FCM(GCM)android消息推送
2016-12-19 09:55
585 查看
FCM(GCM)
1. 进入Firebase的控制台添加我们的项目(firebaseProject)(https://console.firebase.google.com/)
按照官方引导操作就可以了。
如果使用的是AS 2.3以上版本可以按照如下图的步骤进行FirebaseProject的配置(需要测试设备安装了Google play的商店应用或者Google API的模拟器)
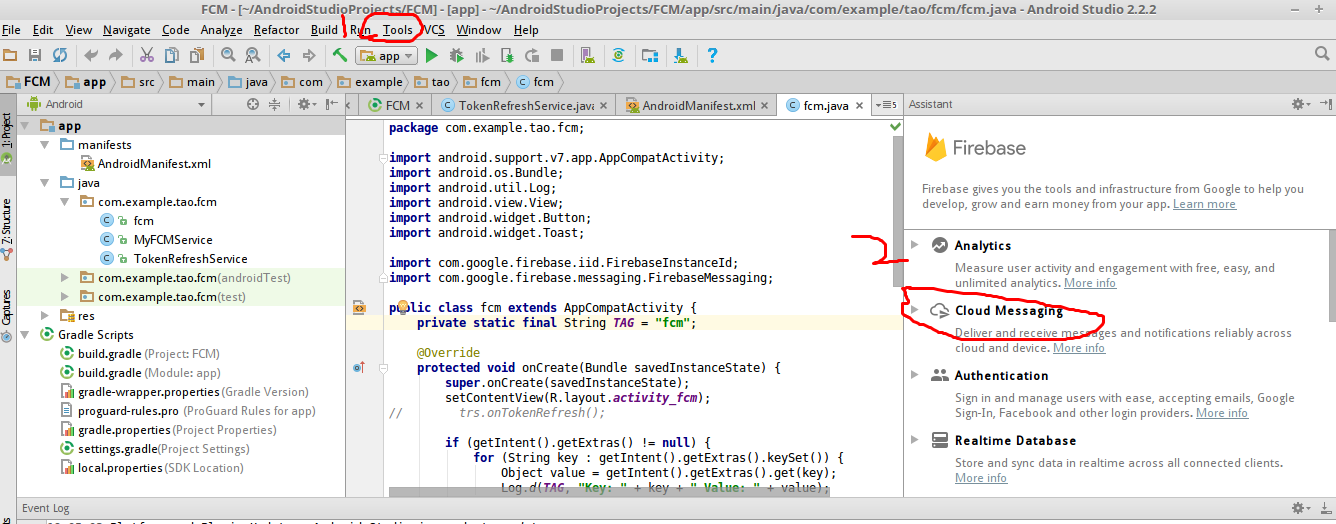
2. 如果是通过AS的引导进行配置项目的话一下步骤可以跳过(AS会自动帮我们配置完成了)
在应用级的build.gradle中添加对FCM的依赖
在dependencies{ ... }中添加
同时在此文件中的最后一行添加
3. 环境部署完之后,现在开始配置我们的项目
按照官方文档上面:
一项可以扩展
一项可以扩展
如果FCM对于 Android 应用的功能至关重要,应确保在清单中设置
Android 应用无法安装在其不能正常运行的环境中
3.1扩展 FirebaseMessagingService:
在我们的项目中new service继承FirebaseMessagingService
重写onMessageReceived方法,和发送通知的方法,完整如下
3.2 扩展FirebaseInstanceIdService
同样创建一个service继承FirebaseInstanceIdService,该service主要是获取目标设备的token,用于定点发送通知
4. 写一下我们的Activity
到目前为止我们的FCM example已经写完了,现在我们看一下测试结果
5. 通过firebase console 进行发送推送通知
第一个是对所有安装过程序的client端进行发送推送
第三个是对指定的Client进行发送,他会需要一个Token值,在我们程序跑起来时,会有一个Token值,获得后出进去就可以了
第二个还没用
第二种方法通过向firebase server发送API再由firebase server发送给Client
我们这边使用的是Http 的方式进行发送,简单一点直接使用postman进行测试,后期可以加入到App server中去

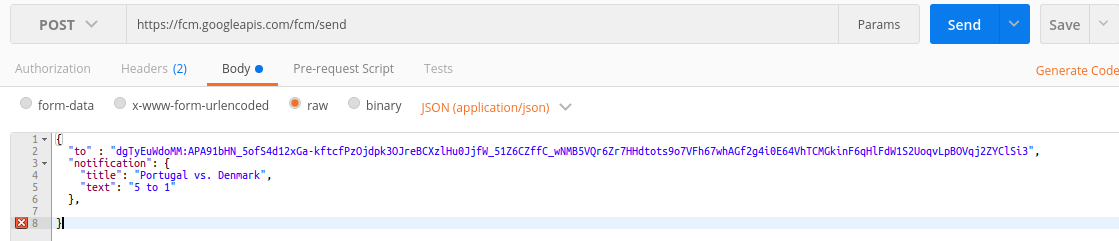
这是我们发送的API
其中Authorization 中key的值就是firebase 中提供的key
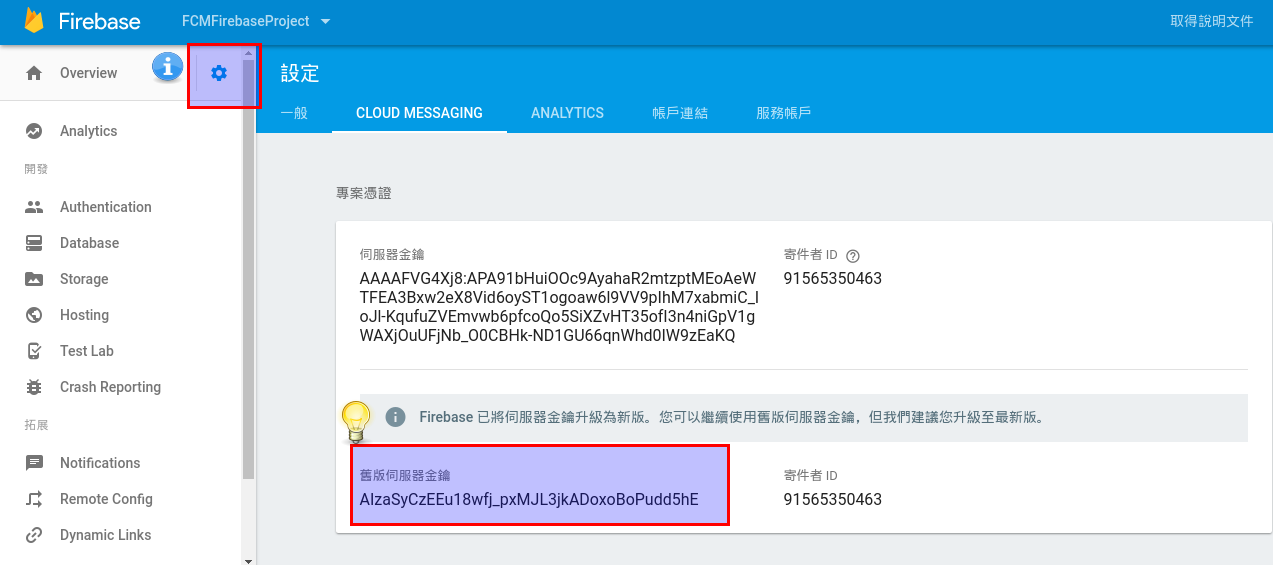
点击左上角设定按钮可以看到我们的秘钥了
在json里面“to”的值就是我们
8e85
Client获得的token
我用的模拟器测试的都可以收到通知。
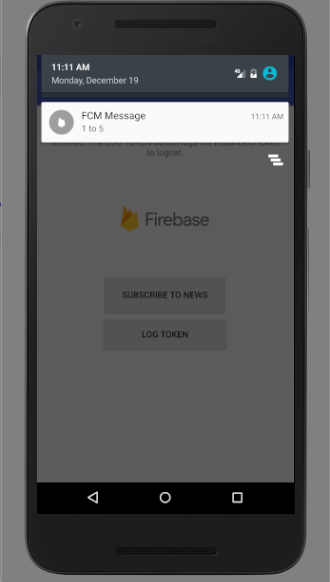
目前通过发送API的方式只测过对单一设备进行发送,还没有对群设备进行发送。
1. 进入Firebase的控制台添加我们的项目(firebaseProject)(https://console.firebase.google.com/)
按照官方引导操作就可以了。
如果使用的是AS 2.3以上版本可以按照如下图的步骤进行FirebaseProject的配置(需要测试设备安装了Google play的商店应用或者Google API的模拟器)
2. 如果是通过AS的引导进行配置项目的话一下步骤可以跳过(AS会自动帮我们配置完成了)
在应用级的build.gradle中添加对FCM的依赖
在dependencies{ ... }中添加
compile 'com.google.firebase:firebase-messaging:10.0.1'
同时在此文件中的最后一行添加
apply plugin: 'com.google.gms.google-services'
3. 环境部署完之后,现在开始配置我们的项目
按照官方文档上面:
一项可以扩展
FirebaseMessagingService的服务。如果您希望在后台进行接收应用通知之外的任何消息处理,则必须添加此服务。要在前台应用中接收通知、接收数据负载以及发送上游消息等,您必须扩展此服务。
一项可以扩展
FirebaseInstanceIdService的服务,用于处理注册令牌的创建、轮转和更新。如果要发送至特定设备或者创建设备群组,则必须添加此服务。
如果FCM对于 Android 应用的功能至关重要,应确保在清单中设置
android:minSdkVersion="8"或更高版本。这可确保
Android 应用无法安装在其不能正常运行的环境中
3.1扩展 FirebaseMessagingService:
在我们的项目中new service继承FirebaseMessagingService
重写onMessageReceived方法,和发送通知的方法,完整如下
