天气查看器
2016-12-25 19:34
489 查看
一、运行结果图
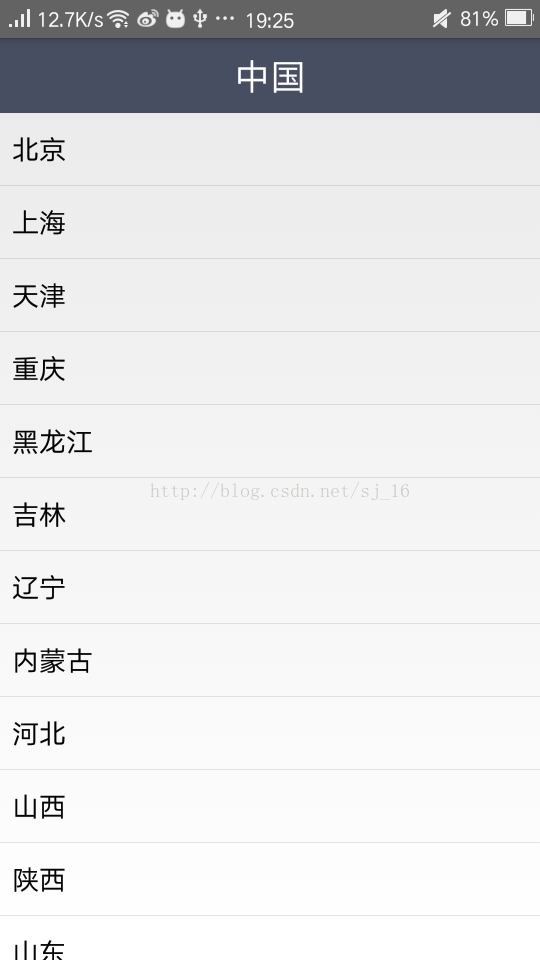
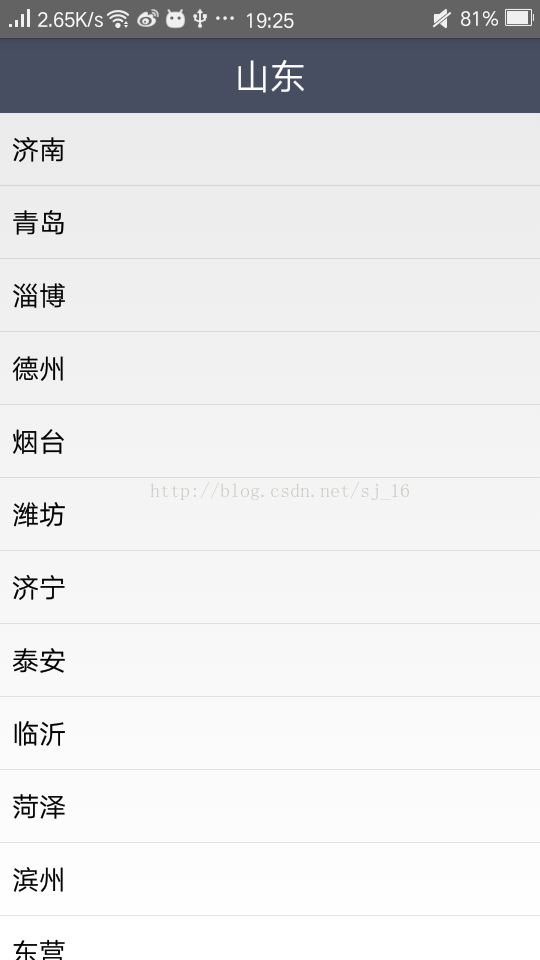
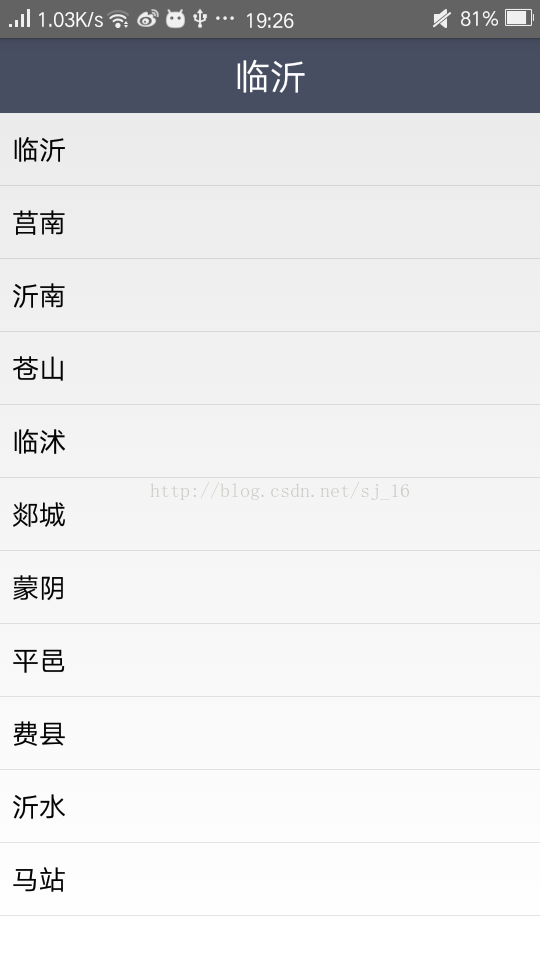
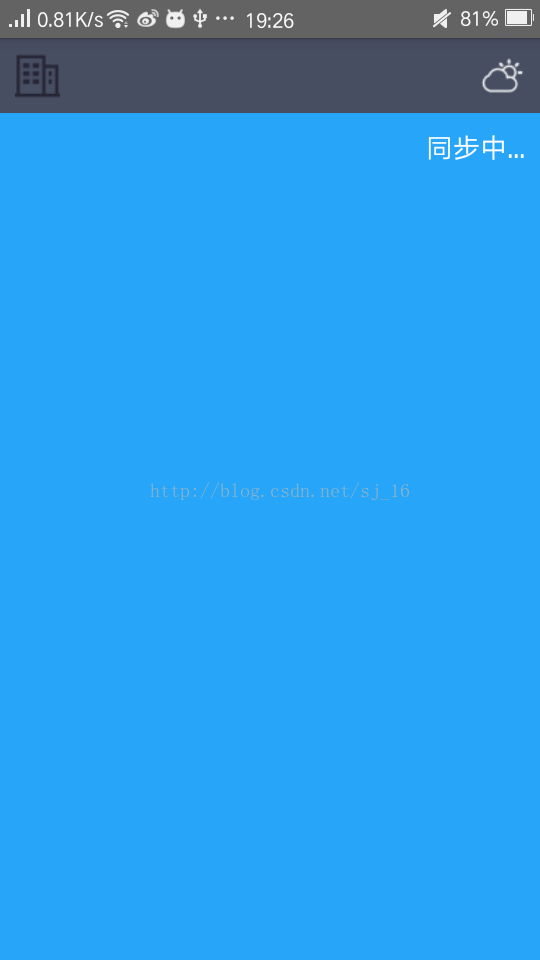
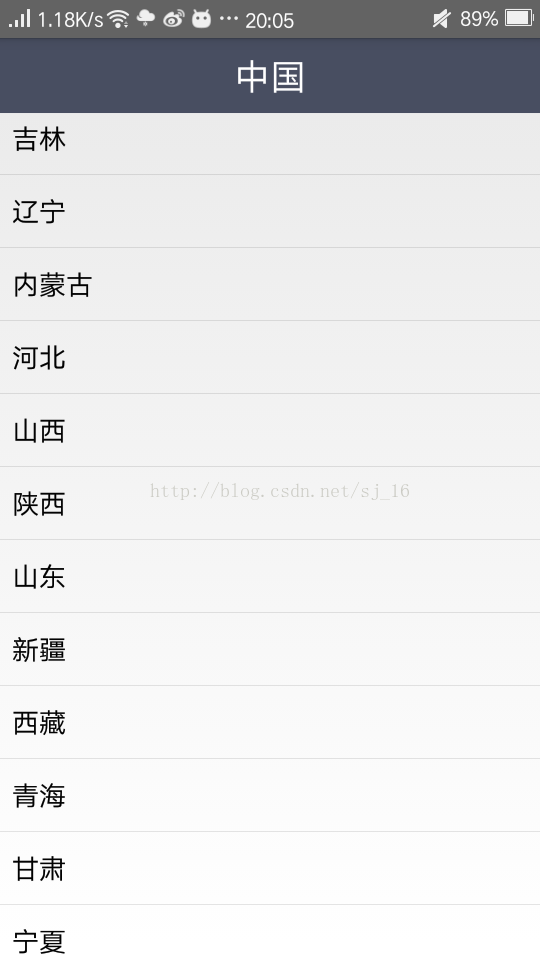
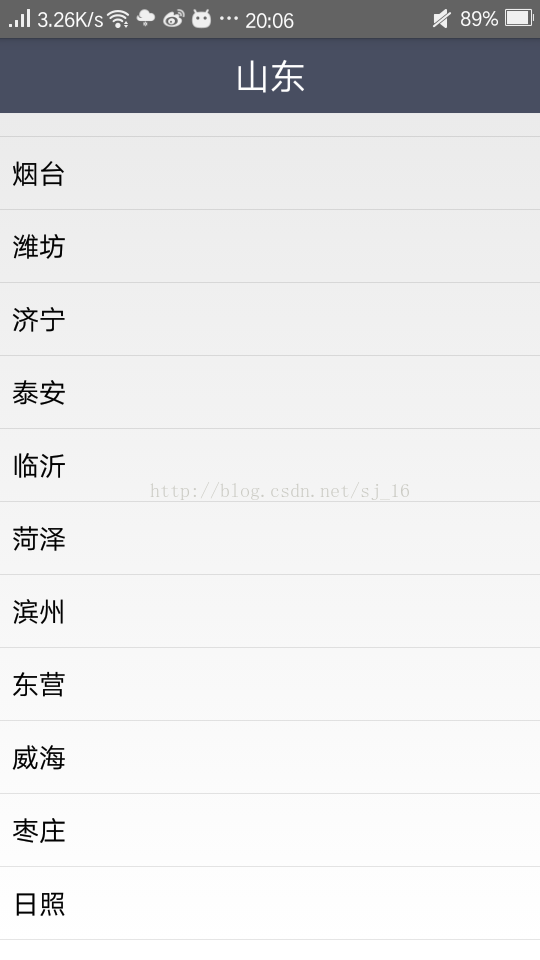
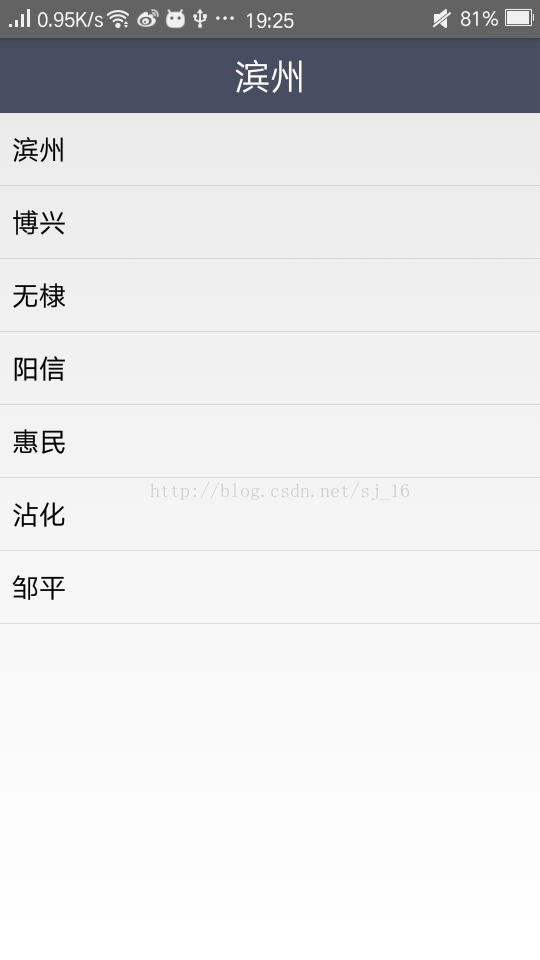
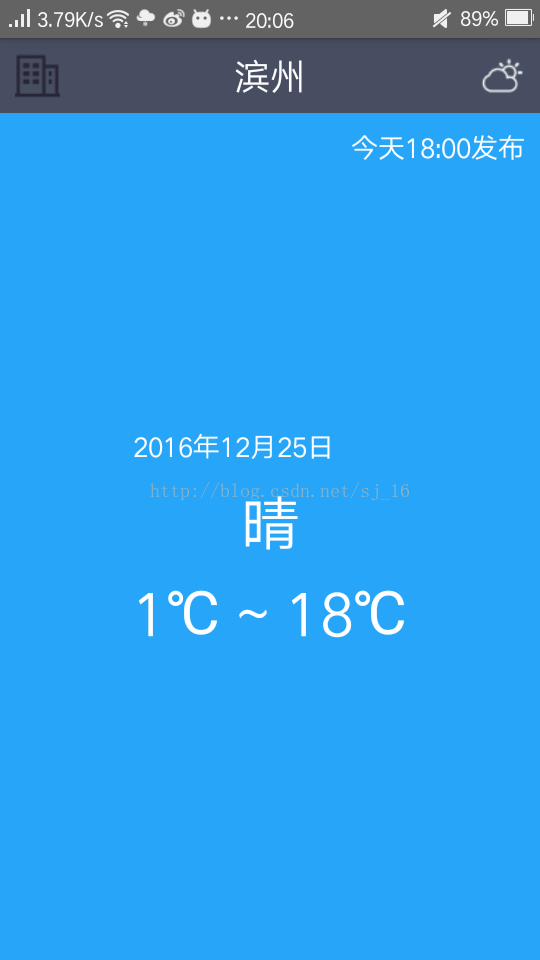
二、.java文件
WeatherOpenHelper.java
AutoUpdateReceiver.java
AutoUpdateService.java
三、.xml文件
choose_area.xml
AndroidManifest.xml
四、出现的问题:
一开始的地区选择运行正常,但是不能同步,一直出现“正在同步···”,经改正(我也不知道到底改了什么)出现了天气情况,但是不准确,明明0~4度,但是显示1~18度,好无奈。。。
二、.java文件
WeatherOpenHelper.java
package com.example.coolweather; import android.content.Context; import android.database.sqlite.SQLiteDatabase; import android.database.sqlite.SQLiteOpenHelper; import android.database.sqlite.SQLiteDatabase.CursorFactory; public class WeatherOpenHelper extends SQLiteOpenHelper{ //Province表 public static final String CREATE_PROVINCE = "create table Province (" + "id integer primary key autoincrement, " + "province_name text, " + "province_code text)"; //City表 public static final String CREATE_CITY = "create table City (" + "id integer primary key autoincrement, " + "city_name text, " + "city_code text, " + "province_id integer)"; //County表 public static final String CREATE_COUNTY = "create table County (" + "id integer primary key autoincrement, " + "county_name text, " + "county_code text, " + "city_id integer)"; public WeatherOpenHelper(Context context, String name, CursorFactory factory, int version) { super(context, name, factory, version); // TODO Auto-generated constructor stub } @Override public void onCreate(SQLiteDatabase db) { // TODO Auto-generated method stub db.execSQL(CREATE_PROVINCE); // 创建Province表 db.execSQL(CREATE_CITY); // 创建City表 db.execSQL(CREATE_COUNTY); // 创建County表 } @Override public void onUpgrade(SQLiteDatabase arg0, int arg1, int arg2) { // TODO Auto-generated method stub } }Province.java
package com.example.coolweather.model; public class Province { private int id; private String provinceName; private String provinceCode; public int getId(){ return id; } public void setId(int id) { this.id = id; } public String getProvinceName() { return provinceName; } public void setProvinceName(String provinceName) { this.provinceName = provinceName; } public String getProvinceCode() { return provinceCode; } public void setProvinceCode(String provinceCode) { this.provinceCode = provinceCode; } }City.java
package com.example.coolweather.model; public class City { private int id; private String cityName; private String cityCode; private int provinceId; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getCityName() { return cityName; } public void setCityName(String cityName) { this.cityName = cityName; } public String getCityCode() { return cityCode; } public void setCityCode(String cityCode) { this.cityCode = cityCode; } public int getProvinceId() { return provinceId; } public void setProvinceId(int provinceId) { this.provinceId = provinceId; } }County.java
package com.example.coolweather.model; public class County { private int id; private String countyName; private String countyCode; private int cityId; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getCountyName() { return countyName; } public void setCountyName(String countyName) { this.countyName = countyName; } public String getCountyCode() { return countyCode; } public void setCountyCode(String countyCode) { this.countyCode = countyCode; } public int getCityId() { return cityId; } public void setCityId(int cityId) { this.cityId = cityId; } }WeatherDB.java
