Spring基于xml配置bean AOP
2017-01-04 00:00
337 查看
摘要: Spring基于xml配置bean AOP
1.。ArithmeticCalculator.java
2。ArithmeticCalculatorImpl.java
3。LoggingAspect.java
4。TestAop.java
5。VlitationAspect.java
applicationContext_xml.xml
运行:
1.。ArithmeticCalculator.java
package com.huangliusong.spring.aop.xml; public interface ArithmeticCalculator { int add(int i, int j); int sub(int i, int j); int mul(int i, int j); int div(int i, int j); }
2。ArithmeticCalculatorImpl.java
package com.huangliusong.spring.aop.xml; public class ArithmeticCalculatorImpl implements ArithmeticCalculator { @Override public int add(int i, int j) { int result = i + j; return result; } @Override public int sub(int i, int j) { int result = i - j; return result; } @Override public int mul(int i, int j) { int result = i * j; return result; } @Override public int div(int i, int j) { int result = i / j; return result; } }
3。LoggingAspect.java
package com.huangliusong.spring.aop.xml; import java.util.Arrays; import org.aspectj.lang.JoinPoint; import org.aspectj.lang.ProceedingJoinPoint; import org.aspectj.lang.annotation.After; import org.aspectj.lang.annotation.AfterReturning; import org.aspectj.lang.annotation.AfterThrowing; import org.aspectj.lang.annotation.Around; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Before; import org.aspectj.lang.annotation.Pointcut; import org.springframework.stereotype.Component; public class LoggingAspect { public void beforeMethod(JoinPoint joinPoint) { String methodName = joinPoint.getSignature().getName(); Object[] args = joinPoint.getArgs(); System.out.println("方法开始——————" + methodName + Arrays.asList(args)); } public void afterMethod(JoinPoint joinPoint) { String methodName = joinPoint.getSignature().getName(); Object[] args = joinPoint.getArgs(); System.out.println("方法结束——————" + methodName + Arrays.asList(args)); } public void afterRunning(JoinPoint joinPoint, Object result) { String methodName = joinPoint.getSignature().getName(); Object[] args = joinPoint.getArgs(); System.out.println("方法结束——————" + methodName + Arrays.asList(args) + result); } public void afterThrowing(JoinPoint joinPoint, Exception ex) { String methodName = joinPoint.getSignature().getName(); Object[] args = joinPoint.getArgs(); System.out .println("方法结束——————" + methodName + Arrays.asList(args) + ex); } public Object aroundMethod(ProceedingJoinPoint point) { Object result = null; String methodName = point.getSignature().getName(); // 执行目标方法 try { // 前置通知 System.out.println("aroundMethod-" + methodName + "-begin with " + Arrays.asList(point.getArgs())); result = point.proceed(); // 后置通知 System.out.println("aroundMethod-" + methodName + " -end with " + Arrays.asList(point.getArgs())); } catch (Throwable e) { // 异常通知 System.err.println(e); e.printStackTrace(); } return result; } }