package com.example.tao.fcm; import android.app.NotificationManager; import android.app.PendingIntent; import android.content.Intent; import android.media.RingtoneManager; import android.net.Uri; import android.support.v4.app.NotificationCompat; import android.util.Log; import android.content.Context; import com.google.firebase.messaging.FirebaseMessagingService; import com.google.firebase.messaging.RemoteMessage; public class MyFCMService extends FirebaseMessagingService { private static final String TAG = "MyFCMService"; public MyFCMService() { } @Override public void onMessageReceived(RemoteMessage remoteMessage) { // [START_EXCLUDE] // There are two types of messages data messages and notification messages. Data messages are handled // here in onMessageReceived whether the app is in the foreground or background. Data messages are the type // traditionally used with GCM. Notification messages are only received here in onMessageReceived when the app // is in the foreground. When the app is in the background an automatically generated notification is displayed. // When the user taps on the notification they are returned to the app. Messages containing both notification // and data payloads are treated as notification messages. The Firebase console always sends notification // messages. For more see: https://firebase.google.com/docs/cloud-messaging/concept-options // [END_EXCLUDE] // TODO(developer): Handle FCM messages here. // Not getting messages here? See why this may be: https://goo.gl/39bRNJ Log.d(TAG, "From: " + remoteMessage.getFrom()); // Check if message contains a data payload. if (remoteMessage.getData().size() > 0) { Log.d(TAG, "Message data payload: " + remoteMessage.getData()); } // Check if message contains a notification payload. if (remoteMessage.getNotification() != null) { Log.d(TAG, "Message Notification Body: " + remoteMessage.getNotification().getBody()); } sendNotification(remoteMessage.getNotification().getBody()); // Also if you intend on generating your own notifications as a result of a received FCM // message, here is where that should be initiated. See sendNotification method below. } private void sendNotification(String messageBody) { Intent intent = new