package com.example.coolweather; import java.util.ArrayList; import java.util.List; import com.example.coolweather.model.City; import com.example.coolweather.model.County; import com.example.coolweather.model.Province; import android.content.ContentValues; import android.content.Context; import android.database.Cursor; import android.database.sqlite.SQLiteDatabase; public class WeatherDB { //数据库名 public static final String DB_NAME = "weather"; //数据库版本 public static final int VERSION = 1; private static WeatherDB weatherDB; private SQLiteDatabase db; //将构造方法私有化 private WeatherDB(Context context) { WeatherOpenHelper dbHelper = new WeatherOpenHelper(context, DB_NAME, null, VERSION); db = dbHelper.getWritableDatabase(); } //获取WeatherDB的实例 public synchronized static WeatherDB getInstance(Context context) { if (weatherDB == null) { weatherDB = new WeatherDB(context); } return weatherDB; } //将Province实例存储到数据库 public void saveProvince(Province province) { if (province != null) { ContentValues values = new ContentValues(); values.put("province_name", province.getProvinceName()); values.put("province_code", province.getProvinceCode()); db.insert("Province", null, values); } } //从数据库读取全国所有的省份信息 public List<Province> loadProvinces() { List<Province> list = new ArrayList<Province>(); Cursor cursor = db .query("Province", null, null, null, null, null, null); if (cursor.moveToFirst()) { do { Province province = new Province(); province.setId(cursor.getInt(cursor.getColumnIndex("id"))); province.setProvinceName(cursor.getString(cursor .getColumnIndex("province_name"))); province.setProvinceCode(cursor.getString(cursor .getColumnIndex("province_code"))); list.add(province); } while (cursor.moveToNext()); } return list; } //将City实例存储到数据库 public void saveCity(City city) { if (city != null) { ContentValues values = new ContentValues(); values.put("city_name", city.getCityName()); values.put("city_code", city.getCityCode()); values.put("province_id", city.getProvinceId()); db.insert("City", null, values); } } //从数据库读取某省下所有的城市信息 public List<City> loadCities(int provinceId) { List<City> list = new ArrayList<City>(); Cursor cursor = db.query("City", null, "province_id = ?", new String[] { String.valueOf(provinceId) }, null, null, null); if (cursor.moveToFirst()) { do { City city = new City(); city.setId(cursor.getInt(cursor.getColumnIndex("id"))); city.setCityName(cursor.getString(cursor .getColumnIndex("city_name"))); city.setCityCode(cursor.getString(cursor .getColumnIndex("city_code"))); city.setProvinceId(provinceId); list.add(city); } while (cursor.moveToNext()); } return list; } //将County实例存储到数据库 public void saveCounty(County county) { if (county != null) { ContentValues values = new ContentValues(); values.put("county_name", county.getCountyName()); values.put("county_code", county.getCountyCode()); values.put("city_id", county.getCityId()); db.insert("County", null, values); } } //从数据库读取某城市下所有的县信息 public List<County> loadCounties(int cityId) { List<County> list = new ArrayList<County>(); Cursor cursor = db.query("County", null, "city_id = ?", new String[] { String.valueOf(cityId) }, n 4000 ull, null, null); if (cursor.moveToFirst()) { do { County county = new County(); county.setId(cursor.getInt(cursor.getColumnIndex("id"))); county.setCountyName(cursor.getString(cursor .getColumnIndex("county_name"))); county.setCountyCode(cursor.getString(cursor .getColumnIndex("county_code"))); county.setCityId(cityId); list.add(county); } while (cursor.moveToNext()); } return list; } }HttpUtil.java
