍历之广度优先搜索和深度优先搜索
2017-03-20 22:35
316 查看
广度优先搜索:从一个顶点开始,搜索所有可到达顶点的方法。利用队列的方法实现
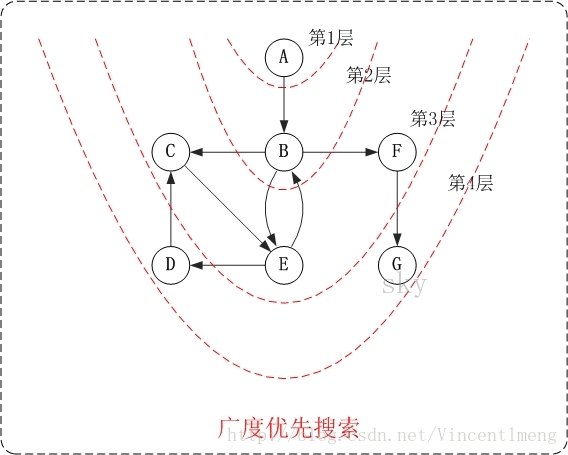
深度优先搜索:从一个顶点出发,向下搜索,到达没有邻接点就向上返回。
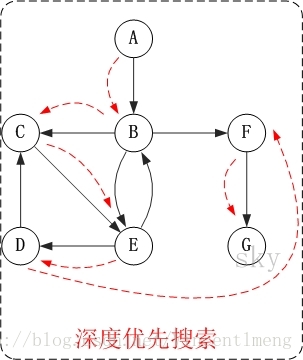
下面给出代码:
深度优先搜索:从一个顶点出发,向下搜索,到达没有邻接点就向上返回。
下面给出代码:
#include<iostream> #include<stack> #include<queue> #define MAX_VERTS 20 using namespace std; class Vertex//顶点 { public: //char Label; Vertex(char lab) { Label = lab; wasVisited = false; } public: bool wasVisited; char Label;//顶点字符 }; class Graph//图 { public: Graph(); ~Graph(); void addVertex(char lab);//增加一个顶点 void addEdge(int start, int end);//增加一条边 void printMatrix();//打印矩阵 void showVertex(int v); void DFS();//深度优先搜索 void BFS(); private: Vertex* vertexList[MAX_VERTS];//指针数组 int nVerts;//实际中顶点的个数 int adjMat[MAX_VERTS][MAX_VERTS];//做邻接矩阵的数组 int getAdjUnvisitedVertex(int v);//邻接的未访问的顶点 }; void Graph::DFS() { stack<int>gStack;//保存的堆栈的下标 vertexList[0]->wasVisited = true;//从第一个顶点开始 showVertex(0); gStack.push(0);//把访问过的压入堆栈 int v; while (gStack.size() > 0)//当堆栈为空是结束 { v = getAdjUnvisitedVertex(gStack.top());//v是顶点下标 if (v == -1) gStack.pop();//向回反 else { vertexList[v]->wasVisited = true; showVertex(v); gStack.push(v); } } cout << endl; for (int j = 0; j < nVerts; j++) vertexList[j]->wasVisited = false; } void Graph::BFS() { queue<int >gQueue; vertexList[0]->wasVisited = true;//从第一个下标开始 showVertex(0); gQueue.push(0);//访问过的每一个顶点都要放入队列 int vert1, vert2; while (gQueue.size()>0)//如果队列中有顶点 { vert1 = gQueue.front();//把A拿出来 gQueue.pop();//把A删除掉 vert2 = getAdjUnvisitedVertex(vert1);//找与A相邻接的 while (vert2 != -1)//找出所有与A相邻接的 { vertexList[vert2]->wasVisited = true;//标记为访问过 showVertex(vert2);//显示 gQueue.push(vert2);//放入队列 vert2 = getAdjUnvisitedVertex(vert1); } } cout << endl; for (int j = 0; j < nVerts;j++) vertexList[j]->wasVisited = false; } int Graph::getAdjUnvisitedVertex(int v) { for (int j = 0; j < nVerts; j++) { if ((adjMat[v][j] == 1) && (vertexList[j]->wasVisited == false)) return j; } return -1; } void Graph::showVertex(int v) { cout << vertexList[v]->Label << " "; } Graph::Graph() { nVerts = 0; for (int i = 0; i < MAX_VERTS; i++) for (int j = 0; j < MAX_VERTS; j++) adjMat[i][j] = 0; } void Graph::addVertex(char lab) { vertexList[nVerts++] = new Vertex(lab); } void Graph::addEdge(int start, int end) { adjMat[start][end] = 1; adjMat[end][start] = 1; } void Graph::printMatrix() { for (int i = 0; i < nVerts; i++) { for (int j = 0; j < nVerts; j++) cout << adjMat[i][j] << " "; cout << endl; } } Graph::~Graph() { for (int i = 0; i < nVerts; i++) delete vertexList[i]; } int main() { Graph g; g.addVertex('A');//0 g.addVertex('B');//1 g.addVertex('C');//2 g.addVertex('D');//3 g.addVertex('E');//4 g.addEdge(0, 1);//A_B g.addEdge(0, 3);//A-D g.addEdge(1, 0);//B-A g.addEdge(1, 4);//B-E g.addEdge(2, 4);//C-E g.addEdge(3, 0);//C-A g.addEdge(3, 4);//C-D g.addEdge(4, 1);//E-B g.addEdge(4, 2);//E-C g.addEdge(4, 3);//E-D g.printMatrix(); cout << "深度优先搜索的结果:" << endl; g.DFS(); cout << "广度优先搜索的结果:" << endl; g.BFS(); system("pause"); return 0; }
相关文章推荐
- 图的遍历之广度优先搜索和深度优先搜索
- 重学数据结构系列之——图的遍历(广度优先搜索和深度优先搜索)
- sdutacm-数据结构实验之图论一:基于邻接矩阵的广度优先搜索遍历
- 数据结构——图(2),深度优先搜索和广度优先搜索
- 数据结构实验之图论二:基于邻接表的广度优先搜索遍历
- 图的深度优先搜索DFS和广度优先搜索BFS
- 数据结构实验图论:基于邻接矩阵/邻接表的广度优先搜索遍历
- 深度优先搜索遍历与广度优先搜索遍历
- 广度优先搜索——BFS遍历
- 数据结构实验之图论一:基于邻接矩阵的广度优先搜索遍历
- 数据结构实验图论一:基于邻接矩阵的广度优先搜索遍历
- 数据结构实验图论一:基于邻接矩阵的广度优先搜索遍历
- 数据结构实验之图论二:基于邻接表的广度优先搜索遍历
- 【经典算法】图的深度优先搜索和广度优先搜索
- 数据结构实验图论一:基于邻接矩阵的广度优先搜索遍历
- 数据结构实验之图论二:基于邻接表的广度优先搜索遍历
- 图遍历之广度优先搜索
- 广度优先搜索——马的遍历
- java学习笔记之图的遍历(广度优先搜索BFS)
- 数据结构实验图论一:基于邻接矩阵的广度优先搜索遍历