Intent(this, fcm.class); intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP); PendingIntent pendingIntent = PendingIntent.getActivity(this, 0 /* Request code */, intent, PendingIntent.FLAG_ONE_SHOT); Uri defaultSoundUri= RingtoneManager.getDefaultUri(RingtoneManager.TYPE_NOTIFICATION); NotificationCompat.Builder notificationBuilder = new NotificationCompat.Builder(this) .setSmallIcon(R.drawable.ic_stat_ic_notification) .setContentTitle("FCM Message") .setContentText(messageBody) .setAutoCancel(true) .setSound(defaultSoundUri) .setContentIntent(pendingIntent); NotificationManager notificationManager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE); notificationManager.notify(0 /* ID of notification */, notificationBuilder.build()); } }
3.2 扩展FirebaseInstanceIdService
同样创建一个service继承FirebaseInstanceIdService,该service主要是获取目标设备的token,用于定点发送通知
package com.example.tao.fcm; import android.util.Log; import com.google.firebase.iid.FirebaseInstanceId; import com.google.firebase.iid.FirebaseInstanceIdService; /** * Created by tao on 16-12-13. */ public class TokenRefreshService extends FirebaseInstanceIdService { private static final String TAG = "TokenRefreshService"; @Override public void onTokenRefresh() { // Get updated InstanceID token. String refreshedToken = FirebaseInstanceId.getInstance().getToken(); Log.d(TAG, "Refreshed token: " + refreshedToken); // If you want to send messages to this application instance or // manage this apps subscriptions on the server side, send the // Instance ID token to your app server. sendRegistrationToServer(refreshedToken); } private void sendRegistrationToServer(String token) { // TODO: Implement this method to send token to your app server. } } 最后在manifests.xml中配置下我们的两个service
<service android:name=".MyFCMService"> <intent-filter> <action android:name="com.google.firebase.MESSAGING_EVENT" /> </intent-filter> </service> <service android:name=".TokenRefreshService"> <intent-filter> <action android:name="com.google.firebase.INSTANCE_ID_EVENT" /> </intent-filter> </service>
4. 写一下我们的Activity