package com.example.coolweather.util; import java.io.BufferedReader; import java.io.InputStream; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; public class HttpUtil { public static void sendHttpRequest(final String address, final HttpCallbackListener listener) { new Thread(new Runnable() { @Override public void run() { HttpURLConnection connection = null; try { URL url = new URL(address); connection = (HttpURLConnection) url.openConnection(); connection.setRequestMethod("GET"); connection.setConnectTimeout(8000); connection.setReadTimeout(8000); InputStream in = connection.getInputStream(); BufferedReader reader = new BufferedReader(new InputStreamReader(in)); StringBuilder response = new StringBuilder(); String line; while ((line = reader.readLine()) != null) { response.append(line); } if (listener != null) { // 回调onFinish()方法 listener.onFinish(response.toString()); } } catch (Exception e) { if (listener != null) { // 回调onError()方法 listener.onError(e); } } finally { if (connection != null) { connection.disconnect(); } } } }).start(); } }HttpCallbackListener.java
package com.example.coolweather.util; public interface HttpCallbackListener { void onFinish(String response); void onError(Exception e); }Utility.java
package com.example.coolweather.util; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Locale; import org.json.JSONException; import org.json.JSONObject; import com.example.coolweather.WeatherDB; import com.example.coolweather.model.City; import com.example.coolweather.model.County; import com.example.coolweather.model.Province; import android.content.Context; import android.content.SharedPreferences; import android.preference.PreferenceManager; import android.text.TextUtils; public class Utility { //解析和处理服务器返回的省级数据 public synchronized static boolean handleProvincesResponse(WeatherDB weatherDB, String response) { if (!TextUtils.isEmpty(response)) { String[] allProvinces = response.split(","); if (allProvinces != null && allProvinces.length > 0) { for (String p : allProvinces) { String[] array = p.split("\\|"); Province province = new Province(); province.setProvinceCode(array[0]); province.setProvinceName(array[1]); // 将解析出来的数据存储到Province表 weatherDB.saveProvince(province); } return true; } } return false; } //解析和处理服务器返回的市级数据 public static boolean handleCitiesResponse(WeatherDB weatherDB, String response, int provinceId) { if (!TextUtils.isEmpty(response)) { String[] allCities = response.split(","); if (allCities != null && allCities.length > 0) { for (String c : allCities) { String[] array = c.split("\\|"); City city = new City(); city.setCityCode(array[0]); city.setCityName(array[1]); city.setProvinceId(provinceId); // 将解析出来的数据存储到City表 weatherDB.saveCity(city); } return true; } } return false; } //解析和处理服务器返回的县级数据 public static boolean handleCountiesResponse(WeatherDB weatherDB, String response, int cityId) { if (!TextUtils.isEmpty(response)) { String[] allCounties = response.split(","); if (allCounties != null && allCounties.length > 0) { for (String c : allCounties) { String[] array = c.split("\\|"); County county = new County(); county.setCountyCode(array[0]); county.setCountyName(array[1]); county.setCityId(cityId); // 将解析出来的数据存储到County表 weatherDB.saveCounty(county); } return true; } } return false; } //解析服务器返回的JSON数据,并将解析出的数据存储到本地 public static void handleWeatherResponse(Context context, String response) { try { JSONObject jsonObject = new JSONObject(response); JSONObject weatherInfo = jsonObject.getJSONObject("weatherinfo"); String cityName = weatherInfo.getString("city"); String weatherCode = weatherInfo.getString("cityid"); String temp1 = weatherInfo.getString("temp1"); String temp2 = weatherInfo.getString("temp2"); String weatherDesp = weatherInfo.getString("weather"); String publishTime = weatherInfo.getString("ptime"); saveWeatherInfo(context, cityName, weatherCode, temp1, temp2, weatherDesp, publishTime); } catch (JSONException e) { e.printStackTrace(); } } //将服务器返回的所有天气信息存储到SharedPreferences文件中 public static void saveWeatherInfo(Context context, String cityName, String weatherCode, String temp1, String temp2, String weatherDesp, String publishTime) { SimpleDateFormat sdf = new SimpleDateFormat("yyyy年M月d日", Locale.CHINA); SharedPreferences.Editor editor = PreferenceManager .getDefaultSharedPreferences(context).edit(); editor.putBoolean("city_selected", true); editor.putString("city_name", cityName); editor.putString("weather_code", weatherCode); editor.putString("temp1", temp1); editor.putString("temp2", temp2); editor.putString("weather_desp", weatherDesp); editor.putString("publish_time", publishTime); editor.putString("current_date", sdf.format(new Date())); editor.commit(); } }ChooseAreaActivity.java
package com.example.coolweather; import java.util.ArrayList; import java.util.List; import com.example.coolweather.model.City; import com.example.coolweather.model.County; import com.example.coolweather.model.Province; import com.example.coolweather.util.HttpCallbackListener; import com.example.coolweather.util.HttpUtil; import com.example.coolweather.util.Utility; import android.app.Activity; import android.app.ProgressDialog; import android.content.Intent; import android.content.SharedPreferences; import android.os.Bundle; import android.preference.PreferenceManager; import android.text.TextUtils; import android.view.Window; import android.view.View; import android.widget.AdapterView; import android.widget.AdapterView.OnItemClickListener; import android.widget.ArrayAdapter; import android.widget.ListView; import android.widget.TextView; import android.widget.Toast; public class ChooseAreaActivity extends Activity { public static final int LEVEL_PROVINCE = 0; public static final int LEVEL_CITY = 1; public static final int LEVEL_COUNTY = 2; private ProgressDialog progressDialog; private TextView titleText; private ListView listView; private ArrayAdapter<String> adapter; private WeatherDB weatherDB; private List<String> dataList = new ArrayList<String>(); private List<Province> provinceList;// 省列表 private List<City> cityList;// 市列表 private List<County> countyList;// 县列表 private Province selectedProvince;// 选中的省份 private City selectedCity;// 选中的城市 private int currentLevel;// 当前选中的级别 private boolean isFromWeatherActivity;// 是否从WeatherActivity中跳转过来 @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); isFromWeatherActivity = getIntent().getBooleanExtra( "from_weather_ activity", false); SharedPreferences prefs = PreferenceManager .getDefaultSharedPreferences(this); if (prefs.getBoolean("city_selected", false) && !isFromWeatherActivity) { Intent intent = new Intent(this, WeatherActivity.class); startActivity(intent); finish(); return; } requestWindowFeature(Window.FEATURE_NO_TITLE); setContentView(R.layout.choose_area); listView = (ListView) findViewById(R.id.list_view); titleText = (TextView) findViewById(R.id.title_text); adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, dataList); listView.setAdapter(adapter); weatherDB = WeatherDB.getInstance(this); listView.setOnItemClickListener(new OnItemClickListener() { @Override public void onItemClick(AdapterView<?