Spring Cloud 基于eureka 服务注册中心
2017-03-16 20:06
621 查看
netflix 下开源组件eureka 提供了微服务注册和发现的解决方案,Spring整合了netflix 下若干组件用于快速构建微服务集群.本节围绕eureka
展开,举例示意使用eureka实现微服务注册和发现的基本操作.
服务注册中心,基于eureka-server 提供,Spring为其提供了简洁的服务管理界面.
依赖如下:
建议添加如下依赖管理
服务注册中心的启用: 由核心注解@EnableEurekaServer 开启服务注册中心,当前项目仅仅作为注册中心使用不提供此外的其它服务.
访问注册中心主管理界面:http://localhost:8761/ ,ip和端口均为注册中心配置的参数,当前没有服务注册
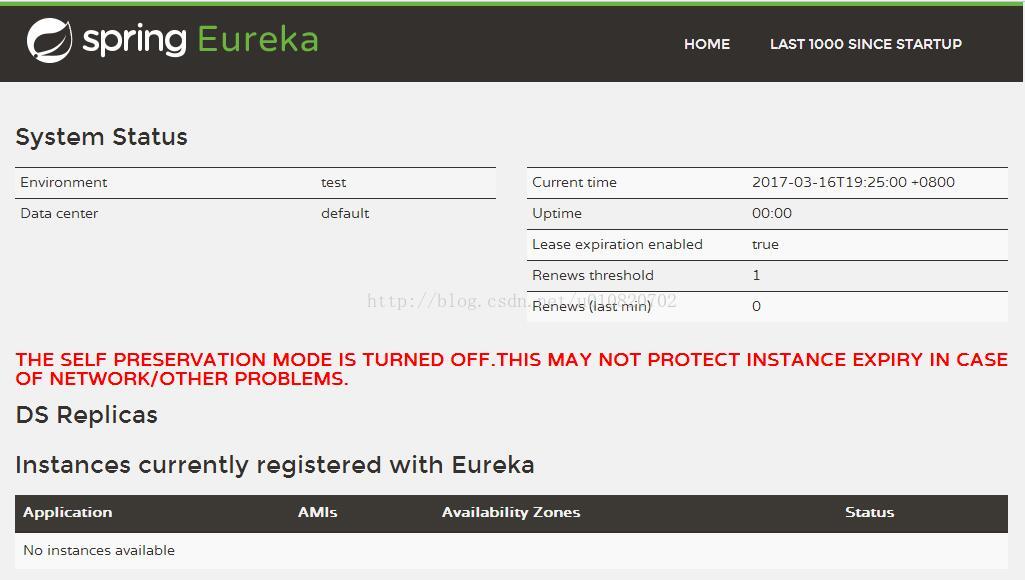
微服务注册,基于eureka和基于zookeeper的注册过程及配置方式有所不同,微服务消费的方式也略有不同,需要加以区分.
依赖如下:
作为服务提供者至少需要启用如下注解:
该服务简单暴露一下接口用于消费.
服务启动完毕后,刷新注册中心界面,发现已注册的服务及相关信息.
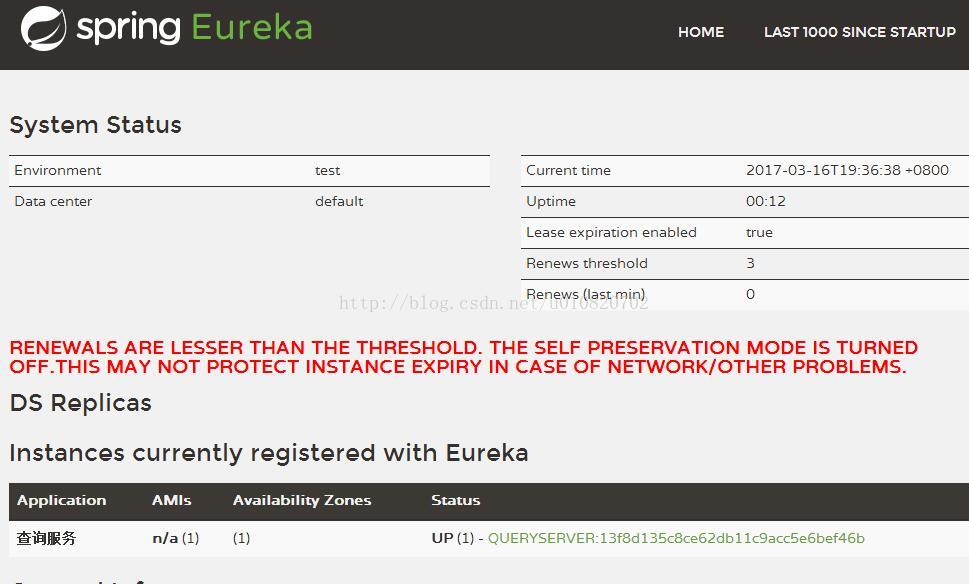
服务消费,下述依赖中部分特性暂时为使用,对于一个完整的微服务放来来说后续有必要进行补充.