4。TestAop.java
package com.huangliusong.spring.aop.xml; import org.junit.Test; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class TestAop { @Test public void test1() { ApplicationContext ctx = new ClassPathXmlApplicationContext( "applicationContext_xml.xml"); ArithmeticCalculator arithmeticCalculator = (ArithmeticCalculator) ctx .getBean("arithmeticCalculator"); System.err.println(arithmeticCalculator.getClass().getName()); int result = arithmeticCalculator.add(11, 11); System.out.println("结果" + result); result = arithmeticCalculator.div(12, 10); System.out.println("结果" + result); } }
5。VlitationAspect.java
package com.huangliusong.spring.aop.xml; import java.util.Arrays; import org.aspectj.lang.JoinPoint; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Before; import org.springframework.core.annotation.Order; import org.springframework.stereotype.Component; /** * 验证通知 可以指定切面的优先级 * 优先级使用Order(1)值越小优先级越高 * @author Administrator * */ public class VlitationAspect { public void validateArgs(JoinPoint joinPoint){ System.err.println("validateArgs"+Arrays.asList(joinPoint.getArgs())); } }
applicationContext_xml.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.1.xsd http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.1.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.1.xsd"> <!-- 配置bean --> <bean id="arithmeticCalculator" class="com.huangliusong.spring.aop.xml.ArithmeticCalculatorImpl"></bean> <!-- 配置切面的bean --> <bean id="loggingAspect" class="com.huangliusong.spring.aop.xml.LoggingAspect"></bean> <bean id="vlidationAspect" class="com.huangliusong.spring.aop.xml.VlitationAspect"></bean> <!-- 配置AOP --> <aop:config> <!-- 配置切点表达式 --> <aop:pointcut expression="execution(public * com.huangliusong.spring.aop.xml.*.*(..))" id="pointcut"/> <!-- 配置切面以及通知 --> <aop:aspect ref="loggingAspect" order="2"> <aop:before method="beforeMethod" pointcut-ref="pointcut"/> <aop:after method="afterMethod" pointcut-ref="pointcut"/> </aop:aspect> <aop:aspect ref="vlidationAspect" order="1"> <aop:before method="validateArgs" pointcut-ref="pointcut"/> </aop:aspect> </aop:config> </beans>
运行:
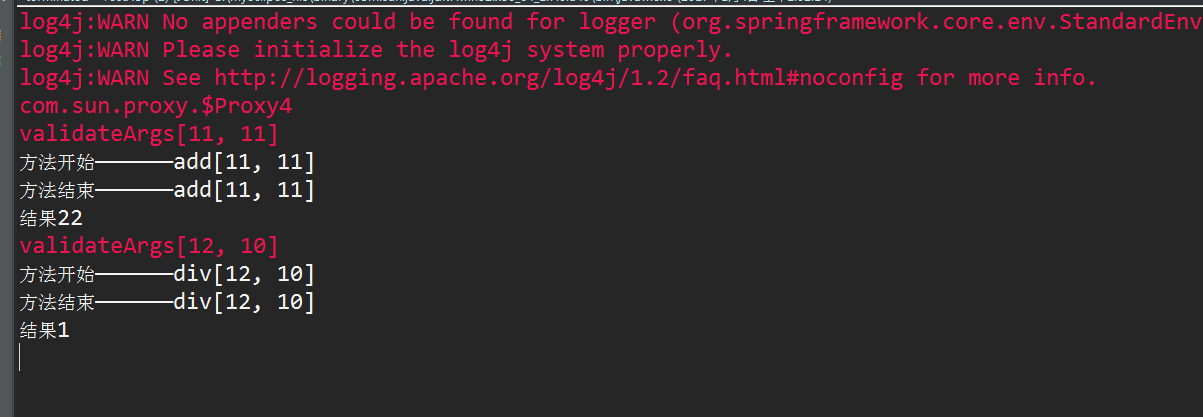
相关文章推荐
- Spring AOP---基于Xml配置应用demo
- springAOP基于xml的配置前后环绕通知
- Spring3.2 中 Bean 定义之基于 XML 配置方式的源码解析
- spring aop的使用(注解方式以及基于xml配置方式)
- Spring3.2 中 Bean 定义之基于 XML 配置方式的源码解析
- Spring的AOP配置(基于xml)
- 基于XML的Spring AOP配置
- spring添加AOP切面-基于XML配置
- spring aop 基于xml配置版
- spring aop的使用(注解方式以及基于xml配置方式)
- Spring3.2 中 Bean 定义之基于 XML 配置方式的源码解析
- 一步一步深入spring(6)--使用基于XML配置的spring实现的AOP
- (一)Spring AOP:基于XML配置文件
- Spring 基于xml配置方式的AOP
- 8.4.6: Spring的AOP---基于XML配置文件的管理方式
- Spring4 学习笔记(3)-Spring 基于 XML 的方式配置 Bean
- Spring 基于XML配置的AOP入门案例
- spring注解时AOP失效,XML配置<bean>正常
- spring aop的使用(注解方式以及基于xml配置方式)
- Spring AOP使用Aspectj基于xml方式,初始化Bean参数