> arg0, View view, int index, long arg3) { if (currentLevel == LEVEL_PROVINCE) { selectedProvince = provinceList.get(index); queryCities(); } else if (currentLevel == LEVEL_CITY) { selectedCity = cityList.get(index); queryCounties(); } else if (currentLevel == LEVEL_COUNTY) { String countyCode = countyList.get(index).getCountyCode(); Intent intent = new Intent(ChooseAreaActivity.this, WeatherActivity.class); intent.putExtra("county_code", countyCode); startActivity(intent); finish(); } } }); queryProvinces(); // 加载省级数据 } // 查询全国所有的省,优先从数据库查询,如果没有查询到再去服务器上查询 private void queryProvinces() { provinceList = weatherDB.loadProvinces(); if (provinceList.size() > 0) { dataList.clear(); for (Province province : provinceList) { dataList.add(province.getProvinceName()); } adapter.notifyDataSetChanged(); listView.setSelection(0); titleText.setText("中国"); currentLevel = LEVEL_PROVINCE; } else { queryFromServer(null, "province"); } } // 查询选中省内所有的市,优先从数据库查询,如果没有查询到再去服务器上查询 private void queryCities() { cityList = weatherDB.loadCities(selectedProvince.getId()); if (cityList.size() > 0) { dataList.clear(); for (City city : cityList) { dataList.add(city.getCityName()); } adapter.notifyDataSetChanged(); listView.setSelection(0); titleText.setText(selectedProvince.getProvinceName()); currentLevel = LEVEL_CITY; } else { queryFromServer(selectedProvince.getProvinceCode(), "city"); } } // 查询选中市内所有的县,优先从数据库查询,如果没有查询到再去服务器上查询 private void queryCounties() { countyList = weatherDB.loadCounties(selectedCity.getId()); if (countyList.size() > 0) { dataList.clear(); for (County county : countyList) { dataList.add(county.getCountyName()); } adapter.notifyDataSetChanged(); listView.setSelection(0); titleText.setText(selectedCity.getCityName()); currentLevel = LEVEL_COUNTY; } else { queryFromServer(selectedCity.getCityCode(), "county"); } } // 根据传入的代号和类型从服务器上查询省市县数据 private void queryFromServer(final String code, final String type) { String address; if (!TextUtils.isEmpty(code)) { address = "http://www.weather.com.cn/data/list3/city" + code + ".xml"; } else { address = "http://www.weather.com.cn/data/list3/city.xml"; } showProgressDialog(); HttpUtil.sendHttpRequest(address, new HttpCallbackListener() { @Override public void onFinish(String response) { boolean result = false; if ("province".equals(type)) { result = Utility.handleProvincesResponse(weatherDB, response); } else if ("city".equals(type)) { result = Utility.handleCitiesResponse(weatherDB, response, selectedProvince.getId()); } else if ("county".equals(type)) { result = Utility.handleCountiesResponse(weatherDB, response, selectedCity.getId()); } if (result) { // 通过runOnUiThread()方法回到主线程处理逻辑 runOnUiThread(new Runnable() { @Override public void run() { closeProgressDialog(); if ("province".equals(type)) { queryProvinces(); } else if ("city".equals(type)) { queryCities(); } else if ("county".equals(type)) { queryCounties(); } } }); } } @Override public void onError(Exception e) { // 通过runOnUiThread()方法回到主线程处理逻辑 runOnUiThread(new Runnable() { @Override public void run() { closeProgressDialog(); Toast.makeText(ChooseAreaActivity.this, "加载失败", Toast.LENGTH_SHORT).show(); } }); } }); } // 显示进度对话框 private void showProgressDialog() { if (progressDialog == null) { progressDialog = new ProgressDialog(this); progressDialog.setMessage("正在加载..."); progressDialog.setCanceledOnTouchOutside(false); } progressDialog.show(); } // 关闭进度对话框 private void closeProgressDialog() { if (progressDialog != null) { progressDialog.dismiss(); } } // 捕获Back按键,根据当前的级别来判断,此时应该返回市列表、省列表、还是直接退出 @Override public void onBackPressed() { if (currentLevel == LEVEL_COUNTY) { queryCities(); } else if (currentLevel == LEVEL_CITY) { queryProvinces(); } else { if (isFromWeatherActivity) { Intent intent = new Intent(this, WeatherActivity.class); startActivity(intent); } finish(); } } }WeatherActivity.java
