java学生信息管理系统MVC架构
2017-03-12 10:17
525 查看
一、项目结构
学生信息管理系统分三层进行实现。student.java主要提供数据,cotroller.java的功能是绑定试图和计算数据。Stuview.java用于单一的用来显示数据。
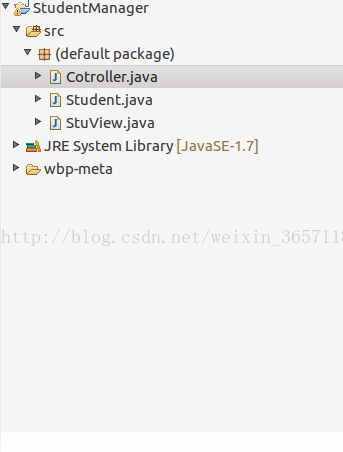
二、源码
1.1、Student 类
1.2、Cotroller类
1.3、StuView类
三、运行效果(初始界面、添加界面、刷新界面)
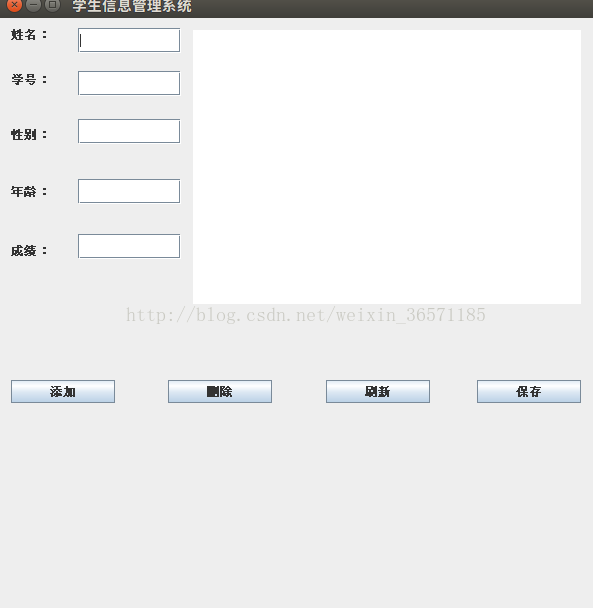
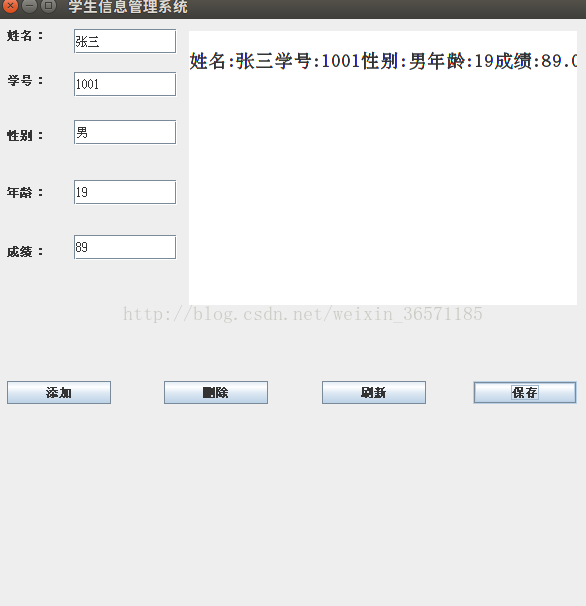
、
学生信息管理系统分三层进行实现。student.java主要提供数据,cotroller.java的功能是绑定试图和计算数据。Stuview.java用于单一的用来显示数据。
二、源码
1.1、Student 类
/* * @FileName: Student.class * @version:1.0 * @author:nazi * 描述:模型层 * */ import java.io.Serializable; /* * Summary: Student类实现序列化接口,用于对象的保存 * @author:nazi * @version:1.0 * */ public class Student implements Serializable { //序列化id private static final long serialVersionUID = 9088453456517873574L; int num; String name; String sex; int age; float grade; public Student(int num ,String nameString,String sexString,int g,float f){ this.num =num; name = nameString; sex =sexString; age =g; grade =f; } public int getNum(){ return num; } public String getName(){ return name; } public String getSex(){ return sex; } public int getAge(){ return age; } public float getGrades(){ return grade; } public String toString(){ return "姓名:"+name+"学号:"+num+"性别:"+sex+"年龄:"+age+"成绩:"+grade; } }
1.2、Cotroller类
/* * 文件名: Cotroller.java * 描述:mvc中的c,用来管理模型层的数据 * @authur:Nazi * function :增、删、改、查、保存、更新 * */ import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; import java.util.ArrayList; import java.util.Iterator; /* * Cotroller类集中对ArrayList<Student>进行操作 * @Author nazi * @version 1.0 * */ public class Cotroller { //student数据集合 private ArrayList<Student> list; public Cotroller(ArrayList<Student> l){ this.list =l; } /* * rturn a ArrayList<Student> * */ public ArrayList<Student> getList() { return list; } /* * 初始化Student数组 * */ public void setList(ArrayList<Student> list) { this.list = list; } /* * add a student to the List * */ public void add(Student s) { list.add(s); } /* * remove the student from list * */ public void remove(int id) { for(Iterator<Student> iter = list.iterator(); iter.hasNext();) { Student s = iter.next(); if(s.getNum() == id) { list.remove(s); } } } /* * print the specific student * */ public String printAll(int i) { return list.get(i).toString(); } /* * 功能简述:将实现序列化后的对象写入到文件中。 * 文件输出地址:"/home/nazi/2.txt" 文件地址可以更改 * */ public void fileOt() throws FileNotFoundException{ FileOutputStream fo = new FileOutputStream("/home/nazi/2.txt"); try { ObjectOutputStream so = new ObjectOutputStream(fo); so.writeObject(list); so.close(); } catch (IOException e) { e.printStackTrace(); } } /* * function: 从指定路径读取文件,然后将对象状态进行赋值使用 * * */ @SuppressWarnings("unchecked") public void fileIn() throws FileNotFoundException{ FileInputStream fi = new FileInputStream("/home/nazi/2.txt"); try { ObjectInputStream si = new ObjectInputStream(fi); list = (ArrayList<Student>) si.readObject(); si.close(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (ClassNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } } }
1.3、StuView类