package com.example.tao.fcm; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.Button; import android.widget.Toast; import com.google.firebase.iid.FirebaseInstanceId; import com.google.firebase.messaging.FirebaseMessaging; public class fcm extends AppCompatActivity { private static final String TAG = "fcm"; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_fcm); // trs.onTokenRefresh(); if (getIntent().getExtras() != null) { for (String key : getIntent().getExtras().keySet()) { Object value = getIntent().getExtras().get(key); Log.d(TAG, "Key: " + key + " Value: " + value); } } // [END handle_data_extras] Button subscribeButton = (Button) findViewById(R.id.subscribeButton); subscribeButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // [START subscribe_topics] FirebaseMessaging.getInstance().subscribeToTopic("news"); // [END subscribe_topics] // Log and toast String msg = getString(R.string.msg_subscribed); Log.d(TAG, msg); Toast.makeText(fcm.this, msg, Toast.LENGTH_SHORT).show(); } }); Button logTokenButton = (Button) findViewById(R.id.logTokenButton); logTokenButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // Get token String token = FirebaseInstanceId.getInstance().getToken(); // Log and toast String msg = getString(R.string.msg_token_fmt, token); Log.d(TAG, msg); Toast.makeText(fcm.this, msg, Toast.LENGTH_SHORT).show(); } }); } }
到目前为止我们的FCM example已经写完了,现在我们看一下测试结果
5. 通过firebase console 进行发送推送通知
第一个是对所有安装过程序的client端进行发送推送
第三个是对指定的Client进行发送,他会需要一个Token值,在我们程序跑起来时,会有一个Token值,获得后出进去就可以了
第二个还没用
第二种方法通过向firebase server发送API再由firebase server发送给Client
我们这边使用的是Http 的方式进行发送,简单一点直接使用postman进行测试,后期可以加入到App server中去
这是我们发送的API
其中Authorization 中key的值就是firebase 中提供的key
点击左上角设定按钮可以看到我们的秘钥了
在json里面“to”的值就是我们
8e85
Client获得的token
我用的模拟器测试的都可以收到通知。
目前通过发送API的方式只测过对单一设备进行发送,还没有对群设备进行发送。
相关文章推荐
- 使用C++实现JNI接口需要注意的事项
- Android IPC进程间通讯机制
- Android Manifest 用法
- [转载]Activity中ConfigChanges属性的用法
- Android之获取手机上的图片和视频缩略图thumbnails
- Android之使用Http协议实现文件上传功能
- Android学习笔记(二九):嵌入浏览器
- android string.xml文件中的整型和string型代替
- i-jetty环境搭配与编译
- android之定时器AlarmManager
- android wifi 无线调试
- Android Native 绘图方法
- Android java 与 javascript互访(相互调用)的方法例子
- android 代码实现控件之间的间距
- android FragmentPagerAdapter的“标准”配置
- Android"解决"onTouch和onClick的冲突问题
- android:installLocation简析
- android searchView的关闭事件