package com.example.coolweather; import com.example.coolweather.service.AutoUpdateService; import com.example.coolweather.util.HttpCallbackListener; import com.example.coolweather.util.HttpUtil; import com.example.coolweather.util.Utility; import android.app.Activity; import android.content.Intent; import android.content.SharedPreferences; import android.os.Bundle; import android.preference.PreferenceManager; import android.text.TextUtils; import android.view.View; import android.view.Window; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.LinearLayout; import android.widget.TextView; public class WeatherActivity extends Activity implements OnClickListener { private LinearLayout weatherInfoLayout; private TextView cityNameText;// 用于显示城市名 private TextView publishText;// 用于显示发布时间 private TextView weatherDespText;// 用于显示天气描述信息 private TextView temp1Text;// 用于显示气温1 private TextView temp2Text;// 用于显示气温2 private TextView currentDateText;// 用于显示当前日期 private Button switchCity;// 切换城市按钮 private Button refreshWeather;// 更新天气按钮 @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); requestWindowFeature(Window.FEATURE_NO_TITLE); setContentView(R.layout.weather_layout); // 初始化各控件 weatherInfoLayout = (LinearLayout) findViewById(R.id.weather_info_layout); cityNameText = (TextView) findViewById(R.id.city_name); publishText = (TextView) findViewById(R.id.publish_text); weatherDespText = (TextView) findViewById(R.id.weather_desp); temp1Text = (TextView) findViewById(R.id.temp1); temp2Text = (TextView) findViewById(R.id.temp2); currentDateText = (TextView) findViewById(R.id.current_date); switchCity = (Button) findViewById(R.id.switch_city); refreshWeather = (Button) findViewById(R.id.refresh_weather); String countyCode = getIntent().getStringExtra("county_code"); if (!TextUtils.isEmpty(countyCode)) { // 有县级代号时就去查询天气 publishText.setText("同步中..."); weatherInfoLayout.setVisibility(View.INVISIBLE); cityNameText.setVisibility(View.INVISIBLE); queryWeatherCode(countyCode); } else { // 没有县级代号时就直接显示本地天气 showWeather(); } switchCity.setOnClickListener(this); refreshWeather.setOnClickListener(this); } @Override public void onClick(View v) { // TODO Auto-generated method stub switch (v.getId()) { case R.id.switch_city: Intent intent = new Intent(this, ChooseAreaActivity.class); intent.putExtra("from_weather_activity c734 ", true); startActivity(intent); finish(); break; case R.id.refresh_weather: publishText.setText("同步中..."); SharedPreferences prefs = PreferenceManager. getDefaultSharedPreferences(this); String weatherCode = prefs.getString("weather_code", ""); if (!TextUtils.isEmpty(weatherCode)) { queryWeatherInfo(weatherCode); } break; default: break; } } // 查询县级代号所对应的天气代号。 private void queryWeatherCode(String countyCode) { String address = "http://www.weather.com.cn/data/list3/city" + countyCode + ".xml"; queryFromServer(address, "countyCode"); } //查询天气代号所对应的天气。 private void queryWeatherInfo(String weatherCode) { String address = "http://www.weather.com.cn/data/cityinfo/" + weatherCode + ".html"; queryFromServer(address, "weatherCode"); } //根据传入的地址和类型去向服务器查询天气代号或者天气信息。 private void queryFromServer(final String address, final String type) { HttpUtil.sendHttpRequest(address, new HttpCallbackListener() { public void onFinish(final String response) { if ("countyCode".equals(type)) { if (!TextUtils.isEmpty(response)) { // 从服务器返回的数据中解析出天气代号 String[] array = response.split("\\|"); if (array != null && array.length == 2) { String weatherCode = array[1]; queryWeatherInfo(weatherCode); } } } else if ("weatherCode".equals(type)) { // 处理服务器返回的天气信息 Utility.handleWeatherResponse(WeatherActivity.this, response); runOnUiThread(new Runnable() { @Override public void run() { showWeather(); } }); } } public void onError(Exception e) { runOnUiThread(new Runnable() { @Override public void run() { publishText.setText("同步失败"); } }); } }); } //从SharedPreferences文件中读取存储的天气信息,并显示到界面上。 