/* * FileName:StuView.class * 描述:以特定的方式展示数据 * @Atuthor:nazi * @version:1.0 * */ import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.io.FileNotFoundException; import java.util.ArrayList; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JTextArea; import javax.swing.JTextField; /* * StuView 类用于展示数据 * @author:nazi * @version:1.0 * */ public class StuView { private static Cotroller cotroller; public static void main(String args[]){ //创建管理者 cotroller = new Cotroller(new ArrayList<Student>()); //界面 initFrame(); } /* * InitFrame()中含有各种类型的控件,以及控件所对应的事件处理步骤 * */ protected static void initFrame(){ JFrame frame = new JFrame("学生信息管理系统"); frame.setSize(600,600); frame.setLocation(500, 100); frame.setLayout(null); //生成组件 final JTextField name = new JTextField(); name.setBounds(79, 10, 103, 25); final JTextField num = new JTextField(); num.setBounds(79, 53, 103, 25); final JTextField sex = new JTextField(); sex.setBounds(79, 101, 103, 25); final JTextField age = new JTextField(); age.setBounds(79, 161, 103, 25); final JTextField g1 = new JTextField(); g1.setBounds(79, 216, 103, 25); final JTextArea show = new JTextArea(); show.setBounds(194, 12, 388, 274); frame.add(show); show.setFont(new Font("Serif",Font.BOLD,18)); frame.add(show); frame.add(name); frame.add(num); frame.add(sex); frame.add(age); frame.add(g1); frame.add(show); JLabel label = new JLabel("学号:"); label.setBounds(12, 55, 63, 13); frame.getContentPane().add(label); JLabel label_1 = new JLabel("姓名:"); label_1.setBounds(12, 10, 63, 13); frame.getContentPane().add(label_1); JLabel label_2 = new JLabel("性别:"); label_2.setBounds(12, 110, 63, 13); frame.getContentPane().add(label_2); JLabel label_3 = new JLabel("年龄:"); label_3.setBounds(12, 167, 63, 13); frame.getContentPane().add(label_3); JLabel label_4 = new JLabel("成绩:"); label_4.setBounds(12, 226, 70, 13); frame.getContentPane().add(label_4); //添加学生 JButton btnAdd =new JButton("添加"); btnAdd.setBounds(12, 362, 104, 23); frame.add(btnAdd); btnAdd.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent arg0) { Student s1 = new Student(Integer.parseInt(num.getText()),name.getText(), sex.getText(),Integer.parseInt(age.getText()),Integer.parseInt(g1.getText())); //放到集合 cotroller.getList().add(s1); //打印 for(int i = 0;i<cotroller.getList().size();i++){ show.append("\n"); show.append(cotroller.printAll(i)); } } }); //保存为文件 JButton btnSave =new JButton("保存");; btnSave.setBounds(478, 362, 104, 23); frame.add(btnSave); btnSave.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent arg0) { try { cotroller.fileOt(); } catch (FileNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } } }); //刷新 JButton btnRefresh = new JButton("刷新"); btnRefresh.setBounds( 4000 327, 362, 104, 23); frame.add(btnRefresh); btnRefresh.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent arg0) { try { cotroller.fileIn(); } catch (FileNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } //打印 for(int i = 0;i<cotroller.getList().size();i++){ show.append("\n"); show.append(cotroller.printAll(i)); } } }); //删除 JButton button_1 = new JButton("删除"); button_1.setBounds(169, 362, 104, 23); button_1.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent arg0) { // TODO Auto-generated method stub } }); frame.add(button_1); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); } }
三、运行效果(初始界面、添加界面、刷新界面)
、
相关文章推荐
- java学生信息管理系统MVC架构详解
- java学生信息管理系统源代码
- 用java开发学生信息管理系统(SIMS,五)
- java学生信息管理系统设计
- Java+MySQL实现学生信息管理系统源码
- JAVA学生信息管理系统(数据库版)
- 用java开发学生信息管理系统(SIMS,一)
- 学生信息管理系统--(Java+MySQL实现)
- 【学习日记】MVC架构+DAO设计小型信息管理系统(一)
- Java 实现学生信息管理系统
- Java(学生信息管理系统)(源代码)
- java写的迷你学生信息管理系统
- Java写的学生信息管理系统
- java学生信息管理系统设计(2)
- 学生信息管理系统--java
- 用java开发学生信息管理系统(SIMS,四)
- Android(java)学习笔记195:学生信息管理系统案例(SQLite + ListView)
- 用java开发学生信息管理系统(SIMS,二)
- 学生信息管理系统【JavaWeb】SSH+Mysql+Jsp
- 学生信息管理系统--(Java+MySQL实现)