依赖:
核心注解,下列@EnableEurekaClient 用于标识获取服务,@EnableFeignClients 启用REST 风格的方式访问服务暴露的接口restTemplate()方法为IOC容器主意RestTemplate Bean实例.
定义服务接口,该例中引入了FeignClients 用于以REST风格同 服务提供者之间进行交互. Feign 允许通过注解的方式来定位服务及访问服务的URL来获取数据,非常便利,极大的简化了传统RPC通信接口需要统一的弊端.
展开,举例示意使用eureka实现微服务注册和发现的基本操作.
服务注册中心,基于eureka-server 提供,Spring为其提供了简洁的服务管理界面.
依赖如下:
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-eureka</artifactId> <scope>runtime</scope> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.cloud/spring-cloud-starter-eureka-server --> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-eureka-server</artifactId> <scope>runtime</scope> </dependency>
建议添加如下依赖管理
<dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-netflix</artifactId> <version>1.2.5.RELEASE</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement>
服务注册中心的启用: 由核心注解@EnableEurekaServer 开启服务注册中心,当前项目仅仅作为注册中心使用不提供此外的其它服务.
@SpringBootApplication @EnableEurekaServer public class App { public static void main(String[] args) { SpringApplication.run(App.class, args); } }服务注册中心配置:Eureka 注册中心避免单点故障采用了对等架构的配置方式,使用yml配置文件能大大简化服务的配置,此处可以定义多个配置文件,指定之间的对等关系,逐个启动不同的节点即可,建议至少启动两个以上的节点.见下application.yml ,至此注册中心搭建完毕.
server: port: 8761 spring: profiles: #当前激活的配置文件 active: peer1 --- #配置文件1 spring: profiles: peer1 eureka: instance: appname: ServerRegister hostname: localhost instance-id: ${spring.application.name}:${random.value} server: #关闭自我保护 enable-self-preservation: false #清除无效节点的时间间隔 eviction-interval-timer-in-ms: 4000 client: register-with-eureka: true fetch-registry: false service-url: defaultZone: http://${eureka.instance.hostname}:${server.port}/eureka/ --- #配置文件2 spring: profiles: peer2 eureka: instance: appname: ServerRegister hostname: localhost instance-id: ${spring.application.name}:${random.value} server: enable-self-preservation: false client: register-with-eureka: true fetch-registry: false service-url: defaultZone: http://${eureka.instance.hostname}:${server.port}/eureka/
访问注册中心主管理界面:http://localhost:8761/ ,ip和端口均为注册中心配置的参数,当前没有服务注册
微服务注册,基于eureka和基于zookeeper的注册过程及配置方式有所不同,微服务消费的方式也略有不同,需要加以区分.
依赖如下:
<dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-eureka</artifactId> </dependency> </dependencies
作为服务提供者至少需要启用如下注解:
@SpringBootApplication @EnableDiscoveryClient @ComponentScan("cn.com.xiaofen") public class ItgsQueryApplication { public static void main(String[] args) { SpringApplication.run(ItgsQueryApplication.class, args); } }配置服务注册到服务注册中心的参数,以及注册中心的位置. 需要主意的是此处标识是相同服务的应该是virtual-host-name ,同一个服务的不同节点分布应该具有不同的instance-id
server: port: 9911 spring: 4000 application: name: QUERYSERVER eureka: instance: #虚拟主机名称 virtual-host-name: QueryServer #心跳时间间隔 lease-renewal-interval-in-seconds: 10 #无心跳多久后,该服务失效 lease-expiration-duration-in-seconds: 30 appname: 查询服务 #Server实例ID instance-id: ${spring.application.name}:${random.value} client: service-url: #默认的服务注册地址 defaultZone: http://host1:8761/eureka/,http://host2:8761/eureka/
该服务简单暴露一下接口用于消费.