private void showWeather() { SharedPreferences prefs = PreferenceManager .getDefaultSharedPreferences(this); cityNameText.setText(prefs.getString("city_name", "")); temp1Text.setText(prefs.getString("temp1", "")); temp2Text.setText(prefs.getString("temp2", "")); weatherDespText.setText(prefs.getString("weather_desp", "")); publishText.setText("今天" + prefs.getString("publish_time", "") + "发布"); currentDateText.setText(prefs.getString("current_date", "")); weatherInfoLayout.setVisibility(View.VISIBLE); cityNameText.setVisibility(View.VISIBLE); Intent intent = new Intent(this, AutoUpdateService.class); startService(intent); } }
AutoUpdateReceiver.java
package com.example.coolweather.receive; import com.example.coolweather.service.AutoUpdateService; import android.content.BroadcastReceiver; import android.content.Context; import android.content.Intent; public class AutoUpdateReceiver extends BroadcastReceiver { @Override public void onReceive(Context context, Intent arg1) { // TODO Auto-generated method stub Intent i = new Intent(context, AutoUpdateService.class); context.startService(i); } }
AutoUpdateService.java
package com.example.coolweather.service; import com.example.coolweather.receive.AutoUpdateReceiver; import com.example.coolweather.util.HttpCallbackListener; import com.example.coolweather.util.HttpUtil; import com.example.coolweather.util.Utility; import android.app.AlarmManager; import android.app.PendingIntent; import android.app.Service; import android.content.Intent; import android.content.SharedPreferences; import android.os.IBinder; import android.os.SystemClock; import android.preference.PreferenceManager; public class AutoUpdateService extends Service { @Override public IBinder onBind(Intent arg0) { // TODO Auto-generated method stub return null; } @Override public int onStartCommand(Intent intent, int flags, int startId) { new Thread(new Runnable() { @Override public void run() { updateWeather(); } }).start(); AlarmManager manager = (AlarmManager) getSystemService(ALARM_SERVICE); int anHour = 8 * 60 * 60 * 1000; // 这是8小时的毫秒数 long triggerAtTime = SystemClock.elapsedRealtime() + anHour; Intent i = new Intent(this, AutoUpdateReceiver.class); PendingIntent pi = PendingIntent.getBroadcast(this, 0, i, 0); manager.set(AlarmManager.ELAPSED_REALTIME_WAKEUP, triggerAtTime, pi); return super.onStartCommand(intent, flags, startId); } /** * 更新天气信息。 */ private void updateWeather() { SharedPreferences prefs = PreferenceManager. getDefaultSharedPreferences(this); String weatherCode = prefs.getString("weather_code", ""); String address = "http://www.weather.com.cn/data/cityinfo/" + weatherCode + ".html"; HttpUtil.sendHttpRequest(address, new HttpCallbackListener() { @Override public void onFinish(String response) { Utility.handleWeatherResponse(AutoUpdateService.this, response); } @Override public void onError(Exception e) { e.printStackTrace(); } }); } }
三、.xml文件
choose_area.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" > <RelativeLayout android:layout_width="match_parent" android:layout_height="50dp" android:background="#484E61" > <TextView android:id="@+id/title_text" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerInParent="true" android:textColor="#fff" android:textSize="24sp" /> </RelativeLayout> <ListView android:id="@+id/list_view" android:layout_width="match_parent" android:layout_height="match_parent" > </ListView> </LinearLayout>weatherlayout.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" > <RelativeLayout android:layout_width="match_parent" android:layout_height="50dp" android:background="#484E61" > <Button android:id="@+id/switch_city" android:layout_width="30dp" android:layout_height="30dp" android:layout_centerVertical="true" android:layout_marginLeft="10dp" android:background="@drawable/icons_weather_city" /> <TextView android:id="@+id/city_name" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerInParent="true" android:textColor="#fff" android:textSize="24sp" /> <Button android:id="@+id/refresh_weather" android:layout_width="30dp" android:layout_height="30dp" android:layout_alignParentRight="true" android:layout_centerVertical="true" android:layout_marginRight="10dp" android:background="@drawable/icons_menu_weather" /> </RelativeLayout> <RelativeLayout android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="1" android:background="#27A5F9" > <TextView android:id="@+id/publish_text" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentRight="true" android:layout_marginRight="10dp" android:layout_marginTop="10dp" android:textColor="#FFF" android:textSize="18sp" /> <LinearLayout android:id="@+id/weather_info_layout" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerInParent="true" android:orientation="vertical" > <TextView android:id="@+id/current_date" android:layout_width="wrap_content" android:layout_height="40dp" android:gravity="center" android:textColor="#FFF" android:textSize="18sp" /> <TextView android:id="@+id/weather_desp" android:layout_width="wrap_content" android:layout_height="60dp" android:layout_gravity="center_horizontal" android:gravity="center" android:textColor="#FFF" android:textSize="40sp" /> <LinearLayout android:layout_width="wrap_content" android:layout_height="60dp" android:layout_gravity="center_horizontal" android:orientation="horizontal" > <TextView android:id="@+id/temp1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:textColor="#FFF" android:textSize="40sp" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:layout_marginLeft="10dp" android:layout_marginRight="10dp" android:text="~" android:textColor="#FFF" android:textSize="40sp" /> <TextView android:id="@+id/temp2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:textColor="#FFF" android:textSize="40sp" /> </LinearLayout> </LinearLayout> </RelativeLayout> </LinearLayout>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.coolweather" android:versionCode="1" android:versionName="1.0" > <uses-sdk android:minSdkVersion="8" android:targetSdkVersion="20" /> <uses-permission android:name="android.permission.INTERNET" /> <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name="com.example.coolweather.ChooseAreaActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name="com.example.coolweather.WeatherActivity" android:label="@string/app_name" > </activity> <service android:name="com.example.coolweather.service.AutoUpdateService"></service> <receiver android:name="com.example.coolweather.receiver.AutoUpdateReceiver"></receiver> </application> </manifest>
四、出现的问题:
一开始的地区选择运行正常,但是不能同步,一直出现“正在同步···”,经改正(我也不知道到底改了什么)出现了天气情况,但是不准确,明明0~4度,但是显示1~18度,好无奈。。。
相关文章推荐
- An Example of Pre-Query and Post-Query Triggers in Oracle Forms With Using Display_Item to Highlight Dynamically
- color 和 mode
- 重温Spring之旅3——装配Bean(手工装配:使用xml的方式)
- 从一个字符数组中读出相应的整数、实数 例如:char cStringArray[10] = {1,2.3,45.6,7.89}
- Date&String时间转换工具类
- 网路故障排除笔记
- 通知
- Centos7下安装配置mysql
- 初学者如何学习人工智能收藏
- 重温Spring之旅2——实现自己的Spring机制
- Hibernate-关联映射
- Flume实时抽取监控目录数据
- Activity基本概念
- POJ 2032 Square Carpets 笔记
- POJ 3061 (尺取法,二分法的应用)
- 神马玩意,EntityFramework Core 1.1又更新了?走,赶紧去围观
- bzoj 3702: 二叉树 (线段树)
- 指定一个文件夹,然后该文件夹下面说有的java文件
- 通过Spring @PostConstruct 和 @PreDestroy 方法 实现初始化和销毁bean之前进行的操作
- WM_PAINT(父子窗口间)