@RestController public class QueryController { @RequestMapping("/query/{id}") public String query(@PathVariable String id) { return "{random:" + Math.random() + ",id:" + id + "}"; } }
服务启动完毕后,刷新注册中心界面,发现已注册的服务及相关信息.
服务消费,下述依赖中部分特性暂时为使用,对于一个完整的微服务放来来说后续有必要进行补充.
依赖:
<dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-eureka</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-feign</artifactId> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.cloud/spring-cloud-starter-ribbon --> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-ribbon</artifactId> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.cloud/spring-cloud-starter-hystrix --> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-hystrix</artifactId> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.cloud/spring-cloud-starter-hystrix-dashboard --> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-hystrix-dashboard</artifactId> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.cloud/spring-cloud-starter-ribbon --> </dependencies>
核心注解,下列@EnableEurekaClient 用于标识获取服务,@EnableFeignClients 启用REST 风格的方式访问服务暴露的接口restTemplate()方法为IOC容器主意RestTemplate Bean实例.
@SpringBootApplication @EnableEurekaClient @EnableFeignClients @RibbonClient(name = "app", configuration = RibbonConfig.class) public class ClientApplication { public static void main(String[] args) { SpringApplication.run(ClientApplication.class, args); } @Bean RestTemplate restTemplate() { return new RestTemplate(); } }
定义服务接口,该例中引入了FeignClients 用于以REST风格同 服务提供者之间进行交互. Feign 允许通过注解的方式来定位服务及访问服务的URL来获取数据,非常便利,极大的简化了传统RPC通信接口需要统一的弊端.
@FeignClient(value = "QueryServer") public interface QueryInterface { @RequestMapping(value = "/query/{id}") public String query(@PathVariable("id") String id); }访问微服,获取服务提供的数据.
@RestController public class TestFeginController { //自动注入服务接口对象,该对象由Fegin框架代理等同于访问接口中申明的服务 @Autowired QueryInterface service; @RequestMapping("/query/{id}") public String query(@PathVariable("id") String id) { return service.query(id); } }配置文件application.yml ,此处主要体现服务注册中心的地址
server: port: 7777 eureka: client: #当前程序不注册到eureka register-with-eureka: false #抓取注册中心的服务列表 fetch-registry: true service-url: defaultZone: http://host1:8761/eureka/,http://host2:8761/eureka/[/code]
访问测试:
关于Spring Cloud 和 阿里Dobbo
个人更倾向于新技术,可以说未来几年基于Spring Cloud的微服务开发会形成一种趋势,Spring Cloud 为微服务提供了一站式的解决方案. 经验有限不能瞎说 可参考如下:
http://blog.didispace.com/microservice-framework/
相关文章推荐
- Spring Cloud系列一 之 eureka服务注册中心
- 用ZooKeeper做为注册中心搭建基于Spring Cloud实现服务注册与发现
- Spring Cloud (19) | Eureka Server 高可用服务注册中心
- 【Spring Cloud】二、Eureka Client 服务注册中心客户端启动注册服务
- springboot填坑之 -- spring cloud基于ip的discovery服务注册中心配置
- springboot填坑之 -- spring cloud基于ip的discovery服务注册中心配置
- 搭建基于Spring Cloud的微服务注册中心
- Spring Cloud(Dalston.SR5)--Eureka 注册中心高可用-服务提供和消费
- 【Spring Cloud】一、Eureka Server 服务注册中心服务端启动
- (1) 服务治理Eureka注册中心
- 【Spring Cloud】Eureka服务注册中心搭建
- 【Spring Cloud】Eureka服务注册中心搭建
- 【Spring Cloud】Eureka服务注册中心搭建
- spring cloud(二)服务(注册)中心Eureka
- Spring-Cloud-Eureka服务注册发现中心server+client案列模拟说明
- 基于spring-cloud实现eureka注册服务小案例
- Spring Cloud 构建微服务-高可用注册中心
- 将spring boot服务注册到Eureka注册中心
- Spring Cloud 多网卡环境下Eureka服务注册IP选择问题
- Spring Cloud Eureka 服务注册与发现中心(一)